
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
ImageReaderSource.h
00001 // -*- mode:c++; tab-width:2; indent-tabs-mode:nil; c-basic-offset:2 -*- 00002 #ifndef __IMAGE_READER_SOURCE_H_ 00003 #define __IMAGE_READER_SOURCE_H_ 00004 /* 00005 * Copyright 2010-2011 ZXing authors 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 00020 #include "mbed.h" 00021 #include <iostream> 00022 #include <fstream> 00023 #include <string> 00024 #include <zxing/LuminanceSource.h> 00025 #include <zxing/common/Counted.h> 00026 #include <zxing/Binarizer.h> 00027 #include <zxing/MultiFormatReader.h> 00028 #include <zxing/Result.h> 00029 #include <zxing/ReaderException.h> 00030 #include <zxing/common/GlobalHistogramBinarizer.h> 00031 #include <zxing/common/HybridBinarizer.h> 00032 #include <exception> 00033 #include <zxing/Exception.h> 00034 #include <zxing/common/IllegalArgumentException.h> 00035 #include <zxing/BinaryBitmap.h> 00036 #include <zxing/DecodeHints.h> 00037 00038 #include <zxing/qrcode/QRCodeReader.h> 00039 #include <zxing/multi/qrcode/QRCodeMultiReader.h> 00040 #include <zxing/multi/ByQuadrantReader.h> 00041 #include <zxing/multi/MultipleBarcodeReader.h> 00042 #include <zxing/multi/GenericMultipleBarcodeReader.h> 00043 00044 using std::string; 00045 using std::ostringstream; 00046 using zxing::Ref; 00047 using zxing::ArrayRef; 00048 using zxing::LuminanceSource; 00049 00050 using namespace std; 00051 using namespace zxing; 00052 using namespace zxing::multi; 00053 using namespace zxing::qrcode; 00054 00055 00056 class ImageReaderSource : public zxing::LuminanceSource { 00057 private: 00058 typedef LuminanceSource Super; 00059 00060 const zxing::ArrayRef<char> image; 00061 const int comps; 00062 00063 char convertPixel(const char* pixel) const; 00064 00065 public: 00066 static zxing::Ref<LuminanceSource> create(char* buf, int buf_size, int width, int height); 00067 00068 ImageReaderSource(zxing::ArrayRef<char> image, int width, int height, int comps); 00069 00070 zxing::ArrayRef<char> getRow(int y, zxing::ArrayRef<char> row) const; 00071 zxing::ArrayRef<char> getMatrix() const; 00072 }; 00073 00074 extern int ex_decode(uint8_t* buf, int buf_size, int width, int height, vector<Ref<Result> > * results); 00075 00076 00077 #endif /* __IMAGE_READER_SOURCE_H_ */
Generated on Tue Jul 12 2022 18:38:05 by
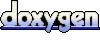