driver for the WNC M14A2A Cellular Data Module
Embed:
(wiki syntax)
Show/hide line numbers
WncController.h
Go to the documentation of this file.
00001 /** 00002 Copyright (c) 2016 Fred Kellerman 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 00022 @file WncController.h 00023 @purpose Controls WNC Cellular Modem 00024 @version 1.0 00025 @date July 2016 00026 @author Fred Kellerman 00027 00028 Notes: This code originates from the following mbed repository: 00029 00030 https://developer.mbed.org/teams/Avnet/code/WncControllerLibrary/ 00031 */ 00032 00033 00034 #ifndef __WNCCONTROLLER_H_ 00035 #define __WNCCONTROLLER_H_ 00036 00037 #include <string> 00038 #include <stdint.h> 00039 00040 namespace WncController_fk { 00041 00042 using namespace std; 00043 00044 /** @defgroup API The WncControllerLibrary API */ 00045 /** @defgroup MISC Misc WncControllerLibrary functions */ 00046 /** @defgroup INTERNALS WncControllerLibrary Internals */ 00047 00048 static const uint8_t MAX_LEN_IP_STR = 16; // Length includes room for the extra NULL 00049 00050 /** \brief Contains info fields for the WNC Internet Attributes */ 00051 struct WncIpStats 00052 { 00053 string wncMAC; 00054 char ip[MAX_LEN_IP_STR]; 00055 char mask[MAX_LEN_IP_STR]; 00056 char gateway[MAX_LEN_IP_STR]; 00057 char dnsPrimary[MAX_LEN_IP_STR]; 00058 char dnsSecondary[MAX_LEN_IP_STR]; 00059 }; 00060 00061 00062 /** 00063 * @author Fred Kellerman 00064 * @see API 00065 * 00066 * <b>WncController</b> This mbed C++ class is for controlling the WNC 00067 * Cellular modem via the serial AT command interface. This was 00068 * developed with respect to version 1.3 of the WNC authored 00069 * unpublished spec. This class is only designed to have 1 instantiation, 00070 * it is also not multi-thread safe. There are no OS specific 00071 * entities being used, there are pure virtual methods that an 00072 * inheriting class must fulfill. That inheriting class will have 00073 * OS and platform specific entities. See WncControllerK64F for an 00074 * example for the NXP K64F Freedom board. 00075 */ 00076 class WncController 00077 { 00078 public: 00079 00080 static const unsigned MAX_NUM_WNC_SOCKETS = 5; // Max number of simultaneous sockets that the WNC supports 00081 static const unsigned MAX_POWERUP_TIMEOUT = 60; // How long the powerUp method will try to turn on the WNC Shield 00082 // (this is the default if the user does not over-ride on power-up 00083 00084 /** Tracks mode of the WNC Shield hardware */ 00085 enum WncState_e { 00086 WNC_OFF = 0, 00087 WNC_ON, // This is intended to mean all systems go, including cell link up but socket may not be open 00088 WNC_ON_NO_CELL_LINK, 00089 WNC_NO_RESPONSE 00090 }; 00091 00092 /** 00093 * 00094 * Constructor for WncController class, sets up internals. 00095 * @ingroup API 00096 * @return none. 00097 */ 00098 WncController (void); 00099 virtual ~WncController ()=0; 00100 00101 /** 00102 * 00103 * Used internally but also make public for a user of the Class to 00104 * interrogate state as well. 00105 * @ingroup API 00106 * @return the current state of the Wnc hardware. 