x
Fork of FT810 by
Embed:
(wiki syntax)
Show/hide line numbers
FT_CoPro_Cmds.cpp
00001 /* mbed Library for FTDI FT813 Enbedded Video Engine "EVE" 00002 * based on Original Code Sample from FTDI 00003 * ported to mbed by Peter Drescher, DC2PD 2014 00004 * Released under the MIT License: http://mbed.org/license/mit */ 00005 00006 #include "FT_Platform.h" 00007 00008 ft_void_t FT813::SendCmd(ft_uint32_t cmd) 00009 { 00010 Transfer32(cmd); 00011 } 00012 00013 ft_void_t FT813::SendStr(const ft_char8_t *s) 00014 { 00015 TransferString(s); 00016 } 00017 00018 ft_void_t FT813::StartFunc(ft_uint16_t count) 00019 { 00020 _count = count; 00021 CheckCmdBuffer(_count); 00022 StartCmdTransfer(FT_GPU_WRITE, _count); 00023 } 00024 00025 ft_void_t FT813::EndFunc() 00026 { 00027 EndTransfer(); 00028 Updatecmdfifo(_count); 00029 } 00030 00031 // Start a new display list 00032 // FT81x Series Programmers Guide Section 5.11 00033 ft_void_t FT813::DLstart() 00034 { 00035 // _address = 0; 00036 StartFunc(FT_CMD_SIZE); 00037 SendCmd(CMD_DLSTART); 00038 EndFunc(); 00039 } 00040 00041 // Swap the current display list with the new display list 00042 // FT81x Series Programmers Guide Section 5.12 00043 ft_void_t FT813::Swap() 00044 { 00045 StartFunc(FT_CMD_SIZE); 00046 SendCmd(CMD_SWAP); 00047 EndFunc(); 00048 } 00049 00050 // Set co-processor engine state to default values 00051 // FT81x Series Programmers Guide Section 5.13 00052 ft_void_t FT813::ColdStart() 00053 { 00054 StartFunc(FT_CMD_SIZE); 00055 SendCmd(CMD_COLDSTART); 00056 EndFunc(); 00057 } 00058 00059 // Trigger interrupt INT_CMDFLAG 00060 // FT81x Series Programmers Guide Section 5.14 00061 ft_void_t FT813::Interrupt(ft_uint32_t ms) 00062 { 00063 StartFunc(FT_CMD_SIZE*2); 00064 SendCmd(CMD_INTERRUPT); 00065 SendCmd(ms); 00066 EndFunc(); 00067 } 00068 00069 // Append more commands to current display list 00070 // FT81x Series Programmers Guide Section 5.15 00071 ft_void_t FT813::Append(ft_uint32_t ptr, ft_uint32_t num) 00072 { 00073 StartFunc(FT_CMD_SIZE*3); 00074 SendCmd(CMD_APPEND); 00075 SendCmd(ptr); 00076 SendCmd(num); 00077 EndFunc(); 00078 } 00079 00080 // Read a register value 00081 // FT81x Series Programmers Guide Section 5.16 00082 ft_void_t FT813::RegRead(ft_uint32_t ptr, ft_uint32_t result) 00083 { 00084 StartFunc(FT_CMD_SIZE*3); 00085 SendCmd(CMD_REGREAD); 00086 SendCmd(ptr); 00087 SendCmd(0); 00088 EndFunc(); 00089 } 00090 00091 /* commands to operate on memory: */ 00092 00093 // Write bytes into memory 00094 // FT81x Series Programmers Guide Section 5.17 00095 ft_void_t FT813::MemWrite(ft_uint32_t ptr, ft_uint32_t num) 00096 { 00097 StartFunc(FT_CMD_SIZE*3); 00098 SendCmd(CMD_MEMWRITE); 00099 SendCmd(ptr); 00100 SendCmd(num); 00101 EndFunc(); 00102 } 00103 00104 // Decompress data into memory 00105 // FT81x Series Programmers Guide Section 5.18 00106 ft_void_t FT813::Inflate(ft_uint32_t ptr) 00107 { 00108 StartFunc(FT_CMD_SIZE*2); 00109 SendCmd(CMD_INFLATE); 