
This sketch read a single byte into a variable, then, the variable is added by two, the result is written into memory. Finally, byte is readed again. the values are printed to serial port too.
Dependencies: Hotboards_eeprom mbed
main.cpp
00001 /* 00002 Hotboards eeprom Library - write_byte 00003 Demonstrates the use a spi eeprom. The Hotboards_eeprom 00004 library works with all Microchip 25xxx serial memory series 00005 like the one presented eeprom board (http://www.hotboards.org). 00006 This sketch read a single byte into a variable, then, the variable is added by two, 00007 the result is written into memory. Finally, byte is readed again. 00008 the values are printed to serial port too. 00009 00010 The circuit: 00011 * VDD --> 3.3v 00012 * SO --> PB_14 00013 * SI --> PB_15 00014 * SCK --> PB_13 00015 * CS --> PC_4 00016 * GND --> GND 00017 00018 Library created by Diego from Hotboards 00019 example added by Pedro from Hotboards 00020 */ 00021 00022 #include "mbed.h" 00023 #include "Hotboards_eeprom.h" 00024 00025 /*Open an instance of serial port*/ 00026 Serial pc(USBTX,USBRX); 00027 /* initialize an instance of SPI bus,setting the SPI pins*/ 00028 SPI device(PB_15,PB_14,PB_13); /* SO, SI, SCK*/ 00029 /*initialize the library with the numbers of the interface pins*/ 00030 Hotboards_eeprom eeprom(device,PC_4,HT_EEPROM25xx_32Kb); /* (spi,Cs,Density) */ 00031 00032 int main() 00033 { 00034 /*SPI transfer at 8 bits, MODE = 3*/ 00035 device.format(8,3); 00036 /* set the spi frequency to 5MHz */ 00037 device.frequency(5000000); 00038 /*initialize CS pin*/ 00039 eeprom.init(); 00040 00041 /* variable to read data*/ 00042 char Data; 00043 00044 /* read firts byte*/ 00045 Data=eeprom.read(0); 00046 /* send to serial port*/ 00047 printf("%X \n",Data); 00048 00049 /* readed data is added by two */ 00050 Data+= 2; 00051 /* write the result*/ 00052 eeprom.write(0,Data); 00053 00054 /* read firts byte again*/ 00055 Data=eeprom.read(0); 00056 /* send to serial port */ 00057 printf("%X \n",Data); 00058 00059 while(1) 00060 { 00061 /*loop*/ 00062 } 00063 00064 }
Generated on Tue Jul 12 2022 19:05:44 by
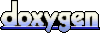