
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
DeviceManagementResponder.cpp
00001 /** 00002 * @file DeviceManagementResponder.cpp 00003 * @brief mbed CoAP Endpoint Device Management Responder class 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2016 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 // Class support 00024 #include "mbed-connector-interface/DeviceManagementResponder.h" 00025 00026 // Endpoint support 00027 #include "mbed-connector-interface/ConnectorEndpoint.h" 00028 00029 // DeviceManager Support 00030 #include "mbed-connector-interface/DeviceManager.h" 00031 00032 // constructor 00033 DeviceManagementResponder::DeviceManagementResponder(const Logger *logger,const Authenticator *authenticator) { 00034 this->m_logger = (Logger *)logger; 00035 this->m_authenticator = (Authenticator *)authenticator; 00036 this->m_endpoint = NULL; 00037 this->m_initialize_fn = NULL; 00038 this->m_reboot_responder_fn = NULL; 00039 this->m_reset_responder_fn = NULL; 00040 this->m_fota_manifest_fn = NULL; 00041 this->m_fota_image_set_fn = NULL; 00042 this->m_fota_invocation_fn = NULL; 00043 this->m_manifest = NULL; 00044 this->m_manifest_length = 0; 00045 this->m_fota_image = NULL; 00046 this->m_fota_image_length = 0; 00047 } 00048 00049 // copy constructor 00050 DeviceManagementResponder::DeviceManagementResponder(const DeviceManagementResponder &responder) { 00051 this->m_logger = responder.m_logger; 00052 this->m_authenticator = responder.m_authenticator; 00053 this->m_endpoint = responder.m_endpoint; 00054 this->m_initialize_fn = responder.m_initialize_fn; 00055 this->m_reboot_responder_fn = responder.m_reboot_responder_fn; 00056 this->m_reset_responder_fn = responder.m_reset_responder_fn; 00057 this->m_fota_manifest_fn = responder.m_fota_manifest_fn; 00058 this->m_fota_image_set_fn = responder.m_fota_image_set_fn; 00059 this->m_fota_invocation_fn = responder.m_fota_invocation_fn; 00060 this->m_manifest = responder.m_manifest; 00061 this->m_manifest_length = responder.m_manifest_length; 00062 this->m_fota_image = responder.m_fota_image; 00063 this->m_fota_image_length = responder.m_fota_image_length; 00064 } 00065 00066 // destructor 00067 DeviceManagementResponder::~DeviceManagementResponder() { 00068 } 00069 00070 // set the Endpoint 00071 void DeviceManagementResponder::setEndpoint(const void *ep) { 00072 this->m_endpoint = (void *)ep; 00073 } 00074 00075 // authenticate 00076 bool DeviceManagementResponder::authenticate(const void *challenge) { 00077 if (this->m_authenticator != NULL) { 00078 return this->m_authenticator->authenticate((void *)challenge); 00079 } 00080 return false; 00081 } 00082 00083 // set the Initialize handler 00084 void DeviceManagementResponder::setInitializeHandler(initialize_fn initialize_fn) { 00085 this->m_initialize_fn = initialize_fn; 00086 if (this->m_initialize_fn != NULL) { 00087 (*this->m_initialize_fn)(this->m_logger); 00088 } 00089 } 00090 00091 // set the Reboot Responder handler 00092 void DeviceManagementResponder::setRebootResponderHandler(responder_fn reboot_responder_fn) { 00093 this->m_reboot_responder_fn = reboot_responder_fn; 00094 } 00095 00096 // set the Reset Responder handler 00097 void DeviceManagementResponder::setResetResponderHandler(responder_fn reset_responder_fn) { 00098 this->m_reset_responder_fn = reset_responder_fn; 00099 } 00100 00101 // set the FOTA manifest handler 00102 void DeviceManagementResponder::setFOTAManifestHandler(manifest_fn fota_manifest_fn) { 00103 this->m_fota_manifest_fn = fota_manifest_fn; 00104 } 00105 00106 // set the FOTA image set handler 00107 void DeviceManagementResponder::setFOTAImageHandler(image_set_fn fota_image_set_fn) { 00108 this->m_fota_image_set_fn = fota_image_set_fn; 00109 } 00110 00111 // set the FOTA invocation handler 00112 void DeviceManagementResponder::setFOTAInvocationHandler(responder_fn fota_invocation_fn) { 00113 this->m_fota_invocation_fn = fota_invocation_fn; 00114 } 00115 00116 // set the FOTA manifest 00117 void DeviceManagementResponder::setFOTAManifest(char *manifest,uint32_t manifest_length) { 00118 this->m_manifest = manifest; 00119 this->m_manifest_length = manifest_length; 00120 if (this->m_fota_manifest_fn != NULL) { 00121 (*this->m_fota_manifest_fn)(this->m_endpoint,this->m_logger,this->m_manifest,this->m_manifest_length); 00122 } 00123 } 00124 00125 // get the FOTA manifest 00126 char *DeviceManagementResponder::getFOTAManifest() { 00127 return this->m_manifest; 00128 } 00129 00130 // get the FOTA manifest 00131 uint32_t DeviceManagementResponder::getFOTAManifestLength() { 