00110 SendCmd(ptr); 00111 EndFunc(); 00112 } 00113 00114 // Load a JPEG or PNG image 00115 // FT81x Series Programmers Guide Section 5.19 00116 ft_void_t FT813::LoadImage(ft_uint32_t ptr, ft_uint32_t options) 00117 { 00118 StartFunc(FT_CMD_SIZE*3); 00119 SendCmd(CMD_LOADIMAGE); 00120 SendCmd(ptr); 00121 SendCmd(options); 00122 EndFunc(); 00123 } 00124 00125 // Set up a streaming media FIFO in RAM_G 00126 // FT81x Series Programmers Guide Section 5.20 00127 ft_void_t FT813::MediaFifo(ft_uint32_t ptr, ft_uint32_t size) 00128 { 00129 StartFunc(FT_CMD_SIZE*3); 00130 SendCmd(CMD_MEDIAFIFO); 00131 SendCmd(ptr); 00132 SendCmd(size); 00133 EndFunc(); 00134 } 00135 00136 // Video playback 00137 // FT81x Series Programmers Guide Section 5.21 00138 ft_void_t FT813::PlayVideo(ft_uint32_t opts) 00139 { 00140 StartFunc(FT_CMD_SIZE*2); 00141 SendCmd(CMD_PLAYVIDEO); 00142 SendCmd(opts); 00143 EndFunc(); 00144 } 00145 00146 // Initialize video frame decoder 00147 // FT81x Series Programmers Guide Section 5.22 00148 ft_void_t FT813::VideoStart() 00149 { 00150 StartFunc(FT_CMD_SIZE); 00151 SendCmd(CMD_VIDEOSTART); 00152 EndFunc(); 00153 } 00154 00155 // Load the next frame of video 00156 // FT81x Series Programmers Guide Section 5.23 00157 ft_void_t FT813::VideoFrame(ft_uint32_t dst, ft_uint32_t ptr) 00158 { 00159 StartFunc(FT_CMD_SIZE*3); 00160 SendCmd(CMD_VIDEOFRAME); 00161 SendCmd(dst); 00162 SendCmd(ptr); 00163 EndFunc(); 00164 } 00165 00166 // Compute a CRC-32 for memory 00167 // FT81x Series Programmers Guide Section 5.24 00168 ft_void_t FT813::MemCrc(ft_uint32_t ptr, ft_uint32_t num, ft_uint32_t result) 00169 { 00170 StartFunc(FT_CMD_SIZE*4); 00171 SendCmd(CMD_MEMCRC); 00172 SendCmd(ptr); 00173 SendCmd(num); 00174 SendCmd(result); 00175 EndFunc(); 00176 } 00177 00178 // Write zero to a block of memory 00179 // FT81x Series Programmers Guide Section 5.25 00180 ft_void_t FT813::MemZero(ft_uint32_t ptr, ft_uint32_t num) 00181 { 00182 StartFunc(FT_CMD_SIZE*3); 00183 SendCmd(CMD_MEMZERO); 00184 SendCmd(ptr); 00185 SendCmd(num); 00186 EndFunc(); 00187 } 00188 00189 // Fill memory with a byte value 00190 // FT81x Series Programmers Guide Section 5.26 00191 ft_void_t FT813::MemSet(ft_uint32_t ptr, ft_uint32_t value, ft_uint32_t num) 00192 { 00193 StartFunc(FT_CMD_SIZE*4); 00194 SendCmd(CMD_MEMSET); 00195 SendCmd(ptr); 00196 SendCmd(value); 00197 SendCmd(num); 00198 EndFunc(); 00199 } 00200 00201 // Cmd_Memcpy - background copy a block of data 00202 // FT81x Series Programmers Guide Section 5.27 00203 ft_void_t FT813::Memcpy(ft_uint32_t dest, ft_uint32_t src, ft_uint32_t num) 00204 { 00205 StartFunc(FT_CMD_SIZE*4); 00206 SendCmd(CMD_MEMCPY); 00207 SendCmd(dest); 00208 SendCmd(src); 00209 SendCmd(num); 00210 EndFunc(); 00211 } 00212 00213 /* commands to draw graphics objects: */ 00214 // ******************** Screen Object Creation CoProcessor Command Functions ****************************** 00215 00216 // Draw a button 00217 // FT81x Series Programmers Guide Section 5.28 00218 ft_void_t FT813::Button(ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_int16_t font, ft_uint16_t options, const ft_char8_t* s) 00219 { 00220 StartFunc(FT_CMD_SIZE*4 + strlen(s) + 1); 00221 SendCmd(CMD_BUTTON); 00222 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00223 SendCmd((((ft_uint32_t)h<<16)|w)); 00224 SendCmd((((ft_uint32_t)options<<16)|font)); // patch from Ivano Pelicella to draw flat buttons 00225 SendStr(s); 00226 EndFunc(); 00227 } 00228 00229 // Draw an analog clock 00230 // FT81x Series Programmers Guide Section 5.29 00231 ft_void_t FT813::Clock(ft_int16_t x, ft_int16_t y, ft_int16_t r, ft_uint16_t options, ft_uint16_t h, ft_uint16_t m, ft_uint16_t s, ft_uint16_t ms) 00232 { 00233 StartFunc(FT_CMD_SIZE*5); 00234 SendCmd(CMD_CLOCK); 00235 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00236 SendCmd((((ft_uint32_t)options<<16)|r)); 00237 SendCmd((((ft_uint32_t)m<<16)|h)); 00238 SendCmd((((ft_uint32_t)ms<<16)|s)); 00239 EndFunc(); 00240 } 00241 00242 // Set the foreground color 00243 // FT81x Series Programmers Guide Section 5.30 00244 ft_void_t FT813::FgColor(ft_uint32_t c) 00245 { 00246 StartFunc(FT_CMD_SIZE*2); 00247 SendCmd(CMD_FGCOLOR); 00248 SendCmd(c); 00249 EndFunc(); 00250 } 00251 00252 // Set the background color 00253 // FT81x Series Programmers Guide Section 5.31 00254 ft_void_t FT813::BgColor(ft_uint32_t c) 00255 { 00256 StartFunc(FT_CMD_SIZE*2); 00257 SendCmd(CMD_BGCOLOR); 00258 SendCmd(c); 00259 EndFunc(); 00260 } 00261 00262 // Set the 3D button highlight color - Set Highlight Gradient Color 00263 // FT81x Series Programmers Guide Section 5.32 00264 ft_void_t FT813::GradColor(ft_uint32_t c) 00265 { 00266 StartFunc(FT_CMD_SIZE*2); 00267 SendCmd(CMD_GRADCOLOR); 00268 SendCmd(c); 00269 EndFunc(); 00270 } 00271 00272 // Draw a gauge 00273 // FT81x Series Programmers Guide Section 5.33 00274 /* Error handling for val is not done, so better to always use range of 65535 in order that needle is drawn within display region */ 00275 ft_void_t FT813::Gauge(ft_int16_t x, ft_int16_t y, ft_int16_t r, ft_uint16_t options, ft_uint16_t major, ft_uint16_t minor, ft_uint16_t val, ft_uint16_t range) 00276 { 00277 StartFunc(FT_CMD_SIZE*5); 00278 SendCmd(CMD_GAUGE); 00279 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00280 SendCmd((((ft_uint32_t)options<<16)|r)); 00281 SendCmd((((ft_uint32_t)minor<<16)|major)); 00282 SendCmd((((ft_uint32_t)range<<16)|val)); 00283 EndFunc(); 00284 } 00285 00286 // Draw a smooth color gradient 00287 // FT81x Series Programmers Guide Section 5.34 00288 ft_void_t FT813::Gradient(ft_int16_t x0, ft_int16_t y0, ft_uint32_t rgb0, ft_int16_t x1, ft_int16_t y1, ft_uint32_t rgb1) 00289 { 00290 StartFunc(FT_CMD_SIZE*5); 00291 SendCmd(CMD_GRADIENT); 00292 SendCmd((((ft_uint32_t)y0<<16)|(x0 & 0xffff))); 00293 SendCmd(rgb0); 00294 SendCmd((((ft_uint32_t)y1<<16)|(x1 & 0xffff))); 00295 SendCmd(rgb1); 