
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_update.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 #include "pal.h" 00018 #include "pal_plat_update.h" 00019 00020 #include "fsl_device_registers.h" 00021 #include "fsl_debug_console.h" 00022 #include "board.h" 00023 #include "clock_config.h" 00024 #include "fsl_flash.h" 00025 00026 00027 00028 00029 #define PAL_UPDATE_JOURNAL_SIZE 0x80000UL 00030 #define PAL_UPDATE_JOURNAL_START_OFFSET 0x80000UL 00031 00032 #if (!defined(PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET)) 00033 #define PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 0x80000UL 00034 #endif 00035 00036 #define SIZEOF_SHA256 256/8 00037 #define FIRMWARE_HEADER_MAGIC 0x5a51b3d4UL 00038 #define FIRMWARE_HEADER_VERSION 1 00039 00040 PAL_PRIVATE FirmwareHeader_t g_palFirmwareHeader; 00041 PAL_PRIVATE bool g_headerWasWritten = false; 00042 00043 PAL_PRIVATE flash_config_t g_flashDriver; 00044 00045 00046 /* 00047 * call back functions 00048 * 00049 */ 00050 00051 PAL_PRIVATE palImageSignalEvent_t g_palUpdateServiceCBfunc; 00052 00053 /* 00054 * WARNING: please do not change this function! 00055 * this function loads a call back function received from the upper layer (service). 00056 * the call back should be called at the end of each function (except pal_plat_imageGetDirectMemAccess) 00057 * the call back receives the event type that just happened defined by the ENUM palImageEvents_t. 00058 * 00059 * if you will not call the call back at the end the service behaver will be undefined 00060 */ 00061 palStatus_t pal_plat_imageInitAPI (palImageSignalEvent_t CBfunction) 00062 { 00063 if (!CBfunction) 00064 { 00065 return PAL_ERR_INVALID_ARGUMENT ; 00066 } 00067 g_palUpdateServiceCBfunc = CBfunction; 00068 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_INIT); 00069 00070 return PAL_SUCCESS; 00071 } 00072 00073 palStatus_t pal_plat_imageDeInit (void) 00074 { 00075 palStatus_t status = PAL_SUCCESS; 00076 return status; 00077 } 00078 00079 00080 palStatus_t pal_plat_imageGetMaxNumberOfImages (uint8_t *imageNumber) 00081 { 00082 return PAL_ERR_NOT_IMPLEMENTED ; 00083 } 00084 00085 palStatus_t pal_plat_imageSetVersion (palImageId_t imageId, const palConstBuffer_t* version) 00086 { 00087 return PAL_ERR_NOT_IMPLEMENTED ; 00088 } 00089 00090 palStatus_t pal_plat_imageGetDirectMemAccess (palImageId_t imageId, void** imagePtr, size_t *imageSizeInBytes) 00091 { 00092 return PAL_ERR_NOT_IMPLEMENTED ; 00093 } 00094 00095 palStatus_t pal_plat_imageActivate (palImageId_t imageId) 00096 { 00097 return PAL_ERR_NOT_IMPLEMENTED ; 00098 } 00099 00100 00101 palStatus_t pal_plat_imageGetActiveHash (palBuffer_t *hash) 00102 { 00103 00104 return PAL_ERR_NOT_IMPLEMENTED ; 00105 } 00106 00107 //Retrieve the version of the active image to version buffer with size set to version bufferLength. 00108 palStatus_t pal_plat_imageGetActiveVersion (palBuffer_t* version) 00109 { 00110 return PAL_ERR_NOT_IMPLEMENTED ; 00111 } 00112 00113 //Writing the value of active image hash stored in hashValue to memory 00114 palStatus_t pal_plat_imageWriteHashToMemory (const palConstBuffer_t* const hashValue) 00115 { 00116 return PAL_ERR_NOT_IMPLEMENTED ; 00117 } 00118 00119 00120 /* 00121 * init apis 00122 */ 00123 00124 00125 00126 //fill the image header data for writing, the writing will occur in the 1st image write 00127 palStatus_t pal_plat_imageSetHeader (palImageId_t imageId,palImageHeaderDeails_t *details) 00128 { 00129 DEBUG_PRINT(">>%s\r\n",__FUNCTION__); 00130 palStatus_t status = PAL_SUCCESS; 00131 g_headerWasWritten = false; // set that the image was not written yet 00132 memset(&g_palFirmwareHeader,0,sizeof(g_palFirmwareHeader)); 00133 g_palFirmwareHeader.totalSize = details->imageSize + sizeof(FirmwareHeader_t); 00134 g_palFirmwareHeader.magic = FIRMWARE_HEADER_MAGIC; 00135 g_palFirmwareHeader.version = FIRMWARE_HEADER_VERSION; 00136 g_palFirmwareHeader.firmwareVersion = details->version; 00137 00138 memcpy(g_palFirmwareHeader.firmwareSHA256,details->hash.buffer,SIZEOF_SHA256); 00139 /* 00140 * calculating and setting the checksum of the header. 