Cmera and GPS labrary
Dependents: HEPTA_SENSOR HEPTA_SENSOR
HeptaCamera_GPS.h
00001 #include "mbed.h" 00002 #include "SerialBuffered.h" 00003 00004 #ifndef HEPTA_SERIAL_H 00005 #define HEPTA_SERIAL_H 00006 00007 class HeptaCamera_GPS 00008 { 00009 public: 00010 00011 enum JpegResolution { 00012 JpegResolution80x64 = 0x01, // unofficial 00013 JpegResolution160x128 = 0x03, // unofficial 00014 JpegResolution320x240 = 0x05, // QVGA 00015 JpegResolution640x480 = 0x07 // VGA 00016 }; 00017 00018 enum ErrorNumber { 00019 NoError = 0x00, 00020 UnexpectedReply = 0x04, 00021 ParameterError = 0x0b, 00022 SendRegisterTimeout = 0x0c, 00023 CommandIdError = 0x0d, 00024 CommandHeaderError = 0xf0, 00025 SetTransferPackageSizeWrong = 0x11 00026 }; 00027 00028 enum Baud { 00029 Baud460800 = 0x02, 00030 Baud230400 = 0x03, 00031 Baud115200 = 0x04, 00032 Baud57600 = 0x05, 00033 Baud28800 = 0x06, 00034 Baud14400 = 0x07 // Default. 00035 }; 00036 00037 enum ResetType { 00038 Nomal = 0x00, 00039 High = 0xff 00040 }; 00041 00042 HeptaCamera_GPS(PinName tx, PinName rx, PinName CAM, PinName GPS); 00043 00044 ~HeptaCamera_GPS(); 00045 00046 ErrorNumber sync(); 00047 ErrorNumber init(Baud baud, JpegResolution jr); 00048 ErrorNumber getJpegSnapshotPicture(FILE*fp); 00049 ErrorNumber getJpegSnapshotPicture_data(FILE*fp); 00050 void setmbedBaud(Baud baud); 00051 void camera_setting(void); 00052 void Sync(void); 00053 void initialize(Baud baud,JpegResolution jr); 00054 void test_jpeg_snapshot_picture(const char *filename); 00055 void test_jpeg_snapshot_data(const char *filename); 00056 void jpeg_callback(char *buf, size_t siz); 00057 void gps_setting(void); 00058 char getc(); 00059 int readable(); 00060 void flushSerialBuffer(void); 00061 void gga_sensing(float *time, float *latitude, char *ns, float *longitude, char *ew, int *quality, int *stnum, float *hacu, float *altitude, char *aunit, int *gps_check); 00062 void lat_log_sensing_u16(char *lat, char *log, char * height); 00063 00064 private: 00065 SerialBuffered serial; 00066 DigitalOut CAM_SW; 00067 DigitalOut GPS_SW; 00068 static const int COMMAND_LENGTH = 6; 00069 static const int SYNCMAX = 60; 00070 static const int packageSize = 256; 00071 //static const int CAPTURE_FRAMES = 3; 00072 00073 char msg[256],msgd[256]; 00074 int i,ite,rlock,stn; 00075 int _quality,_stnum; 00076 float _hacu; 00077 float _altitude; 00078 char _aunit; 00079 int _gps_check; 00080 00081 char c; 00082 char gps_data[7][1000]; 00083 char ns,ew,statas; 00084 float time,hokui,tokei,vel; 00085 float g_hokui,g_tokei; 00086 float d_hokui,m_hokui,d_tokei,m_tokei; 00087 int h_time,m_time,s_time; 00088 float height_1; 00089 int m_height,cm_height; 00090 00091 ErrorNumber sendInitial(Baud band, JpegResolution jr); 00092 ErrorNumber sendGetPicture(void); 00093 ErrorNumber sendSnapshot(void); 00094 ErrorNumber sendSetPackageSize(uint16_t packageSize); 00095 ErrorNumber sendReset(ResetType specialReset); 00096 ErrorNumber recvData(uint32_t *length); 00097 ErrorNumber sendSync(); 00098 ErrorNumber recvSync(); 00099 ErrorNumber sendAck(uint8_t commandId, uint16_t packageId); 00100 ErrorNumber recvAckOrNck(); 00101 00102 bool sendBytes(char *buf, size_t len, int timeout_us = 20000); 00103 bool recvBytes(char *buf, size_t len, int timeout_us = 20000); 00104 bool waitRecv(); 00105 bool waitIdle(); 00106 }; 00107 #endif
Generated on Sat Jul 16 2022 13:32:45 by
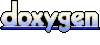