Infrared remote library for Arduino: send and receive infrared signals with multiple protocols Port from Arduino-IRremote https://github.com/z3t0/Arduino-IRremote
IRremote.h
00001 00002 //****************************************************************************** 00003 // IRremote 00004 // Version 2.0.1 June, 2015 00005 // Copyright 2009 Ken Shirriff 00006 // For details, see http://arcfn.com/2009/08/multi-protocol-infrared-remote-library.html 00007 // Edited by Mitra to add new controller SANYO 00008 // 00009 // Interrupt code based on NECIRrcv by Joe Knapp 00010 // http://www.arduino.cc/cgi-bin/yabb2/YaBB.pl?num=1210243556 00011 // Also influenced by http://zovirl.com/2008/11/12/building-a-universal-remote-with-an-arduino/ 00012 // 00013 // JVC and Panasonic protocol added by Kristian Lauszus (Thanks to zenwheel and other people at the original blog post) 00014 // LG added by Darryl Smith (based on the JVC protocol) 00015 // Whynter A/C ARC-110WD added by Francesco Meschia 00016 //****************************************************************************** 00017 00018 #ifndef IRremote_h 00019 #define IRremote_h 00020 00021 //------------------------------------------------------------------------------ 00022 // The ISR header contains several useful macros the user may wish to use 00023 // 00024 #include "IRremoteInt.h" 00025 00026 //------------------------------------------------------------------------------ 00027 // Supported IR protocols 00028 // Each protocol you include costs memory and, during decode, costs time 00029 // Disable (set to 0) all the protocols you do not need/want! 00030 // 00031 #define DECODE_RC5 1 00032 #define SEND_RC5 1 00033 00034 #define DECODE_RC6 1 00035 #define SEND_RC6 1 00036 00037 #define DECODE_NEC 1 00038 #define SEND_NEC 1 00039 00040 #define DECODE_SONY 1 00041 #define SEND_SONY 1 00042 00043 #define DECODE_PANASONIC 1 00044 #define SEND_PANASONIC 1 00045 00046 #define DECODE_JVC 1 00047 #define SEND_JVC 1 00048 00049 #define DECODE_SAMSUNG 1 00050 #define SEND_SAMSUNG 1 00051 00052 #define DECODE_WHYNTER 1 00053 #define SEND_WHYNTER 1 00054 00055 #define DECODE_AIWA_RC_T501 1 00056 #define SEND_AIWA_RC_T501 1 00057 00058 #define DECODE_LG 1 00059 #define SEND_LG 1 00060 00061 #define DECODE_SANYO 1 00062 #define SEND_SANYO 0 // NOT WRITTEN 00063 00064 #define DECODE_MITSUBISHI 1 00065 #define SEND_MITSUBISHI 0 // NOT WRITTEN 00066 00067 #define DECODE_DISH 0 // NOT WRITTEN 00068 #define SEND_DISH 1 00069 00070 #define DECODE_SHARP 0 // NOT WRITTEN 00071 #define SEND_SHARP 1 00072 00073 #define DECODE_DENON 1 00074 #define SEND_DENON 1 00075 00076 #define DECODE_PRONTO 0 // This function doe not logically make sense 00077 #define SEND_PRONTO 1 00078 00079 //------------------------------------------------------------------------------ 00080 // When sending a Pronto code we request to send either the "once" code 00081 // or the "repeat" code 00082 // If the code requested does not exist we can request to fallback on the 00083 // other code (the one we did not explicitly request) 00084 // 00085 // I would suggest that "fallback" will be the standard calling method 00086 // The last paragraph on this page discusses the rationale of this idea: 00087 // http://www.remotecentral.com/features/irdisp2.htm 00088 // 00089 #define PRONTO_ONCE false 00090 #define PRONTO_REPEAT true 00091 #define PRONTO_FALLBACK true 00092 #define PRONTO_NOFALLBACK false 00093 00094 //------------------------------------------------------------------------------ 00095 // An enumerated list of all supported formats 00096 // You do NOT need to remove entries from this list when disabling protocols! 00097 // 00098 typedef 00099 enum { 00100 UNKNOWN = -1, 00101 UNUSED = 0, 00102 RC5, 00103 RC6, 00104 NEC, 00105 SONY, 00106 PANASONIC, 00107 JVC, 00108 SAMSUNG, 00109 WHYNTER, 00110 AIWA_RC_T501, 00111 LG, 00112 SANYO, 00113 MITSUBISHI, 00114 DISH, 00115 SHARP, 00116 DENON, 00117 PRONTO, 00118 } 00119 decode_type_t; 00120 00121 //------------------------------------------------------------------------------ 00122 // Set DEBUG to 1 for lots of lovely debug output 00123 // 00124 #define DEBUG 0 00125 00126 //------------------------------------------------------------------------------ 00127 // Debug directives 00128 // 00129 #if DEBUG 00130 # define DBG_PRINT(...) Serial.print(__VA_ARGS__) 00131 # define DBG_PRINTLN(...) Serial.println(__VA_ARGS__) 00132 #else 00133 # define DBG_PRINT(...) 