
This is the sample program that can see the decode result of barcode data on Watson IoT.
Dependencies: AsciiFont DisplayApp GR-PEACH_video LCD_shield_config LWIPBP3595Interface_STA_for_mbed-os USBDevice
Array.h
00001 // -*- mode:c++; tab-width:2; indent-tabs-mode:nil; c-basic-offset:2 -*- 00002 #ifndef __ARRAY_H__ 00003 #define __ARRAY_H__ 00004 00005 /* 00006 * Array.h 00007 * zxing 00008 * 00009 * Copyright 2010 ZXing authors All rights reserved. 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); 00012 * you may not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, 00019 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 */ 00023 00024 #include <vector> 00025 00026 #include <zxing/common/Counted.h> 00027 00028 namespace zxing { 00029 00030 template<typename T> class Array : public Counted { 00031 protected: 00032 public: 00033 std::vector<T> values_; 00034 Array() {} 00035 Array(int n) : 00036 Counted(), values_(n, T()) { 00037 } 00038 Array(T const* ts, int n) : 00039 Counted(), values_(ts, ts+n) { 00040 } 00041 Array(T const* ts, T const* te) : 00042 Counted(), values_(ts, te) { 00043 } 00044 Array(T v, int n) : 00045 Counted(), values_(n, v) { 00046 } 00047 Array(std::vector<T> &v) : 00048 Counted(), values_(v) { 00049 } 00050 Array(Array<T> &other) : 00051 Counted(), values_(other.values_) { 00052 } 00053 Array(Array<T> *other) : 00054 Counted(), values_(other->values_) { 00055 } 00056 virtual ~Array() { 00057 } 00058 Array<T>& operator=(const Array<T> &other) { 00059 values_ = other.values_; 00060 return *this; 00061 } 00062 Array<T>& operator=(const std::vector<T> &array) { 00063 values_ = array; 00064 return *this; 00065 } 00066 T const& operator[](int i) const { 00067 return values_[i]; 00068 } 00069 T& operator[](int i) { 00070 return values_[i]; 00071 } 00072 int size() const { 00073 return values_.size(); 00074 } 00075 bool empty() const { 00076 return values_.size() == 0; 00077 } 00078 std::vector<T> const& values() const { 00079 return values_; 00080 } 00081 std::vector<T>& values() { 00082 return values_; 00083 } 00084 }; 00085 00086 template<typename T> class ArrayRef : public Counted { 00087 private: 00088 public: 00089 Array<T> *array_; 00090 ArrayRef() : 00091 array_(0) { 00092 } 00093 explicit ArrayRef(int n) : 00094 array_(0) { 00095 reset(new Array<T> (n)); 00096 } 00097 ArrayRef(T *ts, int n) : 00098 array_(0) { 00099 reset(new Array<T> (ts, n)); 00100 } 00101 ArrayRef(Array<T> *a) : 00102 array_(0) { 00103 reset(a); 00104 } 00105 ArrayRef(const ArrayRef &other) : 00106 Counted(), array_(0) { 00107 reset(other.array_); 00108 } 00109 00110 template<class Y> 00111 ArrayRef(const ArrayRef<Y> &other) : 00112 array_(0) { 00113 reset(static_cast<const Array<T> *>(other.array_)); 00114 } 00115 00116 ~ArrayRef() { 00117 if (array_) { 00118 array_->release(); 00119 } 00120 array_ = 0; 00121 } 00122 00123 T const& operator[](int i) const { 00124 return (*array_)[i]; 00125 } 00126 00127 T& operator[](int i) { 00128 return (*array_)[i]; 00129 } 00130 00131 void reset(Array<T> *a) { 00132 if (a) { 00133 a->retain(); 00134 } 00135 if (array_) { 00136 array_->release(); 00137 } 00138 array_ = a; 00139 } 00140 void reset(const ArrayRef<T> &other) { 00141 reset(other.array_); 00142 } 00143 ArrayRef<T>& operator=(const ArrayRef<T> &other) { 00144 reset(other); 00145 return *this; 00146 } 00147 ArrayRef<T>& operator=(Array<T> *a) { 00148 reset(a); 00149 return *this; 00150 } 00151 00152 Array<T>& operator*() const { 00153 return *array_; 00154 } 00155 00156 Array<T>* operator->() const { 00157 return array_; 00158 } 00159 00160 operator bool () const { 00161 return array_ != 0; 00162 } 00163 bool operator ! () const { 00164 return array_ == 0; 00165 } 00166 }; 00167 00168 } // namespace zxing 00169 00170 #endif // __ARRAY_H__
Generated on Tue Jul 12 2022 18:38:05 by
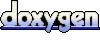