x
Fork of FT810 by
Embed:
(wiki syntax)
Show/hide line numbers
FT_Hal_Utils.cpp
00001 #include "FT_Platform.h" 00002 #include "mbed.h" 00003 //#include "SDFileSystem.h" 00004 00005 #define DEBUG 00006 00007 /* meta-commands, sequences of several display-list entries condensed into simpler to use functions at the price of some overhead */ 00008 00009 ft_void_t FT813::Point(ft_int16_t x, ft_int16_t y, ft_uint16_t size) 00010 { 00011 ft_uint32_t calc; 00012 00013 StartFunc(FT_CMD_SIZE*4); 00014 SendCmd(DL_BEGIN | FT8_POINTS); 00015 calc = POINT_SIZE(size*16); 00016 SendCmd(calc); 00017 calc = VERTEX2F(x * 16, y * 16); 00018 SendCmd(calc); 00019 SendCmd(DL_END); 00020 EndFunc(); 00021 } 00022 00023 ft_void_t FT813::Line(ft_int16_t x0, ft_int16_t y0, ft_int16_t x1, ft_int16_t y1, ft_int16_t width) 00024 { 00025 ft_uint32_t calc; 00026 00027 StartFunc(FT_CMD_SIZE*5); 00028 SendCmd(DL_BEGIN | FT8_LINES); 00029 calc = LINE_WIDTH(width * 16); 00030 SendCmd(calc); 00031 calc = VERTEX2F(x0 * 16, y0 * 16); 00032 SendCmd(calc); 00033 calc = VERTEX2F(x1 * 16, y1 * 16); 00034 SendCmd(calc); 00035 SendCmd(DL_END); 00036 EndFunc(); 00037 } 00038 00039 ft_void_t FT813::Rect(ft_int16_t x0, ft_int16_t y0, ft_int16_t x1, ft_int16_t y1, ft_int16_t corner) 00040 { 00041 ft_uint32_t calc; 00042 00043 StartFunc(FT_CMD_SIZE*5); 00044 SendCmd(DL_BEGIN | FT8_RECTS); 00045 calc = LINE_WIDTH(corner * 16); 00046 SendCmd(calc); 00047 calc = VERTEX2F(x0 * 16, y0 * 16); 00048 SendCmd(calc); 00049 calc = VERTEX2F(x1 * 16, y1 * 16); 00050 SendCmd(calc); 00051 SendCmd(DL_END); 00052 EndFunc(); 00053 } 00054 00055 ft_void_t FT813::RectWH(ft_int16_t x0, ft_int16_t y0, ft_int16_t w, ft_int16_t h, ft_int16_t corner) 00056 { 00057 ft_uint32_t calc; 00058 x0 += corner; 00059 y0 += corner; 00060 w -= corner*2; 00061 h -= corner*2; 00062 ft_int16_t x1 = x0 + w; 00063 ft_int16_t y1 = y0 + h; 00064 StartFunc(FT_CMD_SIZE*5); 00065 SendCmd(DL_BEGIN | FT8_RECTS); 00066 calc = LINE_WIDTH(corner * 16); 00067 SendCmd(calc); 00068 calc = VERTEX2F(x0 * 16, y0 * 16); 00069 SendCmd(calc); 00070 calc = VERTEX2F(x1 * 16, y1 * 16); 00071 SendCmd(calc); 00072 SendCmd(DL_END); 00073 EndFunc(); 00074 } 00075 00076 /* Function to load JPG file from the filesystem into the FT800 buffer. */ 00077 /* First the graphics data have to be load into the FT800 buffer. */ 00078 /* The FT800 will decode the JPG data into a bitmap. */ 00079 /* In the second step this bitmap will displayed on the LCD. */ 00080 /* return 0 if jpg is ok */ 00081 /* return x_size and y_size of jpg */ 00082 ft_uint8_t FT813::LoadJpg(char* filename, ft_int16_t* x_size, ft_int16_t* y_size) 00083 { 00084 // unsigned char pbuff[8192]; 00085 int bufferSize = 8192; 00086 // void* pbuff; 00087 char* pbuff = (char*)malloc(bufferSize); 00088 // char pbuff[BUFFER_SIZE] = {0}; 00089 unsigned short marker; 00090 unsigned short length; 00091 unsigned char data[4]; 00092 00093 ft_uint16_t blocklen; 00094 00095 // printf("LoadJpg: Open filename \"%s\".\n", filename); 00096 00097 FILE *fp = fopen(filename, "rb"); 00098 if(fp == NULL) { 00099 free(pbuff); 00100 printf("LoadJpg: Cannot open file \"%s\".\n", filename); 00101 return (-1); // cannot open file 00102 } 00103 00104 // https://en.wikipedia.org/wiki/JPEG 00105 // search for 0xFFC0 marker 00106 fseek(fp, 0, SEEK_END); 00107 unsigned int filesize = ftell(fp); 00108 printf("LoadJpg: Size: %d.\n", filesize); 00109 00110 fseek(fp, 2, SEEK_SET); // Beginning of file 00111 fread(data, 4, 1, fp); // Read first 4 bytes 00112 marker = data[0] << 8 | data[1]; 00113 length = data[2] << 8 | data[3]; 00114 do { 00115 if(marker == 0xFFC0) 00116 break; 00117 if(marker & 0xFF00 != 0xFF00) 00118 break; 00119 if (fseek(fp, length - 2,SEEK_CUR) != 0) // Skip marker 00120 break; 00121 fread(data, 4, 1, fp); 00122 marker = data[0] << 8 | data[1]; 00123 length = data[2] << 8 | data[3]; 00124 } while(1); 00125 00126 if(marker != 0xFFC0) { 00127 free(pbuff); 00128 printf("LoadJpg: marker != 0xFFC0\n"); 00129 return (-2); // No FFC0 Marker, wrong format no baseline DCT-based JPEG 00130 } 00131 00132 fseek(fp, 1, SEEK_CUR); // Skip marker 00133 fread(data, 8, 1, fp); // Read next 8 bytes & extract height & width 00134 *y_size = (data[0] << 8 | data[1]); 00135 *x_size = (data[2] << 8 | data[3]); 00136 uint16_t size_y = (data[0] << 8 | data[1]); 00137 uint16_t size_x = (data[2] << 8 | data[3]); 00138 00139 uint16_t disp_x = DispWidth; 00140 uint16_t disp_y = DispHeight; 00141 if ((_orientation == FT8_DISPLAY_PORTRAIT_90CW) || (_orientation == FT8_DISPLAY_PORTRAIT_90CCW)) { 00142 disp_x = DispHeight; 00143 disp_y = DispWidth; 00144 } 00145 00146 // http://www.ftdichip.com/Support/Documents/AppNotes/AN_339%20Using%20JPEGs%20with%20the%20FT800%20series.pdf, page 11 00147 // if (marker_next_byte[0] == 0xC2){ //check if progressive JPEG 00148 // error_code = 3; 00149 // } 00150 00151 // if(*x_size > TFT.FT_DispWidth || *y_size > TFT.FT_DispHeight) return (-3); // to big to fit on screen 00152 if(*x_size > disp_x || *y_size > disp_y) 00153 { 00154 free(pbuff); 00155 printf("LoadJpg: Too big to fit on screen\n"); 00156 printf("LoadJpg: JPG (%dx%d) does not fit on TFT (%dx%d)\n", *x_size, *y_size, DispWidth, DispHeight); 00157 return (-3); // Too big to fit on screen 00158 } 00159 00160 // printf("LoadJpg: JPG (%dx%d) fits on TFT (%dx%d)\n", *x_size, *y_size, DispWidth, DispHeight); 00161 00162 fseek(fp, 0, SEEK_SET); 00163 00164 00165 WrCmd32(CMD_LOADIMAGE); 00166 WrCmd32(_address); //destination address of jpg decode 00167 00168 // Keep track of of the start addresses of loaded images 00169 // Increment _address 00170 _addresses[_bitmap_count] = _address; 00171 _bitmap_count++; 00172 _address += (uint32_t) (size_x * size_y * 2); 00173 _addresses[_bitmap_count] = _address; 00174 // 0 OPT_RGB565 00175 // 1 OPT_MONO 00176 // 2 OPT_NODL 00177 // 256 OPT_FLAT 00178 // 256 OPT_SIGNED 00179 // 512 OPT_CENTERX 00180 // 1024 OPT_CENTERY 00181 // 1536 OPT_CENTER 00182 // 2048 OPT_RIGHTX 00183 // 4096 OPT_NOBACK 00184 // 8192 OPT_NOTICKS 00185 WrCmd32(0); //output format of the bitmap - default is rgb565 00186 while(filesize > 0) { 00187 /* download the data into the command buffer by 8kb one shot */ 00188 blocklen = filesize > bufferSize ? bufferSize : filesize; 00189 // printf("LoadJpg: blocklen: %d\n", blocklen); 00190 // printf("LoadJpg: filesize: %d\n", filesize); 00191 00192 /* copy the data into pbuff and then transfter it to command buffer */ 00193 // int size = fread(&pbuff[0], 1, blocklen, fp); 00194 int size = fread(pbuff, 1, blocklen, fp); 00195 // printf("LoadJpg: fread: %d, %d\n", blocklen, size); 00196 filesize -= blocklen; 00197 /* copy data continuously into command memory */ 00198 // TFT.Ft_Gpu_Hal_WrCmdBuf(pbuff, blocklen); //alignment is already taken care by this api 00199 WrCmdBuf((uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00200 } 00201 fclose(fp); 00202 free(pbuff); 00203 00204 return(0); 00205 } 00206 00207 ft_uint8_t FT813::LoadPng(char* filename, ft_int16_t* x_size, ft_int16_t* y_size) 00208 { 00209 int bufferSize = 8192; 00210 ft_uint32_t filesize = 0; 00211 ft_uint16_t blocklen = 0; 00212 uint32_t marker1; 00213 uint32_t marker2; 00214 unsigned short length; 00215 unsigned char data[32]; 00216 00217 // printf("------------------------------\n"); 00218 printf("LoadPng: Filename \"%s\".