
Test
Fork of Nucleo-mbed-os-example-blinky by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut myled(LED1); 00004 InterruptIn event(USER_BUTTON); 00005 00006 event_callback_t spiCb; 00007 00008 #if DEVICE_SPI_ASYNCH 00009 #define SPI_S(obj) (( struct spi_s *)(&(obj->spi))) 00010 #else 00011 #define SPI_S(obj) (( struct spi_s *)(obj)) 00012 #endif 00013 00014 bool pressed = false; 00015 bool transferDone = false; 00016 00017 void KeyPressed() 00018 { 00019 pressed = true; 00020 } 00021 00022 void SPIDone(int events) 00023 { 00024 transferDone = true; 00025 } 00026 00027 int main() { 00028 event.fall(&KeyPressed); 00029 00030 spiCb.attach(SPIDone); 00031 00032 while(1) { 00033 myled = 1; // LED is ON 00034 wait(0.2); // 200 ms 00035 myled = 0; // LED is OFF 00036 wait(1.0); // 1 sec 00037 if (pressed) { 00038 myled = 1; 00039 SPI *s = new SPI(PB_5, PB_4, PB_3); 00040 s->frequency(100000); 00041 00042 struct spi_s *spiobj = SPI_S(((spi_t *)( (char *)s+4) )); 00043 SPI_HandleTypeDef *handle = &(spiobj->handle); 00044 00045 00046 for(int i = 0; i < 400000000; i++) { 00047 uint8_t send[] = { 0x55, 0x55, 0x55, 0x55, 0x55, 0x55, 0x55, 0x55, 0x55, 0x55, 0x55, 0x00 }; 00048 uint8_t rcv[] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; 00049 //s->write(0xff); 00050 HAL_SPI_Transmit(handle, send, 10, 10); 00051 //HAL_SPI_Receive(handle, rcv, 10, 10); 00052 00053 } 00054 //s->transfer((uint8_t*)"aaa", 3, (uint8_t*)NULL, 0, spiCb, SPI_EVENT_COMPLETE); 00055 // s->transfer((uint8_t*)"aaa", 3, (uint8_t*)NULL, 0, spiCb); 00056 delete s; 00057 deepsleep(); 00058 pressed = false; 00059 } 00060 } 00061 }
Generated on Sun Jul 17 2022 17:36:16 by
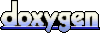