
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
ISM43362.cpp
00001 /* ISM43362 Example 00002 * 00003 * Copyright (c) STMicroelectronics 2018 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include <string.h> 00018 #include "ISM43362.h" 00019 #include "mbed_debug.h" 00020 00021 // activate / de-activate debug 00022 #define ism_debug 0 00023 00024 ISM43362::ISM43362(PinName mosi, PinName miso, PinName sclk, PinName nss, PinName resetpin, PinName datareadypin, PinName wakeup, bool debug) 00025 : _bufferspi(mosi, miso, sclk, nss, datareadypin), 00026 _parser(_bufferspi), 00027 _resetpin(resetpin), 00028 _packets(0), _packets_end(&_packets) 00029 { 00030 DigitalOut wakeup_pin(wakeup); 00031 _bufferspi.format(16, 0); /* 16bits, ploarity low, phase 1Edge, master mode */ 00032 _bufferspi.frequency(20000000); /* up to 20 MHz */ 00033 _active_id = 0xFF; 00034 _FwVersionId = 0; 00035 00036 _ism_debug = debug || ism_debug; 00037 reset(); 00038 } 00039 00040 /** 00041 * @brief Parses and returns number from string. 00042 * @param ptr: pointer to string 00043 * @param cnt: pointer to the number of parsed digit 00044 * @retval integer value. 00045 */ 00046 #define CHARISHEXNUM(x) (((x) >= '0' && (x) <= '9') || \ 00047 ((x) >= 'a' && (x) <= 'f') || \ 00048 ((x) >= 'A' && (x) <= 'F')) 00049 #define CHARISNUM(x) ((x) >= '0' && (x) <= '9') 00050 #define CHAR2NUM(x) ((x) - '0') 00051 00052 00053 extern "C" int32_t ParseNumber(char *ptr, uint8_t *cnt) 00054 { 00055 uint8_t minus = 0, i = 0; 00056 int32_t sum = 0; 00057 00058 if (*ptr == '-') { /* Check for minus character */ 00059 minus = 1; 00060 ptr++; 00061 i++; 00062 } 00063 if (*ptr == 'C') { /* input string from get_firmware_version is Cx.x.x.x */ 00064 ptr++; 00065 } 00066 00067 while (CHARISNUM(*ptr) || (*ptr == '.')) { /* Parse number */ 00068 if (*ptr == '.') { 00069 ptr++; // next char 00070 } else { 00071 sum = 10 * sum + CHAR2NUM(*ptr); 00072 ptr++; 00073 i++; 00074 } 00075 } 00076 00077 if (cnt != NULL) { /* Save number of characters used for number */ 00078 *cnt = i; 00079 } 00080 if (minus) { /* Minus detected */ 00081 return 0 - sum; 00082 } 00083 return sum; /* Return number */ 00084 } 00085 00086 uint32_t ISM43362::get_firmware_version(void) 00087 { 00088 char tmp_buffer[250]; 00089 char *ptr, *ptr2; 00090 char _fw_version[16]; 00091 00092 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00093 if (!(_parser.send("I?") && _parser.recv("%[^\n^\r]\r\n", tmp_buffer) && check_response())) { 00094 debug_if(_ism_debug, "ISM43362: get_firmware_version is FAIL\r\n"); 00095 return 0; 00096 } 00097 debug_if(_ism_debug, "ISM43362: get_firmware_version = %s\r\n", tmp_buffer); 00098 00099 // Get the first version in the string 00100 ptr = strtok((char *)tmp_buffer, ","); 00101 ptr = strtok(NULL, ","); 00102 ptr2 = strtok(NULL, ","); 00103 if (ptr == NULL) { 00104 debug_if(_ism_debug, "ISM43362: get_firmware_version decoding is FAIL\r\n"); 00105 return 0; 00106 } 00107 strncpy(_fw_version, ptr, ptr2 - ptr); 00108 _FwVersionId = ParseNumber(_fw_version, NULL); 00109 00110 return _FwVersionId; 00111 } 00112 00113 bool ISM43362::reset(void) 00114 { 00115 char tmp_buffer[100]; 00116 debug_if(_ism_debug, "ISM43362: Reset Module\r\n"); 00117 _resetpin = 0; 00118 wait_ms(10); 00119 _resetpin = 1; 00120 wait_ms(500); 00121 00122 /* Wait for prompt line : the string is "> ". */ 00123 /* As the space char is not detected by sscanf function in parser.recv, */ 00124 /* we need to use %[\n] */ 00125 if (!