\n", filename); 00219 00220 FILE *fp = fopen(filename, "rb"); 00221 if(fp == NULL) { 00222 printf("LoadPng: Cannot open file \"%s\".\n", filename); 00223 return (-1); // cannot open file 00224 } 00225 00226 // Get file size 00227 fseek(fp, 0, SEEK_END); // End of file 00228 filesize = ftell(fp); 00229 fseek(fp, 0, SEEK_SET); // Beginning of file 00230 printf("LoadPng: Filesize %d\n", filesize); 00231 00232 // search for 0x89504E47 and 0x0D0A1A0A markers "‰PNG...." 00233 fread(data, 1, 32, fp); // Read first 32 bytes 00234 // rewind(fp); // Beginning of file 00235 fseek(fp, 0, SEEK_SET); // Beginning of file 00236 00237 // Check that this is indeed a PNG file 00238 unsigned char png_header[] = {0x89, 0x50, 0x4E, 0x47, 0x0D, 0x0A, 0x1A, 0x0A}; 00239 // Make sure you have an IHDR 00240 unsigned char ihdr_name[] = "IHDR"; 00241 if ((memcmp(data, png_header, 8)) || (memcmp(data+8+4, ihdr_name, 4))) { 00242 printf("LoadPng: Invalid PNG file.\n"); 00243 return (-2); // No FFC0 Marker, wrong format no baseline DCT-based JPEG 00244 } 00245 00246 // PNG actually stores integers in big-endian. 00247 *x_size = ReadBigInt32(data, 24 - 8); 00248 *y_size = ReadBigInt32(data, 24 - 4); 00249 uint16_t size_x = ReadBigInt32(data, 24 - 8); 00250 uint16_t size_y = ReadBigInt32(data, 24 - 4); 00251 00252 uint16_t disp_x = DispWidth; 00253 uint16_t disp_y = DispHeight; 00254 if ((_orientation == FT8_DISPLAY_PORTRAIT_90CW) || (_orientation == FT8_DISPLAY_PORTRAIT_90CCW)) { 00255 disp_x = DispHeight; 00256 disp_y = DispWidth; 00257 } 00258 00259 if(*x_size > disp_x || *y_size > disp_y) 00260 { 00261 printf("LoadPng: Too big to fit on screen\n"); 00262 printf("LoadPng: PNG (%dx%d) does not fit on TFT (%dx%d)\n", *x_size, *y_size, DispWidth, DispHeight); 00263 return (-3); // Too big to fit on screen 00264 } 00265 00266 // printf("LoadPng: PNG (%dx%d) fits on TFT (%dx%d)\n", *x_size, *y_size, DispWidth, DispHeight); 00267 00268 // http://www.ftdichip.com/Support/Documents/AppNotes/AN_339%20Using%20JPEGs%20with%20the%20FT800%20series.pdf 00269 // CMD_LOADIMAGE: This function will decode the JPEG file, produce 00270 // either RGB565 or L8 bitmap data and store this in graphics RAM. 00271 // It also writes commands to the display list to set the source, layout and size of the 00272 // image (BITMAP_SOURCE, BITMAP_LAYOUT and BITMAP_SIZE). 00273 // Note that only a BEGIN and VERTEX2F (or VERTEX2II) display list commands are then 00274 // required to complete the display list needed to render the image. 00275 WrCmd32(CMD_LOADIMAGE); 00276 WrCmd32(_address); //destination address of png decode 00277 // 0 OPT_RGB565 00278 // 1 OPT_MONO 00279 // 2 OPT_NODL 00280 // 256 OPT_FLAT 00281 // 256 OPT_SIGNED 00282 // 512 OPT_CENTERX 00283 // 1024 OPT_CENTERY 00284 // 1536 OPT_CENTER 00285 // 2048 OPT_RIGHTX 00286 // 4096 OPT_NOBACK 00287 // 8192 OPT_NOTICKS 00288 // By default, option OPT_RGB565 means the loaded bitmap is in RGB565 format. 00289 00290 // Keep track of of the start addresses of loaded images 00291 // Increment _address 00292 _addresses[_bitmap_count] = _address; 00293 _bitmap_count++; 00294 _address += (uint32_t) (size_x * size_y * 2); 00295 _addresses[_bitmap_count] = _address; 00296 printf("LoadPng: Bitmap# %d, Res. %dx%d, Address %d-%d\n", _bitmap_count - 1, *x_size, *y_size, _addresses[_bitmap_count-1], _addresses[_bitmap_count]); 00297 00298 WrCmd32(OPT_RGB565); // Output format of the bitmap OPT_RGB565 00299 // unsigned int filesizeCounter = filesize; 00300 char* pbuff = (char*)malloc(bufferSize); 00301 while(filesize > 0) { 00302 blocklen = filesize > bufferSize ? bufferSize : filesize; 00303 int size = fread(pbuff, 1, blocklen, fp); 00304 filesize -= blocklen; 00305 00306 WrCmdBuf((uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00307 } 00308 fclose(fp); 00309 // If the number of bytes in the JPEG file to be written to the command buffer is not a multiple of 00310 // four, then one, two or three bytes (of any value) should be added to ensure four-byte alignment of 00311 // the next command. 00312 // blocklen = filesize % 4; 00313 // memset(pbuff, 0, blocklen); 00314 // WrCmdBuf((uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00315 00316 free(pbuff); 00317 00318 printf("LoadPng: Done.\n"); 00319 00320 return(0); 00321 } 00322 00323 int FT813::LoadRaw(char* filename) 00324 { 00325 ft_uint16_t filesize = 0; 00326 ft_uint16_t blocklen; 00327 ft_uint16_t ram_start = _address; 00328 int bufferSize = 8192; 00329 00330 FILE *fp = fopen(filename, "rb"); // open file 00331 if (fp == NULL) 00332 { 00333 // Cannot open file 00334 printf("Unable to open: %s\n", filename); 00335 return -1; 00336 } 00337 fseek(fp, 0, SEEK_END); // set file position to end of file 00338 filesize = ftell(fp); // determine file size 00339 fseek(fp, 0, SEEK_SET); // return to beginning of file 00340 00341 // Keep track of of the start addresses of loaded images 00342 // Increment _address 00343 _addresses[_bitmap_count] = _address; 00344 _bitmap_count++; 00345 _address += (uint32_t) filesize; 00346 _addresses[_bitmap_count] = _address; 00347 printf("LoadRaw: Bitmap# %d, Address %d-%d\n", _bitmap_count - 1, _addresses[_bitmap_count-1], _addresses[_bitmap_count]); 00348 00349 char* pbuff = (char*)malloc(bufferSize); 00350 while(filesize > 0) 00351 { 00352 //copy the .raw file data to pbuff[8192] in 8k block 00353 blocklen = filesize > bufferSize ? bufferSize : filesize; 00354 fread(pbuff, 1, blocklen, fp); 00355 filesize -= blocklen; 00356 //write pbuff contents to graphics RAM at address ram_start = 0x00 00357 Wr8s(ram_start, (uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00358 ram_start += blocklen; 00359 } 00360 fclose(fp); 00361 free(pbuff); 00362 return 0; 00363 } 00364 00365 int FT813::LoadRawFile(ft_uint32_t address, const char *filename) 00366 { 00367 int bufferSize = 8192; 00368 ft_uint32_t filesize = 0; 00369 ft_uint16_t blocklen = 0; 00370 00371 _address = address; 00372 ft_uint32_t addr = address; 00373 00374 FILE *fp = fopen(filename, "rb"); 00375 if (fp == NULL) 00376 { 00377 printf("LoadRawFile: Cannot open file \"%s\".