
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_startup.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 #include "pal.h" 00018 #include "PlatIncludes.h" 00019 #include "fsl_debug_console.h" 00020 #include "FreeRTOS.h" 00021 #include "task.h" 00022 #include "lwip/sys.h" 00023 #include "pal_BSP.h" 00024 00025 #ifndef TEST_MAIN_THREAD_STACK_SIZE 00026 #define TEST_MAIN_THREAD_STACK_SIZE (1024*8) 00027 #endif 00028 00029 #ifndef TEST_FS_INIT_THREAD_STACK_SIZE 00030 #define TEST_FS_INIT_THREAD_STACK_SIZE (1024*4) 00031 #endif 00032 00033 #ifndef BSP_MAX_INIT_ITERATIONS 00034 #define BSP_MAX_INIT_ITERATIONS 600 00035 #endif 00036 00037 #ifndef BSP_INIT_INTERATION_TIME_MS 00038 #define BSP_INIT_INTERATION_TIME_MS 1000 00039 #endif 00040 00041 00042 00043 00044 extern bool dhcpDone; 00045 extern bool FileSystemInit; 00046 int main(int argc, char * argv[]); 00047 00048 00049 void freeRTOScallMain(void * arg) 00050 { 00051 main(0,NULL); 00052 } 00053 00054 00055 //returns the network context 00056 bspStatus_t initPlatform(void** outputContext) 00057 { 00058 bspStatus_t status = BSP_SUCCESS; 00059 static uint8_t initState = 0; 00060 BaseType_t rtosStatus = pdPASS; 00061 //1st time in init the system is required 00062 if (0 == initState) 00063 { 00064 //set for next initState 00065 initState = 1; 00066 00067 //Init Board 00068 boardInit(); 00069 00070 //Init FileSystem 00071 rtosStatus = xTaskCreate((TaskFunction_t)fileSystemMountDrive, "FileSystemInit", TEST_FS_INIT_THREAD_STACK_SIZE, NULL, tskIDLE_PRIORITY + 3, NULL); 00072 if (pdPASS != rtosStatus) 00073 { 00074 PRINTF("BSP ERROR: failed to create task with error %x \r\n", rtosStatus); 00075 status = BSP_THREAD_CREATION_FAILURE; 00076 goto end; 00077 } 00078 00079 //Init DHCP thread - note: according to LWIP docs this funciton can't fail (states that porting code must asset in case of failure) 00080 sys_thread_new("networkInit", networkInit, NULL, 1024, tskIDLE_PRIORITY + 2); 00081 00082 //Init Unit testing thread 00083 rtosStatus = xTaskCreate(freeRTOScallMain, "main", (uint16_t)PAL_TEST_THREAD_STACK_SIZE, NULL, tskIDLE_PRIORITY + 1, NULL); 00084 if (pdPASS != rtosStatus) 00085 { 00086 PRINTF("BSP ERROR: failed to create task with error %x \r\n", rtosStatus); 00087 status = BSP_THREAD_CREATION_FAILURE; 00088 goto end; 00089 } 00090 00091 //Start OS 00092 vTaskStartScheduler(); 00093 // taks scheduler shouldn't exit unless an error has occured 00094 PRINTF("BSP ERROR: failed to run scheduler \r\n"); 00095 status = BSP_THREAD_CREATION_FAILURE; 00096 goto end; 00097 } 00098 if (1 == initState) 00099 { 00100 uint32_t counter = 0; // limit waiting for FileSystemInit and dhcpDone to 00101 while (((!FileSystemInit) || (!dhcpDone)) && (counter < BSP_MAX_INIT_ITERATIONS)) 00102 { 00103 vTaskDelay(BSP_INIT_INTERATION_TIME_MS); 00104 PRINTF("waiting to file system % network to init\r\n"); 00105 counter++; 00106 } 00107 00108 if ((!dhcpDone) || (!FileSystemInit)) 00109 { 00110 if (!FileSystemInit) 00111 { 00112 status = BSP_FILE_SYSTEM_TIMEOUT; 