
Modified Celikovsky chaotic system - II
Embed:
(wiki syntax)
Show/hide line numbers
TFT_4DGL_Graphics.cpp
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "TFT_4DGL.h" 00021 00022 00023 //**************************************************************************************************** 00024 void TFT_4DGL :: circle(int x, int y , int radius, int color) { // draw a circle in (x,y) 00025 char command[9]= ""; 00026 00027 command[0] = CIRCLE; 00028 00029 command[1] = (x >> 8) & 0xFF; 00030 command[2] = x & 0xFF; 00031 00032 command[3] = (y >> 8) & 0xFF; 00033 command[4] = y & 0xFF; 00034 00035 command[5] = (radius >> 8) & 0xFF; 00036 command[6] = radius & 0xFF; 00037 00038 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00039 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00040 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00041 00042 command[7] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00043 command[8] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00044 00045 writeCOMMAND(command, 9); 00046 } 00047 00048 //**************************************************************************************************** 00049 void TFT_4DGL :: triangle(int x1, int y1 , int x2, int y2, int x3, int y3, int color) { // draw a traingle 00050 char command[15]= ""; 00051 00052 command[0] = TRIANGLE; 00053 00054 command[1] = (x1 >> 8) & 0xFF; 00055 command[2] = x1 & 0xFF; 00056 00057 command[3] = (y1 >> 8) & 0xFF; 00058 command[4] = y1 & 0xFF; 00059 00060 command[5] = (x2 >> 8) & 0xFF; 00061 command[6] = x2 & 0xFF; 00062 00063 command[7] = (y2 >> 8) & 0xFF; 00064 command[8] = y2 & 0xFF; 00065 00066 command[9] = (x3 >> 8) & 0xFF; 00067 command[10] = x3 & 0xFF; 00068 00069 command[11] = (y3 >> 8) & 0xFF; 00070 command[12] = y3 & 0xFF; 00071 00072 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00073 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00074 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00075 00076 command[13] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00077 command[14] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00078 00079 writeCOMMAND(command, 15); 00080 } 00081 00082 //**************************************************************************************************** 00083 void TFT_4DGL :: line(int x1, int y1 , int x2, int y2, int color) { // draw a line 00084 char command[11]= ""; 00085 00086 command[0] = LINE; 00087 00088 command[1] = (x1 >> 8) & 0xFF; 00089 command[2] = x1 & 0xFF; 00090 00091 command[3] = (y1 >> 8) & 0xFF; 00092 command[4] = y1 & 0xFF; 00093 00094 command[5] = (x2 >> 8) & 0xFF; 00095 command[6] = x2 & 0xFF; 00096 00097 command[7] = (y2 >> 8) & 0xFF; 00098 command[8] = y2 & 0xFF; 00099 00100 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00101 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00102 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00103 00104 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00105 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00106 00107 writeCOMMAND(command, 11); 00108 } 00109 00110 //**************************************************************************************************** 00111 void TFT_4DGL :: rectangle(int x1, int y1 , int x2, int y2, int color) { // draw a rectangle 00112 char command[11]= ""; 00113 00114 command[0] = RECTANGLE; 00115 00116 command[1] = (x1 >> 8) & 0xFF; 00117 command[2] = x1 & 0xFF; 00118 00119 command[3] = (y1 >> 8) & 0xFF; 00120 command[4] = y1 & 0xFF; 00121 00122 command[5] = (x2 >> 8) & 0xFF; 00123 command[6] = x2 & 0xFF; 00124 00125 command[7] = (y2 >> 8) & 0xFF; 00126 command[8] = y2 & 0xFF; 00127 00128 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00129 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00130 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00131 00132 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00133 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00134 00135 writeCOMMAND(command, 11); 00136 } 00137 00138 //**************************************************************************************************** 00139 void TFT_4DGL :: ellipse(int x, int y , int radius_x, int radius_y, int color) { // draw an ellipse 00140 char command[11]= ""; 00141 00142 command[0] = ELLIPSE; 00143 00144 command[1] = (x >> 8) & 0xFF; 00145 command[2] = x & 0xFF; 00146 00147 command[3] = (y >> 8) & 0xFF; 00148 