00296 EndFunc(); 00297 } 00298 00299 // Draw a row of keys 00300 // FT81x Series Programmers Guide Section 5.35 00301 ft_void_t FT813::Keys(ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_int16_t font, ft_uint16_t options, const ft_char8_t* s) 00302 { 00303 StartFunc(FT_CMD_SIZE*4 + strlen(s) + 1); 00304 SendCmd(CMD_KEYS); 00305 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00306 SendCmd((((ft_uint32_t)h<<16)|w)); 00307 SendCmd((((ft_uint32_t)options<<16)|font)); 00308 SendStr(s); 00309 EndFunc(); 00310 } 00311 00312 // Draw a progress bar 00313 // FT81x Series Programmers Guide Section 5.36 00314 ft_void_t FT813::Progress(ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_uint16_t options, ft_uint16_t val, ft_uint16_t range) 00315 { 00316 StartFunc(FT_CMD_SIZE*5); 00317 SendCmd(CMD_PROGRESS); 00318 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00319 SendCmd((((ft_uint32_t)h<<16)|w)); 00320 SendCmd((((ft_uint32_t)val<<16)|options)); 00321 SendCmd(range); 00322 EndFunc(); 00323 } 00324 00325 // Draw a scroll bar 00326 // FT81x Series Programmers Guide Section 5.37 00327 ft_void_t FT813::Scrollbar(ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_uint16_t options, ft_uint16_t val, ft_uint16_t size, ft_uint16_t range) 00328 { 00329 StartFunc(FT_CMD_SIZE*5); 00330 SendCmd(CMD_SCROLLBAR); 00331 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00332 SendCmd((((ft_uint32_t)h<<16)|w)); 00333 SendCmd((((ft_uint32_t)val<<16)|options)); 00334 SendCmd((((ft_uint32_t)range<<16)|size)); 00335 EndFunc(); 00336 } 00337 00338 // Draw a slider 00339 // FT81x Series Programmers Guide Section 5.38 00340 ft_void_t FT813::Slider(ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_uint16_t options, ft_uint16_t val, ft_uint16_t range) 00341 { 00342 StartFunc(FT_CMD_SIZE*5); 00343 SendCmd(CMD_SLIDER); 00344 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00345 SendCmd((((ft_uint32_t)h<<16)|w)); 00346 SendCmd((((ft_uint32_t)val<<16)|options)); 00347 SendCmd(range); 00348 EndFunc(); 00349 } 00350 00351 // Draw a rotary dial control 00352 // FT81x Series Programmers Guide Section 5.39 00353 // This is much like a Gauge except for the helpful range parameter. For some reason, all dials are 65535 around. 00354 ft_void_t FT813::Dial(ft_int16_t x, ft_int16_t y, ft_int16_t r, ft_uint16_t options, ft_uint16_t val) 00355 { 00356 StartFunc(FT_CMD_SIZE*4); 00357 SendCmd(CMD_DIAL); 00358 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00359 SendCmd((((ft_uint32_t)options<<16)|r)); 00360 SendCmd(val); 00361 EndFunc(); 00362 } 00363 00364 // Draw a toggle switch 00365 // FT81x Series Programmers Guide Section 5.40 00366 ft_void_t FT813::Toggle(ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t font, ft_uint16_t options, ft_uint16_t state, const ft_char8_t* s) 00367 { 00368 StartFunc(FT_CMD_SIZE*4 + strlen(s) + 1); 00369 SendCmd(CMD_TOGGLE); 00370 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00371 