Infrared remote library for Arduino: send and receive infrared signals with multiple protocols Port from Arduino-IRremote https://github.com/z3t0/Arduino-IRremote
ir_Template.cpp
00001 /* 00002 Assuming the protocol we are adding is for the (imaginary) manufacturer: Shuzu 00003 00004 Our fantasy protocol is a standard protocol, so we can use this standard 00005 template without too much work. Some protocols are quite unique and will require 00006 considerably more work in this file! It is way beyond the scope of this text to 00007 explain how to reverse engineer "unusual" IR protocols. But, unless you own an 00008 oscilloscope, the starting point is probably to use the rawDump.ino sketch and 00009 try to spot the pattern! 00010 00011 Before you start, make sure the IR library is working OK: 00012 # Open up the Arduino IDE 00013 # Load up the rawDump.ino example sketch 00014 # Run it 00015 00016 Now we can start to add our new protocol... 00017 00018 1. Copy this file to : ir_Shuzu.cpp 00019 00020 2. Replace all occurrences of "Shuzu" with the name of your protocol. 00021 00022 3. Tweak the #defines to suit your protocol. 00023 00024 4. If you're lucky, tweaking the #defines will make the default send() function 00025 work. 00026 00027 5. Again, if you're lucky, tweaking the #defines will have made the default 00028 decode() function work. 00029 00030 You have written the code to support your new protocol! 00031 00032 Now you must do a few things to add it to the IRremote system: 00033 00034 1. Open IRremote.h and make the following changes: 00035 REMEMEBER to change occurences of "SHUZU" with the name of your protocol 00036 00037 A. At the top, in the section "Supported Protocols", add: 00038 #define DECODE_SHUZU 1 00039 #define SEND_SHUZU 1 00040 00041 B. In the section "enumerated list of all supported formats", add: 00042 SHUZU, 00043 to the end of the list (notice there is a comma after the protocol name) 00044 00045 C. Further down in "Main class for receiving IR", add: 00046 //...................................................................... 00047 #if DECODE_SHUZU 00048 bool decodeShuzu (decode_results *results) ; 00049 #endif 00050 00051 D. Further down in "Main class for sending IR", add: 00052 //...................................................................... 00053 #if SEND_SHUZU 00054 void sendShuzu (unsigned long data, int nbits) ; 00055 #endif 00056 00057 E. Save your changes and close the file 00058 00059 2. Now open irRecv.cpp and make the following change: 00060 00061 A. In the function IRrecv::decode(), add: 00062 #ifdef DECODE_NEC 00063 DBG_PRINTLN("Attempting Shuzu decode"); 00064 if (decodeShuzu(results)) return true ; 00065 #endif 00066 00067 B. Save your changes and close the file 00068 00069 You will probably want to add your new protocol to the example sketch 00070 00071 3. Open MyDocuments\Arduino\libraries\IRremote\examples\IRrecvDumpV2.ino 00072 00073 A. In the encoding() function, add: 00074 case SHUZU: printf("SHUZU"); break ; 00075 00076 Now open the Arduino IDE, load up the rawDump.ino sketch, and run it. 00077 Hopefully it will compile and upload. 00078 If it doesn't, you've done something wrong. Check your work. 00079 If you can't get it to work - seek help from somewhere. 00080 00081 If you get this far, I will assume you have successfully added your new protocol 00082 There is one last thing to do. 00083 00084 1. Delete this giant instructional comment. 00085 00086 2. Send a copy of your work to us so we can include it in the library and 00087 others may benefit from your hard work and maybe even write a song about how 00088 great you are for helping them! :) 00089 00090 Regards, 00091 BlueChip 00092 */ 00093 00094 #include "IRremote.h" 00095 #include "IRremoteInt.h" 00096 00097 //============================================================================== 00098 // 00099 // 00100 // S H U Z U 00101 // 00102 // 00103 //============================================================================== 00104 00105 #define BITS 32 // The number of bits in the command 00106 00107 #define HDR_MARK 1000 // The length of the Header:Mark 00108 #define HDR_SPACE 2000 // The lenght of the Header:Space 00109 00110 #define BIT_MARK 3000 // The length of a Bit:Mark 00111 #define ONE_SPACE 4000 // The length of a Bit:Space for 1's 00112 #define ZERO_SPACE 5000 // The length of a Bit:Space for 0's 00113 00114 #define OTHER 1234 // Other things you may need to define 00115 00116 //+============================================================================= 00117 // 00118 #if SEND_SHUZU 00119 void IRsend::sendShuzu (unsigned long data, int nbits) 00120 { 00121 // Set IR carrier frequency 00122 enableIROut(38); 00123 00124 // Header 00125 mark (HDR_MARK); 00126 space(HDR_SPACE); 00127 00128 // Data 00129 for (unsigned long mask = 1UL << (nbits - 1); mask; mask >>= 1) { 00130 if (data & mask) { 00131 mark (BIT_MARK); 00132 space(ONE_SPACE); 00133 } else { 00134 mark (BIT_MARK); 00135 space(ZERO_SPACE); 00136 } 00137 } 00138 00139 // Footer 00140 mark(BIT_MARK); 00141 space(0); // Always end with the LED off 00142 } 00143 #endif 00144 00145 //+============================================================================= 00146 // 00147 #if DECODE_SHUZU 00148 bool IRrecv::decodeShuzu (decode_results *results) 00149 { 00150 unsigned long data = 0; // Somewhere to build our code 00151 int offset = 1; // Skip the Gap reading 00152 00153 // Check we have the right amount of data 00154 if (irparams.rawlen != 1 + 2 + (2 * BITS) + 1) return false ; 00155 00156 // Check initial Mark+Space match 00157 if (!MATCH_MARK (results->rawbuf[offset++], HDR_MARK )) return false ; 00158 if (!MATCH_SPACE(results->rawbuf[offset++], HDR_SPACE)) return false ; 00159 00160 // Read the bits in 00161 for (int i = 0; i < SHUZU_BITS; i++) { 00162 // Each bit looks like: MARK + SPACE_1 -> 1 00163 // or : MARK + SPACE_0 -> 0 00164 if (!MATCH_MARK(results->rawbuf[offset++], BIT_MARK)) return false ; 00165 00166 // IR data is big-endian, so we shuffle it in from the right: 00167 if (MATCH_SPACE(results->rawbuf[offset], ONE_SPACE)) data = (data << 1) | 1 ; 00168 else if (MATCH_SPACE(results->rawbuf[offset], ZERO_SPACE)) data = (data << 1) | 0 ; 00169 else return false ; 00170 offset++; 00171 } 00172 00173 // Success 00174 results->bits = BITS; 00175 results->value = data; 00176 results->decode_type = SHUZU; 00177 return true; 00178 } 00179 #endif
Generated on Fri Jul 15 2022 02:03:08 by
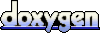