
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
BitArray.h
00001 // -*- mode:c++; tab-width:2; indent-tabs-mode:nil; c-basic-offset:2 -*- 00002 #ifndef __BIT_ARRAY_H__ 00003 #define __BIT_ARRAY_H__ 00004 00005 /* 00006 * Copyright 2010 ZXing authors. All rights reserved. 00007 * 00008 * Licensed under the Apache License, Version 2.0 (the "License"); 00009 * you may not use this file except in compliance with the License. 00010 * You may obtain a copy of the License at 00011 * 00012 * http://www.apache.org/licenses/LICENSE-2.0 00013 * 00014 * Unless required by applicable law or agreed to in writing, software 00015 * distributed under the License is distributed on an "AS IS" BASIS, 00016 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 00021 #include <zxing/ZXing.h> 00022 #include <zxing/common/Counted.h> 00023 #include <zxing/common/IllegalArgumentException.h> 00024 #include <zxing/common/Array.h> 00025 #include <vector> 00026 #include <limits> 00027 #include <iostream> 00028 00029 namespace zxing { 00030 00031 class BitArray : public Counted { 00032 public: 00033 static const int bitsPerWord = std::numeric_limits<unsigned int>::digits; 00034 00035 private: 00036 int size; 00037 ArrayRef<int> bits; 00038 static const int logBits = ZX_LOG_DIGITS(bitsPerWord); 00039 static const int bitsMask = (1 << logBits) - 1; 00040 00041 public: 00042 BitArray(int size); 00043 ~BitArray(); 00044 int getSize() const; 00045 00046 bool get(int i) const { 00047 return (bits[i >> logBits] & (1 << (i & bitsMask))) != 0; 00048 } 00049 00050 void set(int i) { 00051 bits[i >> logBits] |= 1 << (i & bitsMask); 00052 } 00053 00054 int getNextSet(int from); 00055 int getNextUnset(int from); 00056 00057 void setBulk(int i, int newBits); 00058 void setRange(int start, int end); 00059 void clear(); 00060 bool isRange(int start, int end, bool value); 00061 std::vector<int>& getBitArray(); 00062 00063 void reverse(); 00064 00065 class Reverse { 00066 private: 00067 Ref<BitArray> array; 00068 public: 00069 Reverse(Ref<BitArray> array); 00070 ~Reverse(); 00071 }; 00072 00073 private: 00074 static int makeArraySize(int size); 00075 }; 00076 00077 std::ostream& operator << (std::ostream&, BitArray const&); 00078 00079 } 00080 00081 #endif // __BIT_ARRAY_H__
Generated on Tue Jul 12 2022 18:38:05 by
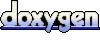