_parser.recv(">%[^\n]", tmp_buffer)) { 00126 debug_if(_ism_debug, "ISM43362: Reset Module failed\r\n"); 00127 return false; 00128 } 00129 return true; 00130 } 00131 00132 void ISM43362::print_rx_buff(void) 00133 { 00134 char tmp[150] = {0}; 00135 uint16_t i = 0; 00136 debug_if(_ism_debug, "ISM43362: "); 00137 while (i < 150) { 00138 int c = _parser.getc(); 00139 if (c < 0) { 00140 break; 00141 } 00142 tmp[i] = c; 00143 debug_if(_ism_debug, "0x%2X ", c); 00144 i++; 00145 } 00146 debug_if(_ism_debug, "\n"); 00147 debug_if(_ism_debug, "ISM43362: Buffer content =====%s=====\r\n", tmp); 00148 } 00149 00150 /* checks the standard OK response of the WIFI module, shouldbe: 00151 * \r\nDATA\r\nOK\r\n>sp 00152 * or 00153 * \r\nERROR\r\nUSAGE\r\n>sp 00154 * function returns true if OK, false otherwise. In case of error, 00155 * print error content then flush buffer */ 00156 bool ISM43362::check_response(void) 00157 { 00158 char tmp_buffer[100]; 00159 if (!_parser.recv("OK\r\n")) { 00160 print_rx_buff(); 00161 _parser.flush(); 00162 return false; 00163 } 00164 00165 /* Then we should get the prompt: "> " */ 00166 /* As the space char is not detected by sscanf function in parser.recv, */ 00167 /* we need to use %[\n] */ 00168 if (!_parser.recv(">%[^\n]", tmp_buffer)) { 00169 debug_if(_ism_debug, "ISM43362: Missing prompt in WIFI resp\r\n"); 00170 print_rx_buff(); 00171 _parser.flush(); 00172 return false; 00173 } 00174 00175 /* Inventek module do stuffing / padding of data with 0x15, 00176 * in case buffer contains such */ 00177 while (1) { 00178 int c = _parser.getc(); 00179 if (c == 0x15) { 00180 // debug_if(_ism_debug, "ISM43362: Flush char 0x%x\n", c); 00181 continue; 00182 } else { 00183 /* How to put it back if needed ? */ 00184 break; 00185 } 00186 } 00187 return true; 00188 } 00189 00190 bool ISM43362::dhcp(bool enabled) 00191 { 00192 return (_parser.send("C4=%d", enabled ? 1 : 0) && check_response()); 00193 } 00194 00195 int ISM43362::connect(const char *ap, const char *passPhrase, ism_security_t ap_sec) 00196 { 00197 char tmp[256]; 00198 00199 if (!(_parser.send("C1=%s", ap) && check_response())) { 00200 return NSAPI_ERROR_PARAMETER; 00201 } 00202 00203 if (!(_parser.send("C2=%s", passPhrase) && check_response())) { 00204 return NSAPI_ERROR_PARAMETER; 00205 } 00206 00207 /* Check security level is acceptable */ 00208 if (ap_sec > ISM_SECURITY_WPA_WPA2) { 00209 debug_if(_ism_debug, "ISM43362: Unsupported security level %d\n", ap_sec); 00210 return NSAPI_ERROR_UNSUPPORTED; 00211 } 00212 00213 if (!(_parser.send("C3=%d", ap_sec) && check_response())) { 00214 return NSAPI_ERROR_PARAMETER; 00215 } 00216 00217 if (_parser.send("C0")) { 00218 while (_parser.recv("%[^\n]\n", tmp)) { 00219 if (strstr(tmp, "OK")) { 00220 _parser.flush(); 00221 return NSAPI_ERROR_OK; 00222 } 00223 if (strstr(tmp, "JOIN")) { 00224 if (strstr(tmp, "Failed")) { 00225 _parser.flush(); 00226 return NSAPI_ERROR_AUTH_FAILURE; 00227 } 00228 } 00229 } 00230 } 00231 00232 return NSAPI_ERROR_NO_CONNECTION; 00233 } 00234 00235 bool ISM43362::disconnect(void) 00236 { 00237 return (_parser.send("CD") && check_response()); 00238 } 00239 00240 const char *ISM43362::getIPAddress(void) 00241 { 00242 char tmp_ip_buffer[250]; 00243 char *ptr, *ptr2; 00244 00245 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00246 if (!