
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
LEDResource.h
00001 /** 00002 * @file LEDResource.h 00003 * @brief mbed CoAP Endpoint LED resource supporting CoAP PUT 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __LED_RESOURCE_H__ 00024 #define __LED_RESOURCE_H__ 00025 00026 // Base class 00027 #include "mbed-connector-interface/DynamicResource.h" 00028 00029 // our LED sensor 00030 DigitalOut __light(LED3); 00031 00032 // possible LED states 00033 #define LEDOFF "1" 00034 #define LEDON "0" 00035 00036 /** LEDResource class 00037 */ 00038 class LEDResource : public DynamicResource 00039 { 00040 00041 public: 00042 /** 00043 Default constructor 00044 @param logger input logger instance for this resource 00045 @param obj_name input the LED Object name 00046 @param res_name input the LED Resource name 00047 @param observable input the resource is Observable (default: FALSE) 00048 */ 00049 LEDResource(const Logger *logger,const char *obj_name,const char *res_name,const bool observable = false) : DynamicResource(logger,obj_name,res_name,"LED",M2MBase::PUT_ALLOWED,observable) { 00050 } 00051 00052 /** 00053 Set the value of the LED 00054 @param string input the string containing "0" (light off) or "1" (light on) 00055 */ 00056 virtual void put(const string value) { 00057 if (value.compare(string(LEDOFF)) == 0) __light = 0; 00058 else __light = 1; 00059 } 00060 }; 00061 00062 #endif // __LED_RESOURCE_H__
Generated on Tue Jul 12 2022 18:38:05 by
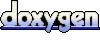