
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
NamedPointer.cpp
00001 /** 00002 * @file NamedPointer.cpp 00003 * @brief mbed CoAP Endpoint Device Management Named Pointer 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2016 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 // Base Class 00024 #include "mbed-connector-interface/ObjectInstanceManager.h" 00025 00026 // constructor 00027 NamedPointer::NamedPointer(string name,void *ptr,int index) { 00028 this->m_name = name; 00029 this->m_ptr = ptr; 00030 this->m_index = index; 00031 this->m_list = (void *)new NamedPointerList(); 00032 } 00033 00034 // copy constructor 00035 NamedPointer::NamedPointer(const NamedPointer &np) { 00036 this->m_name = np.m_name; 00037 this->m_ptr = np.m_ptr; 00038 this->m_index = np.m_index; 00039 this->m_list = this->copyList(np.m_list); 00040 } 00041 00042 // Destructor 00043 NamedPointer::~NamedPointer() { 00044 NamedPointerList *list = (NamedPointerList *)this->m_list; 00045 if (list != NULL) { 00046 delete list; 00047 } 00048 } 00049 00050 // Get the Name 00051 string NamedPointer::name() { 00052 return this->m_name; 00053 } 00054 00055 // Get the Pointer 00056 void *NamedPointer::ptr() { 00057 return this->m_ptr; 00058 } 00059 00060 // Get the Index 00061 int NamedPointer::index() { 00062 return this->m_index; 00063 } 00064 00065 // Get any associated list 00066 void *NamedPointer::list() { 00067 return this->m_list; 00068 } 00069 00070 // Copy the list 00071 void *NamedPointer::copyList(void *list) { 00072 NamedPointerList *npl = new NamedPointerList(); 00073 NamedPointerList *tmp_list = (NamedPointerList *)list; 00074 for(int i=0;tmp_list != NULL && i<(int)tmp_list->size();++i) { 00075 npl->push_back(tmp_list->at(i)); 00076 } 00077 return (void *)npl; 00078 } 00079
Generated on Tue Jul 12 2022 18:38:05 by
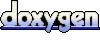