
The MBED firmware used on the Chipin sorter, developed over 12 weeks for a 3rd year university systems project. Chipin is a token sorter, it sorts tokens by colours and dispenses them to order through an online booking system and card reader. This program interfaces with an FPGA, PC and LCD screen to control the sorter. The sorter has an operation mode where it can process orders when a card is entered into the machine. There is also a maintenance mode where the device responds to maintenance instructions such as 'dispense all'. More information at http://www.ionsystems.uk/
Dependencies: MCP23017 TCS3472_I2C WattBob_TextLCD mbed-rtos mbed
serialCommunication.h
00001 #include "cardReader.h" 00002 00003 00004 /* void sendCharacter(char ch) 00005 * Send a character along the serial port to the PC. 00006 */ 00007 void sendCharacter(char ch) 00008 { 00009 pc.putc(ch); 00010 } 00011 00012 /* void sendString(char* ch) 00013 * Send a string along the serial port to the PC. 00014 */ 00015 void sendString(char* ch) 00016 { 00017 pc.puts(ch); 00018 } 00019 /* void processMessage(char c) 00020 * Decide what to do when a character is recieved. 00021 */ 00022 void processMessage(char c) 00023 { 00024 switch(c) { 00025 case 'a' : //Dispense red 00026 dispense(RED); 00027 sendCharacter('A'); //Tell the PC the operation is complete 00028 break; 00029 00030 case 'b' : //dispense green 00031 dispense(GREEN); 00032 sendCharacter('B');//Tell the PC the operation is complete 00033 break; 00034 00035 case 'c': //dispense blue 00036 dispense(BLUE); 00037 sendCharacter('C');//Tell the PC the operation is complete 00038 break; 00039 00040 case 'm':// Read card command 00041 sendCharacter('M'); 00042 int cardNumber = cardAcquisition(); 00043 //Send card number to pc 00044 wait(0.5); 00045 pc.printf("%i\n",cardNumber); 00046 printLCD("Reading Card"); 00047 break; 00048 00049 case 'X': 00050 sendCharacter('X'); //Notify the PC that this is an MBED 00051 printLCD("Connected to PC"); 00052 break; 00053 00054 case 'd': //Sort Bin 00055 sort(BIN); 00056 sendCharacter('D');//Tell the PC the operation is complete 00057 break; 00058 00059 case 'o': //testServoredBlueLeft 00060 maintain(RB_LEFT); 00061 sendCharacter('O');//Tell the PC the operation is complete 00062 break; 00063 00064 case 'p': 00065 maintain(RB_CENTRE); 00066 sendCharacter('P');//Tell the PC the operation is complete 00067 break; 00068 00069 case 'q': 00070 maintain(RB_RIGHT); 00071 sendCharacter('O');//Tell the PC the operation is complete 00072 break; 00073 00074 00075 case 's': 00076 maintain(GO_UP); 00077 sendCharacter('S');//Tell the PC the operation is complete 00078 break; 00079 00080 00081 case 't': 00082 maintain(GO_CENTRE); 00083 sendCharacter('T');//Tell the PC the operation is complete 00084 break; 00085 00086 00087 case 'u': 00088 maintain(GO_DOWN); 00089 sendCharacter('U');//Tell the PC the operation is complete 00090 break; 00091 00092 case 'v': 00093 maintain(BR_LEFT); 00094 sendCharacter('V');//Tell the PC the operation is complete 00095 break; 00096 00097 case 'w': 00098 maintain(BR_RIGHT); 00099 sendCharacter('O');//Tell the PC the operation is complete 00100 break; 00101 00102 case 'j': 00103 sort(RED); 00104 sendCharacter('J');//Tell the PC the operation is complete 00105 break; 00106 00107 case 'k': 00108 sort(GREEN); 00109 sendCharacter('K');//Tell the PC the operation is complete 00110 break; 00111 00112 case 'l': 00113 sort(BLUE); 00114 sendCharacter('L');//Tell the PC the operation is complete 00115 break; 00116 00117 case '0': //Dispense all 00118 dispenseAll(); 00119 sendCharacter('1');//Tell the PC the operation is complete 00120 break; 00121 00122 case '2': 00123 maintain(GO_CENTRE); 00124 maintain(RB_CENTRE); 00125 sendCharacter('3');//Tell the PC the operation is complete 00126 break; 00127 00128 case '4': 00129 lift(); 00130 sendCharacter('5');//Tell the PC the operation is complete 00131 break; 00132 00133 case 'e': 00134 recycle(); 00135 sendCharacter('E');//Tell the PC the operation is complete 00136 break; 00137 } 00138 } 00139 /* If the serial port is readable, get a character and do something, depending on the character recieved. 00140 */ 00141 bool checkSerial() 00142 { 00143 printLCD(""); 00144 if(pc.readable()) { 00145 char c = pc.getc(); 00146 processMessage(c); 00147 return true; 00148 } else { 00149 return false; 00150 } 00151 } 00152 00153 00154 /* Set the PC's and LCD's language to whatever our language is. 00155 */ 00156 void sendLanguageCharacter() 00157 { 00158 switch(currentLanguage) { 00159 case ENGLISH: 00160 pc.putc('Y'); 00161 glcd.putc(27); 00162 glcd.putc(15); 00163 glcd.putc(255); 00164 break; 00165 00166 case FRENCH: 00167 pc.putc('Z'); 00168 glcd.putc(27); 00169 glcd.putc(16); 00170 glcd.putc(255); 00171 break; 00172 00173 case GERMAN: 00174 pc.putc('!'); 00175 glcd.putc(27); 00176 glcd.putc(17); 00177 glcd.putc(255); 00178 break; 00179 } 00180 } 00181 00182
Generated on Thu Jul 14 2022 19:06:18 by
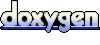