
bug fix
Embed:
(wiki syntax)
Show/hide line numbers
m2mtimer.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_TIMER_H 00017 #define M2M_TIMER_H 00018 00019 #include <stdint.h> 00020 #include "mbed-client/m2mtimerobserver.h" 00021 00022 class M2MTimerPimpl; 00023 00024 /*! \file m2mtimer.h 00025 * \brief M2MTimer. 00026 * Timer class for mbed client. 00027 */ 00028 class M2MTimer 00029 { 00030 private: 00031 // Prevents the use of assignment operator 00032 M2MTimer& operator=(const M2MTimer& other); 00033 00034 // Prevents the use of copy constructor 00035 M2MTimer(const M2MTimer& other); 00036 00037 public: 00038 00039 /** 00040 * Constructor. 00041 */ 00042 M2MTimer(M2MTimerObserver& observer); 00043 00044 /** 00045 * Destructor. 00046 */ 00047 ~M2MTimer(); 00048 00049 /** 00050 * \brief Starts the timer. 00051 * \param interval The timer interval in milliseconds. 00052 * \param single_shot Defines whether the timer is ticked once or restarted every time at expiry. 00053 */ 00054 void start_timer(uint64_t interval, M2MTimerObserver::Type type, bool single_shot = true); 00055 00056 /** 00057 * \brief Starts the timer in DTLS manner. 00058 * \param intermediate_interval The intermediate interval to use, must be smaller than total (usually 1/4 of total). 00059 * \param total_interval The total interval to use; This is the timeout value of a DTLS packet. 00060 * \param type The type of the timer. 00061 */ 00062 void start_dtls_timer(uint64_t intermediate_interval, uint64_t total_interval, M2MTimerObserver::Type type = M2MTimerObserver::Dtls); 00063 00064 /** 00065 * \brief Stops the timer. 00066 * This cancels the ongoing timer. 00067 */ 00068 void stop_timer(); 00069 00070 /** 00071 * \brief Checks if the intermediate interval has passed. 00072 * \return True if the interval has passed, else false. 00073 */ 00074 bool is_intermediate_interval_passed(); 00075 00076 /** 00077 * \brief Checks if the total interval has passed. 00078 * \return True if the interval has passed, else false. 00079 */ 00080 bool is_total_interval_passed(); 00081 00082 private: 00083 00084 M2MTimerObserver& _observer; 00085 M2MTimerPimpl *_private_impl; 00086 friend class Test_M2MTimerImpl_linux; 00087 }; 00088 00089 #endif // M2M_TIMER_H
Generated on Tue Jul 12 2022 21:04:56 by
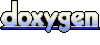