None
Fork of cc3000_hostdriver_mbedsocket by
cc3000_wlan Class Reference
WLAN layer. More...
#include <cc3000.h>
Public Member Functions | |
cc3000_wlan (cc3000_simple_link &simple_link, cc3000_event &event, cc3000_spi &spi, cc3000_hci &hci) | |
Ctor. | |
~cc3000_wlan () | |
Dtor. | |
void | simpleLink_init_start (uint16_t patches_available_host) |
Send SIMPLE LINK START to cc3000. | |
void | start (uint16_t patches_available_host) |
Start wlan device. | |
void | stop (void) |
Stop wlan device. | |
int32_t | connect (uint32_t sec_type, const uint8_t *ssid, int32_t ssid_length, uint8_t *b_ssid, uint8_t *key, int32_t key_len) |
Connect to AP. | |
int32_t | add_profile (uint32_t sec_type, uint8_t *ssid, uint32_t ssid_length, uint8_t *b_ssid, uint32_t priority, uint32_t pairwise_cipher_or_tx_key_len, uint32_t group_cipher_tx_key_index, uint32_t key_mgmt, uint8_t *pf_or_key, uint32_t pass_phrase_length) |
Add profile. | |
int32_t | ioctl_get_scan_results (uint32_t scan_timeout, uint8_t *results) |
Gets entry from scan result table. | |
int32_t | ioctl_set_scan_params (uint32_t enable, uint32_t min_dwell_time, uint32_t max_dwell_time, uint32_t num_probe_requests, uint32_t channel_mask, int32_t rssi_threshold, uint32_t snr_threshold, uint32_t default_tx_power, uint32_t *interval_list) |
Start and stop scan procedure. | |
int32_t | ioctl_statusget (void) |
Get wlan status: disconnected, scanning, connecting or connected. | |
int32_t | connect (const uint8_t *ssid, int32_t ssid_length) |
Connect to AP. | |
int32_t | add_profile (uint32_t sec_type, uint8_t *ssid, uint32_t ssid_length, uint8_t *b_ssid, uint32_t priority, uint32_t pairwise_cipher_or_tx_key_len, uint32_t group_cipher_tx_key_index, uint32_t key_mgmt, uint8_t *pf_or_key, uint32_t pass_phrase_length) |
When auto start is enabled, the device connects to station from the profiles table. | |
int32_t | smart_config_process (void) |
Process the acquired data and store it as a profile. | |
int32_t | disconnect () |
Disconnect connection from AP. | |
int32_t | ioctl_set_connection_policy (uint32_t should_connect_to_open_ap, uint32_t use_fast_connect, uint32_t use_profiles) |
When auto is enabled, the device tries to connect according the following policy: 1) If fast connect is enabled and last connection is valid, the device will try to connect to it without the scanning procedure (fast). | |
int32_t | ioctl_del_profile (uint32_t index) |
Delete WLAN profile. | |
int32_t | set_event_mask (uint32_t mask) |
Mask event according to bit mask. | |
int32_t | smart_config_start (uint32_t encrypted_flag) |
Start to acquire device profile. | |
int32_t | smart_config_stop (void) |
Stop the acquire profile procedure. | |
int32_t | smart_config_set_prefix (uint8_t *new_prefix) |
Configure station ssid prefix. |
Detailed Description
WLAN layer.
Definition at line 1332 of file cc3000.h.
Constructor & Destructor Documentation
cc3000_wlan | ( | cc3000_simple_link & | simple_link, |
cc3000_event & | event, | ||
cc3000_spi & | spi, | ||
cc3000_hci & | hci | ||
) |
Ctor.
- Parameters:
-
simple_link Reference to the simple link object. event Reference to the event object. spi Reference to the spi object. hci Reference to the hci object.
- Returns:
- none
Definition at line 45 of file cc3000_wlan.cpp.
~cc3000_wlan | ( | ) |
Member Function Documentation
int32_t add_profile | ( | uint32_t | sec_type, |
uint8_t * | ssid, | ||
uint32_t | ssid_length, | ||
uint8_t * | b_ssid, | ||
uint32_t | priority, | ||
uint32_t | pairwise_cipher_or_tx_key_len, | ||
uint32_t | group_cipher_tx_key_index, | ||
uint32_t | key_mgmt, | ||
uint8_t * | pf_or_key, | ||
uint32_t | pass_phrase_length | ||
) |
Add profile.
