
The MBED firmware used on the Chipin sorter, developed over 12 weeks for a 3rd year university systems project. Chipin is a token sorter, it sorts tokens by colours and dispenses them to order through an online booking system and card reader. This program interfaces with an FPGA, PC and LCD screen to control the sorter. The sorter has an operation mode where it can process orders when a card is entered into the machine. There is also a maintenance mode where the device responds to maintenance instructions such as 'dispense all'. More information at http://www.ionsystems.uk/
Dependencies: MCP23017 TCS3472_I2C WattBob_TextLCD mbed-rtos mbed
mbedStorage.h
00001 #include "mbed.h" 00002 /* Allows easy enable and disable of log file. 00003 * WARNING: Every time a file is changed on the MBED, it reconnects to the PC, 00004 * resulting in hundrets of Autoplay notices appearing on your desktop window. 00005 * Unplug the MBED from the PC for best results. 00006 * Logging is extremely useful for debugging but should be disabled when not debugging. 00007 */ 00008 bool logEnable = false; 00009 LocalFileSystem local("local"); 00010 00011 /* void writeFile(int r, int g, int b, int re) 00012 * Write the values of each storage tube to the datafile. 00013 * Ensures no negative values are written to the file. 00014 */ 00015 void writeFile(int r, int g, int b, int re) 00016 { 00017 FILE* file = fopen("/local/df.txt","w"); // open file 00018 if(r >= 0) { 00019 fputc(r, file); // put char (data value) into file 00020 } else { 00021 fputc(0, file); 00022 } 00023 if(g >= 0) { 00024 fputc(g, file); 00025 } else { 00026 fputc(0, file); 00027 } 00028 if(b >= 0) { 00029 fputc(b, file); 00030 } else { 00031 fputc(0, file); 00032 } 00033 if(re >= 0) { 00034 fputc(re, file); 00035 } else { 00036 fputc(0, file); 00037 } 00038 fclose(file); // close file 00039 } 00040 /* int readFile(int index) 00041 * Read a character from the datafile and return as an integer. 00042 */ 00043 int readFile(int index) 00044 { 00045 FILE* file = fopen ("/local/df.txt","r"); // open file for reading 00046 int read_var = 0; 00047 for(int i = 0; i <= index; i++) { 00048 read_var = fgetc(file); // read data value 00049 } 00050 fclose(file); // close file 00051 return read_var; 00052 } 00053 00054 /* void log(char* text) 00055 * Append a charcater array on a new line in the log file. 00056 */ 00057 void log(char* text) 00058 { 00059 if(logEnable) { 00060 FILE* file = fopen ("/local/log.txt", "a"); 00061 fputs(text, file); 00062 fputs("\r\n", file); 00063 fclose(file); 00064 } 00065 } 00066 /* void clearLog() 00067 * Clear the log file. 00068 */ 00069 void clearLog() 00070 { 00071 FILE* file = fopen ("/local/log.txt", "w"); 00072 fclose(file); 00073 }
Generated on Thu Jul 14 2022 19:06:18 by
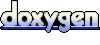