Infrared remote library for Arduino: send and receive infrared signals with multiple protocols Port from Arduino-IRremote https://github.com/z3t0/Arduino-IRremote
ir_Mitsubishi.cpp
00001 #include "IRremote.h" 00002 #include "IRremoteInt.h" 00003 00004 //============================================================================== 00005 // MMMMM IIIII TTTTT SSSS U U BBBB IIIII SSSS H H IIIII 00006 // M M M I T S U U B B I S H H I 00007 // M M M I T SSS U U BBBB I SSS HHHHH I 00008 // M M I T S U U B B I S H H I 00009 // M M IIIII T SSSS UUU BBBBB IIIII SSSS H H IIIII 00010 //============================================================================== 00011 00012 // Looks like Sony except for timings, 48 chars of data and time/space different 00013 00014 #define MITSUBISHI_BITS 16 00015 00016 // Mitsubishi RM 75501 00017 // 14200 7 41 7 42 7 42 7 17 7 17 7 18 7 41 7 18 7 17 7 17 7 18 7 41 8 17 7 17 7 18 7 17 7 00018 // #define MITSUBISHI_HDR_MARK 250 // seen range 3500 00019 #define MITSUBISHI_HDR_SPACE 350 // 7*50+100 00020 #define MITSUBISHI_ONE_MARK 1950 // 41*50-100 00021 #define MITSUBISHI_ZERO_MARK 750 // 17*50-100 00022 // #define MITSUBISHI_DOUBLE_SPACE_USECS 800 // usually ssee 713 - not using ticks as get number wrapround 00023 // #define MITSUBISHI_RPT_LENGTH 45000 00024 00025 //+============================================================================= 00026 #if DECODE_MITSUBISHI 00027 bool IRrecv::decodeMitsubishi (decode_results *results) 00028 { 00029 //printf("?!? decoding Mitsubishi:%d want %d\n", irparams.rawlen, 2 * MITSUBISHI_BITS + 2); 00030 long data = 0; 00031 if (irparams.rawlen < 2 * MITSUBISHI_BITS + 2) return false ; 00032 int offset = 0; // Skip first space 00033 // Initial space 00034 00035 #if 0 00036 // Put this back in for debugging - note can't use #DEBUG as if Debug on we don't see the repeat cos of the delay 00037 printf("IR Gap: %d\n", results->rawbuf[offset]); 00038 printf("test against: %d\n", results->rawbuf[offset]); 00039 #endif 00040 00041 #if 0 00042 // Not seeing double keys from Mitsubishi 00043 if (results->rawbuf[offset] < MITSUBISHI_DOUBLE_SPACE_USECS) { 00044 // Serial.print("IR Gap found: "); 00045 results->bits = 0; 00046 results->value = REPEAT; 00047 results->decode_type = MITSUBISHI; 00048 return true; 00049 } 00050 #endif 00051 00052 offset++; 00053 00054 // Typical 00055 // 14200 7 41 7 42 7 42 7 17 7 17 7 18 7 41 7 18 7 17 7 17 7 18 7 41 8 17 7 17 7 18 7 17 7 00056 00057 // Initial Space 00058 if (!MATCH_MARK(results->rawbuf[offset], MITSUBISHI_HDR_SPACE)) return false ; 00059 offset++; 00060 00061 while (offset + 1 < irparams.rawlen) { 00062 if (MATCH_MARK(results->rawbuf[offset], MITSUBISHI_ONE_MARK)) data = (data << 1) | 1 ; 00063 else if (MATCH_MARK(results->rawbuf[offset], MITSUBISHI_ZERO_MARK)) data <<= 1 ; 00064 else return false ; 00065 offset++; 00066 00067 if (!MATCH_SPACE(results->rawbuf[offset], MITSUBISHI_HDR_SPACE)) break ; 00068 offset++; 00069 } 00070 00071 // Success 00072 results->bits = (offset - 1) / 2; 00073 if (results->bits < MITSUBISHI_BITS) { 00074 results->bits = 0; 00075 return false; 00076 } 00077 00078 results->value = data; 00079 results->decode_type = MITSUBISHI; 00080 return true; 00081 } 00082 #endif 00083
Generated on Fri Jul 15 2022 02:03:08 by
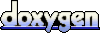