
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
Options.cpp
00001 /** 00002 * @file Options.cpp 00003 * @brief mbed CoAP Options (immutable OptionsBuilder instance) class implementation 00004 * @author Doug Anson/Chris Paola 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 // Class support 00024 #include "mbed-connector-interface/Options.h" 00025 00026 // Utils 00027 #include "mbed-connector-interface/Utils.h" 00028 00029 namespace Connector { 00030 00031 // default constructor 00032 Options::Options() 00033 { 00034 } 00035 00036 // copy constructor 00037 Options::Options(const Options & /* opt */) 00038 { 00039 } 00040 00041 // destructors 00042 Options::~Options() 00043 { 00044 } 00045 00046 // lifetime pointer 00047 int Options::getLifetime() 00048 { 00049 return this->m_lifetime; 00050 } 00051 00052 // NSP domain 00053 string Options::getDomain() 00054 { 00055 return this->m_domain; 00056 } 00057 00058 // Endpoint name 00059 string Options::getEndpointNodename() 00060 { 00061 return this->m_node_name; 00062 } 00063 00064 // Endpoint Type 00065 string Options::getEndpointType() 00066 { 00067 return this->m_endpoint_type; 00068 } 00069 00070 // Connector URL 00071 char *Options::getConnectorURL() 00072 { 00073 return (char *)this->m_connector_url.c_str(); 00074 } 00075 00076 // Connector Port 00077 uint16_t Options::getConnectorPort() 00078 { 00079 return extract_port_from_url(this->getConnectorURL(),DEF_COAP_PORT); 00080 } 00081 00082 // Device Resources Object 00083 void *Options::getDeviceResourcesObject() 00084 { 00085 return this->m_device_resources_object; 00086 } 00087 00088 // Firmware Resources Object 00089 void *Options::getFirmwareResourcesObject() 00090 { 00091 return this->m_firmware_resources_object; 00092 } 00093 00094 // Static Resources 00095 StaticResourcesList *Options::getStaticResourceList() 00096 { 00097 return &this->m_static_resources; 00098 } 00099 00100 // Dynamic Resources 00101 DynamicResourcesList *Options::getDynamicResourceList() 00102 { 00103 return &this->m_dynamic_resources; 00104 } 00105 00106 // WiFi SSID 00107 string Options::getWiFiSSID() { 00108 return this->m_wifi_ssid; 00109 } 00110 00111 // WiFi AuthType 00112 WiFiAuthTypes Options::getWiFiAuthType() { 00113 return this->m_wifi_auth_type; 00114 } 00115 00116 // WiFi AuthKey 00117 string Options::getWiFiAuthKey() { 00118 return this->m_wifi_auth_key; 00119 } 00120 00121 // CoAP Connection Type 00122 CoAPConnectionTypes Options::getCoAPConnectionType() { 00123 return this->m_coap_connection_type; 00124 } 00125 00126 // IP Address Type 00127 IPAddressTypes Options::getIPAddressType() { 00128 return this->m_ip_address_type; 00129 } 00130 00131 // Immediate Observationing Enabled 00132 bool Options::immedateObservationEnabled() { 00133 return this->m_enable_immediate_observation; 00134 } 00135 00136 // Enable/Disable Observation control via GET 00137 bool Options::enableGETObservationControl() { 00138 return this->m_enable_get_obs_control; 00139 } 00140 00141 // Get the Server Certificate 00142 uint8_t *Options::getServerCertificate() { 00143 return this->m_server_cert; 00144 } 00145 00146 // Get the Server Certificate length 00147 int Options::getServerCertificateSize() { 00148 return this->m_server_cert_length; 00149 } 00150 00151 // Get the Client Certificate 00152 uint8_t *Options::getClientCertificate() { 00153 return this->m_client_cert; 00154 } 00155 00156 // Get the Client Certificate length 00157 int Options::getClientCertificateSize() { 00158 return this->m_client_cert_length; 00159 } 00160 00161 // Get the Client Key 00162 uint8_t *Options::getClientKey() { 00163 return this->m_client_key; 00164 } 00165 00166 // Get the Client Key length 00167 int Options::getClientKeySize() { 00168 return this->m_client_key_length; 00169 } 00170 00171 // Get our Endpoint 00172 void *Options::getEndpoint() { 00173 return this->m_endpoint; 00174 } 00175 00176 } // namespace Connector
Generated on Tue Jul 12 2022 18:38:05 by
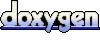