
Chua chaotic oscillator and MCP4922 DAC
Embed:
(wiki syntax)
Show/hide line numbers
MCP4922.cpp
00001 /* 00002 * MCP4922 - DAC library. 00003 */ 00004 00005 #include "mbed.h" 00006 #include "MCP4922.h" 00007 00008 using namespace mbed; 00009 00010 int dac =0; 00011 00012 MCP4922::MCP4922(PinName mosi, PinName sclk, PinName cs) : _spi(mosi, NC, sclk) { 00013 00014 int i; 00015 _ndacs = 1; 00016 _ncs_array = new DigitalOut*[ _ndacs ]; 00017 for (i=0; i<_ndacs; i++) { 00018 _ncs_array[i] = new DigitalOut(cs); 00019 } 00020 00021 // Initialise the DAC SPI interface. 00022 _init(); 00023 } 00024 00025 // Destructor 00026 MCP4922::~MCP4922() { 00027 00028 // Before destroying the object, shut down all the chips. 00029 //shdn_all(); 00030 00031 // Delete all the NCS DigitalOut objects and the array pointing to 00032 // them. 00033 int i; 00034 for (i=0; i<_ndacs; i++) { 00035 delete _ncs_array[i]; 00036 } 00037 delete [] _ncs_array; 00038 00039 // Delete the LDAC DigitalOut object if it exists. 00040 if (_latched ) delete _nldac; 00041 } 00042 00043 // Initialise SPI interface. 00044 void MCP4922::_init() { 00045 00046 // Set up the SPI for 16-bit values (12-bit + 4 command bits) and mode 0. 00047 _spi.format(16, 0); 00048 00049 // Start with all the CS and LDAC signals high (disabled) 00050 int i; 00051 for (i=0; i<_ndacs; i++) { 00052 _ncs_array[i]->write(1); 00053 } 00054 00055 if (_latched ) _nldac->write(1); 00056 return; 00057 } 00058 00059 // Set SPI clock frequency. 00060 void MCP4922::frequency( int freq ) { 00061 00062 // Set the SPI interface clock frequency in Hz. 00063 _spi.frequency( freq ); 00064 return; 00065 } 00066 00067 /* 00068 * Note: There is a lot of code in common between the following 4 functions. 00069 * The code is kept in line to keep it efficient. Could the functions have 00070 * been written as templates? 00071 */ 00072 // Write to DAC channel A with gain 1. 00073 void MCP4922::writeA(int value) { 00074 00075 // Set up the command register with the appropriate value. 00076 // For efficiency, the caller is assumed to have checked dac. 00077 int reg; 00078 //int dac = 0; 00079 reg = (value & 0x0FFF) | MCP4922_REG_A1; 00080 00081 // Select the DAC chip, write to its command register and 00082 // then unselect the DAC chip. 00083 _ncs_array[dac]->write(0); 00084 _spi.write(reg); 00085 _ncs_array[dac]->write(1); 00086 return; 00087 } 00088 00089 // Write to DAC channel B with gain 1. 00090 void MCP4922::writeB(int value) { 00091 00092 // Set up the command register with the appropriate value. 00093 // For efficiency, the caller is assumed to have checked dac. 00094 int reg; 00095 reg = (value & 0x0FFF) | MCP4922_REG_B1; 00096 00097 // Select the DAC chip, write to its command register and then 00098 // unselect the DAC chip. 00099 _ncs_array[dac]->write(0); 00100 _spi.write(reg); 00101 _ncs_array[dac]->write(1); 00102 return; 00103 } 00104 00105 // Write an array of values to the DACs. 00106 void MCP4922::write(int nchans, int values[], int gain, int latch) { 00107 00108 // nchans must be at least 1 but less than or equal to ndacs x 2. 00109 if (nchans < 1) nchans = 1; 00110 const int maxchans = _ndacs * 2; 00111 if (nchans > maxchans) nchans = maxchans; 00112 00113 if (latch && _latched) 00114 latch_disable(); 00115 00116 int i; 00117 00118 for (i=0; i<nchans;) { 00119 dac = i/2; 00120 writeA(values[i]); 00121 i++; 00122 if (i < nchans) { 00123 writeB(values[i]); 00124 i++; 00125 } else break; 00126 } 00127 00128 // Automatically latch the new voltages if the latch flag is 1. 00129 if (latch && _latched) 00130 latch_enable(); 00131 return; 00132 } 00133 00134 // Set latch signal to "enable". 00135 void MCP4922::latch_enable() { 00136 00137 // Latch all chips. There should be a delay of at least T_LS=40 00138 // nanoseconds between the last CS rising edge and the LDAC falling 00139 // edge. The software function calls seem to be sufficient to 00140 // introduce that delay. A delay may be inserted here if this 00141 // software is ported to a faster processor. 00142 if (_latched) _nldac->write(0); 00143 // The LDAC pulse width must be at least T_LD=100 nanoseconds long. 00144 // A delay can be inserted here if necessary, but so far this has 00145 // not been needed (see above). 00146 return; 00147 } 00148 00149 // Set latch signal to "disable". 00150 void MCP4922::latch_disable() { 00151 00152 // Disable latch for all chips. 00153 if (_latched) _nldac->write(1); 00154 return; 00155 } 00156
Generated on Tue Jul 12 2022 21:11:13 by
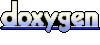