Infrared remote library for Arduino: send and receive infrared signals with multiple protocols Port from Arduino-IRremote https://github.com/z3t0/Arduino-IRremote
irRecv.cpp
00001 #include "IRremote.h" 00002 #include "IRremoteInt.h" 00003 00004 //+============================================================================= 00005 // Decodes the received IR message 00006 // Returns 0 if no data ready, 1 if data ready. 00007 // Results of decoding are stored in results 00008 // 00009 int IRrecv::decode (decode_results *results) 00010 { 00011 results->rawbuf = irparams.rawbuf; 00012 results->rawlen = irparams.rawlen; 00013 00014 results->overflow = irparams.overflow; 00015 00016 if (irparams.rcvstate != STATE_STOP) return false ; 00017 00018 #if DECODE_NEC 00019 DBG_PRINTLN("Attempting NEC decode"); 00020 if (decodeNEC(results)) return true ; 00021 #endif 00022 00023 #if DECODE_SONY 00024 DBG_PRINTLN("Attempting Sony decode"); 00025 if (decodeSony(results)) return true ; 00026 #endif 00027 00028 #if DECODE_SANYO 00029 DBG_PRINTLN("Attempting Sanyo decode"); 00030 if (decodeSanyo(results)) return true ; 00031 #endif 00032 00033 #if DECODE_MITSUBISHI 00034 DBG_PRINTLN("Attempting Mitsubishi decode"); 00035 if (decodeMitsubishi(results)) return true ; 00036 #endif 00037 00038 #if DECODE_RC5 00039 DBG_PRINTLN("Attempting RC5 decode"); 00040 if (decodeRC5(results)) return true ; 00041 #endif 00042 00043 #if DECODE_RC6 00044 DBG_PRINTLN("Attempting RC6 decode"); 00045 if (decodeRC6(results)) return true ; 00046 #endif 00047 00048 #if DECODE_PANASONIC 00049 DBG_PRINTLN("Attempting Panasonic decode"); 00050 if (decodePanasonic(results)) return true ; 00051 #endif 00052 00053 #if DECODE_LG 00054 DBG_PRINTLN("Attempting LG decode"); 00055 if (decodeLG(results)) return true ; 00056 #endif 00057 00058 #if DECODE_JVC 00059 DBG_PRINTLN("Attempting JVC decode"); 00060 if (decodeJVC(results)) return true ; 00061 #endif 00062 00063 #if DECODE_SAMSUNG 00064 DBG_PRINTLN("Attempting SAMSUNG decode"); 00065 if (decodeSAMSUNG(results)) return true ; 00066 #endif 00067 00068 #if DECODE_WHYNTER 00069 DBG_PRINTLN("Attempting Whynter decode"); 00070 if (decodeWhynter(results)) return true ; 00071 #endif 00072 00073 #if DECODE_AIWA_RC_T501 00074 DBG_PRINTLN("Attempting Aiwa RC-T501 decode"); 00075 if (decodeAiwaRCT501(results)) return true ; 00076 #endif 00077 00078 #if DECODE_DENON 00079 DBG_PRINTLN("Attempting Denon decode"); 00080 if (decodeDenon(results)) return true ; 00081 #endif 00082 00083 // decodeHash returns a hash on any input. 00084 // Thus, it needs to be last in the list. 00085 // If you add any decodes, add them before this. 00086 if (decodeHash(results)) return true ; 00087 00088 // Throw away and start over 00089 resume(); 00090 return false; 00091 } 00092 00093 //+============================================================================= 00094 IRrecv::IRrecv (PinName recvpin) : _recvpin(recvpin) 00095 { 00096 _recvpin.mode(PullNone); 00097 } 00098 00099 //+============================================================================= 00100 // enable IR receive 00101 // 00102 void IRrecv::enableIRIn ( ) 00103 { 00104 _ticker.detach(); 00105 _ticker.attach_us(callback(this, &IRrecv::timer_isr), USECPERTICK); 00106 _ticker.attach_us(this, &IRrecv::timer_isr, USECPERTICK); 00107 00108 // Initialize state machine variables 00109 irparams.rcvstate = STATE_IDLE; 00110 irparams.rawlen = 0; 00111 } 00112 00113 //+============================================================================= 00114 // disable IR receive 00115 // 00116 void IRrecv::disableIRIn ( ) 00117 { 00118 _ticker.detach(); 00119 } 00120 00121 //+============================================================================= 00122 // Return if receiving new IR signals 00123 // 00124 bool IRrecv::isIdle ( ) 00125 { 00126 return irparams.rcvstate == STATE_IDLE || irparams.rcvstate == STATE_STOP; 00127 } 00128 00129 //+============================================================================= 00130 // Restart the ISR state machine 00131 // 00132 void IRrecv::resume ( ) 00133 { 00134 irparams.rcvstate = STATE_IDLE; 00135 irparams.rawlen = 0; 00136 } 00137 00138 //+============================================================================= 00139 // hashdecode - decode an arbitrary IR code. 00140 // Instead of decoding using a standard encoding scheme 00141 // (e.g. Sony, NEC, RC5), the code is hashed to a 32-bit value. 00142 // 00143 // The algorithm: look at the sequence of MARK signals, and see if each one 00144 // is shorter (0), the same length (1), or longer (2) than the previous. 00145 // Do the same with the SPACE signals. Hash the resulting sequence of 0's, 00146 // 1's, and 2's to a 32-bit value. This will give a unique value for each 00147 // different code (probably), for most code systems. 00148 // 00149 // http://arcfn.com/2010/01/using-arbitrary-remotes-with-arduino.html 00150 // 00151 // Compare two tick values, returning 0 if newval is shorter, 00152 // 1 if newval is equal, and 2 if newval is longer 00153 // Use a tolerance of 20% 00154 // 00155 int IRrecv::compare (unsigned int oldval, unsigned int newval) 00156 { 00157 if (newval < oldval * .8) return 0 ; 00158 else if (oldval < newval * .8) return 2 ; 00159 else return 1 ; 00160 } 00161 00162 //+============================================================================= 00163 // Use FNV hash algorithm: http://isthe.com/chongo/tech/comp/fnv/#FNV-param 00164 // Converts the raw code values into a 32-bit hash code. 00165 // Hopefully this code is unique for each button. 00166 // This isn't a "real" decoding, just an arbitrary value. 00167 // 00168 #define FNV_PRIME_32 16777619 00169 #define FNV_BASIS_32 2166136261 00170 00171 long IRrecv::decodeHash (decode_results *results) 00172 { 00173 unsigned long hash = FNV_BASIS_32; 00174 00175 // Require at least 6 samples to prevent triggering on noise 00176 if (results->rawlen < 6) return false ; 00177 00178 for (int i = 1; (i + 2) < results->rawlen; i++) { 00179 int value = compare(results->rawbuf[i], results->rawbuf[i+2]); 00180 // Add value into the hash 00181 hash = (hash * FNV_PRIME_32) ^ value; 00182 } 00183 00184 results->value = hash; 00185 results->bits = 32; 00186 results->decode_type = UNKNOWN; 00187 00188 return true; 00189 }
Generated on Fri Jul 15 2022 02:03:08 by
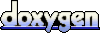