
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_hal_timer.h
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef ARM_HAL_TIMER_H_ 00017 #define ARM_HAL_TIMER_H_ 00018 00019 #include "eventloop_config.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #ifndef NS_EXCLUDE_HIGHRES_TIMER 00026 /** 00027 * \brief This function perform timer init. 00028 */ 00029 extern void platform_timer_enable(void); 00030 00031 /** 00032 * \brief This function is API for set Timer interrupt handler for stack 00033 * 00034 * \param new_fp Function pointer for stack giving timer handler 00035 * 00036 */ 00037 typedef void (*platform_timer_cb)(void); 00038 extern void platform_timer_set_cb(platform_timer_cb new_fp); 00039 00040 /** 00041 * \brief This function is API for stack timer start 00042 * 00043 * \param slots define how many 50us slot time period will be started 00044 * 00045 */ 00046 extern void platform_timer_start(uint16_t slots); 00047 00048 /** 00049 * \brief This function is API for stack timer stop 00050 * 00051 */ 00052 extern void platform_timer_disable(void); 00053 00054 /** 00055 * \brief This function is API for stack timer to read active timer remaining slot count 00056 * 00057 * \return 50us time slot remaining 00058 */ 00059 extern uint16_t platform_timer_get_remaining_slots(void); 00060 00061 #endif // NS_EXCLUDE_HIGHRES_TIMER 00062 00063 #ifdef NS_EVENTLOOP_USE_TICK_TIMER 00064 /** 00065 * \brief This function is API for registering low resolution tick timer callback. Also does 00066 * any necessary initialization of the tick timer. 00067 * 00068 * \return -1 for failure, success otherwise 00069 */ 00070 extern int8_t platform_tick_timer_register(void (*tick_timer_cb_handler)(void)); 00071 00072 /** 00073 * \brief This function is API for starting the low resolution tick timer. The callback 00074 * set with platform_tick_timer_register gets called periodically until stopped 00075 * by calling platform_tick_timer_stop. 00076 * 00077 * \param period_ms define how many milliseconds time period will be started 00078 * \return -1 for failure, success otherwise 00079 */ 00080 extern int8_t platform_tick_timer_start(uint32_t period_ms); 00081 00082 /** 00083 * \brief This function is API for stopping the low resolution tick timer 00084 * 00085 * \return -1 for failure, success otherwise 00086 */ 00087 extern int8_t platform_tick_timer_stop(void); 00088 00089 #endif // NS_EVENTLOOP_USE_TICK_TIMER 00090 00091 #ifdef __cplusplus 00092 } 00093 #endif 00094 00095 #endif /* ARM_HAL_TIMER_H_ */
Generated on Tue Jul 12 2022 19:12:11 by
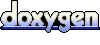