using PWM to control RGB LED for RedBear Demo
Fork of ChainableLED by
RGBLED.h
00001 /* 00002 Copyright (C) 2013 Seeed Technology Inc. 00003 Copyright (C) 2012 Paulo Marques (pjp.marques@gmail.com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of 00006 this software and associated documentation files (the "Software"), to deal in 00007 the Software without restriction, including without limitation the rights to 00008 use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of 00009 the Software, and to permit persons to whom the Software is furnished to do so, 00010 subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in all 00013 copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS 00017 FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR 00018 COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER 00019 IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN 00020 CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00021 */ 00022 00023 /* 00024 * Library for controlling a chain of RGB LEDs based on the P9813 protocol. 00025 * E.g., supports the Grove Chainable RGB LED product. 00026 * 00027 * Information about the P9813 protocol obtained from: 00028 * http://www.seeedstudio.com/wiki/index.php?title=Twig_-_Chainable_RGB_LED 00029 */ 00030 00031 00032 00033 #ifndef __RGBLED_h__ 00034 #define __RGBLED_h__ 00035 00036 #include "mbed.h" 00037 00038 #define _CL_RED 0 00039 #define _CL_GREEN 1 00040 #define _CL_BLUE 2 00041 #define _CLK_PULSE_DELAY 20 00042 00043 class ChainableLED 00044 { 00045 public: 00046 ChainableLED(PinName r_pin, PinName g_pin, PinName b_pin, unsigned int number_of_leds); 00047 ~ChainableLED(); 00048 00049 void setColorRGB(unsigned int led, float red, float green, float blue); 00050 void setColorHSB(unsigned int led, float hue, float saturation, float brightness); 00051 00052 private: 00053 DigitalOut _r_pin; 00054 DigitalOut _g_pin; 00055 DigitalOut _b_pin; 00056 unsigned int _num_leds; 00057 00058 uint8_t _led_state[3]; 00059 00060 void sendColor(uint8_t red, uint8_t green, uint8_t blue); 00061 }; 00062 00063 #endif
Generated on Wed Jul 13 2022 20:23:34 by
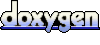