Infrared remote library for Arduino: send and receive infrared signals with multiple protocols Port from Arduino-IRremote https://github.com/z3t0/Arduino-IRremote
ir_Sharp.cpp
00001 #include "IRremote.h" 00002 #include "IRremoteInt.h" 00003 00004 //============================================================================== 00005 // SSSS H H AAA RRRR PPPP 00006 // S H H A A R R P P 00007 // SSS HHHHH AAAAA RRRR PPPP 00008 // S H H A A R R P 00009 // SSSS H H A A R R P 00010 //============================================================================== 00011 00012 // Sharp and DISH support by Todd Treece: http://unionbridge.org/design/ircommand 00013 // 00014 // The send function has the necessary repeat built in because of the need to 00015 // invert the signal. 00016 // 00017 // Sharp protocol documentation: 00018 // http://www.sbprojects.com/knowledge/ir/sharp.htm 00019 // 00020 // Here is the LIRC file I found that seems to match the remote codes from the 00021 // oscilloscope: 00022 // Sharp LCD TV: 00023 // http://lirc.sourceforge.net/remotes/sharp/GA538WJSA 00024 00025 #define SHARP_BITS 15 00026 #define SHARP_BIT_MARK 245 00027 #define SHARP_ONE_SPACE 1805 00028 #define SHARP_ZERO_SPACE 795 00029 #define SHARP_GAP 600000 00030 #define SHARP_RPT_SPACE 3000 00031 00032 #define SHARP_TOGGLE_MASK 0x3FF 00033 00034 //+============================================================================= 00035 #if SEND_SHARP 00036 void IRsend::sendSharpRaw (unsigned long data, int nbits) 00037 { 00038 enableIROut(38); 00039 00040 // Sending codes in bursts of 3 (normal, inverted, normal) makes transmission 00041 // much more reliable. That's the exact behaviour of CD-S6470 remote control. 00042 for (int n = 0; n < 3; n++) { 00043 for (unsigned long mask = 1UL << (nbits - 1); mask; mask >>= 1) { 00044 if (data & mask) { 00045 mark(SHARP_BIT_MARK); 00046 space(SHARP_ONE_SPACE); 00047 } else { 00048 mark(SHARP_BIT_MARK); 00049 space(SHARP_ZERO_SPACE); 00050 } 00051 } 00052 00053 mark(SHARP_BIT_MARK); 00054 space(SHARP_ZERO_SPACE); 00055 wait_ms(40); 00056 00057 data = data ^ SHARP_TOGGLE_MASK; 00058 } 00059 } 00060 #endif 00061 00062 //+============================================================================= 00063 // Sharp send compatible with data obtained through decodeSharp() 00064 // ^^^^^^^^^^^^^ FUNCTION MISSING! 00065 // 00066 #if SEND_SHARP 00067 void IRsend::sendSharp (unsigned int address, unsigned int command) 00068 { 00069 sendSharpRaw((address << 10) | (command << 2) | 2, SHARP_BITS); 00070 } 00071 #endif
Generated on Fri Jul 15 2022 02:03:08 by
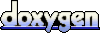