
bug fix
Embed:
(wiki syntax)
Show/hide line numbers
m2mserver.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_SERVER_H 00017 #define M2M_SERVER_H 00018 00019 #include "mbed-client/m2mobject.h" 00020 00021 // FORWARD DECLARATION 00022 class M2MResource; 00023 00024 /*! \file m2mserver.h 00025 * \brief M2MServer. 00026 * This class represents an interface for the Server Object model of the LWM2M framework. 00027 * It handles the server object and all its corresponding 00028 * resources. 00029 */ 00030 00031 class M2MServer : public M2MObject 00032 { 00033 00034 friend class M2MInterfaceFactory; 00035 friend class M2MNsdlInterface; 00036 00037 public: 00038 00039 /** 00040 * \brief Am enum defining all resources associated with 00041 * a Server Object in the LWM2M framework. 00042 */ 00043 typedef enum { 00044 ShortServerID, 00045 Lifetime, 00046 DefaultMinPeriod, 00047 DefaultMaxPeriod, 00048 Disable, 00049 DisableTimeout, 00050 NotificationStorage, 00051 Binding, 00052 RegistrationUpdate 00053 }ServerResource; 00054 00055 private: 00056 00057 /** 00058 * \brief Constructor 00059 */ 00060 M2MServer(); 00061 00062 00063 // Prevents the use of assignment operator. 00064 M2MServer& operator=( const M2MServer& /*other*/ ); 00065 00066 // Prevents the use of copy constructor 00067 M2MServer( const M2MServer& /*other*/ ); 00068 00069 public: 00070 00071 /** 00072 * \brief Destructor 00073 */ 00074 virtual ~M2MServer(); 00075 00076 /** 00077 * \brief Creates a new resource for a given resource enum. 00078 * \param resource With this function, a value can be set to the following resources: 00079 * 'ShortServerID','Lifetime','DefaultMinPeriod','DefaultMaxPeriod','DisableTimeout', 00080 * 'NotificationStorage'. 00081 * \param value The value to be set on the resource, in integer format. 00082 * \return M2MResource if created successfully, else NULL. 00083 */ 00084 M2MResource* create_resource(ServerResource resource, uint32_t value); 00085 00086 /** 00087 * \brief Creates a new resource for a given resource enum. 00088 * \param resource With this function, the following resources can be created: 00089 * 'Disable', 'RegistrationUpdate' 00090 * \return M2MResource if created successfully, else NULL. 00091 */ 00092 M2MResource* create_resource(ServerResource resource); 00093 00094 /** 00095 * \brief Deletes the resource with the given resource enum. 00096 * Mandatory resources cannot be deleted. 00097 * \param resource The name of the resource to be deleted. 00098 * \return True if deleted, else false. 00099 */ 00100 bool delete_resource(ServerResource rescource); 00101 00102 /** 00103 * \brief Sets the value of a given resource enum. 00104 * \param resource With this function, a value can be set on the following resources: 00105 * 'Binding'. 00106 * \param value The value to be set on the resource, in string format. 00107 * \return True if successfully set, else false. 00108 */ 00109 bool set_resource_value(ServerResource resource, 00110 const String &value); 00111 00112 /** 00113 * \brief Sets the value of a given resource enum. 00114 * \param resource With this function, a value can be set to the following resources: 00115 * 'ShortServerID','Lifetime','DefaultMinPeriod','DefaultMaxPeriod','DisableTimeout', 00116 * 'NotificationStorage'. 00117 * \param value The value to be set on the resource, in integer format. 00118 * \return True if successfully set, else false. 00119 */ 00120 bool set_resource_value(ServerResource resource, 00121 uint32_t value); 00122 /** 00123 * \brief Returns the value of the given resource enum, in string format. 00124 * \param resource With this function, the following resources can return a value: 00125 * 'Binding'. 00126 * \return The value associated with the resource. If the resource is not valid an empty string is returned. 00127 */ 00128 String resource_value_string(ServerResource resource) const; 00129 00130 /** 00131 * \brief Returns the value of a given resource name, in integer format. 00132 * \param resource With this function, the following resources can return a value: 00133 * 'ShortServerID','Lifetime','DefaultMinPeriod','DefaultMaxPeriod','DisableTimeout', 00134 * 'NotificationStorage' 00135 * \return The value associated with the resource. If the resource is not valid -1 is returned. 00136 */ 00137 uint32_t resource_value_int(ServerResource resource) const; 00138 00139 /** 00140 * \brief Returns whether the resource instance with the given resource enum exists or not. 00141 * \param resource Resource enum. 00142 * \return True if at least one instance exists, else false. 00143 */ 00144 bool is_resource_present(ServerResource resource)const; 00145 00146 /** 00147 * \brief Returns the total number of resources for the server object. 00148 * \return The total number of resources. 00149 */ 00150 uint16_t total_resource_count()const; 00151 00152 private: 00153 00154 M2MResource* get_resource(ServerResource res) const; 00155 00156 00157 private: 00158 00159 M2MObjectInstance* _server_instance; 00160 00161 friend class Test_M2MServer; 00162 friend class Test_M2MNsdlInterface; 00163 }; 00164 00165 #endif // M2M_SERVER_H
Generated on Tue Jul 12 2022 21:04:56 by
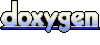