
OK
Dependencies: Cayenne-MQTT-mbed NetworkSocketAPI TMP36 X_NUCLEO_IDW01M1v2 mbed
Fork of Cayenne-X-NUCLEO-IDW01M1-TMP36 by
main.cpp
00001 /** 00002 * Example app for using the Cayenne MQTT mbed library to send data from a TMP36 sensor. This example uses 00003 * the X-NUCLEO-IDW01M1 WiFi expansion board via the X_NUCLEO_IDW01M1v2 library. 00004 */ 00005 00006 #include "MQTTTimer.h" 00007 #include "CayenneMQTTClient.h" 00008 #include "MQTTNetworkIDW01M1.h" 00009 #include "SpwfInterface.h" 00010 #include "TMP36.h" 00011 00012 // WiFi network info. 00013 char* ssid = "Jeff_HomeAP_2EX"; 00014 char* wifiPassword = "iots2480"; 00015 00016 // Cayenne authentication info. This should be obtained from the Cayenne Dashboard. 00017 char* username = "ac38f8d0-027a-11e7-98f3-6b6a2ddab426"; 00018 char* password = "cae33c19fdbaea3fbe427c8cbc3707e02cc5b5a3"; 00019 char* clientID = "139b74f0-8b27-11e7-b7c6-cd9f8fbcf795"; 00020 00021 SpwfSAInterface interface(D8, D2); // TX, RX 00022 MQTTNetwork<SpwfSAInterface> network(interface); 00023 CayenneMQTT::MQTTClient<MQTTNetwork<SpwfSAInterface>, MQTTTimer> mqttClient(network, username, password, clientID); 00024 00025 DigitalOut led1(LED1); 00026 00027 /** 00028 * Print the message info. 00029 * @param[in] message The message received from the Cayenne server. 00030 */ 00031 void outputMessage(CayenneMQTT::MessageData& message) 00032 { 00033 switch (message.topic) { 00034 case COMMAND_TOPIC: 00035 printf("topic=Command"); 00036 break; 00037 case CONFIG_TOPIC: 00038 printf("topic=Config"); 00039 break; 00040 default: 00041 printf("topic=%d", message.topic); 00042 break; 00043 } 00044 printf(" channel=%d", message.channel); 00045 if (message.clientID) { 00046 printf(" clientID=%s", message.clientID); 00047 } 00048 if (message.type) { 00049 printf(" type=%s", message.type); 00050 } 00051 for (size_t i = 0; i < message.valueCount; ++i) { 00052 if (message.getValue(i)) { 00053 printf(" value=%s", message.getValue(i)); 00054 } 00055 if (message.getUnit(i)) { 00056 printf(" unit=%s", message.getUnit(i)); 00057 } 00058 } 00059 if (message.id) { 00060 printf(" id=%s", message.id); 00061 } 00062 printf("\n"); 00063 } 00064 00065 /** 00066 * Handle messages received from the Cayenne server. 00067 * @param[in] message The message received from the Cayenne server. 00068 */ 00069 void messageArrived(CayenneMQTT::MessageData& message) 00070 { 00071 int error = 0; 00072 // Add code to process the message. Here we just ouput the message data. 00073 outputMessage(message); 00074 00075 if (message.topic == COMMAND_TOPIC) { 00076 switch(message.channel) { 00077 case 0: 00078 // Set the onboard LED state 00079 led1 = atoi(message.getValue()); 00080 // Publish the updated LED state 00081 if ((error = mqttClient.publishData(DATA_TOPIC, message.channel, NULL, NULL, message.getValue())) != CAYENNE_SUCCESS) { 00082 printf("Publish LED state failure, error: %d\n", error); 00083 } 00084 break; 00085 } 00086 00087 // If this is a command message we publish a response. Here we are just sending a default 'OK' response. 00088 // An error response should be sent if there are issues processing the message. 00089 if ((error = mqttClient.publishResponse(message.id, NULL, message.clientID)) != CAYENNE_SUCCESS) { 00090 printf("Response failure, error: %d\n", error); 00091 } 00092 } 00093 } 00094 00095 /** 00096 * Connect to the Cayenne server. 00097 * @return Returns CAYENNE_SUCCESS if the connection succeeds, or an error code otherwise. 00098 */ 00099 int connectClient(void) 00100 { 00101 int error = 0; 00102 // Connect to the server. 