
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
ZXing.h
00001 // -*- mode:c++; tab-width:2; indent-tabs-mode:nil; c-basic-offset:2 -*- 00002 /* 00003 * Copyright 2013 ZXing authors All rights reserved. 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __ZXING_H_ 00018 #define __ZXING_H_ 00019 00020 #define ZXING_ARRAY_LEN(v) ((int)(sizeof(v)/sizeof(v[0]))) 00021 #define ZX_LOG_DIGITS(digits) \ 00022 ((digits == 8) ? 3 : \ 00023 ((digits == 16) ? 4 : \ 00024 ((digits == 32) ? 5 : \ 00025 ((digits == 64) ? 6 : \ 00026 ((digits == 128) ? 7 : \ 00027 (-1)))))) 00028 00029 #ifndef ZXING_DEBUG 00030 #define ZXING_DEBUG 0 00031 #endif 00032 00033 namespace zxing { 00034 typedef char byte; 00035 typedef bool boolean; 00036 } 00037 00038 #include <limits> 00039 00040 #if defined(_WIN32) || defined(_WIN64) 00041 00042 #include <float.h> 00043 00044 namespace zxing { 00045 inline bool isnan(float v) {return _isnan(v) != 0;} 00046 inline bool isnan(double v) {return _isnan(v) != 0;} 00047 inline float nan() {return std::numeric_limits<float>::quiet_NaN();} 00048 } 00049 00050 #else 00051 00052 #include <cmath> 00053 00054 namespace zxing { 00055 #if defined(__ICCARM__) || defined( __CC_ARM ) // 00056 #else // 00057 inline bool isnan(float v) {return std::isnan(v);} 00058 inline bool isnan(double v) {return std::isnan(v);} 00059 #endif // 00060 inline float nan() {return std::numeric_limits<float>::quiet_NaN();} 00061 } 00062 #endif 00063 00064 #if ZXING_DEBUG 00065 00066 #include <iostream> 00067 #include <string> 00068 00069 using std::cout; 00070 using std::cerr; 00071 using std::endl; 00072 using std::flush; 00073 using std::string; 00074 using std::ostream; 00075 00076 #if ZXING_DEBUG_TIMER 00077 00078 #include <sys/time.h> 00079 00080 namespace zxing { 00081 00082 class DebugTimer { 00083 public: 00084 DebugTimer(char const* string_) : chars(string_) { 00085 gettimeofday(&start, 0); 00086 } 00087 00088 DebugTimer(std::string const& string_) : chars(0), string(string_) { 00089 gettimeofday(&start, 0); 00090 } 00091 00092 void mark(char const* string) { 00093 struct timeval end; 00094 gettimeofday(&end, 0); 00095 int diff = 00096 (end.tv_sec - start.tv_sec)*1000*1000+(end.tv_usec - start.tv_usec); 00097 00098 cerr << diff << " " << string << '\n'; 00099 } 00100 00101 void mark(std::string string) { 00102 mark(string.c_str()); 00103 } 00104 00105 ~DebugTimer() { 00106 if (chars) { 00107 mark(chars); 00108 } else { 00109 mark(string.c_str()); 00110 } 00111 } 00112 00113 private: 00114 char const* const chars; 00115 std::string string; 00116 struct timeval start; 00117 }; 00118 00119 } 00120 00121 #define ZXING_TIME(string) DebugTimer __timer__ (string) 00122 #define ZXING_TIME_MARK(string) __timer__.mark(string) 00123 00124 #endif 00125 00126 #endif // ZXING_DEBUG 00127 00128 #ifndef ZXING_TIME 00129 #define ZXING_TIME(string) (void)0 00130 #endif 00131 #ifndef ZXING_TIME_MARK 00132 #define ZXING_TIME_MARK(string) (void)0 00133 #endif 00134 00135 #endif
Generated on Tue Jul 12 2022 18:38:06 by
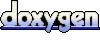