00132 return this->m_manifest_length; 00133 } 00134 00135 // set the FOTA Image 00136 void DeviceManagementResponder::setFOTAImage(void *fota_image,uint32_t fota_image_length) { 00137 this->m_fota_image = fota_image; 00138 this->m_fota_image_length = fota_image_length; 00139 if (this->m_fota_image_set_fn != NULL) { 00140 (*this->m_fota_image_set_fn)(this->m_endpoint,this->m_logger,this->m_fota_image,this->m_fota_image_length); 00141 } 00142 } 00143 00144 // get the FOTA Image 00145 void *DeviceManagementResponder::getFOTAImage() { 00146 return this->m_fota_image; 00147 } 00148 00149 // get the FOTA Image Length 00150 uint32_t DeviceManagementResponder::getFOTAImageLength() { 00151 return this->m_fota_image_length; 00152 } 00153 00154 // ACTION: deregister device 00155 void DeviceManagementResponder::deregisterDevice(const void *challenge) { 00156 // check endpoint 00157 if (this->m_endpoint != NULL) { 00158 // authenticate 00159 if (this->authenticate(challenge)) { 00160 // DEBUG 00161 this->m_logger->logging("DeviceManagementResponder(deregister): de-registering device..."); 00162 00163 // act 00164 ((Connector::Endpoint *)this->m_endpoint)->de_register_endpoint(); 00165 } 00166 else { 00167 // authentication failure 00168 this->m_logger->logging("DeviceManagementResponder(deregister): authentication failed. No action taken."); 00169 } 00170 } 00171 else { 00172 // no endpoint 00173 this->m_logger->logging("DeviceManagementResponder(deregister): No endpoint instance. No action taken."); 00174 } 00175 } 00176 00177 // ACTION: reboot device 00178 void DeviceManagementResponder::rebootDevice(const void *challenge) { 00179 // check our Reboot Responder handler pointer 00180 if (this->m_reboot_responder_fn != NULL) { 00181 // check endpoint 00182 if (this->m_endpoint != NULL) { 00183 // authenticate 00184 if (this->authenticate(challenge)) { 00185 // act 00186 (*this->m_reboot_responder_fn)((const void *)this->m_endpoint,(const void *)this->m_logger,NULL); 00187 } 00188 else { 00189 // authentication failure 00190 this->m_logger->logging("DeviceManagementResponder(reboot): authentication failed. No action taken."); 00191 } 00192 } 00193 else { 00194 // no endpoint 00195 this->m_logger->logging("DeviceManagementResponder(reboot): No endpoint instance. No action taken."); 00196 } 00197 } 00198 else { 00199 // no reset responder handler 00200 this->m_logger->logging("DeviceManagementResponder(reboot): No reboot responder handler pointer. No action taken."); 00201 } 00202 } 00203 00204 // ACTION: reset device 00205 void DeviceManagementResponder::resetDevice(const void *challenge) { 00206 // check our Reset Responder handler pointer 00207 if (this->m_reset_responder_fn != NULL) { 00208 // check endpoint 00209 if (this->m_endpoint != NULL) { 00210 // authenticate 00211 if (this->authenticate(challenge)) { 00212 // act 00213 (*this->m_reset_responder_fn)((const void *)this->m_endpoint,(const void *)this->m_logger,NULL); 00214 } 00215 else { 00216 // authentication failure 00217 this->m_logger->logging("DeviceManagementResponder(reset): authentication failed. No action taken."); 00218 } 00219 } 00220 else { 00221 // no endpoint 00222 this->m_logger->logging("DeviceManagementResponder(reset): No endpoint instance. No action taken."); 00223 } 00224 } 00225 else { 00226 // no reset responder handler 00227 this->m_logger->logging("DeviceManagementResponder(reset): No reset responder handler pointer. No action taken."); 00228 } 00229 } 00230 00231 // ACTION: invoke FOTA 00232 void DeviceManagementResponder::invokeFOTA(const void *challenge) { 00233 // check our FOTA invocation handler pointer 00234 if (this->m_fota_invocation_fn != NULL) { 00235 // check endpoint 00236 if (this->m_endpoint != NULL) { 00237 // authenticate 00238 if (this->authenticate(challenge)) { 00239 // act 00240 (*this->m_fota_invocation_fn)((const void *)this->m_endpoint,(const void *)this->m_logger,this->getFOTAManifest()); 00241 } 00242 else { 00243 // authentication failure 00244 this->m_logger->logging("DeviceManagementResponder(FOTA): authentication failed. No action taken."); 00245 } 00246 } 00247 else { 00248 // no endpoint 00249 this->m_logger->logging("DeviceManagementResponder(FOTA): No endpoint instance. No action taken."); 00250 } 00251 } 00252 else { 00253 // no FOTA invocation handler 00254 this->m_logger->logging("DeviceManagementResponder(FOTA): No FOTA invocation handler pointer. No action taken."); 00255 } 00256 }
Generated on Tue Jul 12 2022 18:38:05 by
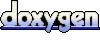