\n", filename); 00378 return 0; 00379 } 00380 fseek(fp, 0, SEEK_END); // End of file 00381 filesize = ftell(fp); 00382 fseek(fp, 0, SEEK_SET); // Beginning of file 00383 00384 // Keep track of of the start addresses of loaded images 00385 // Increment _address 00386 _addresses[_bitmap_count] = _address; 00387 _bitmap_count++; 00388 _address += (uint32_t) filesize; 00389 _addresses[_bitmap_count] = _address; 00390 printf("LoadRawFile: Bitmap# %d, Address %d-%d\n", _bitmap_count - 1, _addresses[_bitmap_count-1], _addresses[_bitmap_count]); 00391 00392 char* pbuff = (char*)malloc(bufferSize); 00393 while (filesize > 0) 00394 { 00395 blocklen = filesize > bufferSize ? bufferSize : filesize; 00396 fread(pbuff, 1, blocklen, fp); 00397 filesize -= blocklen; 00398 WrMem(addr, (ft_uint8_t *)pbuff, blocklen); 00399 addr += blocklen; 00400 // WrCmdBuf((ft_uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00401 } 00402 fclose(fp); 00403 free(pbuff); 00404 00405 printf("LoadRawFile: Done.\n"); 00406 return 1; 00407 } 00408 00409 int FT813::LoadInflateFile(ft_uint32_t address, const char *filename) 00410 { 00411 int bufferSize = 8192; 00412 ft_uint32_t filesize = 0; 00413 ft_uint16_t blocklen = 0; 00414 ft_uint32_t addr = address; 00415 ft_uint32_t filesize_org = address; 00416 00417 _address = address; 00418 00419 FILE *fp = fopen(filename, "rb"); // read Binary (rb) 00420 if (fp == NULL) 00421 { 00422 printf("LoadInflateFile: Cannot open file \"%s\".\n", filename); 00423 return 0; 00424 } 00425 fseek(fp, 0, SEEK_END); // End of file 00426 filesize = ftell(fp); 00427 fseek(fp, 0, SEEK_SET); // Beginning of file 00428 00429 // Keep track of of the start addresses of loaded images 00430 // Increment _address 00431 _addresses[_bitmap_count] = _address; 00432 _bitmap_count++; 00433 // _address += (uint32_t) 0; 00434 _addresses[_bitmap_count] = _address; 00435 // printf("# %d, Addr %d\n", _bitmap_count - 1, _addresses[_bitmap_count-1]); 00436 00437 WrCmd32(CMD_INFLATE); 00438 WrCmd32(address); //destination address of bin decode 00439 00440 char* pbuff = (char*)malloc(bufferSize); 00441 while (filesize > 0) 00442 { 00443 blocklen = filesize > bufferSize ? bufferSize : filesize; 00444 int size = fread(pbuff, 1, blocklen, fp); /* copy the data into pbuff and then transfter it to command buffer */ 00445 filesize -= blocklen; 00446 00447 // WrMem(addr, (ft_uint8_t *)pbuff, blocklen); 00448 // addr += blocklen; 00449 00450 //Do not call "Ft_Gpu_Hal_WrCmdBuf_nowait" because it may cause emulator process hang. 00451 WrCmdBuf((ft_uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00452 // WaitCmdfifo_empty(); 00453 } 00454 // If the number of bytes in the JPEG file to be written to the command buffer is not a multiple of 00455 // four, then one, two or three bytes (of any value) should be added to ensure four-byte alignment of 00456 // the next command. 00457 // blocklen = filesize_org % 4; 00458 // memset(pbuff, 0, blocklen); 00459 // WrMem(addr, (ft_uint8_t *)pbuff, blocklen); 00460 // WrCmdBuf((uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00461 00462 // WaitCmdfifo_empty(); 00463 00464 fclose(fp); /* close the opened jpg file */ 00465 free(pbuff); 00466 return 1; 00467 } 00468 00469 int FT813::LoadImageFile(ft_uint32_t address, const char *filename) 00470 { 00471 int bufferSize = 8192; 00472 ft_uint32_t filesize = 0; 00473 ft_uint16_t blocklen = 0; 00474 00475 _address = address; 00476 00477 FILE *fp = fopen(filename, "rb"); // read Binary (rb) 00478 if (fp == NULL) 00479 { 00480 printf("LoadImageFile: Cannot open file \"%s\".\n", filename); 00481 return 0; 00482 } 00483 // TODO: Let it write into the scratch display list handle, 00484 // and read it out and write into the bitmapInfo the proper 00485 // values to use. Replace compressed bool with uint8 enum to 00486 // specify the loading mechanism 00487 fseek(fp, 0, SEEK_END); // End of file 00488 filesize = ftell(fp); 00489 fseek(fp, 0, SEEK_SET); // Beginning of file 00490 00491 // Keep track of of the start addresses of loaded images 00492 // Increment _address 00493 _addresses[_bitmap_count] = _address; 00494 _bitmap_count++; 00495 _address += (uint32_t) filesize; 00496 _addresses[_bitmap_count] = _address; 00497 printf("LoadImageFile: Bitmap# %d, Address %d-%d\n", _bitmap_count - 1, _addresses[_bitmap_count-1], _addresses[_bitmap_count]); 00498 00499 WrCmd32(CMD_LOADIMAGE); 00500 WrCmd32(address); //destination address of png decode 00501 // 0 OPT_RGB565 00502 // 1 OPT_MONO 00503 // 2 OPT_NODL 00504 // 256 OPT_FLAT 00505 // 256 OPT_SIGNED 00506 // 512 OPT_CENTERX 00507 // 1024 OPT_CENTERY 00508 // 1536 OPT_CENTER 00509 // 2048 OPT_RIGHTX 00510 // 4096 OPT_NOBACK 00511 // 8192 OPT_NOTICKS 00512 // By default, option OPT_RGB565 means the loaded bitmap is in RGB565 format. 00513 WrCmd32(OPT_RGB565); // Output format of the bitmap OPT_RGB565 00514 // WrCmd32(OPT_NODL); 00515 char* pbuff = (char*)malloc(bufferSize); 00516 while (filesize > 0) 00517 { 00518 blocklen = filesize > bufferSize ? bufferSize : filesize; 00519 int size = fread(pbuff, 1, blocklen, fp); /* copy the data into pbuff and then transfter it to command buffer */ 00520 filesize -= blocklen; 00521 // blocklen += 3; 00522 // blocklen -= blocklen % 4; 00523 00524 //Do not call "Ft_Gpu_Hal_WrCmdBuf_nowait" because it may cause emulator process hang. 00525 WrCmdBuf((ft_uint8_t *)pbuff, blocklen); //alignment is already taken care by this api 00526 } 00527 // WaitCmdfifo_empty(); 00528 00529 fclose(fp); /* close the opened jpg file */ 00530 free(pbuff); 00531 return 1; 00532 } 00533 00534 ft_void_t FT813::SetEndAddressForSize(ft_uint32_t addr) 00535 { 00536 _addresses[_bitmap_count] = _addresses[_bitmap_count - 1] + addr; 00537 } 00538 00539 ft_void_t FT813::Wr8s(ft_uint32_t addr, ft_uint8_t *buffer, ft_uint8_t length) 00540 { 00541 // StartTransfer(FT_GPU_WRITE, addr); 00542 // if (FT_GPU_READ == rw) { // if (FT_GPU_READ == FT_GPU_WRITE) 00543 // _ss = 0; // cs low 00544 // _spi.write(addr >> 16); 00545 // _spi.write(addr >> 8); 00546 // _spi.write(addr & 0xff); 00547 // _spi.write(0); //Dummy Read Byte 00548 // status = READING; 00549 // } else { 00550 _ss = 0; // cs low 00551 _spi.write(0x80 | (addr >> 16)); 00552 _spi.write(addr >> 8); 00553 _spi.write(addr & 0xff); 00554 status = WRITING; 00555 // } 00556 00557 // Transfer8(v); 00558 // _spi.write(v); 00559 00560 while (length--) { 00561 // Transfer8(*buffer); 00562 _spi.write(*buffer); 00563 buffer++; 00564 // SizeTransfered++; 00565 } 00566 00567 // EndTransfer(); 00568 _ss = 1; 00569 status = OPENED; 00570 } 00571 00572 //{ 00573 //ft_uint8_t imbuff[8192]; 00574 //ft_uint16_t blocklen; 00575 ////decompress the .bin file using CMD_INFLATE 00576 //WrCmd32(phost,CMD_INFLATE); 00577 ////specify starting address in graphics RAM 00578 //WrCmd32(phost,0L); 00579 ////check filesize and adjust number of bytes to multiple of 4 00580 //chdir("..