command[4] = y & 0xFF; 00149 00150 command[5] = (radius_x >> 8) & 0xFF; 00151 command[6] = radius_x & 0xFF; 00152 00153 command[7] = (radius_y >> 8) & 0xFF; 00154 command[8] = radius_y & 0xFF; 00155 00156 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00157 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00158 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00159 00160 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00161 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00162 00163 writeCOMMAND(command, 11); 00164 } 00165 00166 //**************************************************************************************************** 00167 void TFT_4DGL :: pixel(int x, int y, int color) { // draw a pixel 00168 char command[7]= ""; 00169 00170 command[0] = PIXEL; 00171 00172 command[1] = (x >> 8) & 0xFF; 00173 command[2] = x & 0xFF; 00174 00175 command[3] = (y >> 8) & 0xFF; 00176 command[4] = y & 0xFF; 00177 00178 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00179 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00180 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00181 00182 command[5] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00183 command[6] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00184 00185 writeCOMMAND(command, 7); 00186 } 00187 00188 //****************************************************************************************************** 00189 int TFT_4DGL :: read_pixel(int x, int y) { // read screen info and populate data 00190 00191 char command[5]= ""; 00192 00193 command[0] = READPIXEL; 00194 00195 command[1] = (x >> 8) & 0xFF; 00196 command[2] = x & 0xFF; 00197 00198 command[3] = (y >> 8) & 0xFF; 00199 command[4] = y & 0xFF; 00200 00201 int i, temp = 0, color = 0, resp = 0; 00202 char response[2] = ""; 00203 00204 freeBUFFER(); 00205 00206 for (i = 0; i < 5; i++) { // send all chars to serial port 00207 writeBYTE(command[i]); 00208 } 00209 00210 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00211 00212 while (_cmd.readable()) { 00213 temp = _cmd.getc(); 00214 response[resp++] = (char)temp; 00215 } 00216 00217 color = ((response[0] << 8) + response[1]); 00218 00219 return color; // WARNING : this is 16bits color, not 24bits... need to be fixed 00220 } 00221 00222 //****************************************************************************************************** 00223 void TFT_4DGL :: screen_copy(int xs, int ys , int xd, int yd , int width, int height) { 00224 00225 char command[13]= ""; 00226 00227 command[0] = SCREENCOPY; 00228 00229 command[1] = (xs >> 8) & 0xFF; 00230 command[2] = xs & 0xFF; 00231 00232 command[3] = (ys >> 8) & 0xFF; 00233 command[4] = ys & 0xFF; 00234 00235 command[5] = (xd >> 8) & 0xFF; 00236 command[6] = xd & 0xFF; 00237 00238 command[7] = (yd >> 8) & 0xFF; 00239 command[8] = yd & 0xFF; 00240 00241 command[9] = (width >> 8) & 0xFF; 00242 command[10] = width & 0xFF; 00243 00244 command[11] = (height >> 8) & 0xFF; 00245 command[12] = height & 0xFF; 00246 00247 writeCOMMAND(command, 13); 00248 } 00249 00250 //**************************************************************************************************** 00251 void TFT_4DGL :: pen_size(char mode) { // set pen to SOLID or WIREFRAME 00252 char command[2]= ""; 00253 00254 command[0] = PENSIZE; 00255 command[1] = mode; 00256 00257 writeCOMMAND(command, 2); 00258 } 00259 00260 //**************************************************************************************************** 00261 // This plays a .wav file from the FAT partition of an uSD Card 00262 // Not really recommended as the speaker on the uLCD-32PT is really bad! 00263 //**************************************************************************************************** 00264 void TFT_4DGL :: SD_Card_Wav(char *Filename) { 00265 char command[21]= ""; 00266 int Lgt=0; 00267 00268 command[0] = 0x40; //ext_cmd 00269 command[1] = 0x6C; //Play Audio 00270 command[2] = 0x01; //Option 0x00=Return at end, 0x01=Return now, 0x02=Stop, 0x03=Pause, 0x04=Resume, 0x05=Loop. 00271 for(int i=0;Filename[i]!=0x00;i++){ 00272 command[i+3] = Filename[i]; 00273 Lgt = i; 00274 } 00275 command[Lgt+4] = 0x2E; //. 