
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_fileSystem.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 #include "pal.h" 00017 #include "pal_plat_fileSystem.h" 00018 #include "pal_plat_rtos.h" 00019 00020 00021 #if defined(__GNUC__) && !defined(__CC_ARM) 00022 #include <sys/stat.h> 00023 #include <sys/types.h> 00024 #endif // defined(__GNUC__) && !defined(__CC_ARM) 00025 #include <stdio.h> 00026 #include <string.h> 00027 #include <stdlib.h> 00028 #include "fsl_mpu.h" 00029 #include "ff.h" 00030 #include "diskio.h" 00031 #include "sdhc_config.h" 00032 00033 00034 #define CHK_FD_VALIDITY(x) ((FIL *)x)->fs->id != ((FIL *)x)->id 00035 #define PAL_FS_ALIGNMENT_TO_SIZE 4 //!< This Size control the number of bytes written to the file (bug fix for writing unaligned memory to file) 00036 #define PAL_FS_COPY_BUFFER_SIZE 256 //!< Size of the chunk to copy files 00037 PAL_PRIVATE BYTE g_platOpenModeConvert[] = 00038 { 00039 0, 00040 FA_READ | FA_OPEN_EXISTING, //"r" 00041 FA_WRITE | FA_READ| FA_OPEN_EXISTING, //"r+" 00042 FA_WRITE | FA_READ| FA_CREATE_NEW, //"w+x" 00043 FA_WRITE | FA_READ| FA_CREATE_ALWAYS //"w+" 00044 }; //!< platform convert table for \b fopen() modes 00045 00046 00047 /*! \brief This function find the next file in a directory 00048 * 00049 * @param[in] *dh - Directory handler to an open DIR 00050 * @param[out] CurrentEntry - entry for the file found in Directory (pre allocated) 00051 * 00052 * \return true - upon successful operation.\n 00053 * 00054 */ 00055 PAL_PRIVATE bool pal_plat_findNextFile(DIR *dh, FILINFO * CurrentEntry); 00056 00057 /*! \brief This function translate the platform errors opcode to pal error codes 00058 * 00059 * @param[in] errorOpCode - platform opcode to be translated 00060 * 00061 * \return PAL_SUCCESS upon successful operation.\n 00062 */ 00063 PAL_PRIVATE palStatus_t pal_plat_errorTranslation (int errorOpCode); 00064 00065 /*! \brief This function build the full path name by adding the filename to the working path given in pathName arg 00066 * 00067 * @param[in] *pathName - pointer to the null-terminated string that specifies the directory name. 00068 * @param[in] *fileName - pointer to the file name 00069 * @param[out] *fullPath - pointer to the full path including the filename (pre allocated) 00070 * 00071 * \return PAL_SUCCESS upon successful operation.\n 00072 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t 00073 * 00074 */ 00075 PAL_PRIVATE palStatus_t pal_plat_addFileNameToPath(const char *pathName, const char * fileName, char * fullPath); 00076 00077 /*! \brief This function copy one file from source folder to destination folder 00078 * 00079 * @param[in] pathNameSrc - Pointer to a null-terminated string that specifies the source dir. 00080 * @param[in] pathNameDest - Pointer to a null-terminated string that specifies the destination dir 00081 * @param[in] fileName - pointer the the file name 00082 * 00083 * \return PAL_SUCCESS upon successful operation.\n 00084 * PAL_FILE_SYSTEM_ERROR - see error code description \c palError_t 00085 * 00086 * \note File should not be open.