SendCmd((((ft_uint32_t)font<<16)|w)); 00372 SendCmd((((ft_uint32_t)state<<16)|options)); 00373 SendStr(s); 00374 EndFunc(); 00375 } 00376 00377 // Draw Text 00378 // FT81x Series Programmers Guide Section 5.41 00379 ft_void_t FT813::Text(ft_int16_t x, ft_int16_t y, ft_int16_t font, ft_uint16_t options, const ft_char8_t* s) 00380 { 00381 StartFunc(FT_CMD_SIZE*3 + strlen(s) + 1); 00382 SendCmd(CMD_TEXT); 00383 //Copro_SendCmd((((ft_uint32_t)y<<16)|(ft_uint32_t)x)); 00384 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00385 SendCmd((((ft_uint32_t)options<<16)|(ft_uint32_t)font)); 00386 SendStr(s); 00387 EndFunc(); 00388 } 00389 00390 // Set the base for number output 00391 // FT81x Series Programmers Guide Section 5.42 00392 ft_void_t FT813::SetBase(ft_uint32_t base) 00393 { 00394 StartFunc(FT_CMD_SIZE*2); 00395 SendCmd(CMD_SETBASE); 00396 SendCmd(base); 00397 EndFunc(); 00398 } 00399 00400 // Draw number 00401 // FT81x Series Programmers Guide Section 5.43 00402 ft_void_t FT813::Number(ft_int16_t x, ft_int16_t y, ft_int16_t font, ft_uint16_t options, ft_int32_t n) 00403 { 00404 StartFunc(FT_CMD_SIZE*4); 00405 SendCmd(CMD_NUMBER); 00406 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00407 SendCmd((((ft_uint32_t)options<<16)|font)); 00408 SendCmd(n); 00409 EndFunc(); 00410 } 00411 00412 // Set the current matrix to the identity matrix 00413 // FT81x Series Programmers Guide Section 5.44 00414 ft_void_t FT813::LoadIdentity() 00415 { 00416 StartFunc(FT_CMD_SIZE); 00417 SendCmd(CMD_LOADIDENTITY); 00418 EndFunc(); 00419 } 00420 00421 // Write the current matrix to the display list 00422 // FT81x Series Programmers Guide Section 5.45 00423 ft_void_t FT813::SetMatrix() 00424 { 00425 StartFunc(FT_CMD_SIZE); 00426 SendCmd(CMD_SETMATRIX); 00427 EndFunc(); 00428 } 00429 00430 // Retrieves the current matrix coefficients 00431 // FT81x Series Programmers Guide Section 5.46 00432 ft_void_t FT813::GetMatrix(ft_int32_t a, ft_int32_t b, ft_int32_t c, ft_int32_t d, ft_int32_t e, ft_int32_t f) 00433 { 00434 StartFunc(FT_CMD_SIZE*7); 00435 SendCmd(CMD_GETMATRIX); 00436 SendCmd(a); 00437 SendCmd(b); 00438 SendCmd(c); 00439 SendCmd(d); 00440 SendCmd(e); 00441 SendCmd(f); 00442 EndFunc(); 00443 } 00444 00445 // Get the end memory address of data inflated by CMD_INFLATE - Get the last used address from CoPro operation 00446 // FT81x Series Programmers Guide Section 5.47 00447 ft_void_t FT813::GetPtr(ft_uint32_t result) 00448 { 00449 StartFunc(FT_CMD_SIZE*2); 00450 SendCmd(CMD_GETPTR); 00451 SendCmd(result); 00452 EndFunc(); 00453 } 00454 00455 // Get the image properties decompressed by CMD_LOADIMAGE 00456 // FT81x Series Programmers Guide Section 5.48 00457 // Jack 00458 ft_void_t FT813::GetProps(ft_uint32_t ptr, ft_uint32_t w, ft_uint32_t h) 00459 { 00460 StartFunc(FT_CMD_SIZE*4); 00461 SendCmd(CMD_GETPROPS); 00462 SendCmd(ptr); 00463 SendCmd(w); 00464 SendCmd(h); 00465 EndFunc(); 00466 } 00467 00468 // Apply a