00113 PRINTF("BSP ERROR: timeout while waiting for file system init\r\n"); 00114 00115 } 00116 if (!dhcpDone) 00117 { 00118 status = BSP_NETWORK_TIMEOUT; 00119 PRINTF("BSP ERROR: timeout while waiting for dhcp \r\n"); 00120 } 00121 goto end; 00122 } 00123 00124 } 00125 00126 if (NULL != outputContext) 00127 { 00128 *outputContext = palTestGetNetWorkInterfaceContext(); 00129 } 00130 00131 end: 00132 return status; 00133 00134 } 00135 00136 00137 bool runProgram(testMain_t func, pal_args_t * args) 00138 { 00139 func(args); 00140 return true; 00141 } 00142 00143 #ifndef __CC_ARM /* ARM Compiler */ 00144 /*This is a Hardfault handler to use in debug for more info please read - 00145 * http://www.freertos.org/Debugging-Hard-Faults-On-Cortex-M-Microcontrollers.html */ 00146 /* The prototype shows it is a naked function - in effect this is just an 00147 assembly function. */ 00148 void HardFault_Handler( void ) __attribute__( ( naked ) ); 00149 00150 /* The fault handler implementation calls a function called 00151 prvGetRegistersFromStack(). */ 00152 void HardFault_Handler(void) 00153 { 00154 __asm volatile 00155 ( 00156 " tst lr, #4 \n" 00157 " ite eq \n" 00158 " mrseq r0, msp \n" 00159 " mrsne r0, psp \n" 00160 " ldr r1, [r0, #24] \n" 00161 " ldr r2, handler2_address_const \n" 00162 " bx r2 \n" 00163 " handler2_address_const: .word prvGetRegistersFromStack \n" 00164 ); 00165 } 00166 00167 00168 void prvGetRegistersFromStack( uint32_t *pulFaultStackAddress ) 00169 { 00170 /* These are volatile to try and prevent the compiler/linker optimising them 00171 away as the variables never actually get used. If the debugger won't show the 00172 values of the variables, make them global my moving their declaration outside 00173 of this function. */ 00174 volatile uint32_t r0; 00175 volatile uint32_t r1; 00176 volatile uint32_t r2; 00177 volatile uint32_t r3; 00178 volatile uint32_t r12; 00179 volatile uint32_t lr; /* Link register. */ 00180 volatile uint32_t pc; /* Program counter. */ 00181 volatile uint32_t psr;/* Program status register. */ 00182 00183 r0 = pulFaultStackAddress[ 0 ]; 00184 r1 = pulFaultStackAddress[ 1 ]; 00185 r2 = pulFaultStackAddress[ 2 ]; 00186 r3 = pulFaultStackAddress[ 3 ]; 00187 00188 r12 = pulFaultStackAddress[ 4 ]; 00189 lr = pulFaultStackAddress[ 5 ]; 00190 pc = pulFaultStackAddress[ 6 ]; 00191 psr = pulFaultStackAddress[ 7 ]; 00192 00193 /* When the following line is hit, the variables contain the register values. */ 00194 PRINTF("r0 = %d\r\n" 00195 "r1 = %d\r\n" 00196 "r2 = %d\r\n" 00197 "r3 = %d\r\n" 00198 "r12 = %d\r\n" 00199 "lr = %d\r\n" 00200 "pc = %d\r\n" 00201 "psr = %d\r\n", 00202 r0,r1,r2,r3,r12,lr,pc,psr); 00203 for( ;; ); 00204 } 00205 00206 #endif 00207 // This is used by unity for output. The make file must pass a definition of the following form 00208 // -DUNITY_OUTPUT_CHAR=unity_output_char 00209 void unity_output_char(int c) 00210 { 00211 PUTCHAR(c); 00212 } 00213 00214
Generated on Tue Jul 12 2022 19:12:15 by
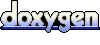