
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
DataWrapper.h
00001 /** 00002 * @file DataWrapper.h 00003 * @brief mbed CoAP Endpoint Data Wrapper class (header) 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __DATA_WRAPPER_H__ 00024 #define __DATA_WRAPPER_H__ 00025 00026 // mbedConnectorInterface configuration 00027 #include "mbed-connector-interface/mbedConnectorInterface.h" 00028 00029 // mbed support 00030 #if defined(MCI_USE_YOTTA) 00031 // mbed support 00032 #include "mbed-drivers/mbed.h" 00033 #else 00034 // mbed support 00035 #include "mbed.h" 00036 #endif 00037 00038 class DataWrapper { 00039 public: 00040 /** 00041 Default constructor 00042 @param data input the buffer to use for operations 00043 @param data_length input the data length 00044 */ 00045 DataWrapper(uint8_t *data,int data_length); 00046 00047 /** 00048 Default constructor (alloc) 00049 @param data_length input the data length (alloc) 00050 */ 00051 DataWrapper(int data_length); 00052 00053 /** 00054 Default copy constructor 00055 @param data input the DataWrapper to copy 00056 */ 00057 DataWrapper(const DataWrapper &data); 00058 00059 /** 00060 Destructor 00061 */ 00062 virtual ~DataWrapper(); 00063 00064 /** 00065 Wrap the data (trivial in base class) 00066 @param data input the data to wrap 00067 @param data_length input the length of the data to wrap 00068 */ 00069 virtual void wrap(uint8_t *data,int data_length); 00070 00071 /** 00072 Unwrap the data (trivial in base class) 00073 @param data input the data to unwrap 00074 @param data_length input the length of the data to unwrap 00075 */ 00076 virtual void unwrap(uint8_t *data,int data_length); 00077 00078 /** 00079 Get the wrap/unwrap result 00080 @return pointer to the data buffer of DataWrapper containing the wrap/unwrap result 00081 */ 00082 uint8_t *get() { return this->m_data; } 00083 00084 /** 00085 Get the wrap/unwrap result length 00086 @return length of the wrap/unwrap data result 00087 */ 00088 int length() { return this->m_data_length; } 00089 00090 /** 00091 Reset the wrapper 00092 */ 00093 void reset(); 00094 00095 /** 00096 Set the new application key 00097 @param appkey input the new appkey (encrypted) to set 00098 @param appkey_length input the new appkey (encrypted) length 00099 */ 00100 virtual void setAppKey(uint8_t *appkey,int appkey_length); 00101 00102 protected: 00103 uint8_t *m_data; 00104 00105 private: 00106 bool m_alloced; 00107 int m_data_length; 00108 int m_data_length_max; 00109 }; 00110 00111 #endif // __DATA_WRAPPER_H__ 00112
Generated on Tue Jul 12 2022 18:38:05 by
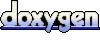