00103 printf("Connecting to %s:%d\n", CAYENNE_DOMAIN, CAYENNE_PORT); 00104 while ((error = network.connect(CAYENNE_DOMAIN, CAYENNE_PORT)) != 0) { 00105 printf("TCP connect failed, error: %d\n", error); 00106 wait(2); 00107 } 00108 00109 if ((error = mqttClient.connect()) != MQTT::SUCCESS) { 00110 printf("MQTT connect failed, error: %d\n", error); 00111 return error; 00112 } 00113 printf("Connected\n"); 00114 00115 // Subscribe to required topics. 00116 if ((error = mqttClient.subscribe(COMMAND_TOPIC, CAYENNE_ALL_CHANNELS)) != CAYENNE_SUCCESS) { 00117 printf("Subscription to Command topic failed, error: %d\n", error); 00118 } 00119 if ((error = mqttClient.subscribe(CONFIG_TOPIC, CAYENNE_ALL_CHANNELS)) != CAYENNE_SUCCESS) { 00120 printf("Subscription to Config topic failed, error:%d\n", error); 00121 } 00122 00123 // Send device info. Here we just send some example values for the system info. These should be changed to use actual system data, or removed if not needed. 00124 mqttClient.publishData(SYS_VERSION_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, CAYENNE_VERSION); 00125 mqttClient.publishData(SYS_MODEL_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, "mbedDevice"); 00126 //mqttClient.publishData(SYS_CPU_MODEL_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, "CPU Model"); 00127 //mqttClient.publishData(SYS_CPU_SPEED_TOPIC, CAYENNE_NO_CHANNEL, NULL, NULL, "1000000000"); 00128 00129 return CAYENNE_SUCCESS; 00130 } 00131 00132 /** 00133 * Main loop where MQTT code is run. 00134 */ 00135 void loop(void) 00136 { 00137 // Start the countdown timer for publishing data every 5 seconds. Change the timeout parameter to publish at a different interval. 00138 MQTTTimer timer(5000); 00139 TMP36 tmpSensor(A5); 00140 00141 while (true) { 00142 // Yield to allow MQTT message processing. 00143 mqttClient.yield(1000); 00144 00145 // Check that we are still connected, if not, reconnect. 00146 if (!network.connected() || !mqttClient.connected()) { 00147 network.disconnect(); 00148 mqttClient.disconnect(); 00149 printf("Reconnecting\n"); 00150 while (connectClient() != CAYENNE_SUCCESS) { 00151 wait(2); 00152 printf("Reconnect failed, retrying\n"); 00153 } 00154 } 00155 00156 // Publish some example data every few seconds. This should be changed to send your actual data to Cayenne. 00157 if (timer.expired()) { 00158 int error = 0; 00159 if ((error = mqttClient.publishData(DATA_TOPIC, 5, TYPE_TEMPERATURE, UNIT_CELSIUS, tmpSensor.read())) != CAYENNE_SUCCESS) { 00160 printf("Publish temperature failed, error: %d\n", error); 00161 } 00162 // Restart the countdown timer for publishing data every 5 seconds. Change the timeout parameter to publish at a different interval. 00163 timer.countdown_ms(5000); 00164 } 00165 } 00166 } 00167 00168 /** 00169 * Main function. 00170 */ 00171 int main() 00172 { 00173 // Initialize the network interface. 00174 printf("Initializing interface\n"); 00175 interface.connect(ssid, wifiPassword, NSAPI_SECURITY_WPA2); 00176 00177 // Set the default function that receives Cayenne messages. 00178 mqttClient.setDefaultMessageHandler(messageArrived); 00179 00180 // Connect to Cayenne. 00181 if (connectClient() == CAYENNE_SUCCESS) { 00182 // Run main loop. 00183 loop(); 00184 } 00185 else { 00186 printf("Connection failed, exiting\n"); 00187 } 00188 00189 if (mqttClient.connected()) 00190 mqttClient.disconnect(); 00191 if (network.connected()) 00192 network.disconnect(); 00193 00194 return 0; 00195 } 00196
Generated on Tue Jul 12 2022 18:51:18 by
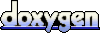