
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
BufferedSpi.h
00001 00002 /** 00003 * @file BufferedSpi.h 00004 * @brief Software Buffer - Extends mbed SPI functionallity 00005 * @author Armelle Duboc 00006 * @version 1.0 00007 * @see 00008 * 00009 * Copyright (c) STMicroelectronics 2017 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); 00012 * you may not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, 00019 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 */ 00023 00024 #ifndef BUFFEREDSPI_H 00025 #define BUFFEREDSPI_H 00026 00027 #include "mbed.h" 00028 #include "MyBuffer.h" 00029 00030 /** A spi port (SPI) for communication with wifi device 00031 * 00032 * Can be used for Full Duplex communication, or Simplex by specifying 00033 * one pin as NC (Not Connected) 00034 * 00035 * Example: 00036 * @code 00037 * #include "mbed.h" 00038 * #include "BufferedSerial.h" 00039 * 00040 * BufferedSerial pc(USBTX, USBRX); 00041 * 00042 * int main() 00043 * { 00044 * while(1) 00045 * { 00046 * Timer s; 00047 * 00048 * s.start(); 00049 * pc.printf("Hello World - buffered\n"); 00050 * int buffered_time = s.read_us(); 00051 * wait(0.1f); // give time for the buffer to empty 00052 * 00053 * s.reset(); 00054 * printf("Hello World - blocking\n"); 00055 * int polled_time = s.read_us(); 00056 * s.stop(); 00057 * wait(0.1f); // give time for the buffer to empty 00058 * 00059 * pc.printf("printf buffered took %d us\n", buffered_time); 00060 * pc.printf("printf blocking took %d us\n", polled_time); 00061 * wait(0.5f); 00062 * } 00063 * } 00064 * @endcode 00065 */ 00066 00067 /** 00068 * @class BufferedSpi 00069 * @brief Software buffers and interrupt driven tx and rx for Serial 00070 */ 00071 class BufferedSpi : public SPI { 00072 private: 00073 DigitalOut nss; 00074 MyBuffer <char> _txbuf; 00075 uint32_t _buf_size; 00076 uint32_t _tx_multiple; 00077 volatile int _timeout; 00078 void txIrq(void); 00079 void prime(void); 00080 00081 InterruptIn *_datareadyInt; 00082 volatile int _cmddata_rdy_rising_event; 00083 void DatareadyRising(void); 00084 int wait_cmddata_rdy_rising_event(void); 00085 int wait_cmddata_rdy_high(void); 00086 00087 00088 Callback<void()> _cbs[2]; 00089 00090 Callback<void()> _sigio_cb; 00091 uint8_t _sigio_event; 00092 00093 public: 00094 MyBuffer <char> _rxbuf; 00095 DigitalIn dataready; 00096 enum IrqType { 00097 RxIrq = 0, 00098 TxIrq, 00099 00100 IrqCnt 00101 }; 00102 00103 /** Create a BufferedSpi Port, connected to the specified transmit and receive pins 00104 * @param SPI mosi pin 00105 * @param SPI miso pin 00106 * @param SPI sclk pin 00107 * @param SPI nss pin 00108 * @param Dataready pin 00109 * @param buf_size printf() buffer size 00110 * @param tx_multiple amount of max printf() present in the internal ring buffer at one time 00111 * @param name optional name 00112 */ 00113 BufferedSpi(PinName mosi, PinName miso, PinName sclk, PinName nss, PinName datareadypin, uint32_t buf_size = 2500, uint32_t tx_multiple = 1, const char *name = NULL); 00114 00115 /** Destroy a BufferedSpi Port 00116 */ 00117 virtual ~BufferedSpi(void); 00118 00119 /** call to SPI frequency Function 00120 */ 00121 virtual void frequency(int hz); 00122 00123 /** clear the transmit buffer 00124 */ 00125 virtual void flush_txbuf(void); 00126 00127 /** call to SPI format function 00128 */ 00129 virtual void format(int bits, int mode); 00130 00131 virtual void enable_nss(void); 00132 00133 virtual void disable_nss(void); 00134 00135 /** Check on how many bytes are in the rx buffer 00136 * @return 1 if something exists, 0 otherwise 00137 */ 00138 virtual int readable(void); 00139 00140 /** Check to see if the tx buffer has room 00141 * @return 1 always has room and can overwrite previous content if too small / slow 00142 */ 00143 virtual