Its a code of W7500 board for the vending machine to vend an item to the user within the organization. When the user is identified with the help of the RFID reader and when the user choose the item . the data is sent serially to WIZ750SR and the item is vended . The LCD displays the details of the transaction.
Dependencies: HCSR04 MFRC522 TextLCD mbed-src
Fork of RFID_copy by
main.cpp
00001 00002 //Connect as follows: 00003 //RFID pins, motor 00004 //---------------------------------------- 00005 //RFID IRQ=pin5 -> Not used. Leave open 00006 //RFID MISO=pin4 -> Nucleo SPI_MISO=PA_6=D12 00007 //RFID MOSI=pin3 -> Nucleo SPI_MOSI=PA_7=D11 00008 //RFID SCK=pin2 -> Nucleo SPI_SCK =PA_5=D13 00009 //RFID SDA=pin1 -> Nucleo SPI_CS =PB_6=D10 00010 //RFID RST=pin7 -> Nucleo =PA_9=D8 00011 //3.3V and Gnd to the respective pins 00012 // 1st Motor transistor to Pin D9 00013 // 2nd Motor transistor to Pin D14 00014 // Pin D0 to pin 2 of the serial Cable 00015 // pin D1 to pin 3 of the serial cable 00016 // pin A0 to the trigger of ultrasonic sensor 1 00017 // pin A1 to the Echo of ultrasonic sensor 1 00018 // pin D2 to RS pin of LCD 00019 // pin D3 to E pin of LCD 00020 // pin D4 to D4 pin of LCD 00021 // pin D5 to D5 pin of LCD 00022 // pin D6 to D6 pin of LCD 00023 // pin D7 to D7 pin of LCD 00024 00025 //--------------------------------------------- 00026 #include "mbed.h" 00027 #include "MFRC522.h" 00028 #include "SPI.h" 00029 #include "hcsr04.h" 00030 #include "TextLCD.h" 00031 #define ECHO_SERVER_PORT 7 00032 #define SPI_MOSI D11 00033 #define SPI_MISO D12 00034 #define SPI_SCK D13 00035 #define SPI_CS D10 00036 #define MF_RESET D8 00037 TextLCD lcd(D2, D3, D4, D5, D6, D7,TextLCD::LCD20x4); // rs, e, d4-d7 00038 DigitalOut myled(D9); 00039 DigitalOut myled1(D14); 00040 //DigitalOut myled2(D15); Can be enabled for 3rd motor slot. 00041 00042 HCSR04 sensor(A0,A1); // Trigger , Echo 00043 HCSR04 sensor1(A2,A3); //Trigger , Echo 00044 //HCSR04 sensor2(A4,A5); //Trigger , Echo Can enable if needed for 3rd slot. 00045 00046 void distance(); 00047 void display(); 00048 00049 //Serial connection to PC for output 00050 Serial pc(USBTX, USBRX); 00051 // Serial connection with WIZ750SR gateway for MQTT communication 00052 Serial serial(D1,D0); //TX and RX 00053 // Initializing RFID chip pins 00054 MFRC522 RfChip (SPI_MOSI, SPI_MISO, SPI_SCK, SPI_CS, MF_RESET); 00055 char c; 00056 int d; 00057 int main(void) { 00058 pc.printf("starting...\n"); 00059 myled=0; 00060 pc.printf("Wait a second...\r\n"); 00061 00062 //Init. RC522 Chip 00063 RfChip.PCD_Init(); 00064 00065 while (true) { 00066 pc.printf("enterd RFID reading loop...\n"); 00067 // Look for new cards 00068 if ( ! RfChip.PICC_IsNewCardPresent()) 00069 { 00070 wait_ms(500); 00071 continue; 00072 } 00073 // Select one of the cards 00074 if ( ! RfChip.PICC_ReadCardSerial()) 00075 { 00076 wait_ms(500); 00077 continue; 00078 } 00079 00080 char data[20]=""; 00081 char data1[20]=""; 00082 00083 // Print Card UID 00084 pc.printf("Card UID: "); 00085 printf("Size of UID: %d \n",RfChip.uid.size); 00086 for (uint8_t i = 0; i < RfChip.uid.size; i++) 00087 { 00088 char temp[5]; 00089 pc.printf(" %X02", RfChip.uid.uidByte[i]); 00090 sprintf(temp,"%X02", RfChip.uid.uidByte[i]); 00091 strcat(data,temp); 00092 } 00093 00094 // Print Card type 00095 uint8_t piccType = RfChip.PICC_GetType(RfChip.uid.sak); 00096 pc.printf(" \nPICC Type: %s \n\r", RfChip.PICC_GetTypeName(piccType)); 00097 wait_ms(1000); 00098 00099 if(strcmp(data,"BA0211026102D302")==0) //Comparing the stored data with the new data read by the RFID reader. 00100 { 00101 strcat(data1,"natsu"); 00102 wait(1); 00103 serial.printf("%s",data1); 00104 wait(2); 00105 distance(); 00106 } 00107 if(strcmp(data,"E8028002AC02E902")==0) 00108 { 00109 strcat(data1,"luffy"); 00110 wait(1); 00111 serial.printf("%s",data1); 00112 wait(2); 00113 distance (); 00114 } 00115 pc.printf("The user name is :%s \n",data1); 00116 } 00117 } 00118 00119 00120 void distance() 00121 { 00122 00123 pc.printf("Entered into distance loop \n"); 00124 while(1){ 00125 long distance = sensor.distance(); 00126 wait(0.5); 00127 long distance1 = sensor1.distance(); 00128 wait(0.5); 00129 // long distance2 = sensor2.distance(); //can be enabled if connected to 3rd Ultrasonic sensor 00130 pc.printf("distance %d \n",distance); 00131 pc.printf("distance %d \n",distance1); 00132 // pc.printf("distance %d \n",distance2); //can be enabled if connected to 3rd Ultrasonic sensor to enable it in the PC. 00133 wait(1); 00134 00135 wait(1.0); // 1 sec 00136 if(distance<=10){ 00137 myled= 1; 00138 wait(3); 00139 myled=0; 00140 serial.printf("/drink"); 00141 c = '\0'; 00142 c = 'd'; 00143 d=15; 00144 display(); 00145 pc.printf("/ item drink /cost 15"); 00146 break; 00147 } 00148 else if(distance1<=10){ 00149 myled1= 1; 00150 wait(3); 00151 myled1=0; 00152 serial.printf("/snack"); 00153 c = '\0'; 00154 c = 's'; 00155 d=10; 00156 display(); 00157 pc.printf("/item snack /cost 10"); 00158 break; 00159 } 00160 /* else if(distance2<=10){ 00161 myled1= 1; 00162 wait(30); 00163 myled1=0; 00164 serial.printf("/choco"); 00165 c = '\0'; 00166 c = 's'; 00167 d=10; 00168 display(); 00169 pc.printf("/snack/10"); 00170 break; 00171 } */ //Can be enabled when 3rd ultrasonic is connected. 00172 00173 } 00174 } 00175 00176 //function for LCD display .... 00177 00178 void display(){ 00179 lcd.locate(3,0); 00180 lcd.printf("Vending Machine\n"); 00181 lcd.locate(0,2); 00182 if(c=='d'){ 00183 lcd.printf("ITEM NAME: drink"); 00184 } 00185 else if(c=='s') 00186 { 00187 lcd.printf("ITEM NAME: Snack"); 00188 } 00189 lcd.locate(0,3); 00190 lcd.printf("ITEM COST: %d",d); 00191 }
Generated on Tue Jul 19 2022 20:40:41 by
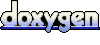