Up to 7 profiles are supported.
- Parameters:
-
sec_type Security option. ssid Up to 32 bytes, ASCII SSID ssid_length Length of SSID b_ssid 6 bytes specified the AP bssid priority Up to 16 bytes specified the AP security key pairwise_cipher_or_tx_key_len Key length group_cipher_tx_key_index Key length for WEP security key_mgmt KEY management pf_or_key Security key pass_phrase_length Security key length for WPA
- Returns:
- On success, zero is returned. On error, negative is returned.
Definition at line 326 of file cc3000_wlan.cpp.
int32_t add_profile | ( | uint32_t | sec_type, |
uint8_t * | ssid, | ||
uint32_t | ssid_length, | ||
uint8_t * | b_ssid, | ||
uint32_t | priority, | ||
uint32_t | pairwise_cipher_or_tx_key_len, | ||
uint32_t | group_cipher_tx_key_index, | ||
uint32_t | key_mgmt, | ||
uint8_t * | pf_or_key, | ||
uint32_t | pass_phrase_length | ||
) |
When auto start is enabled, the device connects to station from the profiles table.
If several profiles configured the device choose the highest priority profile.
- Parameters:
-
sec_type WLAN_SEC_UNSEC,WLAN_SEC_WEP,WLAN_SEC_WPA,WLAN_SEC_WPA2 ssid SSID up to 32 bytes ssid_length SSID length b_ssid bssid 6 bytes priority Profile priority. Lowest priority:0. pairwise_cipher_or_tx_key_len Key length for WEP security group_cipher_tx_key_index Key index key_mgmt KEY management pf_or_key Security key pass_phrase_length Security key length for WPA
- Returns:
- On success, zero is returned. On error, -1 is returned
int32_t connect | ( | const uint8_t * | ssid, |
int32_t | ssid_length | ||
) |
Connect to AP.
- Parameters:
-
ssid Up to 32 bytes and is ASCII SSID of the AP ssid_length Length of the SSID
- Returns:
- On success, zero is returned. On error, negative is returned.
Definition at line 521 of file cc3000_wlan.cpp.
int32_t connect | ( | uint32_t | sec_type, |
const uint8_t * | ssid, | ||
int32_t | ssid_length, | ||
uint8_t * | b_ssid, | ||
uint8_t * | key, | ||
int32_t | key_len | ||
) |
Connect to AP.
- Parameters:
-
sec_type Security option. ssid up to 32 bytes, ASCII SSID ssid_length length of SSID b_ssid 6 bytes specified the AP bssid key up to 16 bytes specified the AP security key key_len key length
- Returns:
- On success, zero is returned. On error, negative is returned.
Definition at line 284 of file cc3000_wlan.cpp.
int32_t disconnect | ( | ) |
Disconnect connection from AP.
- Parameters:
-
none
- Returns:
- 0 if disconnected done, other CC3000 already disconnected.
Definition at line 116 of file cc3000_wlan.cpp.
int32_t ioctl_del_profile | ( | uint32_t | index ) |
Delete WLAN profile.
- Parameters:
-
index Number of profile to delete
- Returns:
- On success, zero is returned. On error, -1 is returned
Definition at line 159 of file cc3000_wlan.cpp.
int32_t ioctl_get_scan_results | ( | uint32_t | scan_timeout, |
uint8_t * | results | ||
) |
Gets entry from scan result table.
The scan results are returned one by one, and each entry represents a single AP found in the area.
- Parameters:
-
scan_timeout Not supported yet results Scan result
- Returns:
- On success, zero is returned. On error, -1 is returned
Definition at line 435 of file cc3000_wlan.cpp.
int32_t ioctl_set_connection_policy | ( | uint32_t | should_connect_to_open_ap, |
uint32_t | use_fast_connect, | ||
uint32_t | use_profiles | ||
) |
When auto is enabled, the device tries to connect according the following policy: 1) If fast connect is enabled and last connection is valid, the device will try to connect to it without the scanning procedure (fast).
The last connection will be marked as invalid, due to adding/removing profile. 2) If profile exists, the device will try to connect it (Up to seven profiles). 3) If fast and profiles are not found, and open mode is enabled, the device will try to connect to any AP. Note that the policy settings are stored in the CC3000 NVMEM.
