
Modified Celikovsky chaotic system - II
Embed:
(wiki syntax)
Show/hide line numbers
TFT_4DGL_Text.cpp
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "TFT_4DGL.h" 00021 00022 //**************************************************************************************************** 00023 void TFT_4DGL :: set_font(char mode) { // set font size 00024 char command[2]= ""; 00025 00026 int w, h, fx = 8, fy = 8; 00027 00028 command[0] = SETFONT; 00029 command[1] = mode; 00030 00031 current_font = mode; 00032 00033 if (current_orientation == IS_PORTRAIT) { 00034 w = SIZE_X; 00035 h = SIZE_Y; 00036 } else { 00037 w = SIZE_Y; 00038 h = SIZE_X; 00039 } 00040 00041 switch (mode) { 00042 case FONT_5X7 : 00043 fx = 6; 00044 fy = 8; 00045 break; 00046 case FONT_8X8 : 00047 fx = 8; 00048 fy = 8; 00049 break; 00050 case FONT_8X12 : 00051 fx = 8; 00052 fy = 12; 00053 break; 00054 case FONT_12X16 : 00055 fx = 12; 00056 fy = 16; 00057 break; 00058 } 00059 00060 max_col = w / fx; 00061 max_row = h / fy; 00062 00063 writeCOMMAND(command, 2); 00064 } 00065 00066 //**************************************************************************************************** 00067 void TFT_4DGL :: text_mode(char mode) { // set text mode 00068 char command[2]= ""; 00069 00070 command[0] = TEXTMODE; 00071 command[1] = mode; 00072 00073 writeCOMMAND(command, 2); 00074 } 00075 00076 //**************************************************************************************************** 00077 void TFT_4DGL :: text_char(char c, char col, char row, int color) { // draw a text char 00078 char command[6]= ""; 00079 00080 command[0] = TEXTCHAR; 00081 00082 command[1] = c; 00083 command[2] = col; 00084 command[3] = row; 00085 00086 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00087 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00088 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00089 00090 command[4] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00091 command[5] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00092 00093 writeCOMMAND(command, 8); 00094 } 00095 00096 //**************************************************************************************************** 00097 void TFT_4DGL :: graphic_char(char c, int x, int y, int color, char width, char height) { // draw a graphic char 00098 char command[10]= ""; 00099 00100 command[0] = GRAPHCHAR; 00101 00102 command[1] = c; 00103 00104 command[2] = (x >> 8) & 0xFF; 00105 command[3] = x & 0xFF; 00106 00107 command[4] = (y >> 8) & 0xFF; 00108 command[5] = y & 0xFF; 00109 00110 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00111 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00112 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00113 00114 command[6] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00115 command[7] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00116 00117 command[8] = width; 00118 00119 command[9] = height; 00120 00121 writeCOMMAND(command, 10); 00122 } 00123 00124 //**************************************************************************************************** 00125 void TFT_4DGL :: text_string(char *s, char col, char row, char font, int color) { // draw a text string 00126 00127 char command[1000]= ""; 00128 int size = strlen(s); 00129 int i = 0; 00130 00131 command[0] = TEXTSTRING; 00132 00133 command[1] = col; 00134 command[2] = row; 00135 00136 command[3] = font; 00137 00138 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00139 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00140 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00141 00142 command[4] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00143 command[5] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00144 00145 for (i=0; i<size; i++) command[6+i] = s[i]; 00146 00147 command[6+size] = 0; 00148 00149 writeCOMMAND(command, 7 + size); 00150 } 00151 00152 //**************************************************************************************************** 00153 void TFT_4DGL :: graphic_string(char *s, int x, int y, char font, int color, char width, char height) { // draw a text string 00154 00155 char command[1000]= ""; 00156 int size = strlen(s); 00157 int i = 0; 00158 00159 command[0] = GRAPHSTRING; 00160 00161 command[1] = (x >> 8) & 0xFF; 00162 command[2] = x & 0xFF; 00163 00164 command[3] = (y >> 8) & 0xFF; 00165 command[4] = y & 0xFF; 00166 00167 command[5] = font; 00168 00169 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00170 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00171 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00172 00173 command[6] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00174 command[7] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00175 00176 command[8] = width; 00177 00178 command[9] = height; 00179 00180 for (i=0; i<size; i++) command[10+i] = s[i]; 00181 00182 command[10+size] = 0; 00183 00184 writeCOMMAND(command, 11 + size); 00185 } 00186 00187 //**************************************************************************************************** 00188 void TFT_4DGL :: text_button(char *s, char mode, int x, int y, int button_color, char font, int text_color, char width, char height) { // draw a text string 00189 00190 char command[1000]= ""; 00191 int size = strlen(s); 00192 int i = 0, red5, green6, blue5; 00193 00194 command[0] = TEXTBUTTON; 00195 00196 command[1] = mode; 00197 00198 command[2] = (x >> 8) & 0xFF; 00199 command[3] = x & 0xFF; 00200 00201 command[4] = (y >> 8) & 0xFF; 00202 command[5] = y & 0xFF; 00203 00204 red5 = (button_color >> (16 + 3)) & 0x1F; // get red on 5 bits 00205 green6 = (button_color >> (8 + 2)) & 0x3F; // get green on 6 bits 00206 blue5 = (button_color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00207 00208 command[6] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00209 command[7] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00210 00211 command[8] = font; 00212 00213 red5 = (text_color >> (16 + 3)) & 0x1F; // get red on 5 bits 00214 green6 = (text_color >> (8 + 2)) & 0x3F; // get green on 6 bits 00215 blue5 = (text_color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00216 00217 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00218 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00219 00220 command[11] = width; 00221 00222 command[12] = height; 00223 00224 for (i=0; i<size; i++) command[13+i] = s[i]; 00225 00226 command[13+size] = 0; 00227 00228 writeCOMMAND(command, 14 + size); 00229 } 00230 00231 //**************************************************************************************************** 00232 void TFT_4DGL :: locate(char col, char row) { // place text curssor at col, row 00233 current_col = col; 00234 current_row = row; 00235 } 00236 00237 //**************************************************************************************************** 00238 void TFT_4DGL :: color(int color) { // set text color 00239 current_color = color; 00240 } 00241 00242 //**************************************************************************************************** 00243 void TFT_4DGL :: putc(char c) { // place char at current cursor position 00244 00245 text_char(c, current_col++, current_row, current_color); 00246 00247 if (current_col == max_col) { 00248 current_col = 0; 00249 current_row++; 00250 } 00251 if (current_row == max_row) { 00252 current_row = 0; 00253 } 00254 } 00255 00256 //**************************************************************************************************** 00257 void TFT_4DGL :: puts(char *s) { // place string at current cursor position 00258 00259 text_string(s, current_col, current_row, current_font, current_color); 00260 00261 current_col += strlen(s); 00262 00263 if (current_col >= max_col) { 00264 current_row += current_col / max_col; 00265 current_col %= max_col; 00266 } 00267 if (current_row >= max_row) { 00268 current_row %= max_row; 00269 } 00270 }
Generated on Thu Jul 21 2022 23:01:50 by
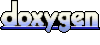