\\..\\..\\Test"); //change directory to location (Test) of .bin file 00581 //pfile = fopen("lenaface40.bin","rb"); 00582 //fseek(pfile,0,SEEK_END); //set file position to end of file 00583 //filesize = ftell(pfile); // determine file size 00584 //fseek(pfile,0,SEEK_SET); // return to beginning of file 00585 //while(filesize > 0) 00586 //{ 00587 // //copy the .raw file data to imbuff[8192] in 8k blocks 00588 //blocklen = filesize>8192?8192:filesize; 00589 //fread(imbuff,1,blocklen,pfile); 00590 //filesize -= blocklen; //reduce filesize by blocklen 00591 ////write imbuff contents to the FT800 FIFO command buffer 00592 //Ft_Gpu_Hal_WrCmdBuf(phost,imbuff,blocklen); 00593 //} 00594 //fclose(pfile); /* close the opened .bin file */ 00595 //} 00596 00597 int FT813::LoadRawFile(ft_uint32_t address, char* filename) 00598 { 00599 ft_uint16_t filesize; 00600 ft_uint16_t blocklen; 00601 ft_uint32_t addr = address; 00602 int bufferSize = 8192; 00603 00604 FILE *fp = fopen(filename, "rb"); // open file 00605 if (fp == NULL) 00606 { 00607 // Cannot open file 00608 printf("Unable to open: %s\n", filename); 00609 return -1; 00610 } 00611 fseek(fp, 0, SEEK_END); // set file position to end of file 00612 filesize = ftell(fp); // determine file size 00613 fseek(fp, 0, SEEK_SET); // return to beginning of file 00614 #ifdef DEBUG 00615 printf("LoadRawFile: %s %d @ %d\n", filename, filesize, address); 00616 #endif 00617 00618 char* pbuff = (char*)malloc(bufferSize); 00619 while(filesize > 0) 00620 { 00621 //copy the .raw file data to pbuff[8192] in 8k block 00622 blocklen = filesize > bufferSize ? bufferSize : filesize; 00623 fread(pbuff, 1, blocklen, fp); 00624 filesize -= blocklen; 00625 //write pbuff contents to graphics RAM at addr 00626 WrMem(addr, (ft_uint8_t *)pbuff, blocklen); 00627 addr += blocklen; 00628 } 00629 fclose(fp); 00630 free(pbuff); 00631 return 0; 00632 } 00633 00634 void FT813::FillBitmap(ft_int16_t bitmap_number) 00635 { 00636 int bufferSize = 8192; 00637 uint8_t* pbuff = (uint8_t*)malloc(bufferSize); 00638 // Clear buffer 00639 memset(pbuff, 0, bufferSize); 00640 00641 ft_int32_t addr_start = _addresses[bitmap_number]; 00642 ft_int32_t addr_end = _addresses[bitmap_number+1]; 00643 printf("FillBitmap %d (%d - %d).\n", bitmap_number, addr_start, addr_end); 00644 // ft_int32_t bufferSize = addr_end - addr_start; 00645 ft_int32_t filesize = addr_end - addr_start; 00646 // ft_int32_t addr = addr_start; 00647 00648 WrCmd32(CMD_LOADIMAGE); 00649 WrCmd32(addr_start); //destination address of png decode 00650 WrCmd32(0); //output format of the bitmap - default is rgb565 00651 printf("filesize = %d\n", filesize); 00652 printf("while(filesize > 0)...\n"); 00653 while(filesize > 0) { 00654 ft_uint16_t blocklen = filesize > bufferSize ? bufferSize : filesize; 00655 // printf("WrCmdBuf %d.\n", bufferSize); 00656 filesize -= blocklen; 00657 printf("blocklen = %d\n", blocklen); 00658 printf("WrCmdBuf...\n"); 00659 WrCmdBuf(pbuff, blocklen); //alignment is already taken care by this api 00660 printf("Done.\n"); 00661 } 00662 printf("free(pbuff)...\n"); 00663 free(pbuff); 00664 printf("Done.\n"); 00665 } 00666 00667 void FT813::ClearBitmapCount(void) 00668 { 00669 _bitmap_count = 0; 00670 } 00671 00672 ft_uint32_t FT813::ReadBigInt32(unsigned char* data, ft_uint32_t offset) 00673 { 00674 return (data[offset + 0] << 24 | data[24 - 4 + 1] << 16 | data[offset + 2] << 8 | data[offset + 3]); 00675 } 00676 00677 // Loads JPG at x, y on the screen 00678 ft_uint8_t FT813::Jpg(char *jpg_filename, int x, int y) 00679 { 00680 ft_int16_t x_size,y_size; 00681 int err; 00682 00683 err = LoadJpg(jpg_filename, & x_size, & y_size); // load graphic data into buffer and decode jpg to bitmap 00684 if(err != 0) { // something is wrong - display error 00685 printf("LoadJpg: Error.\n"); 00686 return(-1); 00687 } 00688 DL(BEGIN(BITMAPS)); 00689 // LoadIdentity(); 00690 // SetMatrix(); 00691 DL(VERTEX2F(x * 16, y * 16)); 00692 00693 // jpg is loaded and decoded into bitmap 00694 // printf("jpg %s is loaded and decoded into bitmap (%dx%d)\n", jpg_filename, x_size, y_size); 00695 00696 return(0); 00697 } 00698 00699 // Loads PNG at x, y on the screen 00700 ft_uint8_t FT813::Png(char *png_filename, int x, int y) 00701 { 00702 ft_int16_t x_size,y_size; 00703 int err; 00704 00705 err = LoadPng(png_filename, & x_size, & y_size); // load graphic data into buffer and decode png to bitmap 00706 if(err != 0) { // something is wrong - display error 00707 printf("LoadPng: Error.\n"); 00708 return(-1); 00709 } 00710 DL(BEGIN(BITMAPS)); 00711 // LoadIdentity(); 00712 // SetMatrix(); 00713 DL(VERTEX2F(x * 16, y * 16)); 00714 00715 // png is loaded and decoded into bitmap 00716 // printf("png %s is loaded and decoded into bitmap (%dx%d)\n", png_filename, x_size, y_size); 00717 00718 return(0); 00719 } 00720 00721 // Loads PNG at x, y on the screen at given address 00722 ft_uint8_t FT813::Png(char *png_filename, int x, int y, ft_int32_t address) 00723 { 00724 ft_int16_t x_size,y_size; 00725 int err; 00726 _address = address; 00727 err = LoadPng(png_filename, & x_size, & y_size); // load graphic data into buffer and decode png to bitmap 00728 if(err != 0) { // something is wrong - display error 00729 printf("LoadPng: Error.