
Sakarya chaotic attractor - uVGAII(SGC) 4DGL version 640x480 pin p9 - rx, pin p10 - tx, pin p11 - rst
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Serial _cmd (p9, p10); 00004 DigitalOut _rst (p11); 00005 00006 void startVGA(); 00007 void baudrate(); 00008 void cls(); 00009 void pixel(int, int, int); 00010 int writeCOM(char *, int); 00011 00012 00013 int main() { 00014 00015 startVGA (); 00016 00017 float x = 1; 00018 float y = -1; 00019 float z = 1; 00020 00021 float ix = 0; 00022 float iy = 0; 00023 float iz = 0; 00024 00025 float a = 0.4; 00026 float b = 0.3; 00027 00028 float dt = 0.001; 00029 00030 while (1) { 00031 00032 ix = x+dt*(-x+y+(y*z)); 00033 iy = y+dt*(-x-y+a*(x*z)); 00034 iz = z+dt*(z-b*(x*y)); 00035 00036 pixel(320+(10*ix),240+(13*iy),65534); 00037 00038 x = ix; 00039 y = iy; 00040 z = iz; 00041 00042 } 00043 } 00044 00045 00046 void startVGA () { 00047 00048 _rst = 1; 00049 _rst = 0; 00050 wait_ms(1); 00051 _rst = 1; 00052 wait(3); 00053 00054 while (_cmd.readable()) _cmd.getc(); 00055 00056 char autobaud[1] = ""; 00057 autobaud[0] = '\x55'; 00058 writeCOM(autobaud, 1); 00059 00060 baudrate(); 00061 00062 cls(); 00063 00064 char dispctr[3]= ""; 00065 dispctr[0] = '\x59'; 00066 dispctr[1] = 0x0c; 00067 dispctr[2] = 0x01; 00068 writeCOM(dispctr, 3); 00069 00070 } 00071 00072 00073 void pixel(int x, int y, int color) { 00074 00075 char pixel[7]= ""; 00076 00077 pixel[0] = '\x50'; 00078 00079 pixel[1] = (x >> 8) & 0xFF; 00080 pixel[2] = x & 0xFF; 00081 00082 pixel[3] = (y >> 8) & 0xFF; 00083 pixel[4] = y & 0xFF; 00084 00085 pixel[5] = (color >> 8) & 0xFF; 00086 pixel[6] = color & 0xFF; 00087 00088 writeCOM(pixel, 7); 00089 } 00090 00091 00092 void baudrate() { 00093 00094 char baudrate[2]= ""; 00095 00096 baudrate[0] = '\x51'; 00097 baudrate[1] = '\x0F'; 00098 00099 int i, resp = 0; 00100 00101 while (_cmd.readable()) _cmd.getc(); 00102 00103 long speed = speed = 281000; 00104 00105 for (i = 0; i <2; i++) _cmd.putc(baudrate[i]); 00106 _cmd.baud(speed); 00107 00108 while (!_cmd.readable()) wait_ms(1); 00109 00110 if (_cmd.readable()) resp = _cmd.getc(); 00111 switch (resp) { 00112 case '\x06' : 00113 resp = 1; 00114 break; 00115 case '\x15' : 00116 resp = -1; 00117 break; 00118 default : 00119 resp = 0; 00120 break; 00121 } 00122 00123 } 00124 00125 00126 int writeCOM(char *command, int number) { 00127 00128 int i, resp = 0; 00129 00130 while (_cmd.readable()) _cmd.getc(); 00131 00132 for (i = 0; i < number; i++) _cmd.putc(command[i]); 00133 00134 while (!_cmd.readable()) wait_ms(1); 00135 if (_cmd.readable()) resp = _cmd.getc(); 00136 switch (resp) { 00137 case '\x06' : 00138 resp = 1; 00139 break; 00140 case '\x15' : 00141 resp = -1; 00142 break; 00143 default : 00144 resp = 0; 00145 break; 00146 } 00147 00148 return resp; 00149 } 00150 00151 00152 void cls() { 00153 char cls[1] = ""; 00154 cls[0] = '\x45'; 00155 writeCOM(cls, 1); 00156 }
Generated on Thu Jul 14 2022 06:01:41 by
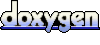