scale to the current matrix 00469 // FT81x Series Programmers Guide Section 5.49 00470 ft_void_t FT813::Scale(ft_int32_t sx, ft_int32_t sy) 00471 { 00472 StartFunc(FT_CMD_SIZE*3); 00473 SendCmd(CMD_SCALE); 00474 SendCmd(sx); 00475 SendCmd(sy); 00476 EndFunc(); 00477 } 00478 00479 // Apply a rotation to the current matrix 00480 // FT81x Series Programmers Guide Section 5.50 00481 ft_void_t FT813::Rotate(ft_int32_t a) 00482 { 00483 StartFunc(FT_CMD_SIZE*2); 00484 SendCmd(CMD_ROTATE); 00485 SendCmd(a); 00486 EndFunc(); 00487 } 00488 00489 // Apply a translation to the current matrix 00490 // FT81x Series Programmers Guide Section 5.51 00491 ft_void_t FT813::Translate(ft_int32_t tx, ft_int32_t ty) 00492 { 00493 StartFunc(FT_CMD_SIZE*3); 00494 SendCmd(CMD_TRANSLATE); 00495 SendCmd(tx); 00496 SendCmd(ty); 00497 EndFunc(); 00498 } 00499 00500 // Execute the touch screen calibration routine 00501 // FT81x Series Programmers Guide Section 5.52 00502 // * This business about "result" in the manual really seems to be simply leftover cruft of no purpose - send zero 00503 ft_void_t FT813::Calibrate(ft_uint32_t result) 00504 { 00505 StartFunc(FT_CMD_SIZE*2); 00506 SendCmd(CMD_CALIBRATE); 00507 SendCmd(result); 00508 EndFunc(); 00509 WaitCmdfifo_empty(); 00510 } 00511 00512 // Rotate the screen 00513 // FT81x Series Programmers Guide Section 5.53 00514 ft_void_t FT813::SetRotate(ft_uint32_t r) 00515 { 00516 StartFunc(FT_CMD_SIZE*2); 00517 SendCmd(CMD_SETROTATE); 00518 SendCmd(r); 00519 EndFunc(); 00520 } 00521 00522 // Start an animated spinner 00523 // FT81x Series Programmers Guide Section 5.54 00524 ft_void_t FT813::Spinner(ft_int16_t x, ft_int16_t y, ft_uint16_t style, ft_uint16_t scale) 00525 { 00526 StartFunc(FT_CMD_SIZE*3); 00527 SendCmd(CMD_SPINNER); 00528 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00529 SendCmd((((ft_uint32_t)scale<<16)|style)); 00530 EndFunc(); 00531 } 00532 00533 // Start an animated screensaver 00534 // FT81x Series Programmers Guide Section 5.55 00535 ft_void_t FT813::ScreenSaver() 00536 { 00537 StartFunc(FT_CMD_SIZE); 00538 SendCmd(CMD_SCREENSAVER); 00539 EndFunc(); 00540 } 00541 00542 // Start a continuous sketch update 00543 // FT81x Series Programmers Guide Section 5.56 00544 ft_void_t FT813::Sketch(ft_int16_t x, ft_int16_t y, ft_uint16_t w, ft_uint16_t h, ft_uint32_t ptr, ft_uint16_t format) 00545 { 00546 StartFunc(FT_CMD_SIZE*5); 00547 SendCmd(CMD_SKETCH); 00548 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00549 SendCmd((((ft_uint32_t)h<<16)|w)); 00550 SendCmd(ptr); 00551 SendCmd(format); 00552 EndFunc(); 00553 } 00554 00555 // Stop any of spinner, screensaver or sketch 00556 // FT81x Series Programmers Guide Section 5.57 00557 ft_void_t FT813::Stop() 00558 { 00559 StartFunc(FT_CMD_SIZE); 00560 SendCmd(CMD_STOP); 00561 EndFunc(); 00562 } 00563 00564 // Set up a custom font 00565 // FT81x Series Programmers Guide Section 5.58 00566 ft_void_t FT813::SetFont(ft_uint32_t