
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
DeviceDeRegisterResource.h
00001 /** 00002 * @file DeviceDeRegisterResource.h 00003 * @brief mbed CoAP Endpoint Device DeRegister Resource 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __DEVICE_DEREGISTER_RESOURCE_H__ 00024 #define __DEVICE_DEREGISTER_RESOURCE_H__ 00025 00026 // Base class 00027 #include "mbed-connector-interface/DynamicResource.h" 00028 00029 // Device Management Responder 00030 #include "mbed-connector-interface/DeviceManagementResponder.h" 00031 00032 /** DeviceDeRegisterResource class 00033 */ 00034 class DeviceDeRegisterResource : public DynamicResource 00035 { 00036 public: 00037 /** 00038 Default constructor 00039 @param logger input logger instance for this resource 00040 @param obj_name input the Light Object name 00041 @param res_name input the Light Resource name 00042 @param dm_responder input the DM responder instance 00043 */ 00044 DeviceDeRegisterResource(const Logger *logger,const char *obj_name,const char *res_name,const void *dm_responder,bool observable = false) : 00045 DynamicResource(logger,obj_name,res_name,"deregistration",M2MBase::POST_ALLOWED,observable) 00046 { 00047 this->m_dm_responder = (DeviceManagementResponder *)dm_responder; 00048 this->m_value = string("OK"); 00049 } 00050 00051 /** 00052 POST handler 00053 @param value input input value (DM authentication challenge) 00054 */ 00055 virtual void post(void *args) { 00056 // perform our action 00057 this->m_dm_responder->deregisterDevice(args); 00058 } 00059 00060 protected: 00061 DeviceManagementResponder *m_dm_responder; 00062 }; 00063 00064 #endif // __DEVICE_DEREGISTER_RESOURCE_H__
Generated on Tue Jul 12 2022 18:38:05 by
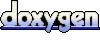