\n"); 00730 return(-1); 00731 } 00732 DL(BEGIN(BITMAPS)); 00733 // LoadIdentity(); 00734 // SetMatrix(); 00735 DL(VERTEX2F(x * 16, y * 16)); 00736 00737 // DL(DISPLAY()); //ends the display,commands after this ignored 00738 00739 // png is loaded and decoded into bitmap 00740 printf("png %s is loaded and decoded into bitmap (%dx%d)\n", png_filename, x_size, y_size); 00741 00742 return(0); 00743 } 00744 00745 // Loads JPG at the center of the screen (splash screen) 00746 // Background color specified 00747 ft_uint8_t FT813::JpgSplash(char *jpg_filename, ft_uint8_t r, ft_uint8_t g, ft_uint8_t b) 00748 { 00749 ft_int16_t x_size,y_size; 00750 int err; 00751 00752 DLstart(); // start a new display command list 00753 DL(CLEAR_COLOR_RGB(r, g, b)); // set the clear color to white 00754 DL(CLEAR(1, 1, 1)); // clear buffers -> color buffer,stencil buffer, tag buffer 00755 00756 err = LoadJpg(jpg_filename, & x_size, & y_size); // load graphic data into buffer and decode jpg to bitmap 00757 if(err != 0) { // something is wrong - display error 00758 printf("LoadJpg: Error.\n"); 00759 return(-1); 00760 } 00761 00762 DL(BEGIN(BITMAPS)); 00763 LoadIdentity(); 00764 SetMatrix(); 00765 int x = (DispWidth - x_size) / 2; 00766 int y = (DispHeight - y_size) / 2; 00767 DL(VERTEX2F(x * 16, y * 16)); 00768 00769 DL(DISPLAY()); // Display the image 00770 Swap(); // Swap the current display list 00771 Flush_Co_Buffer(); // Download the command list into fifo 00772 WaitCmdfifo_empty(); // Wait till coprocessor completes the operation 00773 00774 return(0); 00775 } 00776 00777 // *************************************************************************************************************** 00778 // *** Utility and helper functions ****************************************************************************** 00779 // *************************************************************************************************************** 00780 00781 // Find the space available in the GPU AKA CoProcessor AKA command buffer AKA FIFO 00782 ft_uint16_t FT813::CoProFIFO_FreeSpace(void) 00783 { 00784 ft_uint16_t cmdBufferDiff, cmdBufferRd, cmdBufferWr, retval; 00785 00786 cmdBufferRd = Rd16(REG_CMD_READ + RAM_REG); 00787 cmdBufferWr = Rd16(REG_CMD_WRITE + RAM_REG); 00788 00789 cmdBufferDiff = (cmdBufferWr-cmdBufferRd) % FT_CMD_FIFO_SIZE; // FT81x Programmers Guide 5.1.1 00790 retval = (FT_CMD_FIFO_SIZE - 4) - cmdBufferDiff; 00791 return (retval); 00792 } 00793 00794 // Sit and wait until there are the specified number of bytes free in the <GPU/CoProcessor> incoming FIFO 00795 void FT813::Wait4CoProFIFO(ft_uint32_t room) 00796 { 00797 ft_uint16_t getfreespace; 00798 00799 do { 00800 getfreespace = CoProFIFO_FreeSpace(); 00801 } while(getfreespace < room); 00802 } 00803 00804 // Sit and wait until the CoPro FIFO is empty 00805 void FT813::Wait4CoProFIFOEmpty(void) 00806 { 00807 while(Rd16(REG_CMD_READ + RAM_REG) != Rd16(REG_CMD_WRITE + RAM_REG)); 00808 } 00809 00810 // Check if the CoPro FIFO is empty 00811 // returns 1 if the CoPro FIFO is empty 00812 // returns 0 if the CoPro FIFO is not empty 00813 ft_uint8_t FT813::CheckIfCoProFIFOEmpty(void) 00814 { 00815 return (Rd16(REG_CMD_READ + RAM_REG) == Rd16(REG_CMD_WRITE + RAM_REG)) ? 1 : 0; 00816 } 00817 00818 // Write a block of data into Eve RAM space a byte at a time. 00819 // Return the last written address + 1 (The next available RAM address) 00820 ft_uint32_t FT813::WriteBlockRAM(ft_uint32_t Add, const ft_uint8_t *buff, ft_uint32_t count) 00821 { 00822 ft_uint32_t index; 00823 ft_uint32_t WriteAddress = Add; // I want to return the value instead of modifying the variable in place 00824 00825 for (index = 0; index < count; index++) { 00826 Wr8(WriteAddress++, buff[index]); 00827 } 00828 return (WriteAddress); 00829 } 00830 00831 /* function to load jpg file from filesystem */ 00832 /* return 0 if jpg is ok */ 00833 /* return x_size and y_size of jpg */ 00834 00835 //int FT813::Load_jpg(char* filename, ft_int16_t* x_size, ft_int16_t* y_size, ft_uint32_t address) 00836 //{ 00837 // unsigned char pbuff[8192]; 00838 // unsigned short marker; 00839 // unsigned short length; 00840 // unsigned char data[4]; 00841 // 00842 // ft_uint16_t blocklen; 00843 //// sd.mount(); 00844 // FILE *fp = fopen(filename, "r"); 00845 // if(fp == NULL) return (-1); // connot open file 00846 // 00847 // // search for 0xFFC0 marker 00848 // fseek(fp, 0, SEEK_END); 00849 // unsigned int filesize = ftell(fp); 00850 // fseek(fp, 2, SEEK_SET); 00851 // fread(data,4,1,fp); 00852 // marker = data[0] << 8 | data[1]; 00853 // length = data[2] << 8 | data[3]; 00854 // do { 00855 // if(marker == 0xFFC0) break; 00856 // if(marker & 0xFF00 != 0xFF00) break; 00857 // if (fseek(fp, length - 2,SEEK_CUR) != 0) break; 00858 // fread(data,4,1,fp); 00859 // marker = data[0] << 8 | data[1]; 00860 // length = data[2] << 8 | data[3]; 00861 // } while(1); 00862 // if(marker != 0xFFC0) return (-2); // no FFC0 Marker, wrong format no baseline DCT-based JPEG 00863 // fseek(fp, 1,SEEK_CUR); 00864 // fread(data,4,1,fp); 00865 // *y_size = (data[0] << 8 | data[1]); 00866 // *x_size = (data[2] << 8 | data[3]); 00867 // 00868 // //if(*x_size > DispWidth || *y_size > DispHeight) return (-3); // to big to fit on screen 00869 // 00870 // fseek(fp, 0, SEEK_SET); 00871 // WrCmd32(CMD_LOADIMAGE); // load a JPEG image 00872 // WrCmd32(address); //destination address of jpg decode 00873 // WrCmd32(0); //output format of the bitmap - default is rgb565 00874 // while(filesize > 0) { 00875 // /* download the data into the command buffer by 8kb one shot */ 00876 // blocklen = filesize>8192?8192:filesize; 00877 // /* copy the data into pbuff and then transfter it to command buffer */ 00878 // fread(pbuff,1,blocklen,fp); 00879 // filesize -= blocklen; 00880 // /* copy data continuously into command memory */ 00881 // WrCmdBuf(pbuff, blocklen); //alignment is already taken care by this api 00882 // } 00883 // fclose(fp); 00884 //// sd.unmount(); 00885 // 00886 // return(0); 00887 //} 00888 00889 //int FT813::Load_raw(char* filename) 00890 //{ 00891 // ft_uint8_t imbuff[8192]; 00892 // ft_uint16_t filesize; 00893 // ft_uint16_t blocklen; 00894 //// ft_uint16_t ram_start = 0x00; 00895 // 00896 //// sd.mount(); 00897 // FILE *fp = fopen(filename, "rb"); // open file 00898 //// if(fp == NULL) return (-1); // connot open file 00899 // fseek(fp, 0, SEEK_END); // set file position to end of file 00900 // filesize= ftell(fp); // determine file size 00901 // fseek(fp, 2, SEEK_SET); // return to beginning of file 00902 // 00903 // while(filesize > 0) 00904 // { 00905 // //copy the .raw file data to imbuff[8192] in 8k block 00906 // blocklen = filesize>8192?8192:filesize; 00907 // fread(imbuff,1,blocklen,fp); 00908 // filesize-= blocklen; 00909 // //write imbuff contents to graphics RAM at address ram_start = 0x00 