
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
FileSystemInit.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 #include "pal.h" 00017 #include "FreeRTOS.h" 00018 #include "task.h" 00019 #include "fsl_mpu.h" 00020 #include "ff.h" 00021 #include "diskio.h" 00022 #include "sdhc_config.h" 00023 #include "fsl_debug_console.h" 00024 00025 00026 //uncomment this to create the partitions 00027 //#define PAL_EXAMPLE_GENERATE_PARTITION 1 00028 //Uncomment this to allow format 00029 //#define PAL_EXAMPLE_FORMAT_PARTITION 1 00030 00031 #if (PAL_NUMBER_OF_PARTITIONS > 0) 00032 00033 #ifndef _MULTI_PARTITION 00034 #error "Please Define _MULTI_PARTITION in ffconf.h" 00035 #endif 00036 00037 #if ((PAL_NUMBER_OF_PARTITIONS > 2) || (PAL_NUMBER_OF_PARTITIONS < 0)) 00038 #error "Pal partition number is not supported, please set to a number between 0 and 2" 00039 #endif 00040 00041 PARTITION VolToPart[] = { 00042 #if (PAL_NUMBER_OF_PARTITIONS > 0) 00043 {SDDISK,1}, /* 0: */ 00044 #endif 00045 #if (PAL_NUMBER_OF_PARTITIONS > 1) 00046 {SDDISK,2} /* 1: */ 00047 #endif 00048 }; 00049 #endif 00050 00051 bool FileSystemInit = false; 00052 #define MAX_SD_READ_RETRIES 5 00053 #define LABEL_LENGTH 66 00054 /*! 00055 * @brief Get event instance. 00056 * @param eventType The event type 00057 * @return The event instance's pointer. 00058 */ 00059 PAL_PRIVATE volatile uint32_t *EVENT_GetInstance(event_t eventType); 00060 00061 /*! @brief Transfer complete event. */ 00062 PAL_PRIVATE volatile uint32_t g_eventTransferComplete; 00063 00064 PAL_PRIVATE volatile uint32_t g_eventSDReady; 00065 00066 /*! @brief Time variable unites as milliseconds. */ 00067 PAL_PRIVATE volatile uint32_t g_timeMilliseconds; 00068 00069 /*! @brief Preallocated Work area (file system object) for logical drive, should NOT be free or lost*/ 00070 PAL_PRIVATE FATFS fileSystem[2]; 00071 00072 /*! \brief CallBack function for SD card initialization 00073 * Set systick reload value to generate 1ms interrupt 00074 * @param void 00075 * 00076 * \return void 00077 * 00078 */ 00079 void EVENT_InitTimer(void) 00080 { 00081 /* Set systick reload value to generate 1ms interrupt */ 00082 SysTick_Config(CLOCK_GetFreq(kCLOCK_CoreSysClk) / 1000U); 00083 } 00084 00085 00086 /*! \brief CallBack function for SD card initialization 00087 * 00088 * @param void 00089 * 00090 * \return pointer to the requested instance 00091 * 00092 */ 00093 PAL_PRIVATE volatile uint32_t *EVENT_GetInstance(event_t eventType) 00094 { 00095 volatile uint32_t *event; 00096 00097 switch (eventType) 00098 { 00099 case kEVENT_TransferComplete: 00100 event = &g_eventTransferComplete; 00101 break; 00102 default: 00103 event = NULL; 00104 break; 00105 } 00106 00107 return event; 00108 } 00109 00110 /*! \brief CallBack function for SD card initialization 00111 * 00112 * @param event_t 00113 * 00114 * \return TRUE if instance was found 00115 * 00116 */ 00117 bool EVENT_Create(event_t eventType) 00118 { 00119 volatile uint32_t *event = EVENT_GetInstance(eventType); 00120 00121 if (event) 00122 { 00123 *event = 0; 00124 return true; 00125 } 00126 else 00127 { 00128 return false; 00129 } 00130 } 00131 00132 /*! \brief blockDelay - Blocks the task and count the number of ticks given 00133 * 00134 * @param void 00135 * 00136 * \return TRUE - on success 00137 * 00138 */ 00139 void blockDelay(uint32_t Ticks) 00140 { 00141 uint32_t tickCounts = 0; 00142 for(tickCounts = 0; tickCounts < Ticks; tickCounts++){} 00143 } 00144 00145 00146 /*! \brief CallBack function for SD card initialization 00147 * 00148 * @param void 00149 * 00150 * \return TRUE - on success 00151 * 00152 */ 00153 bool EVENT_Wait(event_t eventType, uint32_t timeoutMilliseconds) 00154 { 00155 uint32_t startTime; 00156 uint32_t elapsedTime; 00157 00158 volatile uint32_t *event = EVENT_GetInstance(eventType); 00159 00160 if (timeoutMilliseconds && event) 00161 { 00162 startTime = g_timeMilliseconds; 00163 do 00164 { 00165 elapsedTime = (g_timeMilliseconds - startTime); 00166 } while ((*event == 0U) && (elapsedTime < timeoutMilliseconds)); 00167 *event = 0U; 00168 00169 return ((elapsedTime < timeoutMilliseconds) ? true : false); 00170 } 00171 else 00172 { 00173 return false; 00174 } 00175 } 00176 00177 /*! \brief CallBack function for SD card initialization 00178 * 00179 * @param eventType 00180 * 00181 * \return TRUE if instance was found 00182 * 00183 */ 00184 bool EVENT_Notify(event_t eventType) 00185 { 00186 volatile uint32_t *event = EVENT_GetInstance(eventType); 00187 00188 if (event) 00189 { 00190 *event = 1U; 00191 return true; 00192 } 00193 else 00194 { 00195 return false; 00196 } 00197 } 00198 00199 /*! \brief CallBack function for SD card initialization 00200 * 00201 * @param eventType 00202 * 00203 * \return void 00204 * 00205 */ 00206 void EVENT_Delete(event_t eventType) 00207 { 00208 volatile uint32_t *event = EVENT_GetInstance(eventType); 00209 00210 if (event) 00211 { 00212 *event = 0U; 00213 } 00214 } 00215 00216 00217 00218 00219 static int initPartition(pal_fsStorageID_t partitionId) 00220 { 00221 char folderName[PAL_MAX_FILE_AND_FOLDER_LENGTH] = {0}; 00222 FRESULT fatResult = FR_OK; 00223 int status = 0; 00224 00225 status = pal_fsGetMountPoint(partitionId,PAL_MAX_FILE_AND_FOLDER_LENGTH,folderName); 00226 if (PAL_SUCCESS == status) 00227 { 00228 fatResult = f_mount(&fileSystem[partitionId], folderName, 1U); 00229 if (FR_OK != fatResult) 00230 { 00231 #ifdef PAL_EXAMPLE_FORMAT_PARTITION 00232 PRINTF("Failed to mount partition %s in disk formating and trying again\r\n",folderName); 00233 fatResult = f_mkfs(folderName, 0, 0); 00234 if (FR_OK == fatResult) 00235 { 00236 fatResult = f_mount(&fileSystem[partitionId], folderName, 1U); 00237 } 00238 #endif 00239 if (FR_OK != fatResult) 00240 { 00241 PRINTF("Failed to format & mount partition %s\r\n",folderName); 00242 } 00243 } 00244 } 00245 else 00246 { 00247 PRINTF("Failed to get mount point for partition %d\r\n",partitionId); 00248 } 00249 return status; 00250 } 00251 00252 /*! \brief This function mount the fatfs on and SD card 00253 * 00254 * @param void 00255 * 00256 * \return palStatus_t - PAL_SUCCESS when mount point succeeded 00257 * 00258 */ 00259 00260 void fileSystemMountDrive(void) 00261 { 00262 PRINTF("%s : Creating FileSystem SetUp thread!\r\n",__FUNCTION__); 00263 int count = 0; 00264 int status = 0; 00265 00266 if (FileSystemInit == false) 00267 { 00268 //Detected SD card inserted 00269 while (!(GPIO_ReadPinInput(BOARD_SDHC_CD_GPIO_BASE, BOARD_SDHC_CD_GPIO_PIN))) 00270 { 00271 blockDelay(1000U); 00272 if (count++ > MAX_SD_READ_RETRIES) 00273 { 00274 break; 00275 } 00276 } 00277 00278 if(count < MAX_SD_READ_RETRIES) 00279 { 00280 /* Delay some time to make card stable. */ 00281 blockDelay(10000000U); 00282 #ifdef PAL_EXAMPLE_GENERATE_PARTITION 00283 #if (PAL_NUMBER_OF_PARTITIONS == 1) 00284 DWORD plist[] = {100,0,0,0}; 00285 #elif (PAL_NUMBER_OF_PARTITIONS == 2) //else of (PAL_NUMBER_OF_PARTITIONS == 1) 00286 DWORD plist[] = {50,50,0,0}; 00287 #endif //(PAL_NUMBER_OF_PARTITIONS == 1) 00288 BYTE work[_MAX_SS]; 00289 00290 status = f_fdisk(SDDISK,plist, work); 00291 PRINTF("f_fdisk fatResult=%d\r\n",status); 00292 if (FR_OK != status) 00293 { 00294 PRINTF("Failed to create partitions in disk\r\n"); 00295 } 00296 #endif //PAL_EXAMPLE_GENERATE_PARTITION 00297 00298 00299 status = initPartition(PAL_FS_PARTITION_PRIMARY); 00300 #if (PAL_NUMBER_OF_PARTITIONS == 2) 00301 status = initPartition(PAL_FS_PARTITION_SECONDARY); 00302 #endif 00303 00304 00305 if (!status) // status will be 0, if all passess 00306 { 00307 FileSystemInit = true; 00308 PRINTF("%s : Exit FileSystem SetUp thread!\r\n",__FUNCTION__); 00309 } 00310 } 00311 } 00312 vTaskDelete( NULL ); 00313 }
Generated on Tue Jul 12 2022 19:12:12 by
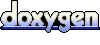