
bug fix
Embed:
(wiki syntax)
Show/hide line numbers
pal_network.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 00018 #include "pal.h" 00019 #include "pal_network.h" 00020 #include "pal_plat_network.h" 00021 00022 typedef struct pal_in_addr { 00023 uint32_t s_addr; // that's a 32-bit int (4 bytes) 00024 } pal_in_addr_t; 00025 00026 #if PAL_SUPPORT_IP_V4 00027 typedef struct pal_socketAddressInternal { 00028 short int pal_sin_family; // address family 00029 unsigned short int pal_sin_port; // port 00030 pal_in_addr_t pal_sin_addr; // ipv4 address 00031 unsigned char pal_sin_zero[8]; // 00032 } pal_socketAddressInternal_t; 00033 #endif 00034 00035 #if PAL_SUPPORT_IP_V6 00036 typedef struct pal_socketAddressInternal6{ 00037 uint16_t pal_sin6_family; // address family, 00038 uint16_t pal_sin6_port; // port number, Network Byte Order 00039 uint32_t pal_sin6_flowinfo; // IPv6 flow information 00040 palIpV6Addr_t pal_sin6_addr; // IPv6 address 00041 uint32_t pal_sin6_scope_id; // Scope ID 00042 } pal_socketAddressInternal6_t; 00043 #endif 00044 00045 #if PAL_NET_DNS_SUPPORT 00046 00047 // structure used by pal_getAddressInfoAsync 00048 #ifndef PAL_DNS_API_V2 00049 typedef struct pal_asyncAddressInfo 00050 { 00051 char* url; 00052 palSocketAddress_t* address; 00053 palSocketLength_t* addressLength; 00054 palGetAddressInfoAsyncCallback_t callback; 00055 void* callbackArgument; 00056 } pal_asyncAddressInfo_t ; 00057 #endif // PAL_DNS_API_V2 00058 #endif // PAL_NET_DNS_SUPPORT 00059 00060 palStatus_t pal_registerNetworkInterface(void* networkInterfaceContext, uint32_t* interfaceIndex) 00061 { 00062 PAL_VALIDATE_ARGUMENTS((networkInterfaceContext == NULL) || (interfaceIndex == NULL)); 00063 palStatus_t result = pal_plat_registerNetworkInterface (networkInterfaceContext, interfaceIndex);; 00064 00065 return result; 00066 } 00067 00068 palStatus_t pal_setSockAddrPort(palSocketAddress_t* address, uint16_t port) 00069 { 00070 palStatus_t result = PAL_SUCCESS; 00071 bool found = false; 00072 PAL_VALIDATE_ARGUMENTS(NULL == address); 00073 00074 #if PAL_SUPPORT_IP_V4 00075 if (address->addressType == PAL_AF_INET) 00076 { 00077 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00078 // Set Linux format 00079 innerAddr->pal_sin_port = PAL_HTONS(port); 00080 found = true; 00081 } 00082 #endif 00083 00084 #if PAL_SUPPORT_IP_V6 00085 if (address->addressType == PAL_AF_INET6 ) 00086 { 00087 pal_socketAddressInternal6_t * innerAddr = (pal_socketAddressInternal6_t*)address; 00088 // Set Linux format 00089 innerAddr->pal_sin6_port = PAL_HTONS(port); 00090 found = true; 00091 } 00092 #endif 00093 if (false == found) 00094 { 00095 result = PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00096 } 00097 00098 return result; 00099 } 00100 00101 00102 00103 #if PAL_SUPPORT_IP_V4 00104 palStatus_t pal_setSockAddrIPV4Addr(palSocketAddress_t* address, palIpV4Addr_t ipV4Addr) 00105 { 00106 PAL_VALIDATE_ARGUMENTS((NULL == address) || (NULL == ipV4Addr)); 00107 00108 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00109 innerAddr->pal_sin_family = PAL_AF_INET; 00110 innerAddr->pal_sin_addr.s_addr = (ipV4Addr[0]) | (ipV4Addr[1] << 8) | (ipV4Addr[2] << 16) | (ipV4Addr[3] << 24); 00111 return PAL_SUCCESS; 00112 } 00113 00114 palStatus_t pal_getSockAddrIPV4Addr(const palSocketAddress_t* address, palIpV4Addr_t ipV4Addr) 00115 { 00116 