\n 00087 * If the Destination file exist then it shall be truncated 00088 * 00089 */ 00090 PAL_PRIVATE palStatus_t pal_plat_fsCpFile(const char *pathNameSrc, char *pathNameDest, char * fileName); 00091 00092 palStatus_t pal_plat_fsMkdir(const char *pathName) 00093 { 00094 palStatus_t ret = PAL_SUCCESS; 00095 FRESULT status = FR_OK; 00096 status = f_mkdir(pathName); 00097 if (status != FR_OK) 00098 { 00099 ret = pal_plat_errorTranslation(status); 00100 } 00101 return ret; 00102 } 00103 00104 00105 palStatus_t pal_plat_fsRmdir(const char *pathName) 00106 { 00107 palStatus_t ret = PAL_SUCCESS; 00108 FRESULT status = FR_OK; 00109 00110 status = f_unlink(pathName); 00111 if (status != FR_OK) 00112 { 00113 if ( status == FR_DENIED) 00114 { 00115 ret = PAL_ERR_FS_DIR_NOT_EMPTY; 00116 } 00117 else if (status == FR_NO_FILE) 00118 { 00119 ret = PAL_ERR_FS_NO_PATH; 00120 } 00121 else 00122 { 00123 ret = pal_plat_errorTranslation(status); 00124 } 00125 } 00126 00127 return ret; 00128 } 00129 00130 00131 palStatus_t pal_plat_fsFopen(const char *pathName, pal_fsFileMode_t mode, palFileDescriptor_t *fd) 00132 { 00133 palStatus_t ret = PAL_SUCCESS; 00134 FRESULT status = FR_OK; 00135 FIL * descriptor = NULL; 00136 00137 descriptor = (FIL *)pal_plat_malloc (sizeof(FIL)); 00138 if (descriptor) 00139 { 00140 *fd = (palFileDescriptor_t)descriptor; 00141 status = f_open((FIL*)*fd, pathName, g_platOpenModeConvert[mode]); 00142 if (FR_OK != status) 00143 { 00144 pal_plat_free (descriptor); 00145 if(FR_NO_PATH == status) 00146 { 00147 ret = PAL_ERR_FS_NO_FILE; 00148 } 00149 else 00150 { 00151 ret = pal_plat_errorTranslation(status); 00152 } 00153 } 00154 } 00155 00156 return ret; 00157 } 00158 00159 00160 palStatus_t pal_plat_fsFclose(palFileDescriptor_t *fd) 00161 { 00162 FRESULT status = FR_OK; 00163 palStatus_t ret = PAL_SUCCESS; 00164 00165 00166 if (CHK_FD_VALIDITY(*fd)) 00167 {//Bad File Descriptor 00168 ret = PAL_ERR_FS_BAD_FD; 00169 return ret; 00170 } 00171 00172 status = f_close((FIL *)*fd); 00173 if (FR_OK != status) 00174 { 00175 ret = pal_plat_errorTranslation(status); 00176 } 00177 else 00178 { 00179 pal_plat_free ((void*)*fd); 00180 } 00181 return ret; 00182 } 00183 00184 00185 palStatus_t pal_plat_fsFread(palFileDescriptor_t *fd, void * buffer, size_t numOfBytes, size_t *numberOfBytesRead) 00186 { 00187 palStatus_t ret = PAL_SUCCESS; 00188 FRESULT status = FR_OK; 00189 uint8_t readaligment[PAL_FS_ALIGNMENT_TO_SIZE] = { 0 }; 00190 uint32_t index = 0; 00191 size_t byteRead = 0; 00192 uint8_t leftover = 0; 00193 00194 if (CHK_FD_VALIDITY(*fd)) 00195 {//Bad File Descriptor 00196 ret = PAL_ERR_FS_BAD_FD; 00197 return ret; 00198 } 00199 00200 leftover = numOfBytes % PAL_FS_ALIGNMENT_TO_SIZE; 00201 for(index = 0; index < (numOfBytes / PAL_FS_ALIGNMENT_TO_SIZE); index++) 00202 { 00203 status = f_read((FIL *)*fd, readaligment, PAL_FS_ALIGNMENT_TO_SIZE, &byteRead); 00204 if (FR_OK != status) 00205 { 00206 ret = pal_plat_errorTranslation(status); 00207 break; 00208 } 00209 else 00210 { 00211 