font, ft_uint32_t ptr) 00567 { 00568 StartFunc(FT_CMD_SIZE*3); 00569 SendCmd(CMD_SETFONT); 00570 SendCmd(font); 00571 SendCmd(ptr); 00572 EndFunc(); 00573 } 00574 00575 // Set up a custom font 00576 // FT81x Series Programmers Guide Section 5.59 00577 ft_void_t FT813::SetFont2(ft_uint32_t font, ft_uint32_t ptr, ft_uint32_t firstchar) 00578 { 00579 StartFunc(FT_CMD_SIZE*4); 00580 SendCmd(CMD_SETFONT2); 00581 SendCmd(font); 00582 SendCmd(ptr); 00583 SendCmd(firstchar); 00584 EndFunc(); 00585 } 00586 00587 // Set the scratch bitmap for widget use 00588 // FT81x Series Programmers Guide Section 5.60 00589 ft_void_t FT813::SetScratch(ft_uint32_t handle) 00590 { 00591 StartFunc(FT_CMD_SIZE*2); 00592 SendCmd(CMD_SETSCRATCH); 00593 SendCmd(handle); 00594 EndFunc(); 00595 } 00596 00597 // Load a ROM font into bitmap handle 00598 // FT81x Series Programmers Guide Section 5.61 00599 ft_void_t FT813::RomFont(ft_uint32_t rom_slot, ft_uint32_t font) 00600 { 00601 StartFunc(FT_CMD_SIZE*3); 00602 SendCmd(CMD_ROMFONT); 00603 SendCmd(rom_slot); 00604 SendCmd(font); 00605 EndFunc(); 00606 } 00607 00608 // Track touches for a graphics object - Make Track (for a slider) 00609 // FT81x Series Programmers Guide Section 5.62 00610 // tag refers to the tag # previously assigned to the object that this track is tracking. 00611 ft_void_t FT813::Track(ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h, ft_int16_t tag) 00612 { 00613 StartFunc(FT_CMD_SIZE*4); 00614 SendCmd(CMD_TRACK); 00615 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00616 SendCmd((((ft_uint32_t)h<<16)|w)); 00617 SendCmd(tag); 00618 EndFunc(); 00619 } 00620 00621 // Take a snapshot of the current screen 00622 // FT81x Series Programmers Guide Section 5.63 00623 ft_void_t FT813::Snapshot(ft_uint32_t ptr) 00624 { 00625 StartFunc(FT_CMD_SIZE*2); 00626 SendCmd(CMD_SNAPSHOT); 00627 SendCmd(ptr); 00628 EndFunc(); 00629 } 00630 00631 // Take a snapshot of part of the current screen 00632 // FT81x Series Programmers Guide Section 5.64 00633 ft_void_t FT813::Snapshot2(ft_uint32_t fmt, ft_uint32_t ptr, ft_int16_t x, ft_int16_t y, ft_int16_t w, ft_int16_t h) 00634 { 00635 StartFunc(FT_CMD_SIZE*5); 00636 SendCmd(CMD_SNAPSHOT2); 00637 SendCmd(fmt); 00638 SendCmd(ptr); 00639 SendCmd((((ft_uint32_t)y<<16)|(x & 0xffff))); 00640 SendCmd((((ft_uint32_t)h<<16)|(w & 0xffff))); 00641 EndFunc(); 00642 } 00643 00644 // Set up display list for bitmap - Cmd_SetBitmap - generate DL commands for bitmap parms 00645 // FT81x Series Programmers Guide Section 5.65 00646 ft_void_t FT813::SetBitmap(ft_int32_t addr, ft_int16_t fmt, ft_uint16_t width, ft_uint16_t height) 00647 { 00648 StartFunc(FT_CMD_SIZE*4); 00649 SendCmd(CMD_SETBITMAP); 00650 SendCmd(addr); 00651 SendCmd((((ft_uint32_t)width<<16)|(fmt & 0xffff))); 00652 SendCmd((((ft_uint32_t)0<<16)|(height & 0xffff))); 00653 EndFunc(); 00654 } 00655 00656 // Play FTDI logo animation 00657 // FT81x Series Programmers Guide Section 5.66 00658 ft_void_t FT813::Logo() 00659 { 00660 StartFunc(FT_CMD_SIZE); 00661 SendCmd(CMD_LOGO); 00662 EndFunc(); 00663 } 00664 00665 // ******************** Miscellaneous Operation CoProcessor Command Functions ****************************** 00666 00667 // ? 