(_parser.send("C?") 00247 && _parser.recv("%[^\n^\r]\r\n", tmp_ip_buffer) 00248 && check_response())) { 00249 debug_if(_ism_debug, "ISM43362: getIPAddress LINE KO: %s\n", tmp_ip_buffer); 00250 return 0; 00251 } 00252 00253 /* Get the IP address in the result */ 00254 /* TODO : check if the begining of the string is always = "eS-WiFi_AP_C47F51011231," */ 00255 ptr = strtok((char *)tmp_ip_buffer, ","); 00256 ptr = strtok(NULL, ","); 00257 ptr = strtok(NULL, ","); 00258 ptr = strtok(NULL, ","); 00259 ptr = strtok(NULL, ","); 00260 ptr = strtok(NULL, ","); 00261 ptr2 = strtok(NULL, ","); 00262 if (ptr == NULL) { 00263 return 0; 00264 } 00265 strncpy(_ip_buffer, ptr, ptr2 - ptr); 00266 00267 tmp_ip_buffer[59] = 0; 00268 debug_if(_ism_debug, "ISM43362: receivedIPAddress: %s\n", _ip_buffer); 00269 00270 return _ip_buffer; 00271 } 00272 00273 const char *ISM43362::getMACAddress(void) 00274 { 00275 if (!(_parser.send("Z5") && _parser.recv("%s\r\n", _mac_buffer) && check_response())) { 00276 debug_if(_ism_debug, "ISM43362: receivedMacAddress LINE KO: %s\n", _mac_buffer); 00277 return 0; 00278 } 00279 00280 debug_if(_ism_debug, "ISM43362: receivedMacAddress:%s, size=%d\r\n", _mac_buffer, sizeof(_mac_buffer)); 00281 00282 return _mac_buffer; 00283 } 00284 00285 const char *ISM43362::getGateway() 00286 { 00287 char tmp[250]; 00288 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00289 if (!(_parser.send("C?") && _parser.recv("%[^\n^\r]\r\n", tmp) && check_response())) { 00290 debug_if(_ism_debug, "ISM43362: getGateway LINE KO: %s\r\n", tmp); 00291 return 0; 00292 } 00293 00294 /* Extract the Gateway in the received buffer */ 00295 char *ptr; 00296 ptr = strtok(tmp, ","); 00297 for (int i = 0; i < 7; i++) { 00298 if (ptr == NULL) { 00299 break; 00300 } 00301 ptr = strtok(NULL, ","); 00302 } 00303 00304 strncpy(_gateway_buffer, ptr, sizeof(_gateway_buffer)); 00305 00306 debug_if(_ism_debug, "ISM43362: getGateway: %s\r\n", _gateway_buffer); 00307 00308 return _gateway_buffer; 00309 } 00310 00311 const char *ISM43362::getNetmask() 00312 { 00313 char tmp[250]; 00314 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00315 if (!(_parser.send("C?") && _parser.recv("%[^\n^\r]\r\n", tmp) && check_response())) { 00316 debug_if(_ism_debug, "ISM43362: getNetmask LINE KO: %s\n", tmp); 00317 return 0; 00318 } 00319 00320 /* Extract Netmask in the received buffer */ 00321 char *ptr; 00322 ptr = strtok(tmp, ","); 00323 for (int i = 0; i < 6; i++) { 00324 if (ptr == NULL) { 00325 break; 00326 } 00327 ptr = strtok(NULL, ","); 00328 } 00329 00330 strncpy(_netmask_buffer, ptr, sizeof(_netmask_buffer)); 00331 00332 debug_if(_ism_debug, "ISM43362: getNetmask: %s\r\n", _netmask_buffer); 00333 00334 return _netmask_buffer; 00335 } 00336 00337 int8_t ISM43362::getRSSI() 00338 { 00339 int8_t rssi; 00340 char tmp[25]; 00341 00342 if (!(_parser.send("CR") && _parser.recv("%s\r\n", tmp) && check_response())) { 00343 debug_if(_ism_debug, "ISM43362: getRSSI LINE KO: %s\r\n", tmp); 00344 return 0; 00345 } 00346 00347 rssi = ParseNumber(tmp, NULL); 00348 00349 debug_if(_ism_debug, "ISM43362: getRSSI: %d\r\n", rssi); 00350 00351 return rssi; 00352 } 00353 /** 00354 * @brief Parses Security type. 00355 * @param ptr: pointer to string 00356 * @retval Encryption type. 00357 */ 00358 extern "C" nsapi_security_t ParseSecurity(char *ptr) 00359 { 00360 if (strstr(ptr, "Open")) { 00361 return