Infrared remote library for Arduino: send and receive infrared signals with multiple protocols Port from Arduino-IRremote https://github.com/z3t0/Arduino-IRremote
IRremoteInt.h
00001 //****************************************************************************** 00002 // IRremote 00003 // Version 2.0.1 June, 2015 00004 // Copyright 2009 Ken Shirriff 00005 // For details, see http://arcfn.com/2009/08/multi-protocol-infrared-remote-library.html 00006 // 00007 // Modified by Paul Stoffregen <paul@pjrc.com> to support other boards and timers 00008 // 00009 // Interrupt code based on NECIRrcv by Joe Knapp 00010 // http://www.arduino.cc/cgi-bin/yabb2/YaBB.pl?num=1210243556 00011 // Also influenced by http://zovirl.com/2008/11/12/building-a-universal-remote-with-an-arduino/ 00012 // 00013 // JVC and Panasonic protocol added by Kristian Lauszus (Thanks to zenwheel and other people at the original blog post) 00014 // Whynter A/C ARC-110WD added by Francesco Meschia 00015 //****************************************************************************** 00016 00017 #ifndef IRremoteint_h 00018 #define IRremoteint_h 00019 00020 //------------------------------------------------------------------------------ 00021 // Include the right mbed header 00022 // 00023 #include "mbed.h" 00024 00025 //------------------------------------------------------------------------------ 00026 // Information for the Interrupt Service Routine 00027 // 00028 #define RAWBUF 101 // Maximum length of raw duration buffer 00029 00030 typedef 00031 struct { 00032 // The fields are ordered to reduce memory over caused by struct-padding 00033 uint8_t rcvstate; // State Machine state 00034 uint8_t rawlen; // counter of entries in rawbuf 00035 unsigned int timer; // State timer, counts 50uS ticks. 00036 unsigned int rawbuf[RAWBUF]; // raw data 00037 uint8_t overflow; // Raw buffer overflow occurred 00038 } 00039 irparams_t; 00040 00041 // ISR State-Machine : Receiver States 00042 #define STATE_IDLE 2 00043 #define STATE_MARK 3 00044 #define STATE_SPACE 4 00045 #define STATE_STOP 5 00046 #define STATE_OVERFLOW 6 00047 00048 //------------------------------------------------------------------------------ 00049 // Pulse parms are ((X*50)-100) for the Mark and ((X*50)+100) for the Space. 00050 // First MARK is the one after the long gap 00051 // Pulse parameters in uSec 00052 // 00053 00054 // Due to sensor lag, when received, Marks tend to be 100us too long and 00055 // Spaces tend to be 100us too short 00056 #define MARK_EXCESS 100 00057 00058 // microseconds per clock interrupt tick 00059 #define USECPERTICK 100 00060 00061 // Upper and Lower percentage tolerances in measurements 00062 #define TOLERANCE 25 00063 #define LTOL (1.0 - (TOLERANCE/100.)) 00064 #define UTOL (1.0 + (TOLERANCE/100.)) 00065 00066 // Minimum gap between IR transmissions 00067 #define _GAP 5000 00068 #define GAP_TICKS (_GAP/USECPERTICK) 00069 00070 #define TICKS_LOW(us) ((int)(((us)*LTOL/USECPERTICK))) 00071 #define TICKS_HIGH(us) ((int)(((us)*UTOL/USECPERTICK + 1))) 00072 00073 //------------------------------------------------------------------------------ 00074 // IR detector output is active low 00075 // 00076 #define MARK 0 00077 #define SPACE 1 00078 00079 #endif
Generated on Fri Jul 15 2022 02:03:08 by
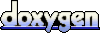