memcpy(&((uint8_t *)buffer)[*numberOfBytesRead], readaligment, PAL_FS_ALIGNMENT_TO_SIZE); 00212 *numberOfBytesRead += byteRead; 00213 } 00214 } 00215 00216 if ((ret == PAL_SUCCESS) && (leftover > 0)) 00217 { 00218 status = f_read((FIL *)*fd, readaligment, PAL_FS_ALIGNMENT_TO_SIZE, &byteRead); 00219 if (FR_OK != status) 00220 { 00221 ret = pal_plat_errorTranslation(status); 00222 } 00223 else 00224 { 00225 memcpy(&((uint8_t *)buffer)[*numberOfBytesRead], readaligment, leftover); 00226 *numberOfBytesRead += leftover; 00227 ret = pal_fsFseek(fd, leftover - PAL_FS_ALIGNMENT_TO_SIZE ,PAL_FS_OFFSET_SEEKCUR); 00228 } 00229 } 00230 00231 return ret; 00232 } 00233 00234 00235 palStatus_t pal_plat_fsFwrite(palFileDescriptor_t *fd, const void *buffer, size_t numOfBytes, size_t *numberOfBytesWritten) 00236 { 00237 palStatus_t ret = PAL_SUCCESS; 00238 FRESULT status = FR_OK; 00239 uint32_t index = 0; 00240 size_t bytesWritten = 0; 00241 uint8_t leftover = 0; 00242 00243 if (CHK_FD_VALIDITY(*fd)) 00244 {//Bad File Descriptor 00245 ret = PAL_ERR_FS_BAD_FD; 00246 return ret; 00247 } 00248 00249 leftover = numOfBytes % PAL_FS_ALIGNMENT_TO_SIZE; 00250 for (index = 0; index < (numOfBytes / PAL_FS_ALIGNMENT_TO_SIZE); index++) 00251 { 00252 status = f_write((FIL *)*fd, ((uint8_t *)buffer + *numberOfBytesWritten), PAL_FS_ALIGNMENT_TO_SIZE, &bytesWritten); 00253 if (FR_OK != status) 00254 { 00255 ret = pal_plat_errorTranslation(status); 00256 break; 00257 } 00258 else if (PAL_FS_ALIGNMENT_TO_SIZE != bytesWritten) 00259 { 00260 ret = PAL_ERR_FS_INSUFFICIENT_SPACE; 00261 } 00262 else 00263 { 00264 *numberOfBytesWritten += bytesWritten; 00265 } 00266 } 00267 00268 if ((ret == PAL_SUCCESS) && (leftover > 0)) 00269 { 00270 status = f_write((FIL *)*fd, ((uint8_t *)buffer + *numberOfBytesWritten), leftover, &bytesWritten); 00271 if (FR_OK != status) 00272 { 00273 ret = pal_plat_errorTranslation(status); 00274 } 00275 else if (leftover != bytesWritten) 00276 { 00277 ret = PAL_ERR_FS_INSUFFICIENT_SPACE; 00278 } 00279 else 00280 { 00281 *numberOfBytesWritten += bytesWritten; 00282 } 00283 } 00284 00285 return ret; 00286 } 00287 00288 00289 palStatus_t pal_plat_fsFseek(palFileDescriptor_t *fd, int32_t offset, pal_fsOffset_t whence) 00290 { 00291 palStatus_t ret = PAL_SUCCESS; 00292 FRESULT status = FR_OK; 00293 uint32_t fatFSOffset = 0; 00294 00295 if (CHK_FD_VALIDITY(*fd)) 00296 {//Bad File Descriptor 00297 ret = PAL_ERR_FS_BAD_FD; 00298 return ret; 00299 } 00300 00301 00302 switch(whence) 00303 { 00304 case PAL_FS_OFFSET_SEEKCUR: 00305 if (((-1)*offset > f_tell((FIL*)*fd) && (offset < 0)) || (offset > f_tell((FIL*)*fd) && (offset > 0))) 00306 { 00307 ret = PAL_ERR_FS_ERROR; 00308 } 00309 else 00310 { 00311 fatFSOffset = f_tell((FIL*)*fd) + offset; 00312 } 00313 break; 00314 00315 case PAL_FS_OFFSET_SEEKEND: 00316 if ((-1)*offset > f_size((FIL*)*fd) || (offset > 0)) 00317 { 00318 ret = PAL_ERR_FS_ERROR; 00319 } 00320 else 00321 { 00322 fatFSOffset = f_size((FIL*)*fd) + offset; 00323 } 00324 break; 00325 00326 case PAL_FS_OFFSET_SEEKSET: 00327 