int writeable(void); 00144 00145 /** Get a single byte from the BufferedSpi Port. 00146 * Should check readable() before calling this. 00147 * @return A byte that came in on the SPI Port 00148 */ 00149 virtual int getc(void); 00150 00151 /** Write a single byte to the BufferedSpi Port. 00152 * @param c The byte to write to the SPI Port 00153 * @return The byte that was written to the SPI Port Buffer 00154 */ 00155 virtual int putc(int c); 00156 00157 /** Write a string to the BufferedSpi Port. Must be NULL terminated 00158 * @param s The string to write to the Spi Port 00159 * @return The number of bytes written to the Spi Port Buffer 00160 */ 00161 virtual int puts(const char *s); 00162 00163 /** Write a formatted string to the BufferedSpi Port. 00164 * @param format The string + format specifiers to write to the Spi Port 00165 * @return The number of bytes written to the Spi Port Buffer 00166 */ 00167 virtual int printf(const char *format, ...); 00168 00169 /** Write data to the Buffered Spi Port 00170 * @param s A pointer to data to send 00171 * @param length The amount of data being pointed to 00172 * @return The number of bytes written to the Spi Port Buffer 00173 */ 00174 virtual ssize_t buffwrite(const void *s, std::size_t length); 00175 00176 /** Send datas to the Spi port that are already present 00177 * in the internal _txbuffer 00178 * @param length 00179 * @return the number of bytes written on the SPI port 00180 */ 00181 virtual ssize_t buffsend(size_t length); 00182 00183 /** Read data from the Spi Port to the _rxbuf 00184 * @param max: optional. = max sieze of the input read 00185 * @return The number of bytes read from the SPI port and written to the _rxbuf 00186 */ 00187 virtual ssize_t read(); 00188 virtual ssize_t read(uint32_t max); 00189 00190 /** 00191 * Allows timeout to be changed between commands 00192 * 00193 * @param timeout timeout of the connection in ms 00194 */ 00195 void setTimeout(int timeout) 00196 { 00197 /* this is a safe guard timeout at SPI level in case module is stuck */ 00198 _timeout = timeout; 00199 } 00200 00201 /** Register a callback once any data is ready for sockets 00202 * @param func Function to call on state change 00203 */ 00204 virtual void sigio(Callback<void()> func); 00205 00206 /** Attach a function to call whenever a serial interrupt is generated 00207 * @param func A pointer to a void function, or 0 to set as none 00208 * @param type Which serial interrupt to attach the member function to (Serial::RxIrq for receive, TxIrq for transmit buffer empty) 00209 */ 00210 virtual void attach(Callback<void()> func, IrqType type = RxIrq); 00211 00212 /** Attach a member function to call whenever a serial interrupt is generated 00213 * @param obj pointer to the object to call the member function on 00214 * @param method pointer to the member function to call 00215 * @param type Which serial interrupt to attach the member function to (Serial::RxIrq for receive, TxIrq for transmit buffer empty) 00216 */ 00217 template <typename T> 00218 void attach(T *obj, void (T::*method)(), IrqType type = RxIrq) 00219 { 00220 attach(Callback<void()>(obj, method), type); 00221 } 00222 00223 /** Attach a member function to call whenever a serial interrupt is generated 00224 * @param obj pointer to the object to call the member function on 00225 * @param method pointer to the member function to call 00226 * @param type Which serial interrupt to attach the member function to (Serial::RxIrq for receive, TxIrq for transmit buffer empty) 00227 */ 00228 template <typename T> 00229 void attach(T *obj, void (*method)(T *), IrqType type = RxIrq) 00230 { 00231 attach(Callback<void()>(obj, method), type); 00232 } 00233 }; 00234 #endif
Generated on Tue Jul 12 2022 19:12:11 by
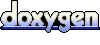