NSAPI_SECURITY_NONE; 00362 } else if (strstr(ptr, "WEP")) { 00363 return NSAPI_SECURITY_WEP; 00364 } else if (strstr(ptr, "WPA2 AES")) { 00365 return NSAPI_SECURITY_WPA2; 00366 } else if (strstr(ptr, "WPA WPA2")) { 00367 return NSAPI_SECURITY_WPA_WPA2; 00368 } else if (strstr(ptr, "WPA2 TKIP")) { 00369 return NSAPI_SECURITY_UNKNOWN; // no match in mbed 00370 } else if (strstr(ptr, "WPA2")) { 00371 return NSAPI_SECURITY_WPA2; // catch any other WPA2 formula 00372 } else if (strstr(ptr, "WPA")) { 00373 return NSAPI_SECURITY_WPA; 00374 } else { 00375 return NSAPI_SECURITY_UNKNOWN; 00376 } 00377 } 00378 00379 /** 00380 * @brief Convert char in Hex format to integer. 00381 * @param a: character to convert 00382 * @retval integer value. 00383 */ 00384 extern "C" uint8_t Hex2Num(char a) 00385 { 00386 if (a >= '0' && a <= '9') { /* Char is num */ 00387 return a - '0'; 00388 } else if (a >= 'a' && a <= 'f') { /* Char is lowercase character A - Z (hex) */ 00389 return (a - 'a') + 10; 00390 } else if (a >= 'A' && a <= 'F') { /* Char is uppercase character A - Z (hex) */ 00391 return (a - 'A') + 10; 00392 } 00393 00394 return 0; 00395 } 00396 00397 /** 00398 * @brief Extract a hex number from a string. 00399 * @param ptr: pointer to string 00400 * @param cnt: pointer to the number of parsed digit 00401 * @retval Hex value. 00402 */ 00403 extern "C" uint32_t ParseHexNumber(char *ptr, uint8_t *cnt) 00404 { 00405 uint32_t sum = 0; 00406 uint8_t i = 0; 00407 00408 while (CHARISHEXNUM(*ptr)) { /* Parse number */ 00409 sum <<= 4; 00410 sum += Hex2Num(*ptr); 00411 ptr++; 00412 i++; 00413 } 00414 00415 if (cnt != NULL) { /* Save number of characters used for number */ 00416 *cnt = i; 00417 } 00418 return sum; /* Return number */ 00419 } 00420 00421 bool ISM43362::isConnected(void) 00422 { 00423 return getIPAddress() != 0; 00424 } 00425 00426 int ISM43362::scan(WiFiAccessPoint *res, unsigned limit) 00427 { 00428 unsigned cnt = 0, num = 0; 00429 char *ptr; 00430 char tmp[256]; 00431 00432 if (!(_parser.send("F0"))) { 00433 debug_if(_ism_debug, "ISM43362: scan error\r\n"); 00434 return 0; 00435 } 00436 00437 /* Parse the received buffer and fill AP buffer */ 00438 /* Use %[^\n] instead of %s to allow having spaces in the string */ 00439 while (_parser.recv("#%[^\n]\n", tmp)) { 00440 if (limit != 0 && cnt >= limit) { 00441 /* reached end */ 00442 break; 00443 } 00444 nsapi_wifi_ap_t ap = {0}; 00445 debug_if(_ism_debug, "ISM43362: received:%s\n", tmp); 00446 ptr = strtok(tmp, ","); 00447 num = 0; 00448 while (ptr != NULL) { 00449 switch (num++) { 00450 case 0: /* Ignore index */ 00451 case 4: /* Ignore Max Rate */ 00452 case 5: /* Ignore Network Type */ 00453 case 7: /* Ignore Radio Band */ 00454 break; 00455 case 1: 00456 ptr[strlen(ptr) - 1] = 0; 00457 strncpy((char *)ap.ssid, ptr + 1, 32); 00458 break; 00459 case 2: 00460 for (int i = 0; i < 6; i++) { 00461 ap.bssid[i] = ParseHexNumber(ptr + (i * 3), NULL); 00462 } 00463 break; 00464 case 3: 00465 ap.rssi = ParseNumber(ptr, NULL); 00466 break; 00467 case 6: 00468 ap.security = ParseSecurity(ptr); 00469 break; 00470 case 8: 00471 ap.channel = ParseNumber(ptr, NULL); 00472 num = 1; 00473 break; 00474 default: 00475 break; 00476 } 00477 ptr = strtok(NULL, ","); 00478 } 00479 if (res != NULL) { 00480 res[cnt] = WiFiAccessPoint(ap); 00481 } 00482 cnt++; 00483 } 00484 00485 /* We may stop before having read all the APs list, so