
Nano RGB LED mesh
Dependencies: BLE_API RGBLED mbed nRF51822
main.cpp
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 #include "mbed.h" 00021 #include "ble/BLE.h" 00022 #include "RGBLED.h" 00023 00024 #define TXRX_BUF_LEN 20 00025 00026 00027 BLE ble; 00028 00029 ChainableLED leds(P0_11, P0_9, P0_10,1); 00030 //Serial pc(USBTX, USBRX); 00031 00032 00033 // The Nordic UART Service 00034 static const uint16_t mesh_base_uuid = 0xFEE4; 00035 static const uint8_t mesh_metadata_uuid[] = {0x2A, 0x1E, 0x00, 0x04, 0xFD, 0x51, 0xD8, 0x82, 0x8B, 0xA8, 0xB9, 0x8C, 0x00, 0x00, 0xCD, 0x1E}; 00036 static const uint8_t mesh_value_uuid[] = {0x2A, 0x1E, 0x00, 0x05, 0xFD, 0x51, 0xD8, 0x82, 0x8B, 0xA8, 0xB9, 0x8C, 0x00, 0x00, 0xCD, 0x1E}; 00037 00038 00039 uint8_t metadataPayload[TXRX_BUF_LEN] = {0,}; 00040 uint8_t valuePayload[TXRX_BUF_LEN] = {0,}; 00041 00042 static uint8_t advData[14]={0x07,0x16,0xE4,0xFE,0,0,0,0,0,0,0,0,0,0}; 00043 00044 //static uint8_t rx_buf[TXRX_BUF_LEN]; 00045 //static uint8_t rx_len=0; 00046 00047 00048 GattCharacteristic metadataCharacteristic (mesh_metadata_uuid, metadataPayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00049 00050 GattCharacteristic valueCharacteristic (mesh_value_uuid, valuePayload, 1, TXRX_BUF_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE| GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00051 00052 GattCharacteristic *meshChars[] = {&metadataCharacteristic, &valueCharacteristic}; 00053 00054 GattService meshService(mesh_base_uuid, meshChars, sizeof(meshChars) / sizeof(GattCharacteristic *)); 00055 00056 00057 00058 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00059 { 00060 //pc.printf("Disconnected \r\n"); 00061 //pc.printf("Restart advertising \r\n"); 00062 ble.gap().startAdvertising(); 00063 } 00064 00065 void WrittenHandler(const GattWriteCallbackParams *Handler) 00066 { 00067 uint8_t buf[TXRX_BUF_LEN]; 00068 uint16_t bytesRead; 00069 00070 if (Handler->handle == valueCharacteristic.getValueAttribute().getHandle()) 00071 { 00072 00073 00074 } 00075 } 00076 00077 00078 void scanCallBack(const Gap::AdvertisementCallbackParams_t *report) 00079 { 00080 /*uint8_t index; 00081 pc.printf("BLE scan \r\n"); 00082 pc.printf("The ADV data: "); 00083 for(index=0; index<report->advertisingDataLen; index++) 00084 { 00085 pc.printf("%x ", report->advertisingData[index]); 00086 00087 } 00088 pc.printf(" \r\n");*/ 00089 00090 if((report->advertisingData[2] == 0x0D)&&(report->advertisingData[3] == 0x16)&&(report->advertisingData[4] == 0xE4)&&(report->advertisingData[5] == 0xFE)) 00091 { 00092 00093 //if(memcmp(report->advertisingData,client_adv_temp,sizeof(report->advertisingData)) != 0) 00094 { 00095 if((advData[8]==report->advertisingData[10])&&(advData[9]==report->advertisingData[11])&&(advData[10]==report->advertisingData[12])&&(advData[11]==report->advertisingData[13])&&(advData[12]==report->advertisingData[14])&&(advData[13]==report->advertisingData[15])) 00096 { 00097 //Serial.println("same data"); 00098 return; 00099 } 00100 //memcpy(advdata_temp,report->advertisingData,report->advDataLen); 00101 if(report->advertisingData[10] == 0x03) 00102 { 00103 ble.stopScan(); 00104 memset(advData,0,sizeof(advData)); 00105 for(uint8_t i = 0;i<14;i++) 00106 { 00107 advData[i]=report->advertisingData[i+2]; 00108 } 00109 00110 /*for(index=0; index<report->advertisingDataLen-2; index++) 00111 { 00112 pc.printf("%x ", advData[index]); 00113 00114 } 00115 pc.printf(" \r\n"); */ 00116 leds.setColorRGB(0, report->advertisingData[13], report->advertisingData[14], report->advertisingData[15]); 00117 ble.clearAdvertisingPayload(); 00118 ble.accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA,advData,14); 00119 ble.startAdvertising(); 00120 wait_ms(50); 00121 ble.stopAdvertising(); 00122 ble.startScan(scanCallBack); 00123 } 00124 00125 } 00126 } 00127 else if((report->advertisingData[2] == 0x07)&&(report->advertisingData[3] == 0x16)&&(report->advertisingData[4] == 0xE4)&&(report->advertisingData[5] == 0xFE)) 00128 { 00129 /*for(index=0; index<14; index++) 00130 { 00131 pc.printf("%x ", advData[index]); 00132 00133 } 00134 pc.printf(" \r\n");*/ 00135 ble.stopScan(); 00136 00137 ble.accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA,advData,14); 00138 ble.startAdvertising(); 00139 wait_ms(50); 00140 ble.stopAdvertising(); 00141 ble.startScan(scanCallBack); 00142 00143 } 00144 00145 00146 } 00147 00148 00149 void m_status_check_handle(void) 00150 { 00151 00152 } 00153 00154 00155 int main(void) 00156 { 00157 Ticker ticker; 00158 ticker.attach_us(m_status_check_handle, 200000); 00159 00160 ble.init(); 00161 ble.onDisconnection(disconnectionCallback); 00162 ble.onDataWritten(WrittenHandler); 00163 00164 //pc.baud(9600); 00165 //pc.printf("SimpleChat Init \r\n"); 00166 00167 00168 // setup advertising 00169 ble.accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA,advData,14); 00170 00171 // 100ms; in multiples of 0.625ms. 00172 ble.setAdvertisingInterval(60); 00173 00174 ble.addService(meshService); 00175 00176 ble.startAdvertising(); 00177 00178 //pc.printf("Advertising Start \r\n"); 00179 ble.setScanParams(60,0x30); 00180 ble.startScan(scanCallBack); 00181 00182 00183 //pc.printf("Advertising Start \r\n"); 00184 00185 while(1) 00186 { 00187 ble.waitForEvent(); 00188 } 00189 } 00190 00191 00192 00193 00194 00195 00196 00197 00198 00199 00200 00201 00202 00203 00204 00205 00206 00207 00208 00209 00210 00211 00212 00213 00214 00215 00216 00217 00218 00219 00220
Generated on Wed Aug 17 2022 10:03:26 by
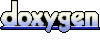