PAL_VALIDATE_ARGUMENTS(NULL == address); 00117 PAL_VALIDATE_CONDITION_WITH_ERROR((address->addressType != PAL_AF_INET),PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ); 00118 palStatus_t result = PAL_SUCCESS; 00119 00120 if (address->addressType == PAL_AF_INET) 00121 { 00122 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00123 ipV4Addr[0] = (innerAddr->pal_sin_addr.s_addr) & 0xFF; 00124 ipV4Addr[1] = (innerAddr->pal_sin_addr.s_addr >> 8) & 0xFF; 00125 ipV4Addr[2] = (innerAddr->pal_sin_addr.s_addr >> 16) & 0xFF; 00126 ipV4Addr[3] = (innerAddr->pal_sin_addr.s_addr >> 24) & 0xFF; 00127 00128 } 00129 00130 return result; 00131 } 00132 #else 00133 palStatus_t pal_setSockAddrIPV4Addr(palSocketAddress_t* address, palIpV4Addr_t ipV4Addr) 00134 { 00135 return PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00136 } 00137 palStatus_t pal_getSockAddrIPV4Addr(const palSocketAddress_t* address, palIpV4Addr_t ipV4Addr) 00138 { 00139 return PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00140 } 00141 00142 #endif 00143 00144 00145 #if PAL_SUPPORT_IP_V6 00146 palStatus_t pal_getSockAddrIPV6Addr(const palSocketAddress_t* address, palIpV6Addr_t ipV6Addr) 00147 { 00148 palStatus_t result = PAL_SUCCESS; 00149 int index = 0; 00150 PAL_VALIDATE_ARGUMENTS (NULL == address); 00151 PAL_VALIDATE_CONDITION_WITH_ERROR((address->addressType != PAL_AF_INET6 ),PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ); 00152 00153 pal_socketAddressInternal6_t * innerAddr = (pal_socketAddressInternal6_t*)address; 00154 for (index = 0; index < PAL_IPV6_ADDRESS_SIZE; index++) // TODO: use mem copy? 00155 { 00156 ipV6Addr[index] = innerAddr->pal_sin6_addr[index]; 00157 } 00158 00159 00160 return result; 00161 } 00162 00163 palStatus_t pal_setSockAddrIPV6Addr(palSocketAddress_t* address, palIpV6Addr_t ipV6Addr) 00164 { 00165 int index; 00166 PAL_VALIDATE_ARGUMENTS((NULL == address) || (NULL == ipV6Addr)); 00167 00168 pal_socketAddressInternal6_t* innerAddr = (pal_socketAddressInternal6_t*)address; 00169 innerAddr->pal_sin6_family = PAL_AF_INET6 ; 00170 for (index = 0; index < PAL_IPV6_ADDRESS_SIZE; index++) // TODO: use mem copy? 00171 { 00172 innerAddr->pal_sin6_addr[index] = ipV6Addr[index]; 00173 } 00174 return PAL_SUCCESS; 00175 } 00176 #else 00177 palStatus_t pal_setSockAddrIPV6Addr(palSocketAddress_t* address, palIpV6Addr_t ipV6Addr) 00178 { 00179 return PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00180 } 00181 00182 palStatus_t pal_getSockAddrIPV6Addr(const palSocketAddress_t* address, palIpV6Addr_t ipV6Addr) 00183 { 00184 return PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00185 } 00186 00187 #endif 00188 00189 00190 palStatus_t pal_getSockAddrPort(const palSocketAddress_t* address, uint16_t* port) 00191 { 00192 bool found = false; 00193 palStatus_t result = PAL_SUCCESS; 00194 00195 PAL_VALIDATE_ARGUMENTS ((NULL == address) || (NULL == port)); 00196 00197 #if PAL_SUPPORT_IP_V4 00198 00199 if (address->addressType == PAL_AF_INET) 00200 { 00201 pal_socketAddressInternal_t* innerAddr = (pal_socketAddressInternal_t*)address; 00202 // Set numeric formal 00203 *port = PAL_NTOHS(innerAddr->pal_sin_port); 00204 found = true; 00205 } 00206 #endif 00207 #if PAL_SUPPORT_IP_V6 00208 if (address->addressType == PAL_AF_INET6 ) 00209 { 00210 pal_socketAddressInternal6_t * innerAddr = (pal_socketAddressInternal6_t*)address; 00211 // Set