00910 // WrCmdBuf(imbuff, blocklen); //alignment is already taken care by this api 00911 //// ram_start += 8192; 00912 // } 00913 // fclose(fp); 00914 //// sd.unmount(); 00915 // 00916 // return 0; 00917 //} 00918 00919 /* calibrate touch */ 00920 ft_void_t FT813::Calibrate() 00921 { 00922 /*************************************************************************/ 00923 /* Below code demonstrates the usage of calibrate function. Calibrate */ 00924 /* function will wait untill user presses all the three dots. Only way to*/ 00925 /* come out of this api is to reset the coprocessor bit. */ 00926 /*************************************************************************/ 00927 DLstart(); // start a new display command list 00928 DL(CLEAR_COLOR_RGB(64,64,64)); // set the clear color R, G, B 00929 DL(CLEAR(1,1,1)); // clear buffers -> color buffer,stencil buffer, tag buffer 00930 DL(COLOR_RGB(0xff,0xff,0xff)); // set the current color R, G, B 00931 Text((DispWidth/2), (DispHeight/2), 27, OPT_CENTER, "Please tap on the dot"); // draw Text at x,y, font 27, centered 00932 Calibrate(0); // start the calibration of touch screen 00933 Flush_Co_Buffer(); // download the commands into FT813 FIFO 00934 WaitCmdfifo_empty(); // Wait till coprocessor completes the operation 00935 } 00936 00937 00938 /* API to give fadeout effect by changing the display PWM from 100 till 0 */ 00939 ft_void_t FT813::fadeout() 00940 { 00941 ft_int32_t i; 00942 00943 for (i = 100; i >= 0; i -= 3) 00944 { 00945 Wr8(REG_PWM_DUTY, i); 00946 Sleep(2);//sleep for 2 ms 00947 } 00948 } 00949 00950 /* API to perform display fadein effect by changing the display PWM from 0 till 100 and finally 128 */ 00951 ft_void_t FT813::fadein() 00952 { 00953 ft_int32_t i; 00954 00955 for (i = 0; i <=100 ; i += 3) 00956 { 00957 Wr8(REG_PWM_DUTY,i); 00958 Sleep(2);//sleep for 2 ms 00959 } 00960 /* Finally make the PWM 100% */ 00961 i = 128; 00962 Wr8(REG_PWM_DUTY,i); 00963 } 00964 00965 ft_uint8_t FT813::read_calibrate_reg(ft_uint8_t i) { 00966 return Rd8(REG_TOUCH_TRANSFORM_A + i); 00967 } 00968 00969 ft_uint32_t FT813::read_calibrate_reg32(ft_uint8_t i) { 00970 return (Rd8(REG_TOUCH_TRANSFORM_A + i)) + // lsb 00971 (Rd8(REG_TOUCH_TRANSFORM_A + i+1) << 8) + 00972 (Rd8(REG_TOUCH_TRANSFORM_A + i+2) << 16) + 00973 (Rd8(REG_TOUCH_TRANSFORM_A + i+3) << 24); // msb 00974 } 00975 00976 ft_void_t FT813::read_calibrate(ft_uint8_t data[24]) { 00977 unsigned int i; 00978 for(i = 0; i < 24; i++) { 00979 data[i] = Rd8(REG_TOUCH_TRANSFORM_A + i); 00980 } 00981 } 00982 00983 ft_void_t FT813::write_calibrate(ft_uint8_t data[24]) { 00984 unsigned int i; 00985 for(i = 0; i < 24; i++) { 00986 Wr8(REG_TOUCH_TRANSFORM_A + i, data[i]); 00987 } 00988 } 00989 00990 ft_void_t FT813::write_calibrate32(ft_uint32_t data[6]) { 00991 unsigned int i; 00992 for(i = 0; i < 6; i++) { 00993 Wr8(REG_TOUCH_TRANSFORM_A + i*4, (data[i]) & 0xff); // lsb 00994 Wr8(REG_TOUCH_TRANSFORM_A + i*4 + 1, (data[i] >> 8) & 0xff); 00995 Wr8(REG_TOUCH_TRANSFORM_A + i*4 + 2, (data[i] >> 16) & 0xff); 00996 Wr8(REG_TOUCH_TRANSFORM_A + i*4 + 3, (data[i] >> 24) & 0xff); // msb 00997 } 00998 } 00999 01000 ft_uint32_t FT813::color_rgb(ft_uint8_t red, ft_uint8_t green, ft_uint8_t blue){ 01001 return ((4UL<<24)|(((red)&255UL)<<16)|(((green)&255UL)<<8)|(((blue)&255UL)<<0)); 01002 } 01003 01004 ft_uint32_t FT813::clear_color_rgb(ft_uint8_t red, ft_uint8_t green, ft_uint8_t blue){ 01005 return ((2UL<<24)|(((red)&255UL)<<16)|(((green)&255UL)<<8)|(((blue)&255UL)<<0)); 01006 } 01007 01008 // Define the backlight PWM output duty cycle. 01009 void FT813::SetBacklight(ft_uint16_t brightness) 01010 { 01011 brightness = brightness * brightness / 255; 01012 if (brightness > 256) 01013 brightness = 256; 01014 if (brightness < 16) 01015 brightness = 16; 01016 Wr16(REG_PWM_DUTY, brightness); // Brightness 01017 } 01018 01019 void FT813::Tag(ft_uint8_t tag) 01020 { 01021 DL(TAG(tag)); 01022 } 01023 01024 void FT813::ClearTag(ft_uint8_t tag) 01025 { 01026 DL(CLEAR_TAG(tag)); 01027 } 01028 01029 void FT813::TagMask(ft_uint8_t mask) 01030 { 01031 DL(TAG_MASK(mask)); 01032 } 01033 01034 void FT813::BitmapLayoutH(ft_uint8_t linestride, ft_uint8_t height) 01035 { 01036 DL((40 << 24) | (((linestride) & 3) << 2) | (((height) & 3) << 0)); 01037 } 01038 01039 void FT813::BitmapSizeH(ft_uint8_t width, ft_uint8_t height) 01040 { 01041 DL((41UL << 24) | (((width) & 3) << 2) | (((height) & 3) << 0)); 01042 } 01043 01044 ft_void_t FT813::SetLoadAddress(ft_uint32_t address) 01045 { 01046 _address = address; // Old, try to get rid of this 01047 _bitmapAddress = address; 01048 } 01049 01050 ft_void_t FT813::SetBitmapCount(ft_uint8_t count) 01051 { 01052 _bitmap_count = count; // Old, try to get rid of this 01053 _bitmapCount = count; 01054 } 01055 01056 ft_uint32_t FT813::GetBitmapAddress(ft_uint8_t bitmap_number) 01057 { 01058 return _addresses[bitmap_number]; 01059 } 01060 01061 void FT813::SetThemeDefaultColor(void) 01062 { 01063 // Default 01064 GradColor(COLOR_RGB(0xff, 0xff, 0xff)); // Default 0xffffff 01065 FgColor(COLOR_RGB(0x00, 0x38, 0x70)); // Default 0x003870 (0, 56, 112) 01066 BgColor(COLOR_RGB(0x00, 0x20, 0x40)); // Default 0x002040 (0, 32, 64) 01067 } 01068 01069 void FT813::SetThemeColor(ft_uint32_t c) 01070 { 01071 GradColor(COLOR_RGB(0xff, 0xff, 0xff)); // Default 0xffffff 01072 ft_uint8_t r = (c >> 16) & 0xff; 01073 ft_uint8_t g = (c >> 8) & 0xff; 01074 ft_uint8_t b = c & 0xff; 01075 ft_uint8_t rfg = r * 112 / 255; 01076 ft_uint8_t gfg = g * 112 / 255; 01077 ft_uint8_t bfg = b * 112 / 255; 01078 ft_uint8_t rbg = r * 64 / 255; 01079 ft_uint8_t gbg = g * 64 / 255; 01080 ft_uint8_t bbg = b * 64 / 255; 01081 FgColor(COLOR_RGB(rfg, gfg, bfg)); // Default 0x003870 01082 BgColor(COLOR_RGB(rbg, gbg, bbg)); // Default 0x002040 01083 } 01084 01085 // ShowCalibration 01086 void FT813::ShowCalibrationInCode(void) 01087 { 01088 // Read calibrate registers 01089 printf("// Calibration values:\n"); 01090 printf(" ft_uint32_t canned_calibration_data[] = {\n"); 01091 for(int i = 0; i < 24; i+=4) { 01092 printf(" "); 01093 printf("0x%08x", read_calibrate_reg32(i)); 01094 if (i < 20) 01095 printf(","); 01096 printf("\n"); 01097 } 01098 printf(" };\n"); 01099 printf(" write_calibrate32(canned_calibration_data);\n"); 01100 } 01101 01102 // Set screen orientation and preload canned touch screen calibration values 01103 void FT813::SetOrientation(ft_uint8_t orientation) 01104 { 01105 // TFT.SetRotate(0); // Standard landscape 01106 // TFT.SetRotate(1); // Rotate 180 to landscape (upside down) 01107 // TFT.SetRotate(2); // Rotate 90 CCW to portrait 01108 // TFT.SetRotate(3); // Rotate 90 CW to portrait 01109 // TFT.SetRotate(4); // Mirror over landscape X 01110 // TFT.SetRotate(5); // Mirror over landscape Y 01111 // TFT.SetRotate(6); // Rotate 90 CCW to portrait and mirror over portrait X 01112 // TFT.SetRotate(7); // Rotate 90 CW to portrait and mirror over portrait X 01113 01114 _orientation = orientation; 01115 01116 SetRotate(orientation); // Standard landscape 01117 // Canned calibration for the orientation 01118 01119 if (orientation == FT8_DISPLAY_LANDSCAPE_0) 01120 { 01121 // Landscape 0 01122 ft_uint32_t canned_calibration_data[] = { 01123 0x000109b0, // 68016 01124 0x0000023d, // 573 01125 0x0000fa64, // 64100 01126 0xffffffcf, // -49 01127 0xfffefc9a, // -66406 01128 0x01ee8754 // 32409428 01129 }; 01130 write_calibrate32(canned_calibration_data); 01131 } 01132 01133 if (orientation == FT8_DISPLAY_PORTRAIT_90CW) 01134 { 01135 ft_uint32_t canned_calibration_data[] = { 01136 0xfffff994, 01137 0xffff07d3, 01138 0x01e4b85c, 01139 0xfffef6f5, 01140 0x000002ad, 01141 0x032eb4d4 01142 }; 01143 write_calibrate32(canned_calibration_data); 01144 } 01145 01146 if (orientation == FT8_DISPLAY_PORTRAIT_90CCW) 01147 { 01148 // Calibration rotate 90 CCW to portrait 01149 ft_uint32_t canned_calibration_data[] = { 01150 0x00000491, 01151 0x0000fd0b, 01152 0xfff6f84b, 01153 0x00010503, 01154 0x000006b7, 01155 0xffeeb0b7 01156 }; 01157 write_calibrate32(canned_calibration_data); 01158 } 01159 } 01160 01161 void FT813::ShowBitmap(ft_uint8_t bitmap, ft_int16_t fmt, ft_uint16_t x, ft_uint16_t y, ft_uint16_t width, ft_uint16_t height) 01162 { 01163 ft_int32_t addr = GetBitmapAddress(bitmap); 01164 DL(BITMAP_SOURCE(addr)); 01165 // ft_int16_t stride = width * 2; 01166 // 1-bits/p L1 01167 // if (fmt == L1) { 01168 // stride = width / 8; 01169 // } 01170 // 2-bits/p L2 01171 // if (fmt == L2) { 01172 // stride = width / 4; 01173 // } 01174 // 4-bits/p L4 01175 // if (fmt == L4) { 01176 // stride = width / 2; 01177 // } 01178 // 8-bits/p L8 RGB332 ARGB2 PALETTED565 PALETTED4444 PALETTED8 01179 // if ((fmt == L8) || 01180 // (fmt == RGB332) || 01181 // (fmt == ARGB2) || 01182 // (fmt == PALETTED565) || 01183 // (fmt == PALETTED4444) || 01184 // (fmt == PALETTED8)) { 01185 // stride = width; 01186 // } 01187 // 16-bits/p ARGB1555 ARGB4 RGB565 01188 // if ((fmt == ARGB1555) || 01189 // (fmt == ARGB4) || 01190 // (fmt == RGB565)) { 01191 // stride = width * 2; 01192 // } 01193 // DL(BITMAP_LAYOUT(fmt, stride, h)); // 10-bit linestride, 9-bit height 01194 // DL(BITMAP_LAYOUT_H(stride >> 10, h >> 9)); // msb bits 01195 // DL(BITMAP_SIZE(NEAREST, BORDER, BORDER, w, h)); // 9-bit width, 9-bit height 01196 // DL(BITMAP_SIZE_H(w >> 9, h >> 9)); // msb bits 01197 01198 SetBitmap(addr, fmt, width, height); 01199 01200 // DL(VERTEX2II(x, y, 0, 0)); 01201 DL(VERTEX2F(x * 16, y * 16)); 01202 } 01203 01204 void FT813::ShowBitmapAtAddress(ft_uint32_t addr, ft_int16_t fmt, ft_uint16_t x, ft_uint16_t y, ft_uint16_t width, ft_uint16_t height) 01205 { 01206 DL(BITMAP_SOURCE(addr)); 01207 // ft_int16_t stride = width * 2; 01208 // 1-bits/p L1 01209 // if (fmt == L1) { 01210 // stride = width / 8; 01211 // } 01212 // 2-bits/p L2 01213 // if (fmt == L2) { 01214 // stride = width / 4; 01215 // } 01216 // 4-bits/p L4 01217 // if (fmt == L4) { 01218 // stride = width / 2; 01219 // } 01220 // 8-bits/p L8 RGB332 ARGB2 PALETTED565 PALETTED4444 PALETTED8 01221 // if ((fmt == L8) || 01222 // (fmt == RGB332) || 01223 // (fmt == ARGB2) || 01224 // (fmt == PALETTED565) || 01225 // (fmt == PALETTED4444) || 01226 // (fmt == PALETTED8)) { 01227 // stride = width; 01228 // } 01229 // 16-bits/p ARGB1555 ARGB4 RGB565 01230 // if ((fmt == ARGB1555) || 01231 // (fmt == ARGB4) || 01232 // (fmt == RGB565)) { 01233 // stride = width * 2; 01234 // } 01235 // DL(BITMAP_LAYOUT(fmt, stride, h)); // 10-bit linestride, 9-bit height 01236 // DL(BITMAP_LAYOUT_H(stride >> 10, h >> 9)); // msb bits 01237 // DL(BITMAP_SIZE(NEAREST, BORDER, BORDER, w, h)); // 9-bit width, 9-bit height 01238 // DL(BITMAP_SIZE_H(w >> 9, h >> 9)); // msb bits 01239 01240 SetBitmap(addr, fmt, width, height); 01241 01242 // DL(VERTEX2II(x, y, 0, 0)); 01243 DL(VERTEX2F(x * 16, y * 16)); 01244 } 01245 01246 int FT813::GetImageIndexFromName(char *name) 01247 { 01248 for (int i = 0; i < _bitmapCount; i++) { 01249 if(strstr(_bitmaps[i].name, name) != NULL) { 01250 return i; 01251 } 01252 } 01253 return -1; 01254 } 01255 01256 int FT813::ResetInflateFileBitmap(void) 01257 { 01258 _bitmapAddress = 0; 01259 _bitmapCount = 0; 01260 } 01261 01262 uint32_t FT813::GetRamUsage(void) 01263 { 01264 return _bitmapAddress; 01265 } 01266 01267 uint16_t FT813::GetRamNoOfBitmaps(void) 01268 { 01269 return _bitmapCount; 01270 } 01271 01272 int FT813::LoadInflateFileBitmap(char *name, uint16_t fmt, uint16_t w, uint16_t h) 01273 { 01274 strcpy(_bitmaps[_bitmapCount].name, name); 01275 _bitmaps[_bitmapCount].fmt = fmt; 01276 _bitmaps[_bitmapCount].w = w; 01277 _bitmaps[_bitmapCount].h = h; 01278 _bitmaps[_bitmapCount].addr = _bitmapAddress; 01279 // 1-bits/p L1 01280 if (_bitmaps[_bitmapCount].fmt == L1) { 01281 _bitmaps[_bitmapCount].size = _bitmaps[_bitmapCount].w * _bitmaps[_bitmapCount].h / 8; 01282 } 01283 // 2-bits/p L2 01284 if (_bitmaps[_bitmapCount].fmt == L2) { 01285 _bitmaps[_bitmapCount].size = _bitmaps[_bitmapCount].w * _bitmaps[_bitmapCount].h / 4; 01286 } 01287 // 4-bits/p L4 01288 if (_bitmaps[_bitmapCount].fmt == L4) { 01289 _bitmaps[_bitmapCount].size = _bitmaps[_bitmapCount].w * _bitmaps[_bitmapCount].h / 2; 01290 } 01291 // 8-bits/p L8 RGB332 ARGB2 PALETTED565 PALETTED4444 PALETTED8 01292 if ((_bitmaps[_bitmapCount].fmt == L8) || 01293 (_bitmaps[_bitmapCount].fmt == RGB332) || 01294 (_bitmaps[_bitmapCount].fmt == ARGB2) || 01295 (_bitmaps[_bitmapCount].fmt == PALETTED565) || 01296 (_bitmaps[_bitmapCount].fmt == PALETTED4444) || 01297 (_bitmaps[_bitmapCount].fmt == PALETTED8)) { 01298 _bitmaps[_bitmapCount].size = _bitmaps[_bitmapCount].w * _bitmaps[_bitmapCount].h; 01299 } 01300 // 16-bits/p ARGB1555 ARGB4 RGB565 01301 if ((_bitmaps[_bitmapCount].fmt == ARGB1555) || 01302 (_bitmaps[_bitmapCount].fmt == ARGB4) || 01303 (_bitmaps[_bitmapCount].fmt == RGB565)) { 01304 _bitmaps[_bitmapCount].size = _bitmaps[_bitmapCount].w * _bitmaps[_bitmapCount].h * 2; 01305 } 01306 LoadInflateFile(_bitmaps[_bitmapCount].addr, _bitmaps[_bitmapCount].name); // x 