00141 * Have to call to crcInit before use. 00142 */ 00143 // crcInit(); 00144 // g_palFirmwareHeader.checksum = crcFast((const unsigned char *)&g_palFirmwareHeader, (int)sizeof(g_palFirmwareHeader)); 00145 return status; 00146 } 00147 00148 00149 00150 palStatus_t pal_plat_imageReserveSpace (palImageId_t imageId, size_t imageSize) 00151 { 00152 status_t result = PAL_SUCCESS; 00153 memset(&g_flashDriver, 0, sizeof(g_flashDriver)); 00154 result = FLASH_Init(&g_flashDriver); 00155 if (kStatus_FLASH_Success != result) 00156 { 00157 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_ERROR); 00158 return PAL_ERR_UPDATE_ERROR ; 00159 } 00160 00161 result = FLASH_Erase(&g_flashDriver, PAL_UPDATE_JOURNAL_START_OFFSET, imageSize, kFLASH_apiEraseKey); 00162 if (kStatus_FLASH_Success != result) 00163 { 00164 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_ERROR); 00165 return PAL_ERR_UPDATE_ERROR ; 00166 } 00167 00168 result = FLASH_VerifyErase(&g_flashDriver, PAL_UPDATE_JOURNAL_START_OFFSET, imageSize, kFLASH_marginValueUser); 00169 if (kStatus_FLASH_Success != result) 00170 { 00171 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_ERROR); 00172 return PAL_ERR_UPDATE_ERROR ; 00173 } 00174 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_PREPARE); 00175 00176 return PAL_SUCCESS; 00177 } 00178 00179 00180 00181 00182 00183 /* 00184 * write API 00185 */ 00186 00187 00188 00189 00190 00191 palStatus_t pal_plat_imageWrite (palImageId_t imageId, size_t offset, palConstBuffer_t *chunk) 00192 { 00193 status_t result = PAL_SUCCESS; 00194 uint32_t failAddr, failDat; 00195 //if header was not written - write header 00196 if (!g_headerWasWritten) 00197 { 00198 result = FLASH_Program( 00199 &g_flashDriver, 00200 PAL_UPDATE_JOURNAL_START_OFFSET, 00201 (uint32_t *)(&g_palFirmwareHeader), 00202 sizeof(g_palFirmwareHeader) 00203 ); 00204 if (kStatus_FLASH_Success != result) 00205 { 00206 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_ERROR); 00207 return PAL_ERR_UPDATE_ERROR ; 00208 } 00209 g_headerWasWritten = true; 00210 } 00211 result = FLASH_Program( 00212 &g_flashDriver, 00213 (PAL_UPDATE_JOURNAL_START_OFFSET + sizeof(g_palFirmwareHeader) + offset), 00214 (uint32_t *)(chunk->buffer), 00215 chunk->bufferLength 00216 ); 00217 if (kStatus_FLASH_Success != result) 00218 { 00219 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_ERROR); 00220 return PAL_ERR_UPDATE_ERROR ; 00221 } 00222 00223 /* Program Check user margin levels */ 00224 result = FLASH_VerifyProgram( 00225 &g_flashDriver, 00226 (PAL_UPDATE_JOURNAL_START_OFFSET + sizeof(g_palFirmwareHeader) + offset), 00227 chunk->bufferLength, 00228 (const uint32_t *)(chunk->buffer), 00229 kFLASH_marginValueUser, 00230 &failAddr, 00231 &failDat 00232 ); 00233 if (kStatus_FLASH_Success != result) 00234 { 00235 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_ERROR); 00236 return PAL_ERR_UPDATE_ERROR ; 00237 } 00238 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_WRITE); 00239 return PAL_SUCCESS; 00240 } 00241 00242 00243 /* 00244 * read APIs 00245 */ 00246 00247 00248 00249 00250 palStatus_t pal_plat_imageReadToBuffer (palImageId_t imageId, size_t offset, palBuffer_t *chunk) 00251 { 00252 uint32_t imageSize = g_palFirmwareHeader.totalSize - sizeof(g_palFirmwareHeader); //totalSize - headerSize 00253 if ((offset + chunk->maxBufferLength) <= imageSize) 00254 { 00255 chunk->bufferLength = chunk->maxBufferLength; 00256 } 00257 else 00258 { 00259 chunk->bufferLength =chunk->maxBufferLength + imageSize - offset; 00260 } 00261 memcpy(chunk->buffer, (void*)(PAL_UPDATE_JOURNAL_START_OFFSET + sizeof(g_palFirmwareHeader) + offset) , chunk->bufferLength); 00262 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_READTOBUFFER); 00263 return PAL_SUCCESS; 00264 } 00265 00266 00267 00268 /* 00269 * commit functions 00270 * */ 00271 00272 00273 00274 palStatus_t pal_plat_imageFlush (palImageId_t package_id) 00275 { 00276 DEBUG_PRINT(">>%s\r\n",__FUNCTION__); 00277 g_palUpdateServiceCBfunc(PAL_IMAGE_EVENT_FINALIZE); 00278 DEBUG_PRINT("<<%s\r\n",__FUNCTION__); 00279 return PAL_SUCCESS; 00280 }
Generated on Tue Jul 12 2022 19:12:14 by
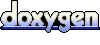