00107 */ 00108 WncState_e getWncStatus(void); 00109 00110 /** 00111 * 00112 * Allows a user to set the WNC modem to use the given Cellular APN 00113 * @ingroup API 00114 * @param apnStr - a null terminated c-string 00115 * @return true if the APN set was succesful, else false 00116 */ 00117 bool setApnName(const char * const apnStr); 00118 00119 /** 00120 * 00121 * Queries the WNC modem for the current RX RSSI in units of coded dBm 00122 * @ingroup API 00123 * @return 0 – -113 dBm or less 00124 * 1 – -111 dBm 00125 * 2...30 – -109 dBm to –53 dBm 00126 * 31 – -51 dBm or greater 00127 * 99 – not known or not detectable 00128 */ 00129 int16_t getDbmRssi(void); 00130 00131 /** 00132 * 00133 * Queries the WNC modem for the current Bit Error Rate 00134 * @ingroup API 00135 * @return 0...7 – as RXQUAL values in the table in 3GPP TS 45.008 00136 * subclause 8.2.4 00137 * 99 – not known or not detectable 00138 */ 00139 int16_t get3gBer(void); 00140 00141 /** 00142 * 00143 * Powers up the WNC modem 00144 * @ingroup API 00145 * @param apn - the apn c-string to set the WNC modem to use 00146 * @param powerUpTimeoutSecs - the amount of time to wait for the WNC modem to turn on 00147 * @return true if powerup was a success, else false. 00148 */ 00149 bool powerWncOn(const char * const apn, uint8_t powerUpTimeoutSecs = MAX_POWERUP_TIMEOUT); 00150 00151 /** 00152 * 00153 * Returns the NAT Self, gateway, masks and dns IP 00154 * @ingroup API 00155 * @param s - a pointer to a struct that will contain the IP info. 00156 * @return true if success, else false. 00157 */ 00158 bool getWncNetworkingStats(WncIpStats * s); 00159 00160 /** 00161 * 00162 * Takes a text URL and converts it internally to an IP address for the 00163 * socket number given. 00164 * @ingroup API 00165 * @param numSock - The number of the socket to lookup the IP address for. 00166 * @param url - a c-string text URL 00167 * @return true if success, else false. 00168 */ 00169 bool resolveUrl(uint16_t numSock, const char * url); 00170 00171 /** 00172 * 00173 * If you know the IP address you can set the socket up to use it rather 00174 * than using a text URL. 00175 * @ingroup API 00176 * @param numSock - The number of the socket to use the IP address for. 00177 * @param ipStr - a c-string text IP addrese like: 192.168.0.1 00178 * @return true if success, else false. 00179 */ 00180 bool setIpAddr(uint16_t numSock, const char * ipStr); 00181 00182 /** 00183 * 00184 * Opens a socket for the given number, port and IP protocol. Before 00185 * using open, you must use either resolveUrl() or setIpAddr(). 00186 * @ingroup API 00187 * @param numSock - The number of the socket to open. 00188 * @param port - the IP port to open 00189 * @param tcp - set true for TCP, false for UDP 00190 * @param timeoutSec - the amount of time in seconds to wait for the open to complete 00191 * @return true if success, else false. 00192 */ 00193 bool openSocket(uint16_t numSock, uint16_t port, bool tcp, uint16_t timeOutSec = 30); 00194 00195 /** 00196 * 00197 * Opens a socket for the given text URL, number, port and IP protocol. 00198 * @ingroup API 00199 * @param numSock - The number of the socket to open. 00200 * @param url - a c-string text URL, the one to open a socket for. 00201 * @param port - the IP port to open. 00202 * @param tcp - set true for TCP, false for UDP. 00203 * @param timeoutSec - the amount of time in seconds to wait for the open to complete. 00204 * @return true if success, else false. 00205 */ 00206 bool openSocketUrl(uint16_t numSock, const char * url, uint16_t port, bool tcp, uint16_t timeOutSec = 30); 00207 00208 /** 00209 * 00210 * Opens a socket for the given text IP address, number, port and IP protocol. 00211 * @ingroup API 00212 * @param numSock - The number of the socket to open. 00213 * @param ipAddr - a c-string text IP address like: "192.168.0.1". 