if (offset > f_size((FIL*)*fd)) 00328 { 00329 ret = PAL_ERR_FS_ERROR; 00330 } 00331 else 00332 { 00333 fatFSOffset = offset; 00334 } 00335 break; 00336 00337 default: 00338 fatFSOffset = 0; 00339 break; 00340 } 00341 00342 if (ret == PAL_SUCCESS) 00343 { 00344 status = f_lseek ((FIL *)*fd, fatFSOffset); 00345 if (FR_OK != status) 00346 { 00347 ret = pal_plat_errorTranslation(status); 00348 } 00349 } 00350 return ret; 00351 } 00352 00353 00354 palStatus_t pal_plat_fsFtell(palFileDescriptor_t *fd, int32_t * pos) 00355 { 00356 palStatus_t ret = PAL_SUCCESS; 00357 00358 if (CHK_FD_VALIDITY(*fd)) 00359 {//Bad File Descriptor 00360 ret = PAL_ERR_FS_BAD_FD; 00361 } 00362 else 00363 { 00364 *pos = f_tell((FIL*)*fd); 00365 } 00366 return ret; 00367 } 00368 00369 00370 palStatus_t pal_plat_fsUnlink(const char *pathName) 00371 { 00372 palStatus_t ret = PAL_SUCCESS; 00373 FRESULT status = FR_OK; 00374 00375 status = f_unlink(pathName); 00376 if (status != FR_OK) 00377 { 00378 if (status == FR_DENIED) 00379 { 00380 ret = PAL_ERR_FS_DIR_NOT_EMPTY; 00381 } 00382 else 00383 { 00384 ret = pal_plat_errorTranslation(status); 00385 } 00386 } 00387 return ret; 00388 } 00389 00390 00391 palStatus_t pal_plat_fsRmFiles(const char *pathName) 00392 { 00393 DIR dh; //Directory handler 00394 palStatus_t ret = PAL_SUCCESS; 00395 FRESULT status = FR_OK; 00396 char buffer[PAL_MAX_FILE_AND_FOLDER_LENGTH] = {0}; //Buffer for coping the name and folder 00397 FILINFO currentEntry = { 0 }; //file Entry 00398 00399 status = f_opendir(&dh, pathName); 00400 if (status != FR_OK) 00401 { 00402 ret = pal_plat_errorTranslation(status); 00403 } 00404 00405 if (ret == PAL_SUCCESS) 00406 { 00407 while(true) 00408 { 00409 if (!pal_plat_findNextFile(&dh, ¤tEntry)) 00410 { 00411 ret = PAL_ERR_FS_ERROR_IN_SEARCHING; 00412 break; 00413 } 00414 pal_plat_addFileNameToPath(pathName, currentEntry.fname, buffer); 00415 if (currentEntry.fname[0] != '\0') 00416 { 00417 if (currentEntry.fattrib & AM_DIR) 00418 { 00419 ret = pal_fsRmFiles(buffer); 00420 if (ret != PAL_SUCCESS) 00421 { 00422 break; 00423 } 00424 ret = pal_fsRmDir(buffer); 00425 if (PAL_SUCCESS != ret) 00426 { 00427 break; 00428 } 00429 } 00430 else 00431 { 00432 status = f_unlink (buffer); 00433 if (status != FR_OK) 00434 { 00435 ret = pal_plat_errorTranslation(status); 00436 break; 00437 } 00438 } 00439 } 00440 else 00441 {//End of directory reached without errors break, close directory and exit 00442 break; 00443 } 00444 }//while() 00445 00446 status = f_closedir (&dh); //Close DIR handler 00447 if (status != FR_OK) 00448 { 00449 ret = pal_plat_errorTranslation(status); 00450 } 00451 } 00452 00453 return ret; 00454 } 00455 00456 00457 palStatus_t pal_plat_fsCpFolder(const char *pathNameSrc, char *pathNameDest) 00458 { 00459 DIR src_dh; //Directory for the source Directory handler 00460 palStatus_t ret = PAL_SUCCESS; 00461 FILINFO currentEntry = { 0 }; //file Entry 00462 FRESULT status = FR_OK; 00463 00464 00465 status = f_opendir(&src_dh, pathNameSrc); 00466 if (status != FR_OK) 00467 { 00468 ret = pal_plat_errorTranslation(status); 00469 } 00470 00471 00472 if (ret == PAL_SUCCESS) 00473 { 00474 while(true) 00475 { 00476 if (!pal_plat_findNextFile(&src_dh, ¤tEntry)) 00477 { 00478 ret = PAL_ERR_FS_ERROR_IN_SEARCHING; 00479 break; 00480 } 00481 if (currentEntry.fname[0] != 0) 00482 { 00483 if (currentEntry.fattrib & AM_DIR) // skip all folder as this is flat copy only 00484 { 00485 continue; 00486 } 00487 //copy the file to the destination 00488 ret = pal_plat_fsCpFile(pathNameSrc, pathNameDest, currentEntry.fname); 00489 if (ret != PAL_SUCCESS) 00490 { 00491 break; 00492 } 00493 } 00494 else 00495 {//End of directory reached without errors break and close directory and exit 00496 break; 00497 } 00498 }//while() 00499 f_closedir(&src_dh); 00500 } 00501 00502 return ret; 00503 } 00504 00505 00506 PAL_PRIVATE palStatus_t pal_plat_fsCpFile(const char *pathNameSrc, char *pathNameDest, char * fileName) 00507 { 00508 palStatus_t ret = PAL_SUCCESS; 00509 FIL src_fd, dst_fd; 00510 char * buffer = NULL; 00511 char buffer_name[PAL_MAX_FILE_AND_FOLDER_LENGTH] = {0}; //Buffer for coping the name and folder 00512 size_t bytesCount = 0; 00513 FRESULT status = FR_OK; 00514 00515 //Add file name to path 00516 pal_plat_addFileNameToPath(pathNameSrc, fileName, buffer_name); 00517 status = f_open(&src_fd, buffer_name, g_platOpenModeConvert[PAL_FS_FLAG_READONLY]); 00518 if (status != FR_OK) 00519 { 00520 ret = pal_plat_errorTranslation(status); 00521 } 00522 else 00523 { 00524 //Add file name to path 00525 pal_plat_addFileNameToPath(pathNameDest, fileName, buffer_name); 00526 status = f_open(&dst_fd, buffer_name, g_platOpenModeConvert[PAL_FS_FLAG_READWRITETRUNC]); 00527 if (status != FR_OK) 00528 { 00529 ret = pal_plat_errorTranslation(status); 00530 } 00531 else 00532 { 00533 buffer = (char*)pal_plat_malloc (PAL_FS_COPY_BUFFER_SIZE); 00534 if (!buffer) 00535 { 00536 ret = PAL_ERR_RTOS_RESOURCE ; 00537 } 00538 } 00539 } 00540 00541 if (ret == PAL_SUCCESS) 00542 { 00543 while (1) 00544 { 00545 status = f_read(&src_fd, buffer, PAL_FS_COPY_BUFFER_SIZE, &bytesCount); 00546 00547 if (status != FR_OK) 00548 { 00549 break; 00550 } 00551 00552 //Check if end of file reached 00553 if (bytesCount == 0) 00554 { 00555 break; 00556 } 00557 00558 status = f_write(&dst_fd, buffer, bytesCount, &bytesCount); 00559 if (status != FR_OK) 00560 { 00561 break; 00562 } 00563 } 00564 if (status != FR_OK) 00565 { 00566 ret = pal_plat_errorTranslation(status); 00567 } 00568 } 00569 00570 00571 00572 f_close(&src_fd); 00573 f_close(&dst_fd); 00574 if (buffer) 00575 { 00576 pal_plat_free (buffer); 00577 } 00578 return ret; 00579 } 00580 00581 const char* pal_plat_fsGetDefaultRootFolder(pal_fsStorageID_t dataID) 00582 { 00583 const char* returnedRoot = NULL; 00584 if (PAL_FS_PARTITION_PRIMARY == dataID) 00585 { 00586 returnedRoot = PAL_FS_MOUNT_POINT_PRIMARY; 00587 } 00588 else if (PAL_FS_PARTITION_SECONDARY == dataID) 00589 { 00590 returnedRoot = PAL_FS_MOUNT_POINT_SECONDARY; 00591 } 00592 return returnedRoot; 00593 } 00594 00595 00596 PAL_PRIVATE bool