flush the rest of 00486 * it as well as OK commands */ 00487 _parser.flush(); 00488 00489 debug_if(_ism_debug, "ISM43362: End of Scan: cnt=%d\n", cnt); 00490 00491 return cnt; 00492 00493 } 00494 00495 bool ISM43362::open(const char *type, int id, const char *addr, int port) 00496 { 00497 /* TODO : This is the implementation for the client socket, need to check if need to create openserver too */ 00498 //IDs only 0-3 00499 if ((id < 0) || (id > 3)) { 00500 debug_if(_ism_debug, "ISM43362: open: wrong id\n"); 00501 return false; 00502 } 00503 /* Set communication socket */ 00504 _active_id = id; 00505 if (!(_parser.send("P0=%d", id) && check_response())) { 00506 debug_if(_ism_debug, "ISM43362: open: P0 issue\n"); 00507 return false; 00508 } 00509 /* Set protocol */ 00510 if (!(_parser.send("P1=%s", type) && check_response())) { 00511 debug_if(_ism_debug, "ISM43362: open: P1 issue\n"); 00512 return false; 00513 } 00514 /* Set address */ 00515 if (!(_parser.send("P3=%s", addr) && check_response())) { 00516 debug_if(_ism_debug, "ISM43362: open: P3 issue\n"); 00517 return false; 00518 } 00519 if (!(_parser.send("P4=%d", port) && check_response())) { 00520 debug_if(_ism_debug, "ISM43362: open: P4 issue\n"); 00521 return false; 00522 } 00523 /* Start client */ 00524 if (!(_parser.send("P6=1") && check_response())) { 00525 debug_if(_ism_debug, "ISM43362: open: P6 issue\n"); 00526 return false; 00527 } 00528 00529 /* request as much data as possible - i.e. module max size */ 00530 if (!(_parser.send("R1=%d", ES_WIFI_MAX_RX_PACKET_SIZE) && check_response())) { 00531 debug_if(_ism_debug, "ISM43362: open: R1 issue\n"); 00532 return -1; 00533 } 00534 00535 /* Non blocking mode : set Read Transport Timeout to 1ms */ 00536 if (!(_parser.send("R2=1") && check_response())) { 00537 debug_if(_ism_debug, "ISM43362: open: R2 issue\n"); 00538 return -1; 00539 } 00540 00541 debug_if(_ism_debug, "ISM43362: open ok with id %d type %s addr %s port %d\n", id, type, addr, port); 00542 00543 return true; 00544 } 00545 00546 bool ISM43362::dns_lookup(const char *name, char *ip) 00547 { 00548 char tmp[30]; 00549 00550 if (!(_parser.send("D0=%s", name) && _parser.recv("%s\r\n", tmp) 00551 && check_response())) { 00552 debug_if(_ism_debug, "ISM43362 dns_lookup: D0 issue: %s\n", tmp); 00553 return 0; 00554 } 00555 00556 strncpy(ip, tmp, sizeof(tmp)); 00557 00558 debug_if(_ism_debug, "ISM43362 dns_lookup: %s ok\n", ip); 00559 return 1; 00560 } 00561 00562 bool ISM43362::send(int id, const void *data, uint32_t amount) 00563 { 00564 // The Size limit has to be checked on caller side. 00565 if (amount > ES_WIFI_MAX_TX_PACKET_SIZE) { 00566 debug_if(_ism_debug, "ISM43362 send: max issue\n"); 00567 return false; 00568 } 00569 00570 /* Activate the socket id in the wifi module */ 00571 if ((id < 0) || (id > 3)) { 00572 return false; 00573 } 00574 if (_active_id != id) { 00575 _active_id = id; 00576 if (!