- Parameters:
-
should_connect_to_open_ap Enable(1), disable(0) connect to any available AP. use_fast_connect Enable(1), disable(0). if enabled, tries to connect to the last connected AP. use_profiles Enable(1), disable(0) auto connect after reset. and periodically reconnect if needed.
- Returns:
- On success, zero is returned. On error, -1 is returned
Definition at line 133 of file cc3000_wlan.cpp.
int32_t ioctl_set_scan_params | ( | uint32_t | enable, |
uint32_t | min_dwell_time, | ||
uint32_t | max_dwell_time, | ||
uint32_t | num_probe_requests, | ||
uint32_t | channel_mask, | ||
int32_t | rssi_threshold, | ||
uint32_t | snr_threshold, | ||
uint32_t | default_tx_power, | ||
uint32_t * | interval_list | ||
) |
Start and stop scan procedure.
Set scan parameters.
- Parameters:
-
enable Start/stop application scan min_dwell_time Minimum dwell time value to be used for each channel, in ms. (Default: 20) max_dwell_time Maximum dwell time value to be used for each channel, in ms. (Default: 30) num_probe_requests Max probe request between dwell time. (Default:2) channel_mask Bitwise, up to 13 channels (0x1fff). rssi_threshold RSSI threshold. Saved: yes (Default: -80) snr_threshold NSR threshold. Saved: yes (Default: 0) default_tx_power probe Tx power. Saved: yes (Default: 205) interval_list Pointer to array with 16 entries (16 channels)
- Returns:
- On success, zero is returned. On error, -1 is returned.
Definition at line 454 of file cc3000_wlan.cpp.
int32_t ioctl_statusget | ( | void | ) |
Get wlan status: disconnected, scanning, connecting or connected.
- Parameters:
-
none
- Returns:
- WLAN_STATUS_DISCONNECTED, WLAN_STATUS_SCANING, STATUS_CONNECTING or WLAN_STATUS_CONNECTED
Definition at line 491 of file cc3000_wlan.cpp.
int32_t set_event_mask | ( | uint32_t | mask ) |
Mask event according to bit mask.
In case that event is masked (1), the device will not send the masked event to host.
- Parameters:
-
mask event mask
- Returns:
- On success, zero is returned. On error, -1 is returned
Definition at line 180 of file cc3000_wlan.cpp.
void simpleLink_init_start | ( | uint16_t | patches_available_host ) |
Send SIMPLE LINK START to cc3000.
- Parameters:
-
patches_available_host Flag to indicate if patches are available.
- Returns:
- none
Definition at line 54 of file cc3000_wlan.cpp.
int32_t smart_config_process | ( | void | ) |
Process the acquired data and store it as a profile.
- Parameters:
-
none
- Returns:
- On success, zero is returned. On error, -1 is returned.
Definition at line 557 of file cc3000_wlan.cpp.
int32_t smart_config_set_prefix | ( | uint8_t * | new_prefix ) |
Configure station ssid prefix.
- Parameters:
-
new_prefix 3 bytes identify the SSID prefix for the Smart Config.
- Returns:
- On success, zero is returned. On error, -1 is returned.
Definition at line 255 of file cc3000_wlan.cpp.
int32_t smart_config_start | ( | uint32_t | encrypted_flag ) |
Start to acquire device profile.
The device acquire its own profile, if profile message is found.
- Parameters:
-
encrypted_flag Indicates whether the information is encrypted
- Returns:
- On success, zero is returned. On error, -1 is returned.
Definition at line 218 of file cc3000_wlan.cpp.
int32_t smart_config_stop | ( | void | ) |
Stop the acquire profile procedure.
- Parameters:
-
none
- Returns:
- On success, zero is returned. On error, -1 is returned
Definition at line 240 of file cc3000_wlan.cpp.
void start | ( | uint16_t | patches_available_host ) |
Start wlan device.
Blocking call until init is completed.
- Parameters:
-
patches_available_host Flag to indicate if patches are available.
- Returns:
- none
Definition at line 68 of file cc3000_wlan.cpp.
void stop | ( | void | ) |
Generated on Fri Jul 15 2022 17:19:25 by
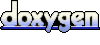