00134 # define DBG_PRINTLN(...) 00135 #endif 00136 00137 //------------------------------------------------------------------------------ 00138 // Mark & Space matching functions 00139 // 00140 int MATCH (int measured, int desired) ; 00141 int MATCH_MARK (int measured_ticks, int desired_us) ; 00142 int MATCH_SPACE (int measured_ticks, int desired_us) ; 00143 00144 //------------------------------------------------------------------------------ 00145 // Results returned from the decoder 00146 // 00147 class decode_results 00148 { 00149 public: 00150 decode_type_t decode_type; // UNKNOWN, NEC, SONY, RC5, ... 00151 unsigned int address; // Used by Panasonic & Sharp [16-bits] 00152 unsigned long value; // Decoded value [max 32-bits] 00153 int bits; // Number of bits in decoded value 00154 unsigned int *rawbuf; // Raw intervals in 50uS ticks 00155 int rawlen; // Number of records in rawbuf 00156 int overflow; // true iff IR raw code too long 00157 }; 00158 00159 //------------------------------------------------------------------------------ 00160 // Decoded value for NEC when a repeat code is received 00161 // 00162 #define REPEAT 0xFFFFFFFF 00163 00164 //------------------------------------------------------------------------------ 00165 // Main class for receiving IR 00166 // 00167 class IRrecv 00168 { 00169 public: 00170 IRrecv (PinName recvpin) ; 00171 int decode (decode_results *results) ; 00172 void enableIRIn ( ) ; 00173 void disableIRIn ( ) ; 00174 bool isIdle ( ) ; 00175 void resume ( ) ; 00176 00177 private: 00178 void timer_isr (); 00179 long decodeHash (decode_results *results) ; 00180 int compare (unsigned int oldval, unsigned int newval) ; 00181 00182 //...................................................................... 00183 # if (DECODE_RC5 || DECODE_RC6) 00184 // This helper function is shared by RC5 and RC6 00185 int getRClevel (decode_results *results, int *offset, int *used, int t1) ; 00186 # endif 00187 # if DECODE_RC5 00188 bool decodeRC5 (decode_results *results) ; 00189 # endif 00190 # if DECODE_RC6 00191 bool decodeRC6 (decode_results *results) ; 00192 # endif 00193 //...................................................................... 00194 # if DECODE_NEC 00195 bool decodeNEC (decode_results *results) ; 00196 # endif 00197 //...................................................................... 00198 # if DECODE_SONY 00199 bool decodeSony (decode_results *results) ; 00200 # endif 00201 //...................................................................... 00202 # if DECODE_PANASONIC 00203 bool decodePanasonic (decode_results *results) ; 00204 # endif 00205 //...................................................................... 00206 # if DECODE_JVC 00207 bool decodeJVC (decode_results *results) ; 00208 # endif 00209 //...................................................................... 00210 # if DECODE_SAMSUNG 00211 bool decodeSAMSUNG (decode_results *results) ; 00212 # endif 00213 //...................................................................... 00214 # if DECODE_WHYNTER 00215 bool decodeWhynter (decode_results *results) ; 00216 # endif 00217 //...................................................................... 00218 # if DECODE_AIWA_RC_T501 00219 bool decodeAiwaRCT501 (decode_results *results) ; 00220 # endif 00221 //...................................................................... 00222 # if DECODE_LG 00223 bool decodeLG (decode_results *results) ; 00224 # endif 00225 //...................................................................... 00226 # if DECODE_SANYO 00227 bool decodeSanyo (decode_results *results) ; 00228 # endif 00229 //...................................................................... 00230 # if DECODE_MITSUBISHI 00231 bool decodeMitsubishi (decode_results *results) ; 00232 # endif 00233 //...................................................................... 