
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
x509write_csr.c
00001 /* 00002 * X.509 Certificate Signing Request writing 00003 * 00004 * Copyright (C) 2006-2014, Brainspark B.V. 00005 * 00006 * This file is part of PolarSSL (http://www.polarssl.org) 00007 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00008 * 00009 * All rights reserved. 00010 * 00011 * This program is free software; you can redistribute it and/or modify 00012 * it under the terms of the GNU General Public License as published by 00013 * the Free Software Foundation; either version 2 of the License, or 00014 * (at your option) any later version. 00015 * 00016 * This program is distributed in the hope that it will be useful, 00017 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00018 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00019 * GNU General Public License for more details. 00020 * 00021 * You should have received a copy of the GNU General Public License along 00022 * with this program; if not, write to the Free Software Foundation, Inc., 00023 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00024 */ 00025 /* 00026 * References: 00027 * - CSRs: PKCS#10 v1.7 aka RFC 2986 00028 * - attributes: PKCS#9 v2.0 aka RFC 2985 00029 */ 00030 00031 #if !defined(POLARSSL_CONFIG_FILE) 00032 #include "polarssl/config.h" 00033 #else 00034 #include POLARSSL_CONFIG_FILE 00035 #endif 00036 00037 #if defined(POLARSSL_X509_CSR_WRITE_C) 00038 00039 #include "polarssl/x509_csr.h" 00040 #include "polarssl/oid.h" 00041 #include "polarssl/asn1write.h" 00042 00043 #if defined(POLARSSL_PEM_WRITE_C) 00044 #include "polarssl/pem.h" 00045 #endif 00046 00047 #include <string.h> 00048 #include <stdlib.h> 00049 00050 void x509write_csr_init( x509write_csr *ctx ) 00051 { 00052 memset( ctx, 0, sizeof(x509write_csr) ); 00053 } 00054 00055 void x509write_csr_free( x509write_csr *ctx ) 00056 { 00057 asn1_free_named_data_list( &ctx->subject ); 00058 asn1_free_named_data_list( &ctx->extensions ); 00059 00060 memset( ctx, 0, sizeof(x509write_csr) ); 00061 } 00062 00063 void x509write_csr_set_md_alg( x509write_csr *ctx, md_type_t md_alg ) 00064 { 00065 ctx->md_alg = md_alg; 00066 } 00067 00068 void x509write_csr_set_key( x509write_csr *ctx, pk_context *key ) 00069 { 00070 ctx->key = key; 00071 } 00072 00073 int x509write_csr_set_subject_name( x509write_csr *ctx, 00074 const char *subject_name ) 00075 { 00076 return x509_string_to_names( &ctx->subject, subject_name ); 00077 } 00078 00079 int x509write_csr_set_extension( x509write_csr *ctx, 00080 const char *oid, size_t oid_len, 00081 const unsigned char *val, size_t val_len ) 00082 { 00083 return x509_set_extension( &ctx->extensions, oid, oid_len, 00084 0, val, val_len ); 00085 } 00086 00087 int x509write_csr_set_key_usage( x509write_csr *ctx, unsigned char key_usage ) 00088 { 00089 unsigned char buf[4]; 00090 unsigned char *c; 00091 int ret; 00092 00093 c = buf + 4; 00094 00095 if( ( ret = asn1_write_bitstring( &c, buf, &key_usage, 7 ) ) != 4 ) 00096 return( ret ); 00097 00098 ret = x509write_csr_set_extension( ctx, OID_KEY_USAGE, 00099 OID_SIZE( OID_KEY_USAGE ), 00100 buf, 4 ); 00101 if( ret != 0 ) 00102 return( ret ); 00103 00104 return( 0 ); 00105 } 00106 00107 int x509write_csr_set_ns_cert_type( x509write_csr *ctx, 00108 unsigned char ns_cert_type ) 00109 { 00110 unsigned char buf[4]; 00111 unsigned char *c; 00112 int ret; 00113 00114 c = buf + 4; 00115 00116 if( ( ret = asn1_write_bitstring( &c, buf, &ns_cert_type, 8 ) ) != 4 ) 00117 return( ret ); 00118 00119 ret = x509write_csr_set_extension( ctx, OID_NS_CERT_TYPE, 00120 OID_SIZE( OID_NS_CERT_TYPE ), 00121 buf, 4 ); 00122 if( ret != 0 ) 00123 return( ret ); 00124 00125 return( 0 ); 00126 } 00127 00128 int x509write_csr_der( x509write_csr *ctx, unsigned char *buf, size_t size, 00129 int (*f_rng)(void *, unsigned char *, size_t), 