00276 command[Lgt+5] = 0x77; //w 00277 command[Lgt+6] = 0x61; //a 00278 command[Lgt+7] = 0x76; //v 00279 command[Lgt+8] = 0x00; //terminator 00280 00281 writeCOMMAND(command, Lgt+9); 00282 } 00283 00284 //**************************************************************************************************** 00285 // This sets the volume for the speaker on the uLCD-32PT 00286 //**************************************************************************************************** 00287 void TFT_4DGL :: Set_Volume(char vol) { 00288 00289 char command[2]= ""; 00290 00291 command[0] = 0x76; //cmd 00292 command[1] = vol; //set volume 00293 00294 writeCOMMAND(command, 2); 00295 } 00296 00297 //**************************************************************************************************** 00298 // This displays an image on the screen that is stored on the FAT partition of an uSD Card 00299 // Sent Filename, X-pos, Y-pos, Sector Address - Display from the RAW partition is quicker 00300 //**************************************************************************************************** 00301 void TFT_4DGL :: uSD_FAT_Image(char *Filename, int x, int y, long s) { 00302 char X_MSB, X_LSB, Y_MSB, Y_LSB, S0, S1, S2, S3; 00303 char command[25]= ""; 00304 int Lgt=0; 00305 00306 X_LSB = x&0x00FF; 00307 X_MSB = (x >> 8); 00308 Y_LSB = y&0x00FF; 00309 Y_MSB = (y >> 8); 00310 00311 S0 = (s >> 20)&0x000000FF; 00312 S1 = (s >> 16)&0x0000FF; 00313 S2 = (s >> 8)&0x0000FF; 00314 S3 = s&0x0000FF;; 00315 00316 command[0] = '@'; //ext_cmd 00317 command[1] = 'm'; //FAT Image 00318 for(int i=0;Filename[i]!=0x00;i++){ 00319 command[i+2] = Filename[i]; 00320 Lgt = i; 00321 } 00322 command[Lgt+3] = '.'; //. 00323 command[Lgt+4] = 'G'; //G 00324 command[Lgt+5] = 'C'; //C 00325 command[Lgt+6] = 'I'; //I 00326 command[Lgt+7] = 0x00; //Terminator 00327 command[Lgt+8] = X_MSB; //X-Position MSB 00328 command[Lgt+9] = X_LSB; //X-Position LSB 00329 command[Lgt+10] = Y_MSB; //Y-Position MSB 00330 command[Lgt+11] = Y_LSB; //Y-Position LSB 00331 command[Lgt+12] = S0; //Sector Address 4 bytes 00332 command[Lgt+13] = S1; 00333 command[Lgt+14] = S2; 00334 command[Lgt+15] = S3; 00335 00336 writeCOMMAND(command, Lgt+16); 00337 } 00338 00339 //**************************************************************************************************** 00340 // This displays an image on the screen in the NEW FORMAT 00341 // Sent X-pos, Y-pos, Sector Address - This is the recommended way to display images 00342 //**************************************************************************************************** 00343 void TFT_4DGL :: uSD_Image(int x, int y, long s) { 00344 char S1, S2, S3; 00345 char X_MSB, X_LSB, Y_MSB, Y_LSB; 00346 char command[9]= ""; 00347 00348 X_LSB = x&0x00FF; //Work out the x position 00349 X_MSB = (x >> 8); 00350 Y_LSB = y&0x00FF; //Work out the y position 00351 Y_MSB = (y >> 8); 00352 00353 S1 = (s >> 16)&0x0000FF; //Work out the sector address 00354 S2 = (s >> 8)&0x0000FF; 00355 S3 = s&0x0000FF; 00356 00357 command[0] = 0x40; //ext_cmd 00358 command[1] = 0x49; //Display image 00359 command[2] = X_MSB; //X position - 2 bytes 00360 command[3] = X_LSB; 00361 command[4] = Y_MSB; //Y position - 2 bytes 00362 command[5] = Y_LSB; 00363 command[6] = S1; //Sector address - 3 bytes 00364 command[7] = S2; 00365 command[8] = S3; 00366 00367 writeCOMMAND(command, 9); 00368 } 00369 00370 //**************************************************************************************************** 00371 // This displays an video on the screen in the NEW FORMAT 00372 // Sent X-pos, Y-pos, Sector Address - This is the recommended way to display video 00373 //**************************************************************************************************** 00374 void TFT_4DGL :: uSD_Video(int x, int y, long s) { 00375 char S1, S2, S3; 00376 char X_MSB, X_LSB, Y_MSB, Y_LSB; 00377 char command[10]= ""; 00378 00379 X_LSB = x&0x00FF; 00380 X_MSB = (x >> 8); 00381 Y_LSB = y&0x00FF; 00382 Y_MSB = (y >> 8); 00383 00384 S1 = (s >> 16)&0x0000FF; 00385 S2 = (s >> 8)&0x0000FF; 00386 S3 = s&0x0000FF; 00387 00388 command[0] = 0x40; //ext_cmd 00389 command[1] = 0x56; //Display video 00390 command[2] = X_MSB; //X position - 2 bytes 00391 command[3] = X_LSB; 00392 command[4] = Y_MSB; //Y position - 2 bytes 00393 command[5] = Y_LSB; 00394 command[6] = 0x00; //delay between frames 00395 command[7] = S1; //Sector address - 3 bytes 00396 command[8] = S2; 00397 command[9] = S3; 00398 00399 writeCOMMAND(command, 10); 00400 }
Generated on Thu Jul 21 2022 23:01:50 by
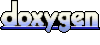