00214 * @param port - the IP port to open. 00215 * @param tcp - set true for TCP, false for UDP. 00216 * @param timeoutSec - the amount of time in seconds to wait for the open to complete. 00217 * @return true if success, else false. 00218 */ 00219 bool openSocketIpAddr(uint16_t numSock, const char * ipAddr, uint16_t port, bool tcp, uint16_t timeOutSec = 30); 00220 00221 00222 /** 00223 * 00224 * Write data bytes to a Socket, the Socket must already be open. 00225 * @ingroup API 00226 * @param numSock - The number of the socket to open. 00227 * @parma s - an array of bytes to write to the socket. 00228 * @param n - the number of bytes to write. 00229 * @return true if success, else false. 00230 */ 00231 bool write(uint16_t numSock, const uint8_t * s, uint32_t n); 00232 00233 /** 00234 * 00235 * Poll to read available data bytes from an already open Socket. This method 00236 * will retry reads to what setReadRetries() sets it to and the delay in between 00237 * retries that is set with setReadRetryWait() 00238 * @ingroup API 00239 * @param numSock - The number of the socket to open. 00240 * @parma readBuf - a pointer to where read will put the data. 00241 * @param maxReadBufLen - The number of bytes readBuf has room for. 00242 * @return the number of bytes actually read into readBuf. 0 is a valid value if no data is available. 00243 */ 00244 size_t read(uint16_t numSock, uint8_t * readBuf, uint32_t maxReadBufLen); 00245 00246 /** 00247 * 00248 * Poll to read available data bytes from an already open Socket. This method 00249 * will retry reads to what setReadRetries() sets it to and the delay in between 00250 * retries that is set with setReadRetryWait() 00251 * @ingroup API 00252 * @param numSock - The number of the socket to open. 00253 * @parma readBuf - a pointer to pointer that will be set to point to an internal byte buffer that contains any read data. 00254 * @return the number of bytes actually read into the pointer that readBuf points to. 0 is a valid value if no data is available. 00255 */ 00256 size_t read(uint16_t numSock, const uint8_t ** readBuf); 00257 00258 /** 00259 * 00260 * Set the number of retries that the read methods will use. If a read returns 0 data this setting will have the read 00261 * re-read to see if new data is available. 00262 * @ingroup API 00263 * @param numSock - The number of the socket to open. 00264 * @parma retries - the number of retries to perform. 00265 * @return none. 00266 */ 00267 void setReadRetries(uint16_t numSock, uint16_t retries); 00268 00269 /** 00270 * 00271 * Set the time between retires that the read methods will use. If a read returns 0 data this setting will have the read 00272 * re-read and use this amount of delay in between the re-reads. 00273 * @ingroup API 00274 * @param numSock - The number of the socket to open. 00275 * @parma waitMs - the amount of time in mS to wait between retries. 00276 * @return none. 00277 */ 00278 void setReadRetryWait(uint16_t numSock, uint16_t waitMs); 00279 00280 /** 00281 * 00282 * Closes an already open Socket. 00283 * @ingroup API 00284 * @param numSock - The number of the socket to open. 00285 * @return true if success else false. 00286 */ 00287 bool closeSocket(uint16_t numSock); 00288 00289 /** 00290 * 00291 * Sets the amount of time to wait between the raw AT commands that are sent to the WNC modem. 00292 * Generally you don't want to use this but it is here just in case. 00293 * @ingroup API 00294 * @param toMs - num mS to wait between the AT cmds. 00295 * @return none. 00296 */ 00297 void setWncCmdTimeout(uint16_t toMs); 00298 00299 /** 00300 * 00301 * Gets the IP address of the given socket number. 