
Example program to test AES-GCM functionality. Used for a workshop
Embed:
(wiki syntax)
Show/hide line numbers
version.h
Go to the documentation of this file.
00001 /** 00002 * \file version.h 00003 * 00004 * \brief Run-time version information 00005 * 00006 * Copyright (C) 2006-2014, Brainspark B.V. 00007 * 00008 * This file is part of PolarSSL (http://www.polarssl.org) 00009 * Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org> 00010 * 00011 * All rights reserved. 00012 * 00013 * This program is free software; you can redistribute it and/or modify 00014 * it under the terms of the GNU General Public License as published by 00015 * the Free Software Foundation; either version 2 of the License, or 00016 * (at your option) any later version. 00017 * 00018 * This program is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00021 * GNU General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU General Public License along 00024 * with this program; if not, write to the Free Software Foundation, Inc., 00025 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00026 */ 00027 /* 00028 * This set of compile-time defines and run-time variables can be used to 00029 * determine the version number of the PolarSSL library used. 00030 */ 00031 #ifndef POLARSSL_VERSION_H 00032 #define POLARSSL_VERSION_H 00033 00034 #if !defined(POLARSSL_CONFIG_FILE) 00035 #include "config.h" 00036 #else 00037 #include POLARSSL_CONFIG_FILE 00038 #endif 00039 00040 /** 00041 * The version number x.y.z is split into three parts. 00042 * Major, Minor, Patchlevel 00043 */ 00044 #define POLARSSL_VERSION_MAJOR 1 00045 #define POLARSSL_VERSION_MINOR 3 00046 #define POLARSSL_VERSION_PATCH 7 00047 00048 /** 00049 * The single version number has the following structure: 00050 * MMNNPP00 00051 * Major version | Minor version | Patch version 00052 */ 00053 #define POLARSSL_VERSION_NUMBER 0x01030700 00054 #define POLARSSL_VERSION_STRING "1.3.7" 00055 #define POLARSSL_VERSION_STRING_FULL "PolarSSL 1.3.7" 00056 00057 #if defined(POLARSSL_VERSION_C) 00058 00059 #ifdef __cplusplus 00060 extern "C" { 00061 #endif 00062 00063 /** 00064 * Get the version number. 00065 * 00066 * \return The constructed version number in the format 00067 * MMNNPP00 (Major, Minor, Patch). 00068 */ 00069 unsigned int version_get_number( void ); 00070 00071 /** 00072 * Get the version string ("x.y.z"). 00073 * 00074 * \param string The string that will receive the value. 00075 * (Should be at least 9 bytes in size) 00076 */ 00077 void version_get_string( char *string ); 00078 00079 /** 00080 * Get the full version string ("PolarSSL x.y.z"). 00081 * 00082 * \param string The string that will receive the value. The PolarSSL version 00083 * string will use 18 bytes AT MOST including a terminating 00084 * null byte. 00085 * (So the buffer should be at least 18 bytes to receive this 00086 * version string). 00087 */ 00088 void version_get_string_full( char *string ); 00089 00090 /** 00091 * \brief Check if support for a feature was compiled into this 00092 * PolarSSL binary. This allows you to see at runtime if the 00093 * library was for instance compiled with or without 00094 * Multi-threading support. 00095 * 00096 * Note: only checks against defines in the sections "System 00097 * support", "PolarSSL modules" and "PolarSSL feature 00098 * support" in config.h 00099 * 00100 * \param feature The string for the define to check (e.g. "POLARSSL_AES_C") 00101 * 00102 * \return 0 if the feature is present, -1 if the feature is not 00103 * present and -2 if support for feature checking as a whole 00104 * was not compiled in. 00105 */ 00106 int version_check_feature( const char *feature ); 00107 00108 #ifdef __cplusplus 00109 } 00110 #endif 00111 00112 #endif /* POLARSSL_VERSION_C */ 00113 00114 #endif /* version.h */ 00115 00116
Generated on Tue Jul 12 2022 19:40:21 by
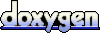