
bug fix
Embed:
(wiki syntax)
Show/hide line numbers
arm_uc_pal_linux_extensions.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #if defined(TARGET_IS_PC_LINUX) 00020 00021 #include "update-client-pal-filesystem/arm_uc_pal_extensions.h" 00022 00023 #include "update-client-common/arm_uc_metadata_header_v2.h" 00024 #include "update-client-common/arm_uc_types.h" 00025 #include "update-client-common/arm_uc_utilities.h" 00026 #include "arm_uc_pal_filesystem_utils.h" 00027 00028 #include "pal.h" 00029 00030 #include "mbed-trace/mbed_trace.h" 00031 #define TRACE_GROUP "update-client-extensions" 00032 00033 #include <stdlib.h> 00034 #include <inttypes.h> 00035 #include <limits.h> 00036 #include <stdio.h> 00037 00038 #ifndef MBED_CONF_UPDATE_CLIENT_APPLICATION_DETAILS 00039 #define MBED_CONF_UPDATE_CLIENT_APPLICATION_DETAILS 0 00040 #endif 00041 00042 #ifndef MBED_CONF_UPDATE_CLIENT_BOOTLOADER_DETAILS 00043 #define MBED_CONF_UPDATE_CLIENT_BOOTLOADER_DETAILS 0 00044 #endif 00045 00046 static void (*arm_ucex_linux_callback)(uint32_t) = NULL; 00047 static palImageId_t arm_ucex_activate_image_id; 00048 00049 #ifndef PAL_UPDATE_ACTIVATE_SCRIPT 00050 #define PAL_UPDATE_ACTIVATE_SCRIPT "./activate_script" 00051 #endif 00052 00053 arm_uc_error_t pal_ext_imageInitAPI(void (*callback)(uint32_t)) 00054 { 00055 arm_uc_error_t result = { .code = ERR_NONE }; 00056 00057 arm_ucex_linux_callback = callback; 00058 00059 return result; 00060 } 00061 00062 arm_uc_error_t pal_ext_imageGetActiveDetails(arm_uc_firmware_details_t* details) 00063 { 00064 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00065 00066 if (details) 00067 { 00068 /* dummy implementation, return 0 */ 00069 memset(details, 0, sizeof(arm_uc_firmware_details_t)); 00070 00071 result.code = ERR_NONE; 00072 00073 if (arm_ucex_linux_callback) 00074 { 00075 arm_ucex_linux_callback(ARM_UC_PAAL_EVENT_GET_ACTIVE_FIRMWARE_DETAILS_DONE); 00076 } 00077 } 00078 00079 return result; 00080 } 00081 00082 arm_uc_error_t pal_ext_installerGetDetails(arm_uc_installer_details_t* details) 00083 { 00084 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00085 00086 if (details) 00087 { 00088 /* dummy implementation, return 0 */ 00089 memset(details, 0, sizeof(arm_uc_installer_details_t)); 00090 00091 result.code = ERR_NONE; 00092 00093 if (arm_ucex_linux_callback) 00094 { 00095 arm_ucex_linux_callback(ARM_UC_PAAL_EVENT_GET_INSTALLER_DETAILS_DONE); 00096 } 00097 } 00098 00099 return result; 00100 } 00101 00102 static void pal_ext_imageActivationWorker(void* location) 00103 { 00104 char cmd_buf[sizeof(PAL_UPDATE_ACTIVATE_SCRIPT) + 1 + PAL_MAX_FILE_AND_FOLDER_LENGTH + 1]; 00105 char path_buf[PAL_MAX_FILE_AND_FOLDER_LENGTH]; 00106 00107 arm_uc_error_t result = arm_uc_pal_filesystem_get_path(*(palImageId_t*)location, FIRMWARE_IMAGE_ITEM_DATA, 00108 path_buf, PAL_MAX_FILE_AND_FOLDER_LENGTH); 00109 palStatus_t rc = PAL_ERR_GENERIC_FAILURE; 00110 00111 if (result.code == ERR_NONE) 00112 { 00113 int err = snprintf(cmd_buf, sizeof(cmd_buf), "%s %s", 00114 PAL_UPDATE_ACTIVATE_SCRIPT, path_buf); 00115 if (err > 0) 00116 { 00117 rc = PAL_SUCCESS; 00118 } 00119 else 00120 { 00121 tr_err("snprintf failed with err %i", err); 00122 rc = PAL_ERR_GENERIC_FAILURE; 00123 } 00124 } 00125 00126 00127 if (rc == PAL_SUCCESS) 00128 { 00129 tr_debug("Activate by executing %s", cmd_buf); 00130 int err = system(cmd_buf); 00131 err = WEXITSTATUS(err); 00132 00133 if (err != -1) 00134 { 00135 tr_debug("Activate completed with %" PRId32, err); 00136 rc = PAL_SUCCESS; 00137 } 00138 else 00139 { 00140 tr_err("system call failed with err %" PRId32, err); 00141 rc = PAL_ERR_GENERIC_FAILURE; 00142 } 00143 } 00144 fflush(stdout); 00145 sleep(1); 00146 00147 if (arm_ucex_linux_callback) 00148 { 00149 uint32_t event = (rc == PAL_SUCCESS? ARM_UC_PAAL_EVENT_ACTIVATE_DONE : ARM_UC_PAAL_EVENT_ACTIVATE_ERROR); 00150 arm_ucex_linux_callback(event); 00151 } 00152 } 00153 00154 arm_uc_error_t pal_ext_imageActivate(uint32_t location) 00155 { 00156 arm_uc_error_t err = { .code = ERR_INVALID_PARAMETER }; 00157 00158 memcpy(&arm_ucex_activate_image_id, &location, sizeof(palImageId_t)); 00159 00160 palThreadID_t thread_id = 0; 00161 palStatus_t rc = pal_osThreadCreateWithAlloc(pal_ext_imageActivationWorker, &arm_ucex_activate_image_id, 00162 PAL_osPriorityBelowNormal, PTHREAD_STACK_MIN, NULL, &thread_id); 00163 if (rc != PAL_SUCCESS) 00164 { 00165 tr_err("Thread creation failed with %x", rc); 00166 err.code = ERR_INVALID_PARAMETER; 00167 } 00168 else 00169 { 00170 tr_debug("Activation thread created, thread ID: %" PRIu32, thread_id); 00171 err.code = ERR_NONE; 00172 } 00173 00174 return err; 00175 } 00176 00177 #endif
Generated on Tue Jul 12 2022 21:04:54 by
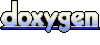