00302 * @ingroup API 00303 * @param numSock - The number of the socket to open. 00304 * @param myIpAddr - a c-string that contains the socket's IP address. 00305 * @return true if success else false. 00306 */ 00307 bool getIpAddr(uint16_t numSock, char myIpAddr[MAX_LEN_IP_STR]); 00308 00309 /** 00310 * 00311 * Enables debug output from this class. 00312 * @ingroup API 00313 * @param on - true enables debug output, false disables 00314 * @param moreDebugOn - true enables verbose debug, false truncates debug output. 00315 * @return none. 00316 */ 00317 void enableDebug(bool on, bool moreDebugOn); 00318 00319 /////////////////////////////////////////// 00320 // SMS messaging 00321 /////////////////////////////////////////// 00322 00323 static const uint16_t MAX_WNC_SMS_MSG_SLOTS = 3; // How many SMS messages the WNC can store and receive at a time. 00324 static const uint16_t MAX_WNC_SMS_LENGTH = 160; // The maximum length of a 7-bit SMS message the WNC can send and receive. 00325 00326 /** Struct for SMS messages */ 00327 struct WncSmsInfo 00328 { 00329 // Content 00330 char idx; 00331 string number; 00332 string date; 00333 string time; 00334 string msg; 00335 00336 // Attributes 00337 bool incoming; 00338 bool unsent; 00339 bool unread; 00340 bool pduMode; 00341 bool msgReceipt; 00342 }; 00343 00344 /** Struct to contain a list of SMS message structs */ 00345 struct WncSmsList 00346 { 00347 uint8_t msgCount; 00348 WncSmsInfo e[MAX_WNC_SMS_MSG_SLOTS]; 00349 }; 00350 00351 /** 00352 * 00353 * Sends an SMS text message to someone. 00354 * @ingroup API 00355 * @param phoneNum - c-string 15 digit MSISDN number or ATT Jasper number (standard phone number not supported because ATT IoT SMS does not support it). 00356 * @param text - the c-string text to send to someone. 00357 * @return true if success else false. 00358 */ 00359 bool sendSMSText(const char * const phoneNum, const char * const text); 00360 00361 /** 00362 * 00363 * Incoming messages are stored in a log in the WNC modem, this will read that 00364 * log. 00365 * @ingroup API 00366 * @param log - the log contents if reading it was successful. 00367 * @return true if success else false. 00368 */ 00369 bool readSMSLog(struct WncSmsList * log); 00370 00371 /** 00372 * 00373 * Incoming messages are stored in a log in the WNC modem, this will read out 00374 * messages that are unread and also then mark them read. 00375 * @ingroup API 00376 * @param w - a list of SMS messages that unread messages will be put into. 00377 * @param deleteRead - if a message is read and this is set true the message will be deleted from the WNC modem log. 00378 * If it is false the message will remain in the internal log but be marked as read. 00379 * @return true if success else false. 00380 */ 00381 bool readUnreadSMSText(struct WncSmsList * w, bool deleteRead = true); 00382 00383 /** 00384 * 00385 * Saves a text message into internal SIM card memory of the WNC modem. 00386 * There are only 3 slots available this is for unread, read and saved. 00387 * @ingroup API 00388 * @param phoneNum - c-string 15 digit MSISDN number or ATT Jasper number (standard phone number not supported because ATT IoT SMS does not support it). 00389 * @param text - the c-string text to send to someone. 00390 * @param msgIdx - the slot position to save the message: '1', '2', '3' 00391 * @return true if success else false. 