pal_plat_findNextFile(DIR *dh, FILINFO *CurrentEntry) 00597 { 00598 bool ret = true; 00599 bool skip = false; 00600 bool foundFile = false; 00601 FRESULT status; 00602 00603 do 00604 { 00605 status = f_readdir(dh, CurrentEntry); 00606 if (status == FR_OK) 00607 { 00608 if ((CurrentEntry)->fname[0] == 0) 00609 {//End Of Directory 00610 ret = true; 00611 break; 00612 } 00613 00614 /* Skip the names "." and ".." as we don't want to remove them. also make sure that the current entry point to REGULER file*/ 00615 skip = (!strcmp((CurrentEntry)->fname, ".")) || (!strcmp((CurrentEntry)->fname, "..")); 00616 if (skip) 00617 { 00618 continue; 00619 } 00620 else 00621 { 00622 foundFile = true; 00623 } 00624 } 00625 else 00626 {//NOT!!! EOF other error 00627 ret = false; 00628 break; //Break from while 00629 } 00630 } 00631 while((!foundFile) && (ret)); //While file has been found or ret is set to false 00632 return ret; 00633 } 00634 00635 PAL_PRIVATE palStatus_t pal_plat_addFileNameToPath(const char *pathName, const char * fileName, char * fullPath) 00636 { 00637 palStatus_t ret = PAL_SUCCESS; 00638 00639 if ((strlen(pathName) >= PAL_MAX_FOLDER_DEPTH_CHAR) || (strlen(fileName) >= PAL_MAX_FULL_FILE_NAME)) 00640 { 00641 ret = PAL_ERR_FS_FILENAME_LENGTH; 00642 } 00643 else if (fullPath) 00644 { 00645 strcpy(fullPath, pathName); 00646 strcat(fullPath, "/"); 00647 strcat(fullPath, fileName); 00648 } 00649 else 00650 { 00651 ret = PAL_ERR_RTOS_RESOURCE ; 00652 } 00653 return ret; 00654 } 00655 00656 00657 PAL_PRIVATE palStatus_t pal_plat_errorTranslation (int errorOpCode) 00658 { 00659 palStatus_t ret = PAL_SUCCESS; 00660 00661 switch(errorOpCode) 00662 { 00663 case 0: 00664 break; 00665 case FR_DENIED: 00666 case FR_WRITE_PROTECTED: 00667 case FR_LOCKED: 00668 ret = PAL_ERR_FS_ACCESS_DENIED; 00669 break; 00670 00671 case FR_NOT_READY : 00672 ret = PAL_ERR_FS_BUSY; 00673 break; 00674 00675 case FR_EXIST: 00676 ret = PAL_ERR_FS_NAME_ALREADY_EXIST; 00677 break; 00678 00679 case FR_INVALID_NAME: 00680 case FR_INVALID_OBJECT: 00681 case FR_INVALID_DRIVE: 00682 ret = PAL_ERR_FS_INVALID_ARGUMENT; 00683 break; 00684 00685 case FR_NO_FILE: 00686 ret = PAL_ERR_FS_NO_FILE; 00687 break; 00688 00689 case FR_NO_PATH: 00690 ret = PAL_ERR_FS_NO_PATH; 00691 break; 00692 00693 default: 00694 ret = PAL_ERR_FS_ERROR; 00695 break; 00696 } 00697 return ret; 00698 } 00699 00700 00701 size_t pal_plat_fsSizeCheck(const char *stringToChk) 00702 { 00703 size_t length = 0; 00704 length = strlen(stringToChk); 00705 return length; 00706 } 00707 00708 00709 00710 palStatus_t pal_plat_fsFormat(pal_fsStorageID_t dataID) 00711 { 00712 const char* partitionNames[] ={ 00713 PAL_FS_MOUNT_POINT_PRIMARY, 00714 PAL_FS_MOUNT_POINT_SECONDARY 00715 }; 00716 palStatus_t result = PAL_SUCCESS; 00717 const char* partName = partitionNames[dataID]; 00718 FRESULT res = f_mkfs(partName, 0, 0); 00719 if (FR_OK != res) 00720 { 00721 result = PAL_ERR_FS_ERROR; 00722 } 00723 return result; 00724 }
Generated on Tue Jul 12 2022 19:12:14 by
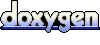