Improved, thread compatible. Adds new features
Fork of GroveGPS by
GroveGPS.h
00001 #ifndef _GROVE_GPS_H_ 00002 #define _GROVE_GPS_H_ 00003 00004 #include "mbed.h" 00005 #include <stdlib.h> 00006 #include <string> 00007 00008 00009 class GroveGPS { 00010 00011 public: 00012 00013 GroveGPS() : _last_line("") { 00014 00015 } 00016 00017 std::string _last_line; 00018 00019 struct GGA { 00020 double utc_time; // Format: hhmmss.sss 00021 double latitude; // Format: ddmm.mmmm 00022 char ns_indicator; // Format: N=north or S=south 00023 double longitude; // Format: dddmm.mmmm 00024 char ew_indicator; // Format: E=east or W=west 00025 int position_fix; // Options: [0=not available, 1=GPS SPS mode, 2=Differential GPS, 6=dead reckoning] 00026 int sats_used; // Range: 0-12 00027 double hdop; // Horizontal Dilution of Precision 00028 double msl_altitude; 00029 char msl_altitude_units; 00030 double geoid_separation; 00031 char geoid_separation_units; 00032 long age_of_diff; 00033 long diff_ref_station_id; 00034 int new_flag; 00035 std::string checksum; 00036 } gps_gga; 00037 00038 struct ZDA { 00039 double utc_time; // Format: hhmmss.sss 00040 int day; 00041 int month; 00042 int year; 00043 int new_flag; 00044 std::string checksum; 00045 } gps_zda; 00046 00047 struct VTG { 00048 double course1; 00049 int truec; 00050 double course2; 00051 int magnetic; 00052 double speed1; 00053 int knots; 00054 double speed2; 00055 int kmh; 00056 int new_flag; 00057 std::string checksum; 00058 } gps_vtg; 00059 00060 void getTimestamp(char* buffer) { 00061 if (gps_gga.position_fix==0) 00062 sprintf(buffer, "N/A"); 00063 else 00064 sprintf(buffer, "%f", gps_gga.utc_time); 00065 } 00066 00067 void getAltitude(char* buffer) { 00068 if (gps_gga.position_fix==0) 00069 sprintf(buffer, "N/A"); 00070 else 00071 sprintf(buffer, "%f", gps_gga.msl_altitude); 00072 } 00073 00074 void getCourse(char* buffer) { 00075 if (gps_gga.position_fix==0) 00076 sprintf(buffer, "N/A"); 00077 else 00078 sprintf(buffer, "%f", gps_vtg.course1); 00079 } 00080 00081 void getSpeed(char* buffer) { 00082 if (gps_gga.position_fix==0) 00083 sprintf(buffer, "N/A"); 00084 else 00085 sprintf(buffer, "%f", gps_vtg.speed1); 00086 } 00087 00088 00089 void getUncertanty(char* buffer) { 00090 if (gps_gga.position_fix==0) 00091 sprintf(buffer, "N/A"); 00092 else 00093 sprintf(buffer, "%f", gps_gga.hdop); 00094 } 00095 00096 void getLatitude(char* buffer) { 00097 double coordinate = convertGPSToDecimal(gps_gga.latitude); 00098 if (gps_gga.position_fix==0) { 00099 sprintf(buffer, "N/A"); 00100 } else { 00101 if(gps_gga.ns_indicator == 'S') coordinate *= -1.000; 00102 sprintf(buffer, "%f", coordinate); 00103 } 00104 } 00105 00106 void getLongitude(char* buffer) { 00107 double coordinate = convertGPSToDecimal(gps_gga.longitude); 00108 if (gps_gga.position_fix==0) 00109 sprintf(buffer, "N/A"); 00110 else { 00111 if(gps_gga.ew_indicator == 'W') coordinate *= -1.0000; 00112 sprintf(buffer, "%f", coordinate); 00113 } 00114 } 00115 00116 00117 double convertGPSToDecimal(double coordinate) { 00118 int degrees = coordinate/100.0; 00119 int minutes = ((int)coordinate) % 100; 00120 double seconds = coordinate - ((int)coordinate); 00121 return degrees + (minutes+seconds)/60; 00122 00123 } 00124 00125 void parseVTG() { 00126 char* pEnd; 00127 for (int i=0; i<5; i++) { 00128 std::string current_item = _last_line.substr(0,_last_line.find(",")); 00129 _last_line = _last_line.substr(_last_line.find(",")+1); 00130 if (i==0) { // NMEA Tag 00131 } else if (i==1) { // UTC time 00132 gps_vtg.course1 = strtod(current_item.c_str(), &pEnd); 00133 } else if (i==3) { 00134 gps_vtg.course2 = strtod(current_item.c_str(), &pEnd); 00135 } else if (i==5) { 00136 gps_vtg.speed1 = strtod(current_item.c_str(), &pEnd); 00137 } else if (i==7) { 00138 gps_vtg.speed2 = strtod(current_item.c_str(), &pEnd); 00139 } 00140 if(gps_vtg.course2 > gps_vtg.course1) gps_vtg.course1 = gps_vtg.course2; 00141 if(gps_vtg.speed2 > gps_vtg.speed1) gps_vtg.speed1 = gps_vtg.speed2; 00142 } 00143 gps_vtg.new_flag = 1; 00144 } 00145 00146 void parseZDA() { 00147 char* pEnd; 00148 for (int i=0; i<5; i++) { 00149 std::string current_item = _last_line.substr(0,_last_line.find(",")); 00150 _last_line = _last_line.substr(_last_line.find(",")+1); 00151 if (i==0) { // NMEA Tag 00152 } else if (i==1) { // UTC time 00153 gps_zda.utc_time = strtod(current_item.c_str(), &pEnd); 00154 } else if (i==2) { 00155 gps_zda.day = strtod(current_item.c_str(), &pEnd); 00156 } else if (i==3) { 00157 gps_zda.month = strtod(current_item.c_str(), &pEnd); 00158 } else if (i==4) { 00159 gps_zda.year = strtod(current_item.c_str(), &pEnd); 00160 } 00161 } 00162 gps_zda.new_flag = 1; 00163 } 00164 00165 void parseGGA() { 00166 char* pEnd; 00167 for (int i=0; i<14; i++) { 00168 std::string current_item = _last_line.substr(0,_last_line.find(",")); 00169 _last_line = _last_line.substr(_last_line.find(",")+1); 00170 if (i==0) { // NMEA Tag 00171 } else if (i==1) { // UTC time 00172 gps_gga.utc_time = strtod(current_item.c_str(), &pEnd); 00173 } else if (i==2) { // Latitude 00174 gps_gga.latitude = strtod(current_item.c_str(), &pEnd); 00175 } else if (i==3) { // Latitude North/South indicator 00176 gps_gga.ns_indicator = current_item[0]; 00177 } else if (i==4) { // Longitude 00178 gps_gga.longitude = strtod(current_item.c_str(), &pEnd); 00179 } else if (i==5) { // Longitude indicator 00180 gps_gga.ew_indicator = current_item[0]; 00181 } else if (i==6) { 00182 gps_gga.position_fix = strtod(current_item.c_str(), &pEnd); 00183 }else if (i==7) { 00184 gps_gga.sats_used = strtod(current_item.c_str(), &pEnd); 00185 } else if (i==8) { // Uncertanty 00186 gps_gga.hdop = strtod(current_item.c_str(), &pEnd); 00187 } else if (i==9) { // Altitude mean sea level 00188 gps_gga.msl_altitude = strtod(current_item.c_str(), &pEnd); 00189 } else if (i==11) { 00190 gps_gga.geoid_separation = strtod(current_item.c_str(), &pEnd); 00191 gps_gga.msl_altitude += gps_gga.geoid_separation; 00192 } 00193 } 00194 gps_gga.new_flag = 1; 00195 } 00196 00197 }; 00198 00199 #endif
Generated on Thu Jul 14 2022 02:19:20 by
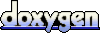