00392 */ 00393 bool saveSMSText(const char * const phoneNum, const char * const text, char * msgIdx); 00394 00395 /** 00396 * 00397 * Sends a prior stored a text message from internal SIM card memory of the WNC modem. 00398 * If no messages are stored the behaviour of this method is undefined. 00399 * @ingroup API 00400 * @param msgIdx - the slot position to save the message: '1', '2', '3' 00401 * @return true if success else false. 00402 */ 00403 bool sendSMSTextFromMem(char msgIdx); 00404 00405 /** 00406 * 00407 * Deletes a prior stored a text message from internal SIM card memory of the WNC modem. 00408 * If no messages are stored the behaviour of this method is undefined. 00409 * @ingroup API 00410 * @param msgIdx - the slot position to save the message: '1', '2', '3' or '*' deletes them all. 00411 * @return true if success else false. 00412 */ 00413 bool deleteSMSTextFromMem(char msgIdx); 00414 00415 /** 00416 * 00417 * Retreives the SIM card ICCID number. 00418 * @ingroup API 00419 * @param iccid - a pointer to C++ string that contains the retrieved number. 00420 * @return true if success else false. 00421 */ 00422 bool getICCID(string * iccid); 00423 00424 /** 00425 * 00426 * Converts an ICCID number into a MSISDN number. The ATT SMS system for IoT only allows use of the 15-digit MSISDN number. 00427 * @ingroup API 00428 * @param iccid - the number to convert. 00429 * @param msisdn - points to a C++ string that has the converted number. 00430 * @return true if success else false. 00431 */ 00432 bool convertICCIDtoMSISDN(const string & iccid, string * msisdn); 00433 00434 /////////////////////////////////////////// 00435 // Neighborhood Cell Info 00436 /////////////////////////////////////////// 00437 00438 /** 00439 * 00440 * Fetches the signal quality log from the WNC modem. 00441 * @ingroup API 00442 * @param log - a pointer to an internal buffer who's contents contain the signal quality metrics. 00443 * @return The number of chars in the log. 00444 */ 00445 size_t getSignalQuality(const char ** log); 00446 00447 /** A struct for the WNC modem Date and Time */ 00448 struct WncDateTime 00449 { 00450 uint8_t year; 00451 uint8_t month; 00452 uint8_t day; 00453 uint8_t hour; 00454 uint8_t min; 00455 uint8_t sec; 00456 }; 00457 00458 /** 00459 * 00460 * Fetches the cell tower's time and date. The time is accurate when read 00461 * but significant delays exist between the time it is read and returned. 00462 * @ingroup API 00463 * @param tod - User supplies a pointer to a tod struct and this method fills it in. 00464 * @return true if success else false. 00465 */ 00466 bool getTimeDate(struct WncDateTime * tod); 00467 00468 /** 00469 * 00470 * ICMP Pings a URL, the results are only output to the debug log for now! 00471 * @ingroup API 00472 * @param url - a c-string whose URL is to be pinged. 00473 * @return true if success else false. 00474 */ 00475 bool pingUrl(const char * url); 00476 00477 /** 00478 * 00479 * ICMP Pings an IP, the results are only output to the debug log for now! 00480 * @ingroup API 00481 * @param ip - a c-string whose IP is to be pinged. 00482 * @return true if success else false. 00483 */ 00484 bool pingIp(const char * ip); 00485 00486 /** 00487 * 00488 * Allows a user to send a raw AT command to the WNC modem. 00489 * @ingroup API 00490 * @param cmd - the c-string cmd to send like: "AT" 00491 * @param resp - a pointer to the c-string cmd's response. 00492 * @param sizeRespBuf - how large the command response buffer is, sets the max response length. 00493 * @param ms_timeout - how long to wait for the WNC to respond to your command. 