ID the MBED processor on the network if you have more than one. View MBED name on DHCP of your Router - Example \"MBED PE\" Change your ID in Core / host.h
netdb.h
00001 /* 00002 * Redistribution and use in source and binary forms, with or without modification, 00003 * are permitted provided that the following conditions are met: 00004 * 00005 * 1. Redistributions of source code must retain the above copyright notice, 00006 * this list of conditions and the following disclaimer. 00007 * 2. Redistributions in binary form must reproduce the above copyright notice, 00008 * this list of conditions and the following disclaimer in the documentation 00009 * and/or other materials provided with the distribution. 00010 * 3. The name of the author may not be used to endorse or promote products 00011 * derived from this software without specific prior written permission. 00012 * 00013 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00014 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00015 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00016 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00017 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00018 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00019 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00020 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00021 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00022 * OF SUCH DAMAGE. 00023 * 00024 * This file is part of the lwIP TCP/IP stack. 00025 * 00026 * Author: Simon Goldschmidt 00027 * 00028 */ 00029 #ifndef __LWIP_NETDB_H__ 00030 #define __LWIP_NETDB_H__ 00031 00032 #include "lwip/opt.h" 00033 00034 #if LWIP_DNS && LWIP_SOCKET 00035 00036 #include <stddef.h> /* for size_t */ 00037 00038 #include "lwip/inet.h" 00039 #include "lwip/sockets.h" 00040 00041 /* some rarely used options */ 00042 #ifndef LWIP_DNS_API_DECLARE_H_ERRNO 00043 #define LWIP_DNS_API_DECLARE_H_ERRNO 1 00044 #endif 00045 00046 #ifndef LWIP_DNS_API_DEFINE_ERRORS 00047 #define LWIP_DNS_API_DEFINE_ERRORS 1 00048 #endif 00049 00050 #ifndef LWIP_DNS_API_DECLARE_STRUCTS 00051 #define LWIP_DNS_API_DECLARE_STRUCTS 1 00052 #endif 00053 00054 #if LWIP_DNS_API_DEFINE_ERRORS 00055 /** Errors used by the DNS API functions, h_errno can be one of them */ 00056 #define EAI_NONAME 200 00057 #define EAI_SERVICE 201 00058 #define EAI_FAIL 202 00059 #define EAI_MEMORY 203 00060 00061 #define HOST_NOT_FOUND 210 00062 #define NO_DATA 211 00063 #define NO_RECOVERY 212 00064 #define TRY_AGAIN 213 00065 #endif /* LWIP_DNS_API_DEFINE_ERRORS */ 00066 00067 #if LWIP_DNS_API_DECLARE_STRUCTS 00068 struct hostent { 00069 char *h_name; /* Official name of the host. */ 00070 char **h_aliases; /* A pointer to an array of pointers to alternative host names, 00071 terminated by a null pointer. */ 00072 int h_addrtype; /* Address type. */ 00073 int h_length; /* The length, in bytes, of the address. */ 00074 char **h_addr_list; /* A pointer to an array of pointers to network addresses (in 00075 network byte order) for the host, terminated by a null pointer. */ 00076 #define h_addr h_addr_list[0] /* for backward compatibility */ 00077 }; 00078 00079 struct addrinfo { 00080 int ai_flags; /* Input flags. */ 00081 int ai_family; /* Address family of socket. */ 00082 int ai_socktype; /* Socket type. */ 00083 int ai_protocol; /* Protocol of socket. */ 00084 socklen_t ai_addrlen; /* Length of socket address. */ 00085 struct sockaddr *ai_addr; /* Socket address of socket. */ 00086 char *ai_canonname; /* Canonical name of service location. */ 00087 struct addrinfo *ai_next; /* Pointer to next in list. */ 00088 }; 00089 #endif /* LWIP_DNS_API_DECLARE_STRUCTS */ 00090 00091 #if LWIP_DNS_API_DECLARE_H_ERRNO 00092 /* application accessable error code set by the DNS API functions */ 00093 extern int h_errno; 00094 #endif /* LWIP_DNS_API_DECLARE_H_ERRNO*/ 00095 00096 struct hostent *lwip_gethostbyname(const char *name); 00097 int lwip_gethostbyname_r(const char *name, struct hostent *ret, char *buf, 00098 size_t buflen, struct hostent **result, int *h_errnop); 00099 void lwip_freeaddrinfo(struct addrinfo *ai); 00100 int lwip_getaddrinfo(const char *nodename, 00101 const char *servname, 00102 const struct addrinfo *hints, 00103 struct addrinfo **res); 00104 00105 #if LWIP_COMPAT_SOCKETS 00106 #define gethostbyname(name) lwip_gethostbyname(name) 00107 #define gethostbyname_r(name, ret, buf, buflen, result, h_errnop) \ 00108 lwip_gethostbyname_r(name, ret, buf, buflen, result, h_errnop) 00109 #define freeaddrinfo(addrinfo) lwip_freeaddrinfo(addrinfo) 00110 #define getaddrinfo(nodname, servname, hints, res) \ 00111 lwip_getaddrinfo(nodname, servname, hints, res) 00112 #endif /* LWIP_COMPAT_SOCKETS */ 00113 00114 #endif /* LWIP_DNS && LWIP_SOCKET */ 00115 00116 #endif /* __LWIP_NETDB_H__ */
Generated on Tue Jul 12 2022 20:22:58 by
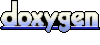