numeric formal 00212 *port = PAL_NTOHS(innerAddr->pal_sin6_port); 00213 found = true; 00214 } 00215 #endif 00216 if (false == found) 00217 { 00218 result = PAL_ERR_SOCKET_INVALID_ADDRESS_FAMILY ; 00219 } 00220 00221 return result; 00222 } 00223 00224 00225 palStatus_t pal_socket(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palSocket_t* socket) 00226 { 00227 PAL_VALIDATE_ARGUMENTS (NULL == socket); 00228 00229 palStatus_t result = pal_plat_socket (domain, type, nonBlockingSocket, interfaceNum, socket);; 00230 00231 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00232 } 00233 00234 00235 palStatus_t pal_setSocketOptions(palSocket_t socket, int optionName, const void* optionValue, palSocketLength_t optionLength) 00236 { 00237 00238 PAL_VALIDATE_ARGUMENTS (NULL == optionValue); 00239 00240 palStatus_t result = PAL_SUCCESS; 00241 result = pal_plat_setSocketOptions ( socket, optionName, optionValue, optionLength); 00242 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00243 } 00244 00245 palStatus_t pal_isNonBlocking(palSocket_t socket, bool* isNonBlocking) 00246 { 00247 PAL_VALIDATE_ARGUMENTS (NULL == isNonBlocking); 00248 00249 palStatus_t result = pal_plat_isNonBlocking (socket, isNonBlocking);; 00250 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00251 } 00252 00253 00254 palStatus_t pal_bind(palSocket_t socket, palSocketAddress_t* myAddress, palSocketLength_t addressLength) 00255 { 00256 00257 PAL_VALIDATE_ARGUMENTS(NULL == myAddress); 00258 00259 palStatus_t result = PAL_SUCCESS; 00260 result = pal_plat_bind (socket, myAddress, addressLength); 00261 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00262 } 00263 00264 00265 palStatus_t pal_receiveFrom(palSocket_t socket, void* buffer, size_t length, palSocketAddress_t* from, palSocketLength_t* fromLength, size_t* bytesReceived) 00266 { 00267 00268 PAL_VALIDATE_ARGUMENTS((NULL == buffer) || (NULL == bytesReceived)); 00269 00270 palStatus_t result = PAL_SUCCESS; 00271 result = pal_plat_receiveFrom (socket, buffer, length, from, fromLength, bytesReceived); 00272 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00273 } 00274 00275 00276 palStatus_t pal_sendTo(palSocket_t socket, const void* buffer, size_t length, const palSocketAddress_t* to, palSocketLength_t toLength, size_t* bytesSent) 00277 { 00278 00279 PAL_VALIDATE_ARGUMENTS((NULL == buffer) || (NULL == bytesSent) || (NULL == to)); 00280 00281 palStatus_t result = PAL_SUCCESS; 00282 result = pal_plat_sendTo (socket, buffer, length, to, toLength, bytesSent); 00283 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00284 } 00285 00286 00287 palStatus_t pal_close(palSocket_t* socket) 00288 { 00289 00290 PAL_VALIDATE_ARGUMENTS(NULL == socket); 00291 00292 palStatus_t result = PAL_SUCCESS; 00293 result = pal_plat_close (socket); 00294 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00295 } 00296 00297 00298 palStatus_t pal_getNumberOfNetInterfaces( uint32_t* numInterfaces) 00299 { 00300 00301 PAL_VALIDATE_ARGUMENTS(NULL == numInterfaces); 00302 00303 palStatus_t result = PAL_SUCCESS; 00304 result = pal_plat_getNumberOfNetInterfaces (numInterfaces); 00305 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00306 } 00307 00308 00309 palStatus_t pal_getNetInterfaceInfo(uint32_t interfaceNum, palNetInterfaceInfo_t * interfaceInfo) 00310 { 00311 PAL_VALIDATE_ARGUMENTS(NULL == interfaceInfo) 00312 00313 palStatus_t result = PAL_SUCCESS; 00314 result = pal_plat_getNetInterfaceInfo (interfaceNum, interfaceInfo); 00315 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00316 } 00317 00318 00319 #if PAL_NET_TCP_AND_TLS_SUPPORT // functionality below supported only in case TCP is supported. 