(_parser.send("P0=%d", id) && check_response())) { 00577 debug_if(_ism_debug, "ISM43362 send: P0 issue\n"); 00578 return false; 00579 } 00580 } 00581 00582 /* set Write Transport Packet Size */ 00583 int i = _parser.printf("S3=%d\r", (int)amount); 00584 if (i < 0) { 00585 debug_if(_ism_debug, "ISM43362 send: S3 issue\n"); 00586 return false; 00587 } 00588 i = _parser.write((const char *)data, amount, i); 00589 if (i < 0) { 00590 return false; 00591 } 00592 00593 if (!check_response()) { 00594 return false; 00595 } 00596 00597 debug_if(_ism_debug, "ISM43362 send: id %d amount %d\n", id, amount); 00598 return true; 00599 } 00600 00601 int ISM43362::check_recv_status(int id, void *data) 00602 { 00603 int read_amount; 00604 00605 debug_if(_ism_debug, "ISM43362 check_recv_status: id %d\r\n", id); 00606 00607 /* Activate the socket id in the wifi module */ 00608 if ((id < 0) || (id > 3)) { 00609 debug_if(_ism_debug, "ISM43362 check_recv_status: ERROR with id %d\r\n", id); 00610 return -1; 00611 } 00612 00613 if (_active_id != id) { 00614 _active_id = id; 00615 if (!(_parser.send("P0=%d", id) && check_response())) { 00616 return -1; 00617 } 00618 } 00619 00620 00621 if (!_parser.send("R0")) { 00622 return -1; 00623 } 00624 read_amount = _parser.read((char *)data); 00625 00626 if (read_amount < 0) { 00627 debug_if(_ism_debug, "ISM43362 check_recv_status: ERROR in data RECV, timeout?\r\n"); 00628 return -1; /* nothing to read */ 00629 } 00630 00631 /* If there are spurious 0x15 at the end of the data, this is an error 00632 * we hall can get rid off of them :-( 00633 * This should not happen, but let's try to clean-up anyway 00634 */ 00635 char *cleanup = (char *) data; 00636 while ((read_amount > 0) && (cleanup[read_amount - 1] == 0x15)) { 00637 // debug_if(_ism_debug, "ISM43362 check_recv_status: spurious 0X15 trashed\r\n"); 00638 /* Remove the trailling char then search again */ 00639 read_amount--; 00640 } 00641 00642 if ((read_amount >= 6) && (strncmp("OK\r\n> ", (char *)data, 6) == 0)) { 00643 // debug_if(_ism_debug, "ISM43362 check_recv_status: recv 2 nothing to read=%d\r\n", read_amount); 00644 // read_amount -= 6; 00645 return 0; /* nothing to read */ 00646 } else if ((read_amount >= 8) && (strncmp((char *)((uint32_t) data + read_amount - 8), "\r\nOK\r\n> ", 8)) == 0) { 00647 /* bypass ""\r\nOK\r\n> " if present at the end of the chain */ 00648 read_amount -= 8; 00649 } else { 00650 debug_if(_ism_debug, "ISM43362 check_recv_status: ERROR, flushing %d bytes: ", read_amount); 00651 // for (int i = 0; i < read_amount; i++) { 00652 // debug_if(_ism_debug, "%2X ", cleanup[i]); 00653 // } 00654 // debug_if(_ism_debug, "\r\n (ASCII)", cleanup); 00655 cleanup[read_amount] = 0; 00656 debug_if(_ism_debug, "%s\r\n", cleanup); 00657 return -1; /* nothing to read */ 00658 } 00659 00660 debug_if(_ism_debug, "ISM43362 check_recv_status: id %d read_amount=%d\r\n", id, read_amount); 00661 return read_amount; 00662 } 00663 00664 bool ISM43362::close(int id) 00665 { 00666 if ((id < 0) || (id > 3)) { 00667 debug_if(_ism_debug, "ISM43362: Wrong socket number\n"); 00668 return false; 00669 } 00670 /* Set connection on this socket */ 00671 debug_if(_ism_debug, "ISM43362: CLOSE socket id=%d\n", id); 00672 _active_id = id; 00673 if (!(_parser.send("P0=%d", id) && check_response())) { 00674 return false; 00675 } 00676 /* close this socket */ 00677 if (!(_parser.send("P6=0") && check_response())) { 00678 return false; 00679 } 00680 return true; 00681 } 00682 00683 bool ISM43362::readable() 00684 { 00685 /* not applicable with SPI api */ 00686 return true; 00687 } 00688 00689 bool ISM43362::writeable() 00690 { 00691 /* not applicable with SPI api */ 00692 return true; 00693 } 00694 00695 void ISM43362::attach(Callback<void()> func) 00696 { 00697 /* not applicable with SPI api */ 00698 } 00699
Generated on Tue Jul 12 2022 19:12:12 by
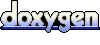