00668 // FT81x Series Programmers Guide Section ? 00669 ft_void_t FT813::TouchTransform(ft_int32_t x0, ft_int32_t y0, ft_int32_t x1, ft_int32_t y1, ft_int32_t x2, ft_int32_t y2, ft_int32_t tx0, ft_int32_t ty0, ft_int32_t tx1, ft_int32_t ty1, ft_int32_t tx2, ft_int32_t ty2, ft_uint16_t result) 00670 { 00671 StartFunc(FT_CMD_SIZE*6*2+FT_CMD_SIZE*2); 00672 SendCmd(CMD_TOUCH_TRANSFORM); 00673 SendCmd(x0); 00674 SendCmd(y0); 00675 SendCmd(x1); 00676 SendCmd(y1); 00677 SendCmd(x2); 00678 SendCmd(y2); 00679 SendCmd(tx0); 00680 SendCmd(ty0); 00681 SendCmd(tx1); 00682 SendCmd(ty1); 00683 SendCmd(tx2); 00684 SendCmd(ty2); 00685 SendCmd(result); 00686 EndFunc(); 00687 } 00688 00689 // ? 00690 // FT81x Series Programmers Guide Section ? 00691 ft_void_t FT813::BitmapTransform(ft_int32_t x0, ft_int32_t y0, ft_int32_t x1, ft_int32_t y1, ft_int32_t x2, ft_int32_t y2, ft_int32_t tx0, ft_int32_t ty0, ft_int32_t tx1, ft_int32_t ty1, ft_int32_t tx2, ft_int32_t ty2, ft_uint16_t result) 00692 { 00693 StartFunc(FT_CMD_SIZE*6*2+FT_CMD_SIZE*2); 00694 SendCmd(CMD_BITMAP_TRANSFORM); 00695 SendCmd(x0); 00696 SendCmd(y0); 00697 SendCmd(x1); 00698 SendCmd(y1); 00699 SendCmd(x2); 00700 SendCmd(y2); 00701 SendCmd(tx0); 00702 SendCmd(ty0); 00703 SendCmd(tx1); 00704 SendCmd(ty1); 00705 SendCmd(tx2); 00706 SendCmd(ty2); 00707 SendCmd(result); 00708 EndFunc(); 00709 } 00710 00711 ft_void_t FT813::DL(ft_uint32_t cmd) 00712 { 00713 WrCmd32(cmd); 00714 /* Increment the command index */ 00715 CmdBuffer_Index += FT_CMD_SIZE; 00716 } 00717 00718 ft_void_t FT813::WrDlCmd_Buffer(ft_uint32_t cmd) 00719 { 00720 Wr32((RAM_DL+DlBuffer_Index), cmd); 00721 /* Increment the command index */ 00722 DlBuffer_Index += FT_CMD_SIZE; 00723 } 00724 00725 ft_void_t FT813::Flush_DL_Buffer() 00726 { 00727 DlBuffer_Index = 0; 00728 } 00729 00730 ft_void_t FT813::Flush_Co_Buffer() 00731 { 00732 CmdBuffer_Index = 0; 00733 } 00734 00735 /* API to check the status of previous DLSWAP and perform DLSWAP of new DL */ 00736 /* Check for the status of previous DLSWAP and if still not done wait for few ms and check again */ 00737 ft_void_t FT813::DLSwap(ft_uint8_t DL_Swap_Type) 00738 { 00739 ft_uint8_t Swap_Type = DLSWAP_FRAME, Swap_Done = DLSWAP_FRAME; 00740 00741 if(DL_Swap_Type == DLSWAP_LINE) { 00742 Swap_Type = DLSWAP_LINE; 00743 } 00744 00745 /* Perform a new DL swap */ 00746 Wr8(REG_DLSWAP, Swap_Type); 00747 00748 /* Wait till the swap is done */ 00749 while(Swap_Done) { 00750 Swap_Done = Rd8(REG_DLSWAP); 00751 00752 if(DLSWAP_DONE != Swap_Done) { 00753 Sleep(10);//wait for 10ms 00754 } 00755 } 00756 } 00757 00758 /* Nothing beyond this */
Generated on Tue Jul 19 2022 11:45:29 by
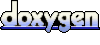