00130 void *p_rng ) 00131 { 00132 int ret; 00133 const char *sig_oid; 00134 size_t sig_oid_len = 0; 00135 unsigned char *c, *c2; 00136 unsigned char hash[64]; 00137 unsigned char sig[POLARSSL_MPI_MAX_SIZE]; 00138 unsigned char tmp_buf[2048]; 00139 size_t pub_len = 0, sig_and_oid_len = 0, sig_len; 00140 size_t len = 0; 00141 pk_type_t pk_alg; 00142 00143 /* 00144 * Prepare data to be signed in tmp_buf 00145 */ 00146 c = tmp_buf + sizeof( tmp_buf ); 00147 00148 ASN1_CHK_ADD( len, x509_write_extensions( &c, tmp_buf, ctx->extensions ) ); 00149 00150 if( len ) 00151 { 00152 ASN1_CHK_ADD( len, asn1_write_len( &c, tmp_buf, len ) ); 00153 ASN1_CHK_ADD( len, asn1_write_tag( &c, tmp_buf, ASN1_CONSTRUCTED | 00154 ASN1_SEQUENCE ) ); 00155 00156 ASN1_CHK_ADD( len, asn1_write_len( &c, tmp_buf, len ) ); 00157 ASN1_CHK_ADD( len, asn1_write_tag( &c, tmp_buf, ASN1_CONSTRUCTED | 00158 ASN1_SET ) ); 00159 00160 ASN1_CHK_ADD( len, asn1_write_oid( &c, tmp_buf, OID_PKCS9_CSR_EXT_REQ, 00161 OID_SIZE( OID_PKCS9_CSR_EXT_REQ ) ) ); 00162 00163 ASN1_CHK_ADD( len, asn1_write_len( &c, tmp_buf, len ) ); 00164 ASN1_CHK_ADD( len, asn1_write_tag( &c, tmp_buf, ASN1_CONSTRUCTED | 00165 ASN1_SEQUENCE ) ); 00166 } 00167 00168 ASN1_CHK_ADD( len, asn1_write_len( &c, tmp_buf, len ) ); 00169 ASN1_CHK_ADD( len, asn1_write_tag( &c, tmp_buf, ASN1_CONSTRUCTED | 00170 ASN1_CONTEXT_SPECIFIC ) ); 00171 00172 ASN1_CHK_ADD( pub_len, pk_write_pubkey_der( ctx->key, 00173 tmp_buf, c - tmp_buf ) ); 00174 c -= pub_len; 00175 len += pub_len; 00176 00177 /* 00178 * Subject ::= Name 00179 */ 00180 ASN1_CHK_ADD( len, x509_write_names( &c, tmp_buf, ctx->subject ) ); 00181 00182 /* 00183 * Version ::= INTEGER { v1(0), v2(1), v3(2) } 00184 */ 00185 ASN1_CHK_ADD( len, asn1_write_int( &c, tmp_buf, 0 ) ); 00186 00187 ASN1_CHK_ADD( len, asn1_write_len( &c, tmp_buf, len ) ); 00188 ASN1_CHK_ADD( len, asn1_write_tag( &c, tmp_buf, ASN1_CONSTRUCTED | 00189 ASN1_SEQUENCE ) ); 00190 00191 /* 00192 * Prepare signature 00193 */ 00194 md( md_info_from_type( ctx->md_alg ), c, len, hash ); 00195 00196 pk_alg = pk_get_type( ctx->key ); 00197 if( pk_alg == POLARSSL_PK_ECKEY ) 00198 pk_alg = POLARSSL_PK_ECDSA; 00199 00200 if( ( ret = pk_sign( ctx->key, ctx->md_alg, hash, 0, sig, &sig_len, 00201 f_rng, p_rng ) ) != 0 || 00202 ( ret = oid_get_oid_by_sig_alg( pk_alg, ctx->md_alg, 00203 &sig_oid, &sig_oid_len ) ) != 0 ) 00204 { 00205 return( ret ); 00206 } 00207 00208 /* 00209 * Write data to output buffer 00210 */ 00211 c2 = buf + size; 00212 ASN1_CHK_ADD( sig_and_oid_len, x509_write_sig( &c2, buf, 00213 sig_oid, sig_oid_len, sig, sig_len ) ); 00214 00215 c2 -= len; 00216 memcpy( c2, c, len ); 00217 00218 len += sig_and_oid_len; 00219 ASN1_CHK_ADD( len, asn1_write_len( &c2, buf, len ) ); 00220 ASN1_CHK_ADD( len, asn1_write_tag( &c2, buf, ASN1_CONSTRUCTED | 00221 ASN1_SEQUENCE ) ); 00222 00223 return( (int) len ); 00224 } 00225 00226 #define PEM_BEGIN_CSR "-----BEGIN CERTIFICATE REQUEST-----\n" 00227 #define PEM_END_CSR "-----END CERTIFICATE REQUEST-----\n" 00228 00229 #if defined(POLARSSL_PEM_WRITE_C) 00230 int x509write_csr_pem( x509write_csr *ctx, unsigned char *buf, size_t size, 00231 int (*f_rng)(void *, unsigned char *, size_t), 00232 void *p_rng ) 00233 { 00234 int ret; 00235 unsigned char output_buf[4096]; 00236 size_t olen = 0; 00237 00238 if( ( ret = x509write_csr_der( ctx, output_buf, sizeof(output_buf), 00239 f_rng, p_rng ) ) < 0 ) 00240 { 00241 return( ret ); 00242 } 00243 00244 if( ( ret = pem_write_buffer( PEM_BEGIN_CSR, PEM_END_CSR, 00245 output_buf + sizeof(output_buf) - ret, 00246 ret, buf, size, &olen ) ) != 0 ) 00247 { 00248 return( ret ); 00249 } 00250 00251 return( 0 ); 00252 } 00253 #endif /* POLARSSL_PEM_WRITE_C */ 00254 00255 #endif /* POLARSSL_X509_CSR_WRITE_C */ 00256 00257
Generated on Tue Jul 12 2022 19:40:21 by
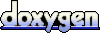