00494 * @return the number of characters in the response from the WNC modem. 00495 */ 00496 size_t sendCustomCmd(const char * cmd, char * resp, size_t sizeRespBuf, int ms_timeout); 00497 00498 protected: 00499 00500 // Debug output methods 00501 int dbgPutsNoTime(const char * s, bool crlf = true); 00502 int dbgPuts(const char * s, bool crlf = true); 00503 const char * _to_string(int64_t value); 00504 const char * _to_hex_string(uint8_t value); 00505 00506 // Sends commands to WNC via 00507 enum AtCmdErr_e { 00508 WNC_AT_CMD_OK, 00509 WNC_AT_CMD_ERR, 00510 WNC_AT_CMD_ERREXT, 00511 WNC_AT_CMD_ERRCME, 00512 WNC_AT_CMD_INVALID_RESPONSE, 00513 WNC_AT_CMD_TIMEOUT, 00514 WNC_AT_CMD_NO_CELL_LINK, 00515 WNC_AT_CMD_WNC_NOT_ON 00516 }; 00517 00518 bool waitForPowerOnModemToRespond(uint8_t powerUpTimeoutSecs); 00519 AtCmdErr_e sendWncCmd(const char * const s, string ** r, int ms_timeout); 00520 00521 // Users must define these functionalities in the inheriting class: 00522 // General I/O and timing: 00523 virtual int putc(char c) = 0; 00524 virtual int puts(const char * s) = 0; 00525 virtual char getc(void) = 0; 00526 virtual int charReady(void) = 0; 00527 virtual int dbgWriteChar(char b) = 0; 00528 virtual int dbgWriteChars(const char *b) = 0; 00529 virtual void waitMs(int t) = 0; 00530 virtual void waitUs(int t) = 0; 00531 virtual bool initWncModem(uint8_t powerUpTimeoutSecs) = 0; 00532 00533 // Isolate OS timers 00534 virtual int getLogTimerTicks(void) = 0; 00535 virtual void startTimerA(void) = 0; 00536 virtual void stopTimerA(void) = 0; 00537 virtual int getTimerTicksA_mS(void) = 0; 00538 virtual void startTimerB(void) = 0; 00539 virtual void stopTimerB(void) = 0; 00540 virtual int getTimerTicksB_mS(void) = 0; 00541 00542 private: 00543 00544 bool softwareInitMdm(void); 00545 bool checkCellLink(void); 00546 AtCmdErr_e mdmSendAtCmdRsp(const char * cmd, int timeout_ms, string * rsp, bool crLf = true); 00547 size_t mdmGetline(string * buff, int timeout_ms); 00548 bool at_at_wnc(void); 00549 bool at_init_wnc(bool hardReset = false); 00550 int16_t at_sockopen_wnc(const char * const ip, uint16_t port, uint16_t numSock, bool tcp, uint16_t timeOutSec); 00551 bool at_sockclose_wnc(uint16_t numSock); 00552 bool at_dnsresolve_wnc(const char * s, string * ipStr); 00553 AtCmdErr_e at_sockwrite_wnc(const uint8_t * s, uint16_t n, uint16_t numSock, bool isTcp); 00554 AtCmdErr_e at_sockread_wnc(uint8_t * pS, uint16_t * numRead, uint16_t n, uint16_t numSock, bool isTcp); 00555 AtCmdErr_e at_sockread_wnc(string * pS, uint16_t numSock, bool isTcp); 00556 bool at_reinitialize_mdm(void); 00557 AtCmdErr_e at_send_wnc_cmd(const char * s, string ** r, int ms_timeout); 00558 bool at_setapn_wnc(const char * const apnStr); 00559 bool at_sendSMStext_wnc(const char * const phoneNum, const char * const text); 00560 bool at_get_wnc_net_stats(WncIpStats * s); 00561 bool at_readSMSlog_wnc(string ** log); 00562 size_t at_readSMStext_wnc(const char ** log); 00563 size_t at_readSMStext_wnc(const char n, const char ** log); 00564 bool at_getrssiber_wnc(int16_t * dBm, int16_t * ber3g); 00565 void closeOpenSocket(uint16_t numSock); 00566 bool sockWrite(const uint8_t * const s, uint16_t n, uint16_t numSock, bool isTcp); 00567 bool at_sendSMStextMem_wnc(char n); 00568 bool at_deleteSMSTextFromMem_wnc(char n); 00569 bool at_saveSMStext_wnc(const char * const phoneNum, const char * const text, char * msgIdx); 00570 size_t at_getSignalQuality_wnc(const char ** log); 00571 bool at_gettimedate_wnc(struct