A copy of the mbed USBDevice with USBSerial library
Dependents: STM32L0_LoRa Smartage STM32L0_LoRa Turtle_RadioShuttle
USBSerialBuffered.h
00001 /* 00002 * $Id: bulkserial.h,v 1.3 2018/02/23 15:04:29 grimrath Exp $ 00003 * This is an unpublished work copyright (c) 2018 HELIOS Software GmbH 00004 * 30827 Garbsen, Germany 00005 */ 00006 #ifndef __USBSERIALBUFFERED_H__ 00007 #define __USBSERIALBUFFERED_H__ 00008 00009 #include "USBSerial.h" 00010 00011 /** This class is a wrapper around @ref USBSerial such that sending 00012 * of serial data over USB is supported in (and outside) of interrupt context. 00013 * In addition it buffers characters (similiar to the I/O buffering of stdio) 00014 * before starting a USB data transmit. Silently discards data if the 00015 * @ref USBSerial object is not connected to the USB host. 00016 */ 00017 class USBSerialBuffered : public USBSerial { 00018 public: 00019 USBSerialBuffered(int MaxBuffSize = 128, uint16_t vendor_id = 0x16c0, uint16_t product_id = 0x05e1, uint16_t product_release = 0x0001, bool connect_blocking = false); 00020 ~USBSerialBuffered(); 00021 00022 /** sends internally queued but not yet sent data. Is blocking. Must not 00023 * be called from interrupt context. */ 00024 void flush(); 00025 00026 /** Writes a formatted string into an internal buffer for later sending. 00027 * with e.g. @ref flush . Explicitly designed to be called from interrupt 00028 * context. If the string does not fit into the internal buffer it is 00029 * silently truncated. */ 00030 int printf_irqsafe(const char *fmt, ...) __attribute__((format(printf,2,3))); 00031 00032 /** the varargs variant of printf_ */ 00033 int vprintf_irqsafe(const char *fmt, std::va_list ap); 00034 00035 protected: 00036 /** Called from @ref Stream::printf and other stdio-like methods from the base class @ref Stream . */ 00037 virtual int _putc(int c); 00038 00039 private: 00040 enum { 00041 CorkBuffSize = MAX_PACKET_SIZE_EPBULK, 00042 }; 00043 00044 static inline bool RunningInInterrupt() { 00045 return SCB->ICSR & SCB_ICSR_VECTACTIVE_Msk; 00046 } 00047 00048 int irqbuff_acquire(); 00049 void irqbuff_release(int buffused); 00050 00051 void putc_normal(int c); 00052 00053 private: 00054 int mFullBuffSize; 00055 char *m_buff; 00056 int m_irq_buffused; 00057 }; 00058 00059 #endif // __USBSERIALBUFFERED_H__
Generated on Fri Jul 15 2022 20:07:58 by
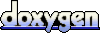