00234 # if DECODE_DISH 00235 bool decodeDish (decode_results *results) ; // NOT WRITTEN 00236 # endif 00237 //...................................................................... 00238 # if DECODE_SHARP 00239 bool decodeSharp (decode_results *results) ; // NOT WRITTEN 00240 # endif 00241 //...................................................................... 00242 # if DECODE_DENON 00243 bool decodeDenon (decode_results *results) ; 00244 # endif 00245 00246 DigitalIn _recvpin; 00247 Ticker _ticker; 00248 irparams_t irparams; 00249 } ; 00250 00251 //------------------------------------------------------------------------------ 00252 // Main class for sending IR 00253 // 00254 class IRsend 00255 { 00256 public: 00257 IRsend (PinName sendpin) : _pwm(sendpin) { } 00258 00259 void custom_delay_usec (unsigned long uSecs); 00260 void enableIROut (int khz) ; 00261 void mark (unsigned int usec) ; 00262 void space (unsigned int usec) ; 00263 void sendRaw (unsigned int buf[], unsigned int len, unsigned int hz) ; 00264 00265 //...................................................................... 00266 # if SEND_RC5 00267 void sendRC5 (unsigned long data, int nbits) ; 00268 # endif 00269 # if SEND_RC6 00270 void sendRC6 (unsigned long data, int nbits) ; 00271 # endif 00272 //...................................................................... 00273 # if SEND_NEC 00274 void sendNEC (unsigned long data, int nbits) ; 00275 # endif 00276 //...................................................................... 00277 # if SEND_SONY 00278 void sendSony (unsigned long data, int nbits) ; 00279 # endif 00280 //...................................................................... 00281 # if SEND_PANASONIC 00282 void sendPanasonic (unsigned int address, unsigned long data) ; 00283 # endif 00284 //...................................................................... 00285 # if SEND_JVC 00286 // JVC does NOT repeat by sending a separate code (like NEC does). 00287 // The JVC protocol repeats by skipping the header. 00288 // To send a JVC repeat signal, send the original code value 00289 // and set 'repeat' to true 00290 void sendJVC (unsigned long data, int nbits, bool repeat) ; 00291 # endif 00292 //...................................................................... 00293 # if SEND_SAMSUNG 00294 void sendSAMSUNG (unsigned long data, int nbits) ; 00295 # endif 00296 //...................................................................... 00297 # if SEND_WHYNTER 00298 void sendWhynter (unsigned long data, int nbits) ; 00299 # endif 00300 //...................................................................... 00301 # if SEND_AIWA_RC_T501 00302 void sendAiwaRCT501 (int code) ; 00303 # endif 00304 //...................................................................... 00305 # if SEND_LG 00306 void sendLG (unsigned long data, int nbits) ; 00307 # endif 00308 //...................................................................... 00309 # if SEND_SANYO 00310 void sendSanyo ( ) ; // NOT WRITTEN 00311 # endif 00312 //...................................................................... 00313 # if SEND_MISUBISHI 00314 void sendMitsubishi ( ) ; // NOT WRITTEN 00315 # endif 00316 //...................................................................... 00317 # if SEND_DISH 00318 void sendDISH (unsigned long data, int nbits) ; 00319 # endif 00320 //...................................................................... 00321 # if SEND_SHARP 00322 void sendSharpRaw (unsigned long data, int nbits) ; 00323 void sendSharp (unsigned int address, unsigned int command) ; 00324 # endif 00325 //...................................................................... 00326 # if SEND_DENON 00327 void sendDenon (unsigned long data, int nbits) ; 00328 # endif 00329 //...................................................................... 00330 # if SEND_PRONTO 00331 void sendPronto (char* code, bool repeat, bool fallback) ; 00332 # endif 00333 00334 PwmOut _pwm; 00335 } ; 00336 00337 #endif
Generated on Fri Jul 15 2022 02:03:08 by
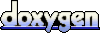