
bug fix
Embed:
(wiki syntax)
Show/hide line numbers
pal_internalFlash.h
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 #ifndef PAL_FLASH_H 00017 #define PAL_FLASH_H 00018 00019 #ifdef __cplusplus 00020 extern "C" { 00021 #endif 00022 00023 00024 #ifndef _PAL_H 00025 #error "Please do not include this file directly, use pal.h instead" 00026 #endif 00027 00028 00029 00030 #define PAL_INT_FLASH_BLANK_VAL 0xFF 00031 00032 00033 /*! \brief This function initialized the flash API module, 00034 * And should be called prior flash APIs calls 00035 * 00036 * \return PAL_SUCCESS upon successful operation. \n 00037 * PAL_FILE_SYSTEM_ERROR - see error code \c palError_t. 00038 * 00039 * \note should be called only once unless \c pal_internalFlashDeinit function is called 00040 * \note This function is Blocking till completion!! 00041 * 00042 */ 00043 palStatus_t pal_internalFlashInit(void); 00044 00045 /*! \brief This function destroy the flash module 00046 * 00047 * \return PAL_SUCCESS upon successful operation. \n 00048 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00049 * 00050 * \note Should be called only after \c pal_internalFlashinit() is called. 00051 * \note Flash APIs will not work after calling this function 00052 * \note This function is Blocking till completion!! 00053 * 00054 */ 00055 palStatus_t pal_internalFlashDeInit(void); 00056 00057 /*! \brief This function writes to the internal flash 00058 * 00059 * @param[in] buffer - pointer to the buffer to be written 00060 * @param[in] size - the size of the buffer in bytes. 00061 * @param[in] address - the address of the internal flash, must be aligned to minimum writing unit (page size). 00062 * 00063 * \return PAL_SUCCESS upon successful operation. \n 00064 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00065 * 00066 * \note ALL address can be written to!! No protection to boot loader, program or other... 00067 * \note This function is Blocking till completion!! 00068 * \note This function is Thread Safe!! 00069 */ 00070 palStatus_t pal_internalFlashWrite(const size_t size, const uint32_t address, const uint32_t * buffer); 00071 00072 /*! \brief This function copies the memory data into the user given buffer 00073 * 00074 * @param[in] size - the size of the buffer in bytes. 00075 * @param[in] address - the address of the internal flash. 00076 * @param[out] buffer - pointer to the buffer to write to 00077 * 00078 * \return PAL_SUCCESS upon successful operation. \n 00079 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00080 * \note This function is Blocking till completion!! 00081 * \note This function is Thread Safe!! 00082 * 00083 */ 00084 palStatus_t pal_internalFlashRead(const size_t size, const uint32_t address, uint32_t * buffer); 00085 00086 /*! \brief This function Erase the sector 00087 * 00088 * @param[in] size - the size to be erased 00089 * @param[in] address - sector start address to be erased, must be align to sector. 00090 * 00091 * \return PAL_SUCCESS upon successful operation. \n 00092 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00093 * 00094 * \note ALL sectors can be erased!! No protection to bootloader, program or other... 00095 * \note This function is Blocking till completion!! 00096 * \note Only one sector can be erased in each function call 00097 * \note This function is Thread Safe!! 00098 */ 00099 palStatus_t pal_internalFlashErase(uint32_t address, size_t size); 00100 00101 00102 /*! \brief This function returns the minimum writing unit to the flash 00103 * 00104 * \return size_t the 2, 4, 8.... 00105 */ 00106 size_t pal_internalFlashGetPageSize(void); 00107 00108 00109 /*! \brief This function returns the sector size for the given address 00110 * 00111 * @param[in] the starting address of the sector is question 00112 * 00113 * \return size of sector, 0 if error 00114 */ 00115 size_t pal_internalFlashGetSectorSize(uint32_t address); 00116 00117 00118 /////////////////////////////////////////////////////////////// 00119 ////-------------------SOTP functions------------------------// 00120 /////////////////////////////////////////////////////////////// 00121 00122 /*! \brief This function return the SOTP section data 00123 * 00124 * @param[in] section - the section number (0 or 1) 00125 * @param[out] data - the information about the section 00126 * 00127 * \return PAL_SUCCESS upon successful operation. \n 00128 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00129 * 00130 */ 00131 palStatus_t pal_internalFlashGetAreaInfo(uint8_t section, palSotpAreaData_t *data); 00132 00133 #ifdef __cplusplus 00134 } 00135 #endif 00136 #endif //PAL_FLASH_H
Generated on Tue Jul 12 2022 21:04:57 by
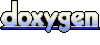