0, y 0, w 32, h 32, size 1024 01307 _bitmapAddress += _bitmaps[_bitmapCount].size; 01308 _bitmapCount++; 01309 } 01310 01311 int FT813::ShowBitmapByName(char *name, uint16_t x, uint16_t y) 01312 { 01313 int index = GetImageIndexFromName(name); 01314 ShowBitmapAtAddress(_bitmaps[index].addr, _bitmaps[index].fmt, x, y, _bitmaps[index].w, _bitmaps[index].h); 01315 } 01316 01317 uint16_t FT813::GetTouchedTag(void) 01318 { 01319 // REG_TOUCH_TAG 01320 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01321 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01322 // REG_TOUCH_TAG_XY 01323 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01324 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01325 uint32_t TrackRegisterVal = Rd32(REG_TRACKER); // check if one of the tracking fields is touched 01326 return TrackRegisterVal & 0xffff; 01327 } 01328 01329 uint16_t FT813::GetTouchedTag(uint8_t point_number) 01330 { 01331 // REG_TOUCH_TAG 01332 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01333 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01334 // REG_TOUCH_TAG_XY 01335 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01336 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01337 // check if one of the tracking fields is touched 01338 uint32_t TrackRegisterVal = 0; 01339 switch (point_number) { 01340 case 0: 01341 TrackRegisterVal = Rd32(REG_TRACKER); // First point 01342 break; 01343 case 1: 01344 TrackRegisterVal = Rd32(REG_TRACKER_1); // Second point 01345 break; 01346 case 2: 01347 TrackRegisterVal = Rd32(REG_TRACKER_2); // Third point 01348 break; 01349 case 3: 01350 TrackRegisterVal = Rd32(REG_TRACKER_3); // Fourth point 01351 break; 01352 case 4: 01353 TrackRegisterVal = Rd32(REG_TRACKER_4); // Fift point 01354 break; 01355 } 01356 return TrackRegisterVal & 0xffff; 01357 } 01358 01359 uint8_t FT813::GetTag(void) 01360 { 01361 // REG_TOUCH_TAG 01362 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01363 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01364 // REG_TOUCH_TAG_XY 01365 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01366 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01367 uint32_t TagRegisterVal = Rd32(REG_TAG); // check if one of the tracking fields is touched 01368 return TagRegisterVal & 0xff; 01369 } 01370 01371 uint16_t FT813::GetTagX(void) 01372 { 01373 // REG_TOUCH_TAG 01374 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01375 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01376 // REG_TOUCH_TAG_XY 01377 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01378 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01379 uint32_t TagRegisterVal = Rd32(REG_TAG_X); // check if one of the tracking fields is touched 01380 return TagRegisterVal & 0x7ff; 01381 } 01382 01383 uint16_t FT813::GetTagY(void) 01384 { 01385 // REG_TOUCH_TAG 01386 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01387 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01388 // REG_TOUCH_TAG_XY 01389 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01390 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01391 uint32_t TagRegisterVal = Rd32(REG_TAG_Y); // check if one of the tracking fields is touched 01392 return TagRegisterVal & 0x7ff; 01393 } 01394 01395 uint8_t FT813::GetTouchTag(void) 01396 { 01397 // REG_TOUCH_TAG 01398 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01399 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01400 // REG_TOUCH_TAG_XY 01401 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01402 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01403 uint32_t TagRegisterVal = Rd32(REG_TOUCH_TAG); // check if one of the tracking fields is touched 01404 return TagRegisterVal & 0xff; 01405 } 01406 01407 uint16_t FT813::GetTouchTagX(void) 01408 { 01409 // REG_TOUCH_TAG 01410 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01411 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01412 // REG_TOUCH_TAG_XY 01413 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01414 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01415 uint32_t TagRegisterVal = Rd32(REG_TOUCH_TAG_XY); // check if one of the tracking fields is touched 01416 return (TagRegisterVal >> 16) & 0xffff; 01417 } 01418 01419 uint16_t FT813::GetTouchTagY(void) 01420 { 01421 // REG_TOUCH_TAG 01422 // If the screen is being touched, the screen coordinates are looked up in the screen's tag buffer, 01423 // delivering a final 8-bit tag value, in REG_TOUCH_TAG. 01424 // REG_TOUCH_TAG_XY 01425 // Because the tag lookup takes a full frame, and touch coordinates change continuously, 01426 // the original (x; y) used for the tag lookup is also available in REG_TOUCH_TAG_XY. 01427 uint32_t TagRegisterVal = Rd32(REG_TOUCH_TAG_XY); // check if one of the tracking fields is touched 01428 return TagRegisterVal & 0xffff; 01429 } 01430 01431 uint16_t FT813::GetTouchConfig(void) 01432 { 01433 // Read 0x0381 01434 // 0000 0011 1000 0001 01435 // bit 15 0: capacitive, 1: resistive 01436 // bit 10 - 4: I2C address of touch screen module: 0x011 1000 0x38 01437 // bit 3: 0: FocalTech, 1: Azoteq 01438 // bit 1 - 0: sampler clocks 0x01 01439 uint32_t TouchConfigVal = Rd32(REG_TOUCH_CONFIG); // check if one of the tracking fields is touched 01440 return TouchConfigVal & 0xffff; 01441 } 01442 01443 void FT813::SetTouchConfig(uint16_t TouchConfigVal = VAL_TOUCH_CONFIG) 01444 { 01445 Wr32(REG_TOUCH_CONFIG, (uint32_t) TouchConfigVal); 01446 } 01447 01448 01449 01450 01451 01452 void FT813::screenShot(void) 01453 { 01454 int bufferSize = 800*480; 01455 char* pbuff = (char*)malloc(bufferSize); 01456 01457 // wr8(REG_SCREENSHOT_EN, 1); 01458 // for (int ly = 0; ly < SCREEN_HEIGHT; ly++) { 01459 // wr16(REG_SCREENSHOT_Y, ly); 01460 // wr8(REG_SCREENSHOT_START, 1); 01461 // 01462 // //Read 64 bit registers to see if it is busy 01463 // while (rd32(REG_SCREENSHOT_BUSY) | rd32(REG_SCREENSHOT_BUSY + 4)); 01464 // 01465 // wr8(REG_SCREENSHOT_READ , 1); 01466 // for (int lx = 0; lx < SCREEN_WIDTH; lx ++) { 01467 // //Read 32 bit pixel value from RAM_SCREENSHOT 01468 // //The pixel format is BGRA: Blue is in lowest address and Alpha 01469 // is in highest address 01470 // screenshot[ly*SCREEN_HEIGHT + lx] = rd32(RAM_SCREENSHOT + lx*4); 01471 // } 01472 // wr8(REG_SCREENSHOT_READ, 0); 01473 // } 01474 // wr8(REG_SCREENSHOT_EN, 0); 01475 01476 free(pbuff); 01477 }
Generated on Tue Jul 19 2022 11:45:29 by
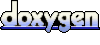