WncDateTime * tod); 00572 bool at_ping_wnc(const char * ip); 00573 bool at_geticcid_wnc(string * iccid); 00574 00575 // Utility methods 00576 void sendCmd(const char * cmd, bool crLf); 00577 void sendCmd(const char * cmd, unsigned n, unsigned wait_uS, bool crLf); 00578 inline void rx_char_wait(void) { 00579 // waitUs(1000); 00580 } 00581 00582 // Important constants 00583 static const uint16_t MAX_WNC_READ_BYTES = 1500; // This bounds the largest amount of data that the WNC read from a socket will return 00584 static const uint16_t MAX_WNC_WRITE_BYTES = MAX_WNC_READ_BYTES; // This is the largest amount of data that the WNC can write per sockwrite. 00585 static const uint16_t MAX_LEN_WNC_CMD_RESPONSE = (MAX_WNC_READ_BYTES * 2 + 100); // Max number of text characters in a WNC AT response *2 because bytes are converted into 2 hex-digits +100 for other AT@ chars. 00586 static const uint16_t WNC_AUTO_POLL_MS = 250; // Sets default (may be overriden with method) poll interval (currently not used, future possible feature. 00587 static const uint16_t WNC_CMD_TIMEOUT_MS = 40000; // Sets default (may be overriden) time that the software waits for an AT response from the WNC. 00588 static const uint16_t WNC_QUICK_CMD_TIMEOUT_MS = 2000; // Used for simple commands that should immediately respond such as "AT", cmds that are quicker than WNC_CMD_TIMEOUT_MS. 00589 static const uint16_t WNC_WAIT_FOR_AT_CMD_MS = 0; // Wait this much between multiple in a row AT commands to the WNC. 00590 static const uint16_t WNC_SOFT_INIT_RETRY_COUNT = 10; // How many times the WNC will be tried to revive if it stops responding. 00591 static const uint16_t WNC_DNS_RESOLVE_WAIT_MS = 60000; // How much time to wait for the WNC to respond to a DNS resolve/lookup. 00592 static const uint16_t WNC_TRUNC_DEBUG_LENGTH = 80; // Always make this an even number, how many chars for the debug output before shortening the debug ouput, this is used when moreDebug = false. 00593 static const uint16_t WNC_APNSET_TIMEOUT_MS = 60000; // How long to wait for the WNC to respond to setting the APN string. 00594 static const uint16_t WNC_PING_CMD_TIMEOUT_MS = 60000; // Amount of time to wait for the WNC to respond to AT@PINGREQ (with cmd default params for timeout, does not change WNC cmd's timeout) 00595 static const int WNC_REINIT_MAX_TIME_MS = 60000; // How long to wait for the WNC to reset after it was already up and running after power-up. 00596 static const uint16_t WNC_SOCK_CLOSE_RETRY_CNT = 3; // How many times to try to close the socket if the WNC gives an error. 00597 static const char * const INVALID_IP_STR; // Just a string set to an IP address when DNS resolve fails. 00598 00599 struct WncSocketInfo_s { 00600 int16_t numWncSock; 00601 bool open; 00602 string myIpAddressStr; 00603 uint16_t myPort; 00604 uint8_t readRetries; 00605 uint16_t readRetryWaitMs; 00606 bool isTcp; 00607 uint16_t timeOutSec; 00608 }; 00609 00610 static WncSocketInfo_s m_sSock[MAX_NUM_WNC_SOCKETS]; 00611 static const WncSocketInfo_s defaultSockStruct; 00612 static WncState_e m_sState; 00613 static uint16_t m_sCmdTimeoutMs; 00614 static string m_sApnStr; 00615 static string m_sWncStr; 00616 static uint8_t m_sPowerUpTimeoutSecs; 00617 static bool m_sDebugEnabled; 00618 static bool m_sMoreDebugEnabled; 00619 static bool m_sCheckNetStatus; 00620 static bool m_sReadyForSMS; 00621 }; 00622 00623 }; // End namespace WncController_fk 00624 00625 #endif 00626
Generated on Sat Jul 16 2022 16:26:31 by
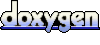