00320 00321 palStatus_t pal_listen(palSocket_t socket, int backlog) 00322 { 00323 palStatus_t result = pal_plat_listen (socket, backlog); 00324 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00325 } 00326 00327 00328 palStatus_t pal_accept(palSocket_t socket, palSocketAddress_t* address, palSocketLength_t* addressLen, palSocket_t* acceptedSocket) 00329 { 00330 00331 PAL_VALIDATE_ARGUMENTS ((NULL == acceptedSocket) || (NULL == address)|| (NULL == addressLen)); 00332 00333 palStatus_t result = PAL_SUCCESS; 00334 result = pal_plat_accept (socket, address, addressLen, acceptedSocket); 00335 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00336 } 00337 00338 00339 palStatus_t pal_connect(palSocket_t socket, const palSocketAddress_t* address, palSocketLength_t addressLen) 00340 { 00341 PAL_VALIDATE_ARGUMENTS(NULL == address); 00342 00343 palStatus_t result = PAL_SUCCESS; 00344 00345 result = pal_plat_connect ( socket, address, addressLen); 00346 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00347 } 00348 00349 00350 palStatus_t pal_recv(palSocket_t socket, void* buf, size_t len, size_t* recievedDataSize) 00351 { 00352 PAL_VALIDATE_ARGUMENTS((NULL == recievedDataSize) || (NULL == buf)); 00353 00354 palStatus_t result = PAL_SUCCESS; 00355 result = pal_plat_recv (socket, buf, len, recievedDataSize); 00356 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00357 } 00358 00359 00360 palStatus_t pal_send(palSocket_t socket, const void* buf, size_t len, size_t* sentDataSize) 00361 { 00362 00363 PAL_VALIDATE_ARGUMENTS((NULL == buf) || (NULL == sentDataSize)); 00364 00365 palStatus_t result = PAL_SUCCESS; 00366 result = pal_plat_send ( socket, buf, len, sentDataSize); 00367 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00368 } 00369 00370 00371 #endif //PAL_NET_TCP_AND_TLS_SUPPORT 00372 00373 00374 #if PAL_NET_ASYNCHRONOUS_SOCKET_API 00375 00376 palStatus_t pal_asynchronousSocket(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback, palSocket_t* socket) 00377 { 00378 PAL_VALIDATE_ARGUMENTS((NULL == socket) || (NULL == callback)); 00379 00380 palStatus_t result = PAL_SUCCESS; 00381 result = pal_plat_asynchronousSocket (domain, type, nonBlockingSocket, interfaceNum, callback, NULL, socket); 00382 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00383 } 00384 00385 palStatus_t pal_asynchronousSocketWithArgument(palSocketDomain_t domain, palSocketType_t type, bool nonBlockingSocket, uint32_t interfaceNum, palAsyncSocketCallback_t callback, void* callbackArgument, palSocket_t* socket) 00386 { 00387 PAL_VALIDATE_ARGUMENTS((NULL == socket) || (NULL == callback)); 00388 00389 palStatus_t result = PAL_SUCCESS; 00390 result = pal_plat_asynchronousSocket (domain, type, nonBlockingSocket, interfaceNum, callback, callbackArgument, socket); 00391 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00392 } 00393 00394 #endif 00395 00396 #if PAL_NET_DNS_SUPPORT 00397 00398 palStatus_t pal_getAddressInfo(const char *url, palSocketAddress_t *address, palSocketLength_t* addressLength) 00399 { 00400 PAL_VALIDATE_ARGUMENTS ((NULL == url) || (NULL == address) || (NULL == addressLength)); 00401 00402 palStatus_t result = PAL_SUCCESS; 00403 result = pal_plat_getAddressInfo (url, address, addressLength); 00404 return result; // TODO(nirson01) ADD debug print for error propagation(once debug print infrastructure is finalized) 00405 } 00406 00407 // the function invoked by the thread created in pal_getAddressInfoAsync 00408 #ifndef PAL_DNS_API_V2 00409 PAL_PRIVATE void getAddressInfoAsyncThreadFunc(void const* arg) 00410 { 00411 pal_asyncAddressInfo_t* info = (pal_asyncAddressInfo_t*)arg; 00412 palStatus_t status = pal_getAddressInfo(info->url, info->address, info->addressLength); 00413 if (PAL_SUCCESS != status) 00414 { 00415 PAL_LOG(ERR, "getAddressInfoAsyncThreadFunc: pal_getAddressInfo failed\n"); 00416 } 00417 info->callback(info->url, info->address, info->addressLength, status, info->callbackArgument); // invoke callback 00418 free(info); 00419 } 00420 00421 palStatus_t pal_getAddressInfoAsync(const char* url, 00422 palSocketAddress_t* address, 00423 palSocketLength_t* addressLength, 00424 palGetAddressInfoAsyncCallback_t callback, 00425 void* callbackArgument) 00426 { 00427 PAL_VALIDATE_ARGUMENTS ((NULL == url) || (NULL == address) || (NULL == addressLength) || (NULL == callback)) 00428 00429 palStatus_t status; 00430 palThreadID_t threadID = NULLPTR; 00431 00432 pal_asyncAddressInfo_t* info = (pal_asyncAddressInfo_t*)malloc(sizeof(pal_asyncAddressInfo_t)); // thread function argument allocation 00433 if (NULL == info) { 00434 status = PAL_ERR_NO_MEMORY ; 00435 } 00436 else { 00437 info->url = (char*)url; 00438 info->address = address; 00439 info->addressLength = addressLength; 00440 info->callback = callback; 00441 info->callbackArgument = callbackArgument; 00442 00443 status = pal_osThreadCreateWithAlloc(getAddressInfoAsyncThreadFunc, info, PAL_osPriorityReservedDNS, PAL_NET_ASYNC_DNS_THREAD_STACK_SIZE, NULL, &threadID); 00444 if (PAL_SUCCESS != status) { 00445 free(info); // free memory allocation in case thread creation failed 00446 } 00447 } 00448 return status; 00449 } 00450 #else 00451 #ifndef TARGET_LIKE_MBED 00452 #error "PAL_DNS_API_V2 is only supported with mbed-os" 00453 #endif 00454 palStatus_t pal_getAddressInfoAsync(const char* url, 00455 palSocketAddress_t* address, 00456 palGetAddressInfoAsyncCallback_t callback, 00457 void* callbackArgument, 00458 palDNSQuery_t* queryHandle) 00459 { 00460 PAL_VALIDATE_ARGUMENTS ((NULL == url) || (NULL == address) || (NULL == callback)) 00461 00462 palStatus_t status; 00463 00464 pal_asyncAddressInfo_t* info = (pal_asyncAddressInfo_t*)malloc(sizeof(pal_asyncAddressInfo_t)); 00465 if (NULL == info) { 00466 status = PAL_ERR_NO_MEMORY ; 00467 } 00468 else { 00469 info->url = (char*)url; 00470 info->address = address; 00471 info->callback = callback; 00472 info->callbackArgument = callbackArgument; 00473 info->queryHandle = queryHandle; 00474 status = pal_plat_getAddressInfoAsync (info); 00475 if (status != PAL_SUCCESS) { 00476 free(info); 00477 } 00478 } 00479 return status; 00480 } 00481 00482 palStatus_t pal_cancelAddressInfoAsync(palDNSQuery_t queryHandle) 00483 { 00484 return pal_plat_cancelAddressInfoAsync (queryHandle); 00485 } 00486 #endif // #ifndef PAL_DNS_API_V2 00487 00488 #endif // PAL_NET_DNS_SUPPORT
Generated on Tue Jul 12 2022 21:04:57 by
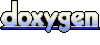